In PowerShell, "meaning" embodies the intent and functionality behind commands, enabling users to execute complex tasks efficiently with simplicity and clarity.
Write-Host 'Hello, World!'
Understanding PowerShell Commands
What Are Cmdlets?
Cmdlets are fundamental building blocks in PowerShell. They are lightweight, single-function commands that follow a specific verb-noun naming convention. This structure enhances readability and understanding.
For example, the cmdlet `Get-Process` retrieves a list of all processes currently running on the system:
Get-Process
When you execute this command, PowerShell communicates with the system to gather information about each process as an object, making it easy to manipulate or filter as needed.
The Structure of PowerShell Commands
To effectively utilize PowerShell, it’s essential to understand the structure of its commands. Each command typically consists of three parts:
- Verb: Describes the action you want to perform (e.g., `Get`, `Set`, `Remove`).
- Noun: Specifies the object you want to act upon (e.g., `Service`, `Process`, `User`).
- Parameters: Additional options that refine the command's action.
As an example, consider the command:
Get-Service -Name "wuauserv"
Here, `Get` is the verb, `Service` is the noun, and `-Name "wuauserv"` is a parameter that filters the output to a specific service. Understanding this structure is key to mastering the meaning in PowerShell.
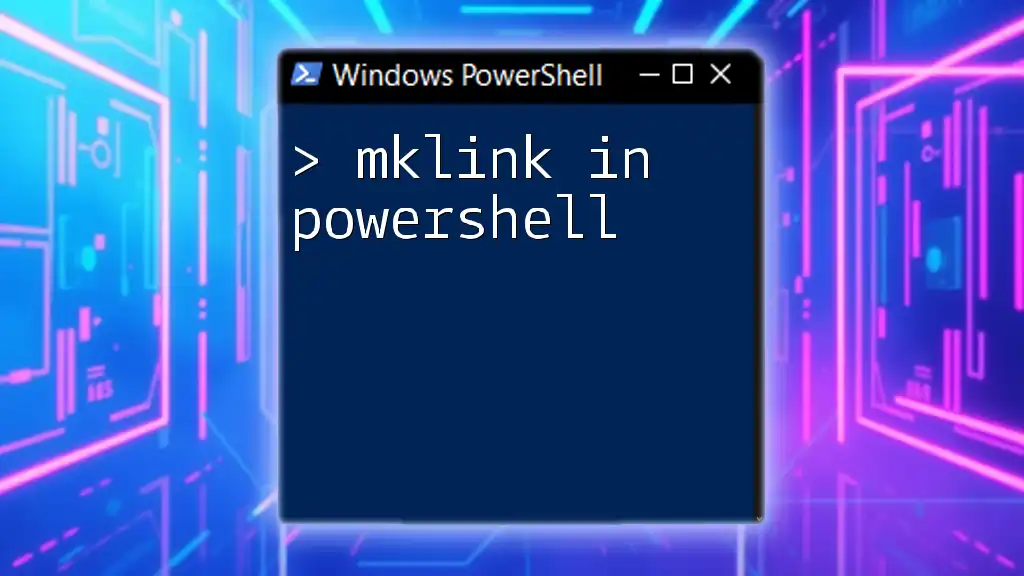
PowerShell Objects and Pipes
Understanding Objects in PowerShell
One of PowerShell’s powerful features is its use of objects. Unlike traditional command-line interfaces that rely heavily on text output, PowerShell represents data as objects, which contain properties and methods.
For instance, when you retrieve services using the `Get-Service` cmdlet:
$service = Get-Service
Each service is represented as an object, and you can access its properties—like `Status` and `DisplayName`—easily. This object-oriented approach provides a rich and powerful way to handle data.
Using Pipes to Pass Objects
The pipe (`|`) operator is one of PowerShell's most significant features, enabling you to pass the output of one command directly as input to another. This allows for powerful command chaining and data manipulation.
For example, if you want to filter running services, you can combine the `Get-Service` cmdlet with `Where-Object`:
Get-Service | Where-Object {$_.Status -eq "Running"}
In this pipeline, `Get-Service` retrieves all services, and `Where-Object` filters that list by checking the `Status` property of each service object. Mastering pipes is crucial for executing complex tasks efficiently, embodying the meaning in PowerShell.
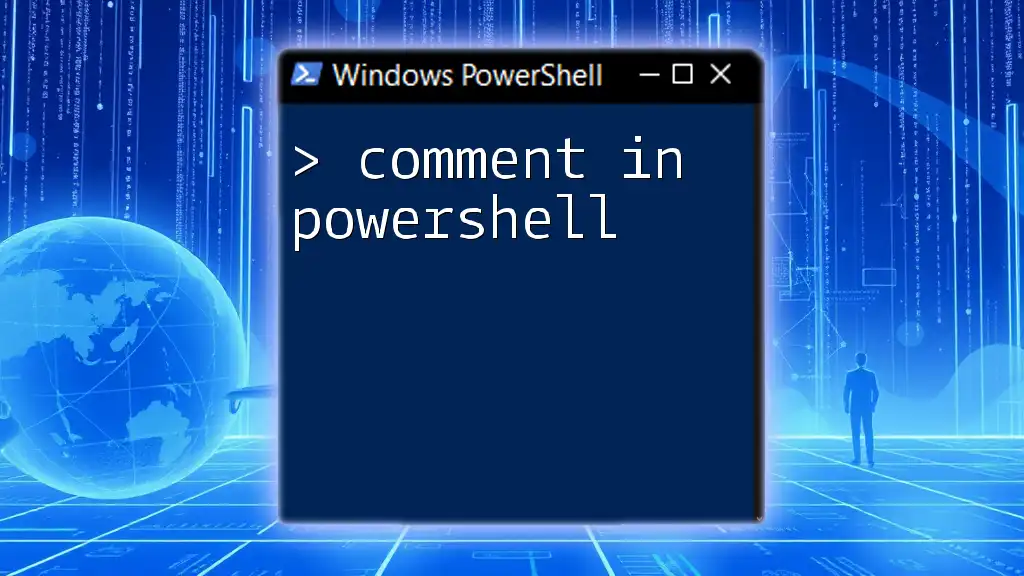
Variables and Their Meanings
Creating and Using Variables
Variables in PowerShell are used to store data that you can manipulate later. They are denoted by the `$` symbol, followed by the variable name.
For example, to assign a string to a variable:
$myVar = "Hello, PowerShell!"
You can then use `$myVar` in future commands, making it a flexible way to manage data efficiently.
Scope of Variables
Understanding variable scope is essential for effective scripting in PowerShell. Variables can exist in different scopes, such as global, script, and local.
Here’s how you can declare a global variable:
$global:myGlobalVar = "Global Variable"
Variables with different scopes behave differently; for instance, a global variable is accessible throughout your entire PowerShell session, while a local variable is limited to the script or function in which it was defined. The scope you choose influences how data flows within your scripts, adding depth to the meaning in PowerShell.
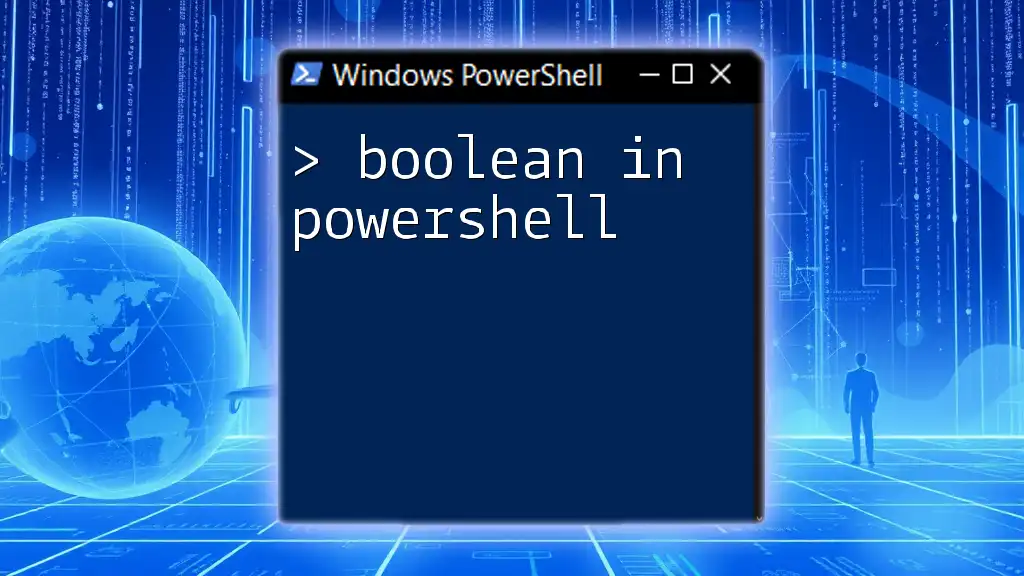
Functions and Their Significance
Defining Functions in PowerShell
Functions allow you to encapsulate code for reuse, making your scripts more organized and efficient. In PowerShell, you can define a function as follows:
function Get-Greeting {
param($name)
"Hello, $name!"
}
Now, calling `Get-Greeting 'Alice'` will output "Hello, Alice!". Functions help you abstract repetitive tasks and simplify complex scripts.
Function Parameters and Return Values
Parameters enable functions to be dynamic and adaptable. You can define parameters as mandatory or optional based on your needs. For instance:
function Get-Sum {
param($a, $b)
return $a + $b
}
This function takes two numbers as input and returns their sum. Understanding how to utilize parameters and return values effectively enhances the meaning in PowerShell, allowing you to create modular and reusable code.
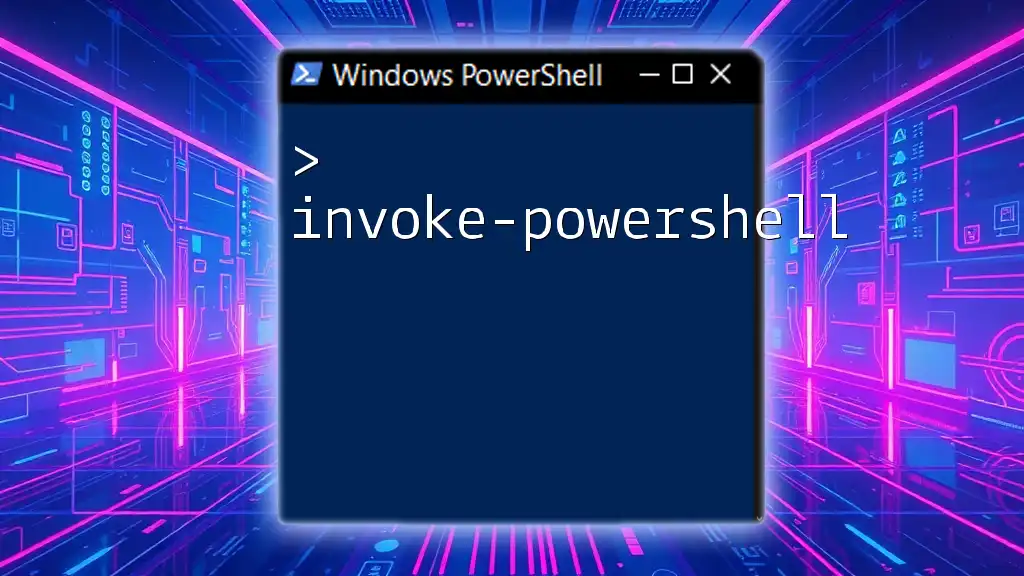
Error Handling in PowerShell
Understanding Error Types
In any scripting environment, handling errors is vital for creating robust scripts. PowerShell differentiates between terminating errors, which stop execution, and non-terminating errors, which allow the script to continue. Recognizing these error types helps you manage your scripts more gracefully.
Using Try/Catch for Error Handling
To handle potential errors, PowerShell employs structured error handling using the `try` and `catch` blocks. Here’s a simple example:
try {
Get-Content "nonexistentfile.txt"
} catch {
Write-Host "An error occurred: $_"
}
In this snippet, if `Get-Content` fails due to the file not existing, the error is captured and handled without halting the script. This capability empowers you to build more resilient scripts and understand the meaning in PowerShell.
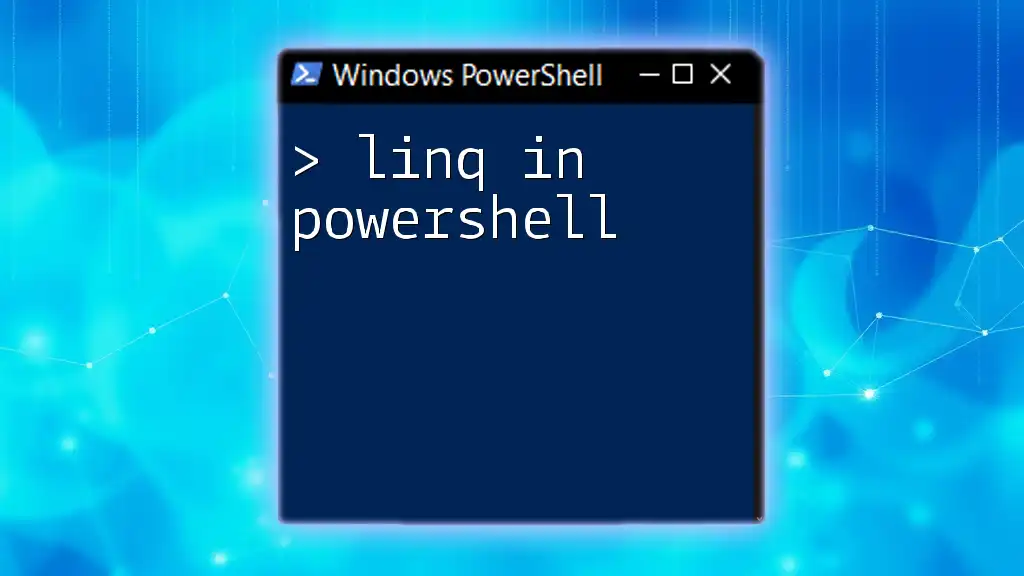
Conclusion
Understanding the meaning in PowerShell is about grasping its foundational elements like cmdlets, objects, variables, functions, and error handling. Each aspect contributes to the overall power and versatility of PowerShell, enabling users to automate tasks efficiently and effectively.
By mastering these concepts, you gain the ability to craft scripts that not only meet immediate needs but also evolve with your increasing comfort and familiarity with the language. Remember, the key to success in PowerShell lies in consistent practice and a commitment to understanding its deeper meanings.