In PowerShell, you can count the number of items in an array or collection using the `Count` property or the `Measure-Object` cmdlet.
Here's a code snippet to demonstrate both methods:
# Method 1: Using the Count property
$array = 1..10
$numberOfItems = $array.Count
Write-Host "Number of items in the array: $numberOfItems"
# Method 2: Using Measure-Object cmdlet
$numberOfItems2 = $array | Measure-Object | Select-Object -ExpandProperty Count
Write-Host "Number of items in the array using Measure-Object: $numberOfItems2"
Understanding Counting in PowerShell
What Does "Count" Mean in PowerShell?
In PowerShell, counting refers to the process of determining the number of objects within a collection, array, or pipeline output. This fundamental concept is essential for users who want to analyze data, automate tasks, and create efficient scripts. By counting objects, you can gather valuable insights about data sets, make informed decisions, and streamline your automation processes.
The Count Property
Every collection in PowerShell has a Count property, which allows you to quickly find out how many items are present. This property is particularly useful when working with arrays or lists.
For instance, consider an array of numbers:
$array = @(1, 2, 3, 4, 5)
$array.Count
This code snippet will output `5`, indicating that there are five items in the `$array`. Utilizing the Count property is a straightforward way to gauge the size of your data set and ensures that you can manage it effectively.
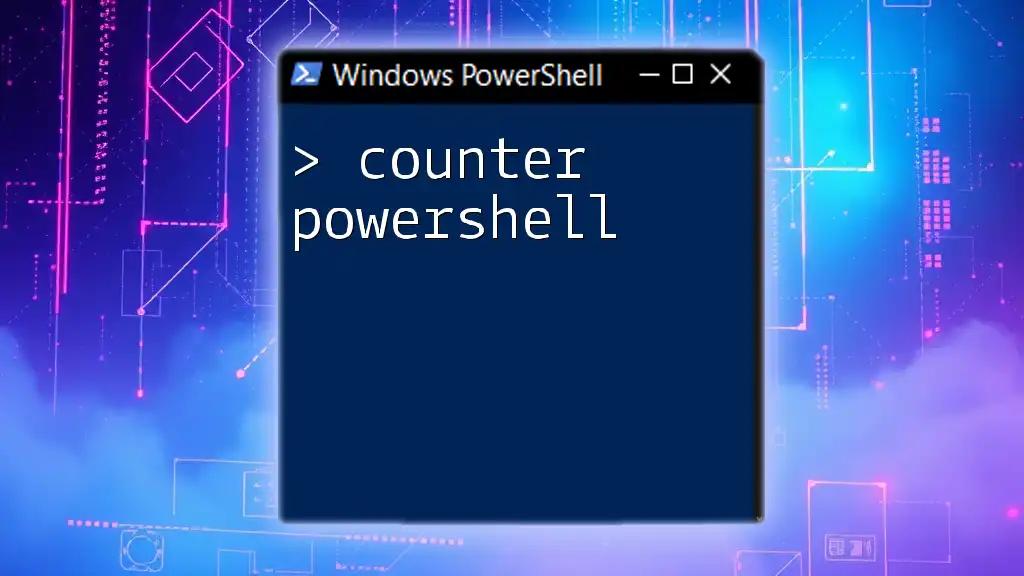
Counting Objects in PowerShell
Using Measure-Object
One of the most powerful cmdlets for counting objects in PowerShell is Measure-Object. This cmdlet can analyze different types of input objects and gather statistical information, including counts, sums, averages, and more.
Here's an example of using `Measure-Object` to count the total number of processes currently running on your system:
Get-Process | Measure-Object
When this command is executed, you'll receive an output indicating the total number of processes, along with other statistical information. Measure-Object is versatile, allowing you to count not only processes but also any other type of objects that can be enumerated.
Counting Specific Attributes
In addition to counting all objects, PowerShell allows you to use `Measure-Object` to count specific attributes, such as the number of files with a particular extension.
Consider this example where we want to count all `.txt` files in a specific directory:
Get-ChildItem -Path "C:\Path\To\Directory" -Filter "*.txt" | Measure-Object
In this case, `Get-ChildItem` retrieves a list of files in the specified directory that match the `.txt` filter. When passed to `Measure-Object`, it returns the count of `.txt` files, giving you an efficient way to analyze file types.
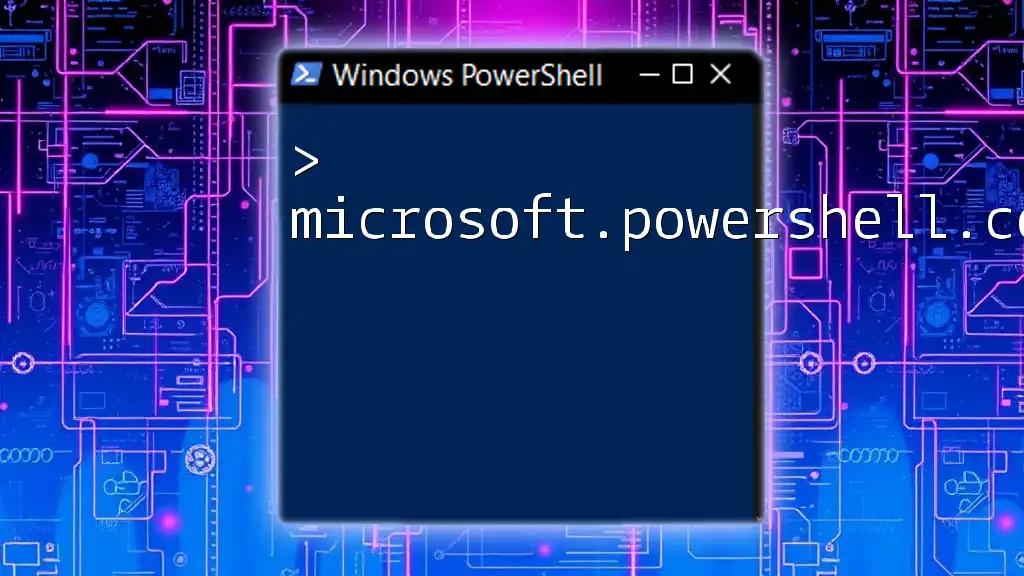
Advanced Counting Techniques
Using the Group-Object Cmdlet
For more advanced counting, Group-Object is an excellent cmdlet that groups objects based on a specified property. This grouping can quickly provide counts of occurrences for each distinct value in a dataset.
For example, you may want to count the number of different logon event types in the Windows Security Event Log:
Get-EventLog -LogName Security | Group-Object -Property EventID
This command retrieves entries from the Security log, groups them by EventID, and provides a count of how many times each event occurred, enabling deeper insights into user activity and system events.
Filtering with Where-Object
PowerShell’s ability to filter objects allows you to count based on specific conditions. This is accomplished with the Where-Object cmdlet, which can filter results before passing them to `Measure-Object`.
As an example, if you wanted to count processes consuming more than 100MB of memory, you could use the following command:
Get-Process | Where-Object { $_.WorkingSet -gt 100MB } | Measure-Object
In this example, `Where-Object` filters the results of `Get-Process`, allowing only those processes with a working set size greater than 100MB to be counted. This is a powerful way to monitor resource usage on your system and take action based on the results.
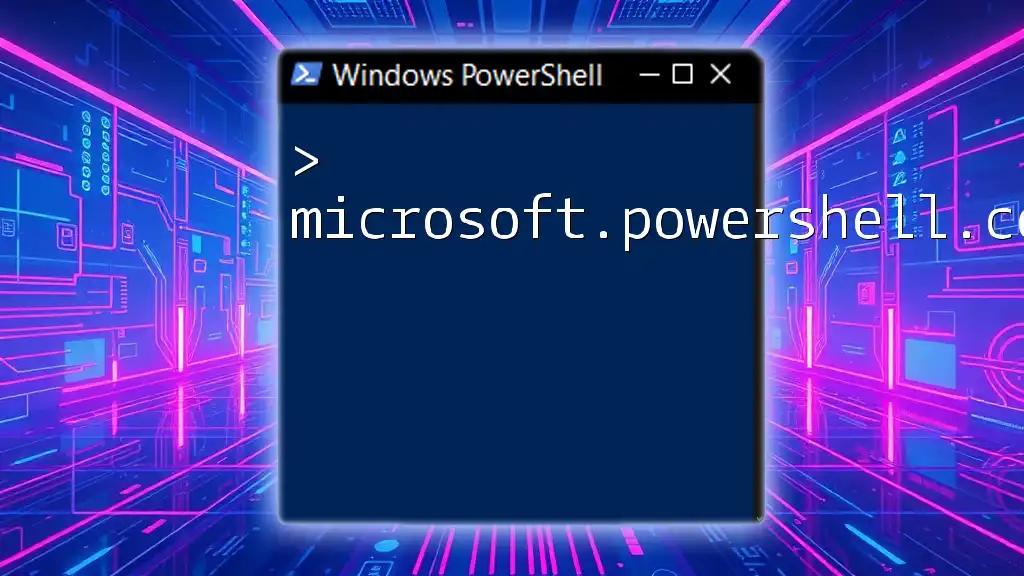
Tips for Effective Counting in PowerShell
Optimize Your Scripts
When writing PowerShell scripts that involve counting, it’s important to keep performance in mind. Using cmdlets like Measure-Object and Group-Object can optimize your scripts since they are specifically designed to handle large data sets efficiently. Additionally, avoid unnecessary processing of data by filtering results early in the pipeline.
Common Errors and Troubleshooting
When working with counting objects, be aware of common errors. For instance, if you attempt to use `Count` on a pipeline output without storing it in a variable first, you may receive unexpected results.
For effective troubleshooting:
- Check Data Types: Ensure the objects you are counting are compatible with the cmdlets you are using.
- Validate Inputs: Always validate any input data to avoid runtime errors and ensure your commands operate smoothly.
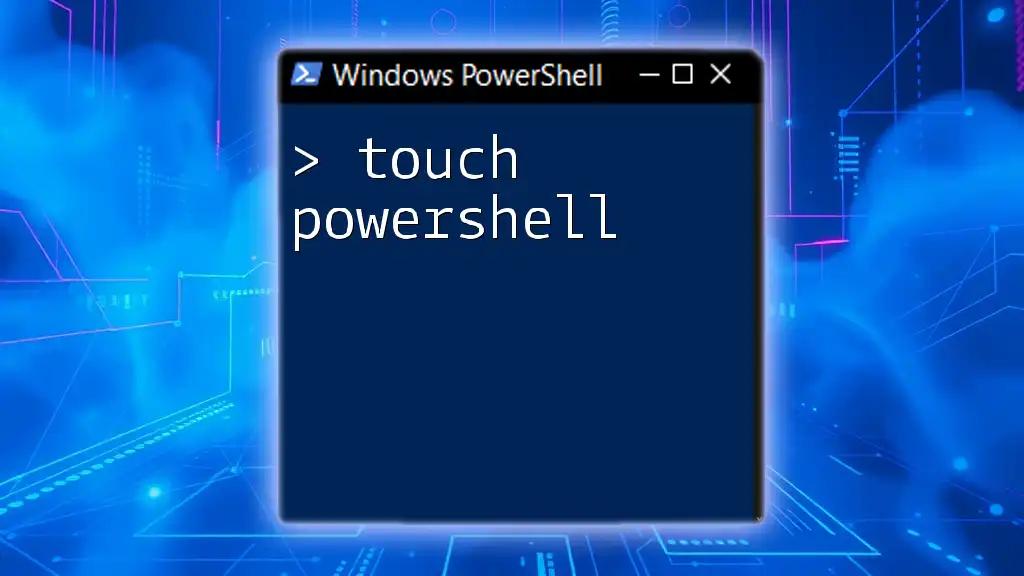
Summary
In this article, we've delved into the various methods and techniques for counting objects in PowerShell. Understanding how to effectively use the `Count` property, `Measure-Object`, and `Group-Object` cmdlets, along with filtering capabilities, empowers you to gather insights and automate tasks in a streamlined manner. By applying these techniques, you can enhance your PowerShell scripting capabilities and optimize your workflows.
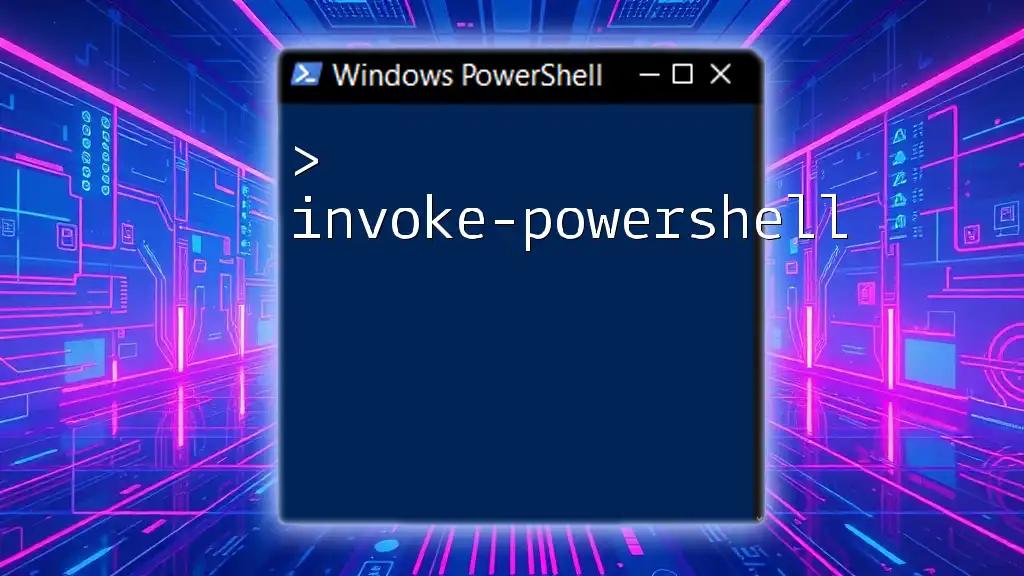
Call to Action
Now that you have a comprehensive understanding of counting in PowerShell, I encourage you to practice these commands and techniques in your own scripts. Experiment with different object types and attributes to gain a deeper understanding of their applications. Don’t forget to subscribe for more PowerShell tips and tutorials to take your skills to the next level!
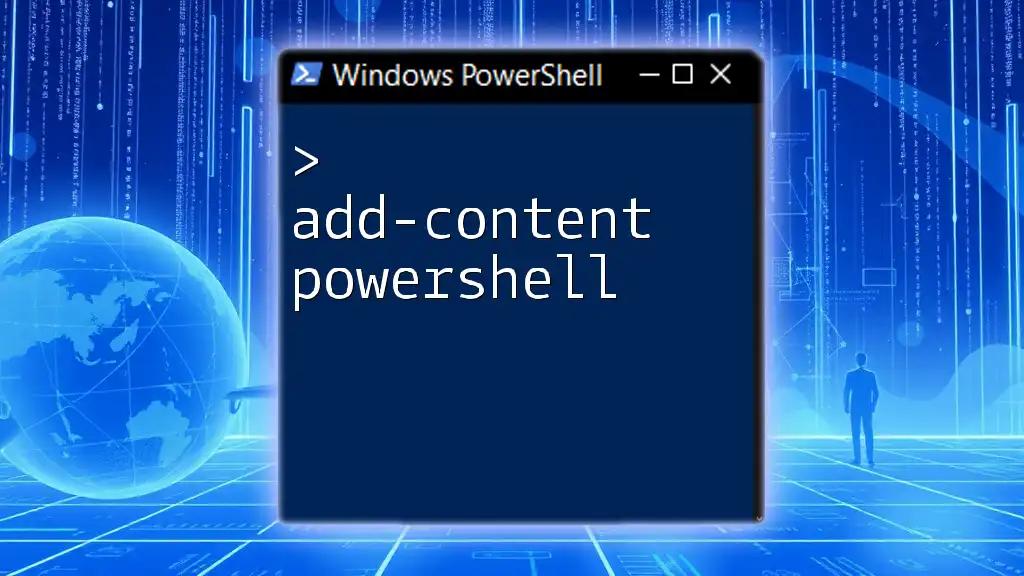
Further Reading and Resources
For those interested in deepening their knowledge of PowerShell, consider exploring the official Microsoft documentation, reputable PowerShell books, and engaging with online communities and forums. These resources can provide you with additional insights and support as you refine your PowerShell expertise.