In PowerShell, the `Length` property is used to determine the number of characters in a string or the number of elements in an array.
Here's a quick example to illustrate the use of `Length` with a string:
$string = "Hello, World!"
$length = $string.Length
Write-Host "The length of the string is: $length"
What is Length in PowerShell?
In PowerShell, length refers to the measurement of how many elements are contained within a data type, be it a string, an array, or a collection. Understanding the concept of length is essential for efficient scripting, as it allows you to manipulate data, validate inputs, and perform various operations more effectively.
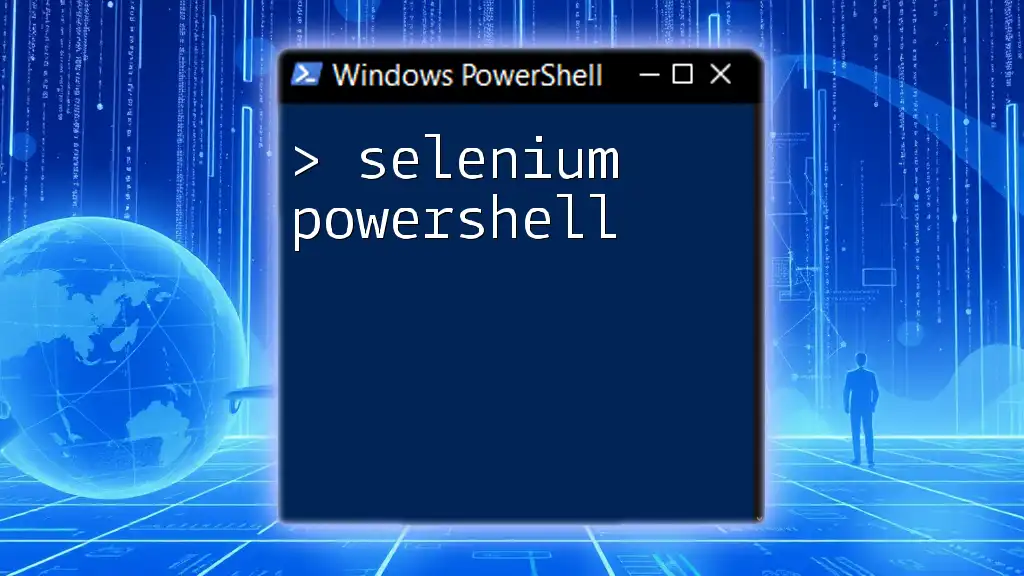
What is the `Len` Function?
The `Len` function is a built-in function in PowerShell that retrieves the length of a string. It serves as a straightforward way to determine how many characters are in a string. This function can be applied when validating user input, checking for string limits, or performing data processing.
Basic Syntax of the `Len` Function
The syntax is very simple:
Len(string)
This function takes a string as an argument and returns its length.
Common Use Cases for `Len`
The `Len` function is commonly used in scenarios like:
- Input validation for user entries.
- Checking text for formatting.
- Determining if a string meets length requirements for processing.
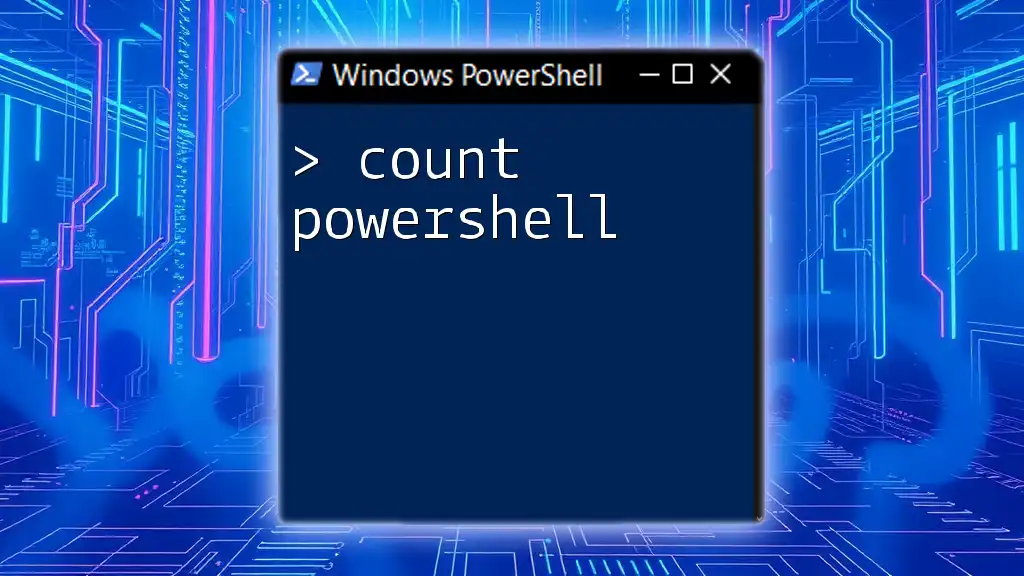
Using Length with Different Data Types
Strings
To find the length of a string, you can use both the `Len` function and the `.Length` property. Each provides the same result but differs slightly in syntax.
Example Code Snippet:
$string = "Hello PowerShell"
$lengthUsingLen = Len($string)
$lengthUsingProperty = $string.Length
Write-Output "Length using Len: $lengthUsingLen"
Write-Output "Length using .Length property: $lengthUsingProperty"
In this example, both methods return the length of the string "Hello PowerShell" as 16.
Arrays
To determine the length of an array, PowerShell provides the `.Length` property, which returns the number of items contained within the array.
Example Code Snippet:
$array = 1, 2, 3, 4, 5
$length = $array.Length
Write-Output "Array Length: $length"
Here, the output will indicate that the array has a length of 5.
Collections
PowerShell also allows you to check the length of various collections like `ArrayList` or `HashTable`.
Example Code Snippet for a HashTable:
$hashTable = @{"One"=1; "Two"=2}
$length = $hashTable.Count
Write-Output "HashTable Length: $length"
In this case, the output shows that the HashTable contains 2 key-value pairs.
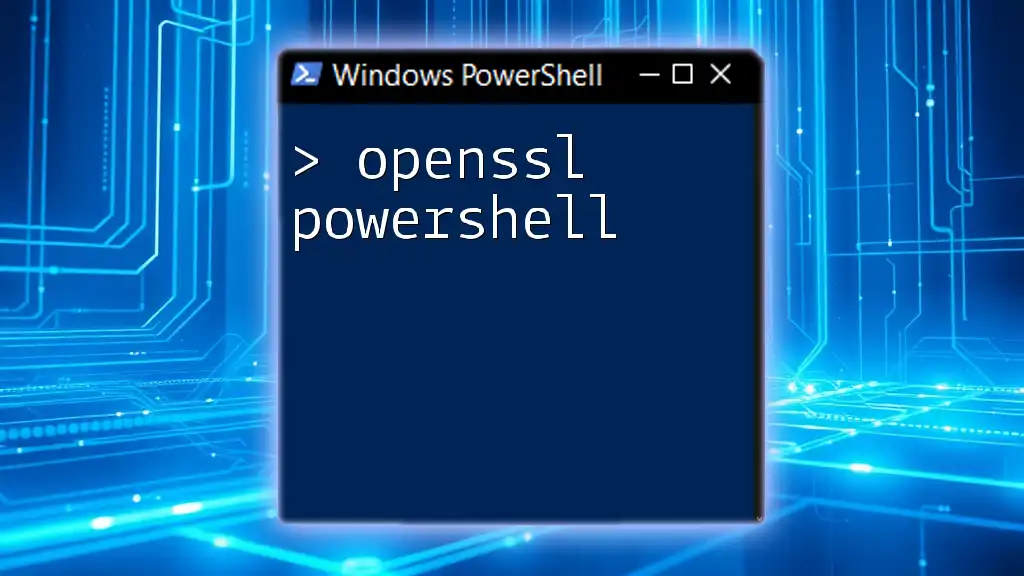
Advanced Usage of Length in PowerShell
Using Length in Conditional Statements
Understanding the length of strings or collections can be integral to your script logic. For instance, you can implement length checks directly in conditional statements.
Example Code Snippet:
if ($string.Length -gt 10) {
Write-Output "String is longer than 10 characters."
}
This snippet will execute a specific action only if the string exceeds 10 characters in length.
Filtering Data Based on Length
You can also utilize length to filter data based on certain criteria. This can enhance the functionality of your scripts by enabling targeted operations.
Example Code Snippet:
$filteredStrings = $array | Where-Object { $_.Length -gt 3 }
Write-Output "Filtered Strings: $filteredStrings"
In this example, you'll filter out any elements in the `$array` with length greater than 3, which can be invaluable when working with larger datasets.
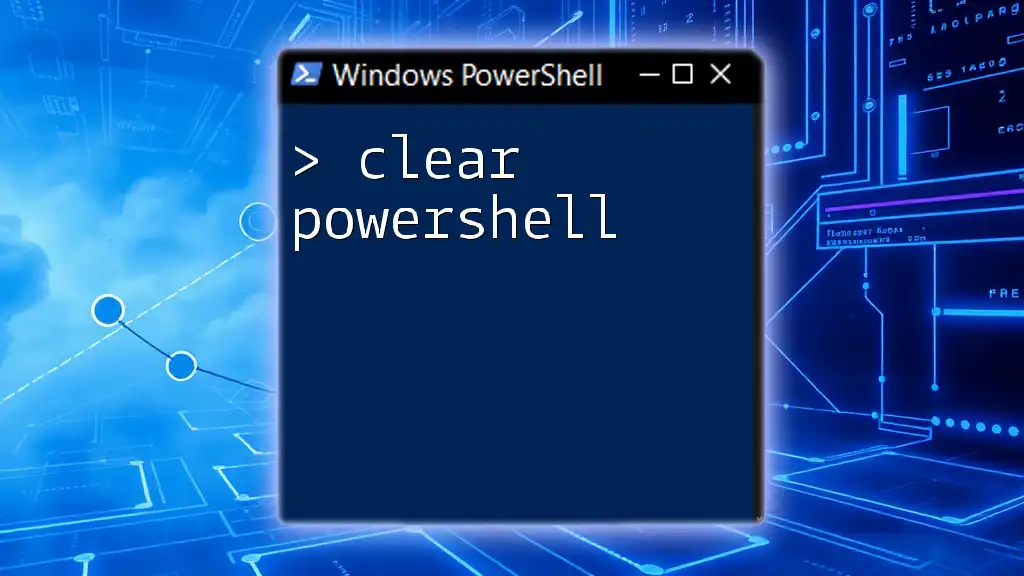
Practical Applications of Length in PowerShell
Validating Input Length
Validating the length of user inputs is essential for security and integrity within your scripts. Ensuring that inputs meet specific length requirements can help avoid errors down the line.
Example Code Snippet:
$userInput = Read-Host "Enter your name"
if ($userInput.Length -lt 5) {
Write-Output "Name must be at least 5 characters."
}
This code checks if the input length is less than five characters, providing immediate feedback and validation.
Parsing Data Files
Length can also aid in parsing data files where consistency and validity of data entries are vital.
Example Code Snippet:
$csvData = Import-Csv "data.csv"
foreach ($row in $csvData) {
if ($row.FieldName.Length -gt 10) {
Write-Output "Field exceeds length limit: $($row.FieldName)"
}
}
This use case can highlight fields in your data that exceed a specified length, allowing for more controlled data management.
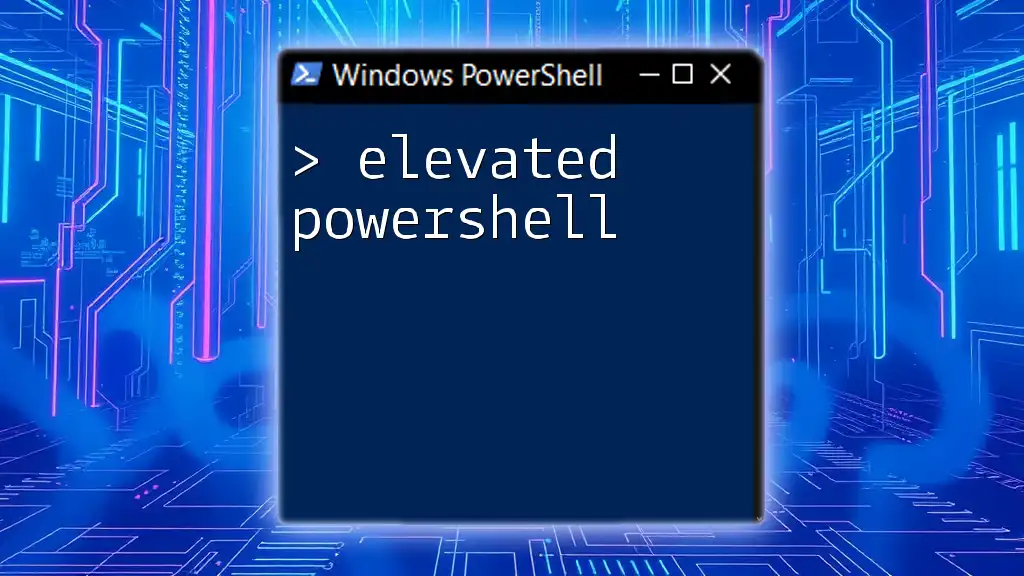
Summary of Key Points
Understanding length in PowerShell is key to developing efficient scripts. Whether you’re working with strings, arrays, or other collections, knowing how to determine and utilize length can greatly enhance your coding capabilities.
Feel encouraged to practice using length in your own scripts to fully grasp its capabilities.
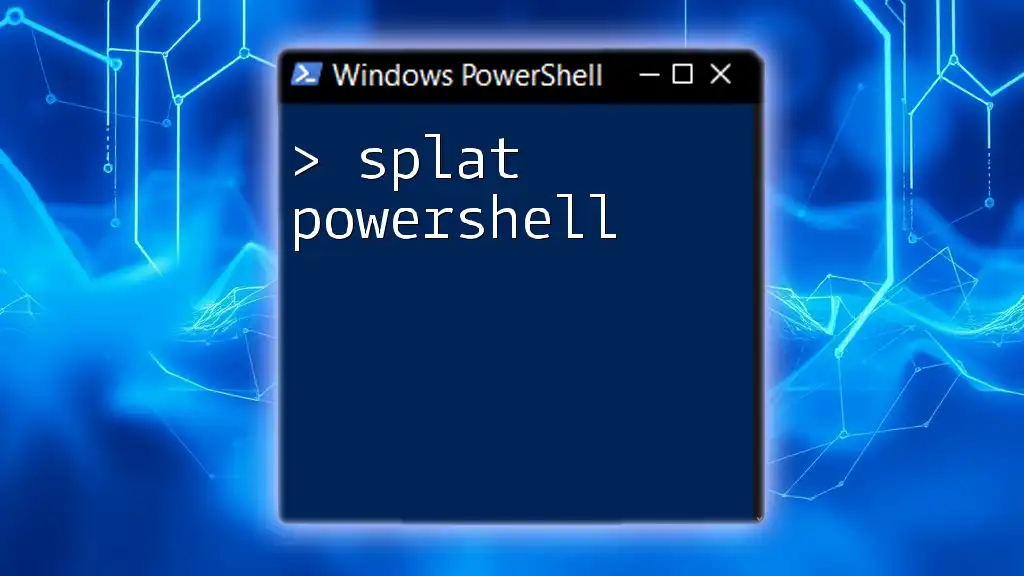
FAQs About Length in PowerShell
What is the difference between Length and Count?
In PowerShell, Length is used primarily with strings and arrays to denote the number of elements or characters, while Count is used with collections such as HashTables to indicate the number of key-value pairs.
Can Length be used with custom objects?
Yes, you can determine the length of properties of custom objects, provided those properties are of type string or array.
Why does length matter in scripting?
Length matters because it enhances the reliability and performance of your scripts by preventing errors associated with unexpected input sizes and enabling better data handling. Understanding how to implement length checks creates more robust and foolproof code.