Splatting in PowerShell allows you to pass a collection of parameters to a command as a hashtable, simplifying the syntax and enhancing readability.
Here's a code snippet demonstrating splatting:
$params = @{
Name = 'Hello, World!'
Count = 5
}
Write-Host @params
What is PowerShell Splatting?
Splatting is a powerful feature in PowerShell that allows you to pass parameters to functions or cmdlets in a more manageable and organized way. Instead of providing parameters inline, you can use a hash table or an array to package your parameters together, making your commands cleaner and easier to read.
Benefits of Using Splatting in PowerShell Scripts
- Readability: Splatting can greatly enhance the readability of your scripts, especially when dealing with a command that has many parameters.
- Maintainability: When you need to adjust parameters, it’s simpler to modify values in a hash table instead of rewriting the entire command.
- Efficiency: Splatting allows for a quicker input of parameters, reducing the chance of errors associated with manual parameter entry.
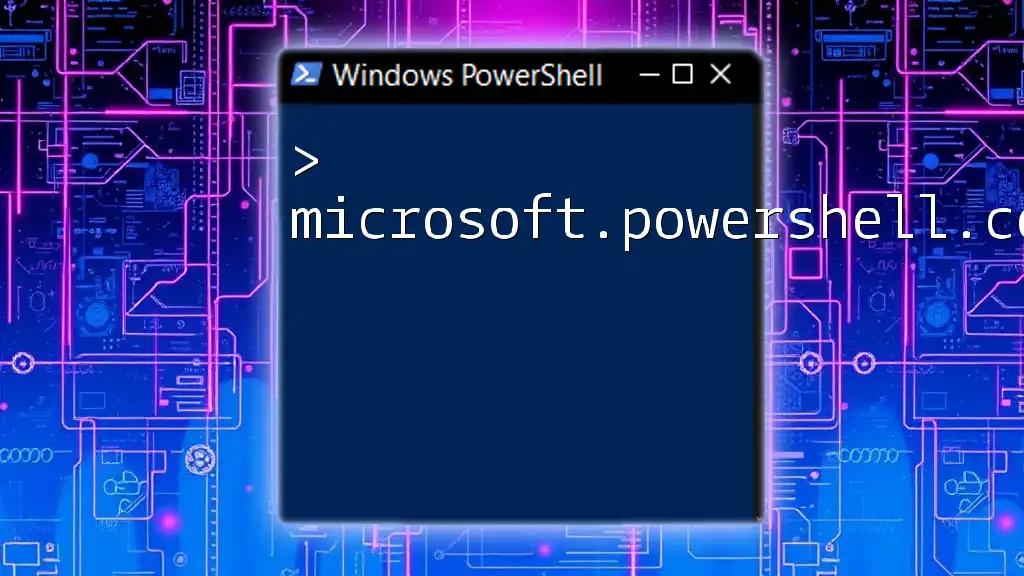
Understanding PowerShell Parameters
Defining Parameters in PowerShell
Parameters are variables defined in cmdlets or functions that specify inputs for those commands. PowerShell supports both positional and named parameters.
- Positional Parameters: These are identified by their position in the command. For example, `Get-Process -Name "explorer"` assigns "explorer" to the -Name parameter.
- Named Parameters: Although less flexible regarding order, named parameters lead to clearer code, as you specify which parameter you are assigning a value to directly.
How Splatting Relates to Parameters
Splatting simplifies the passing of parameters to cmdlets and functions. Instead of listing parameters individually, you can use a hash table to group them together. This means that with a single command, you can provide multiple parameters conveniently.

The Basics of Splatting in PowerShell
Using Hash Tables for Splatting
A hash table in PowerShell is a collection of key-value pairs. Each key represents a parameter name, while the corresponding value is assigned to that parameter.
Example of Using a Hash Table for Splatting
$params = @{
Name = "John Doe"
Age = 30
City = "New York"
}
New-Object -TypeName PSObject -Property $params
In this example, `$params` is a hash table that includes keys `Name`, `Age`, and `City` with their corresponding values. When you invoke `New-Object`, the `-Property` parameter can simply take `$params`, allowing for cleaner syntax and better organization.
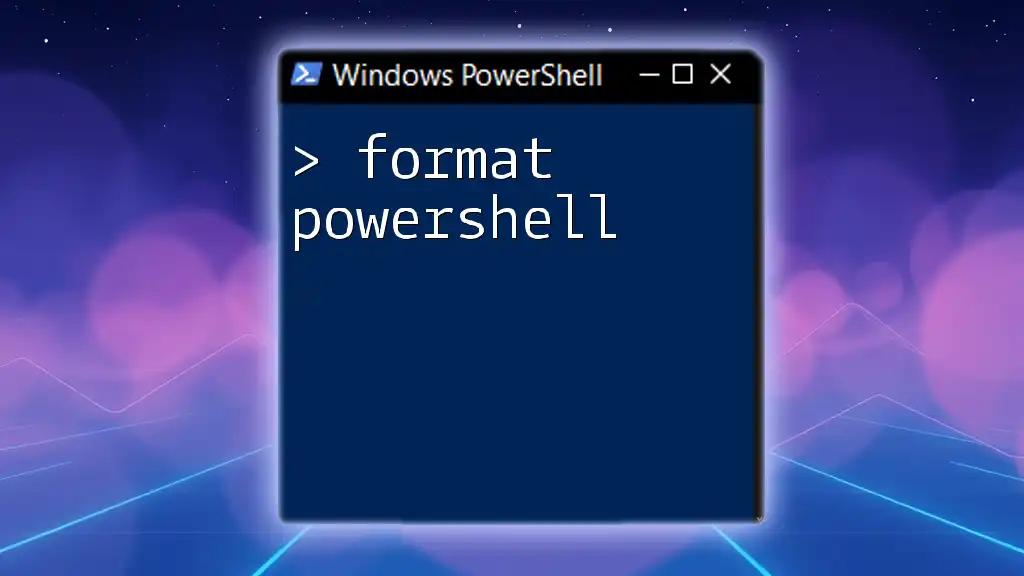
Implementing Splatting in Your Scripts
Creating and Using a Hash Table for Splat
To utilize splatting, you first create your hash table with the designated parameters. Here's a concise guide:
- Define the hash table using `@{}`.
- Specify each parameter as a key-value pair.
- Utilize the hash table with the `@` symbol before the variable.
Example: Splatting with Common Cmdlets
Using `Get-ChildItem`
$pathParams = @{
Path = "C:\Path\To\Directory"
Recurse = $true
Filter = "*.txt"
}
Get-ChildItem @pathParams
In this example, `$pathParams` encapsulates the `Path`, `Recurse`, and `Filter` parameters for the `Get-ChildItem` cmdlet. By using `@pathParams`, you pass all necessary criteria neatly, enhancing clarity and efficiency.
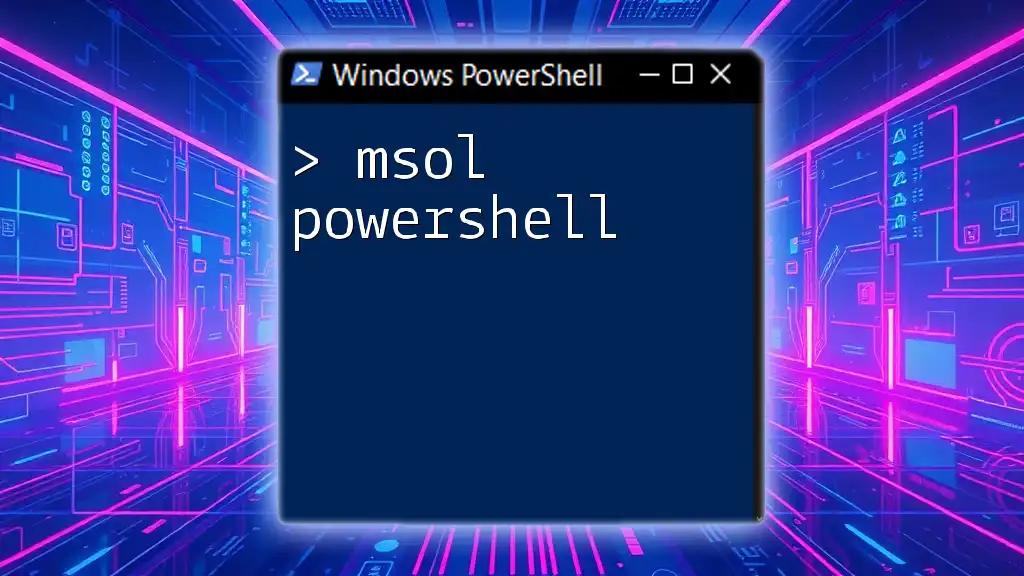
Advanced Splatting Techniques
Using Arrays for Splatting
Similar to hash tables, arrays can also be used for splatting. An array is a collection of items, which can be particularly useful for passing multiple arguments, especially in commands that expect a list or sequence.
Example of Using Arrays with Splatting
$groupParams = @("John Doe", 30)
New-Object -TypeName PSObject -Property @{
Name = $groupParams[0]
Age = $groupParams[1]
}
In this example, `$groupParams` holds an array of values. The individual elements can be accessed by their index, which allows efficient organization and retrieval.
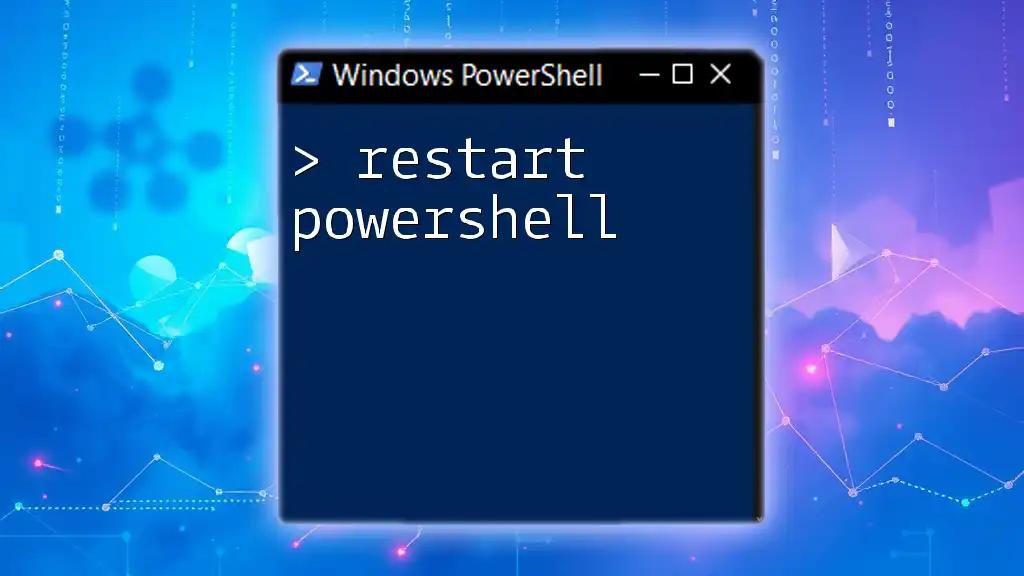
Error Handling with Splatting
Common Errors When Splatting
As with any programming technique, errors can occur with splatting. Users should be aware of the following common issues:
- Misunderstanding Parameter Types: You might pass data types that are incompatible with the expected parameter type.
- Omitting Required Parameters: Some cmdlets require certain parameters. If they're missing in your hash table, PowerShell will throw an error.
Best Practices for Successful Splatting
To decrease the likelihood of errors and improve your PowerShell scripting:
- Use Clear and Meaningful Parameter Names: Descriptive names facilitate understanding later.
- Validate Inputs Before Passing to Commands: Ensuring parameter integrity helps prevent execution errors.
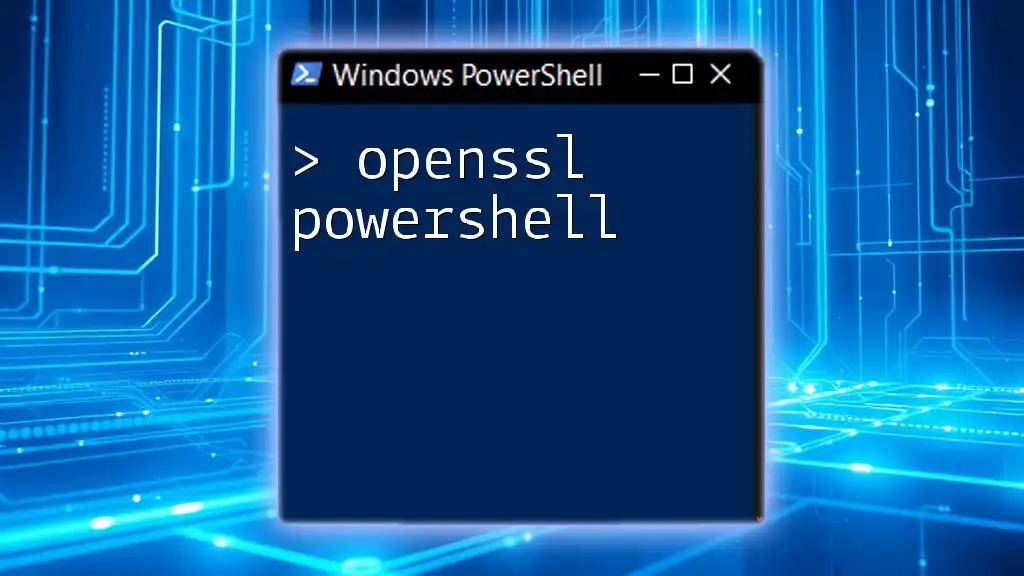
Real-World Applications of Splatting
Splatting is not just a syntactical convenience; it has real-world applications that enhance productivity. For example, automating regular tasks can save time and minimize human error.
Example: Automating System Info Gathering
$systemInfoParams = @{
ComputerName = "localhost"
Credential = (Get-Credential)
}
Get-WmiObject -Class Win32_OperatingSystem @systemInfoParams
In the above, `$systemInfoParams` neatly encapsulates the parameters needed for gathering system information. Using splatting improves the legibility and manageability of the script while effectively querying the Windows Management Instrumentation (WMI).
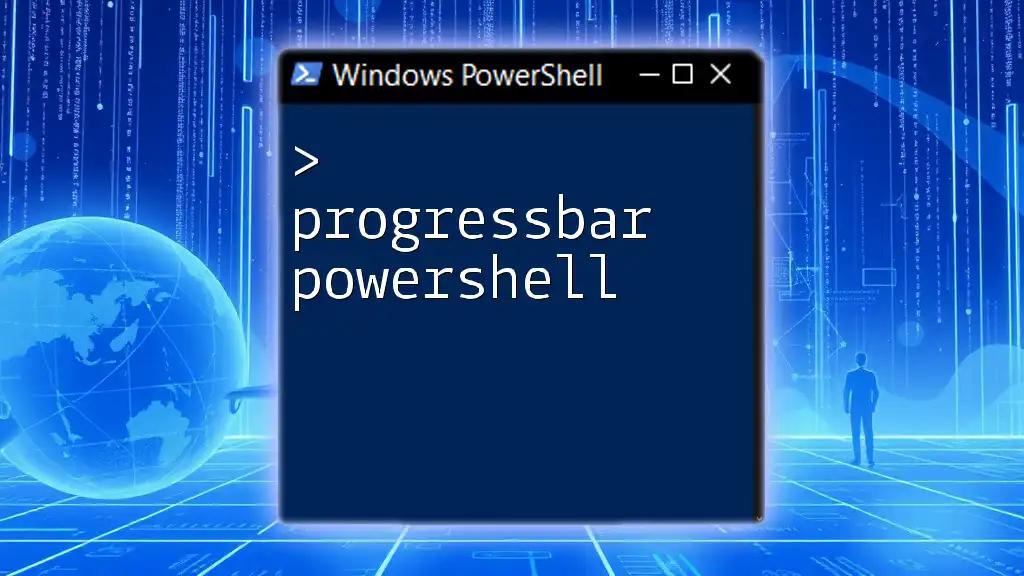
Conclusion
Splatting is an immensely useful feature in PowerShell that not only improves your scripting efficiency but also enhances code readability and maintainability. By allowing the grouping of parameters through either hash tables or arrays, splatting lets you construct cleaner commands and avoids the pitfalls of manual parameter entry.
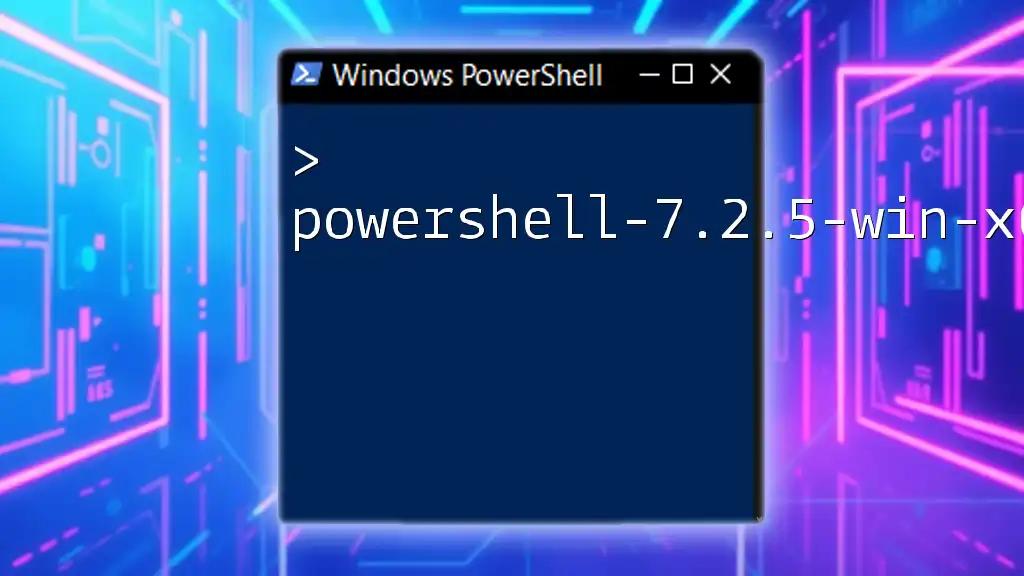
Additional Resources
For those looking to delve deeper into PowerShell and splatting, resources such as online tutorials, community forums, and relevant literature can prove invaluable. Engaging with expert content, practicing regularly, and interacting with other learners will further enhance your PowerShell capabilities.
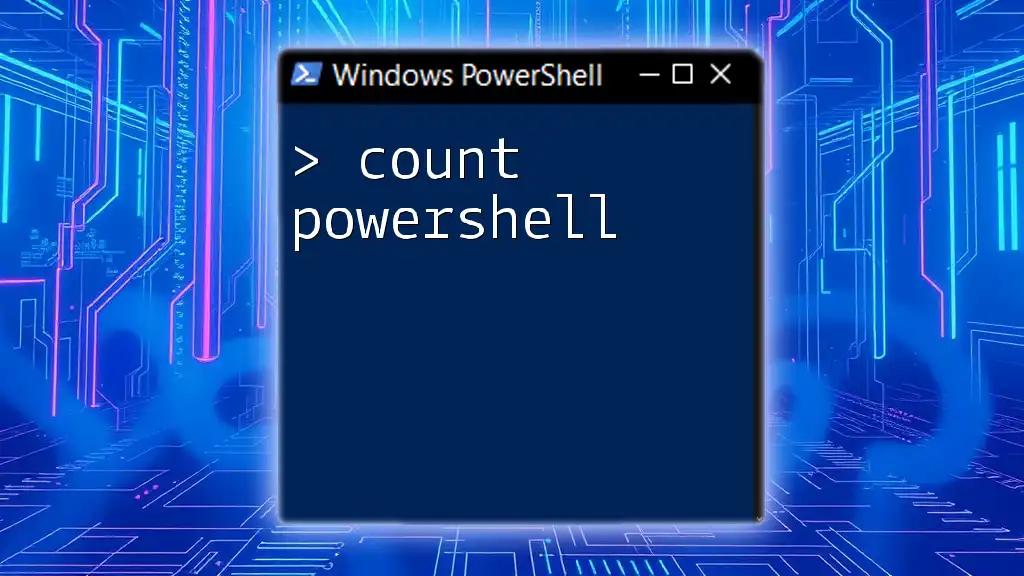
Call to Action
Join our PowerShell learning community today! Subscribe for more tutorials and tips to sharpen your skills and optimize your scripting practices.