In PowerShell, you can create a simple progress bar using the `Write-Progress` cmdlet to visually indicate the progress of a script or task.
Here’s a basic example:
for ($i = 1; $i -le 100; $i++) {
Write-Progress -Status "Processing" -PercentComplete $i
Start-Sleep -Milliseconds 50 # Simulates work being done
}
This code snippet gradually updates the progress bar from 1% to 100% over a short duration.
What is a Progress Bar?
A progress bar is a graphical representation that indicates the progress of a task through a visual cue. In the context of PowerShell, it provides essential feedback during the execution of scripts, especially when those scripts involve lengthy operations. This immediate visual feedback is critical as it keeps users informed about how long they might need to wait for the action to complete, significantly enhancing user experience.
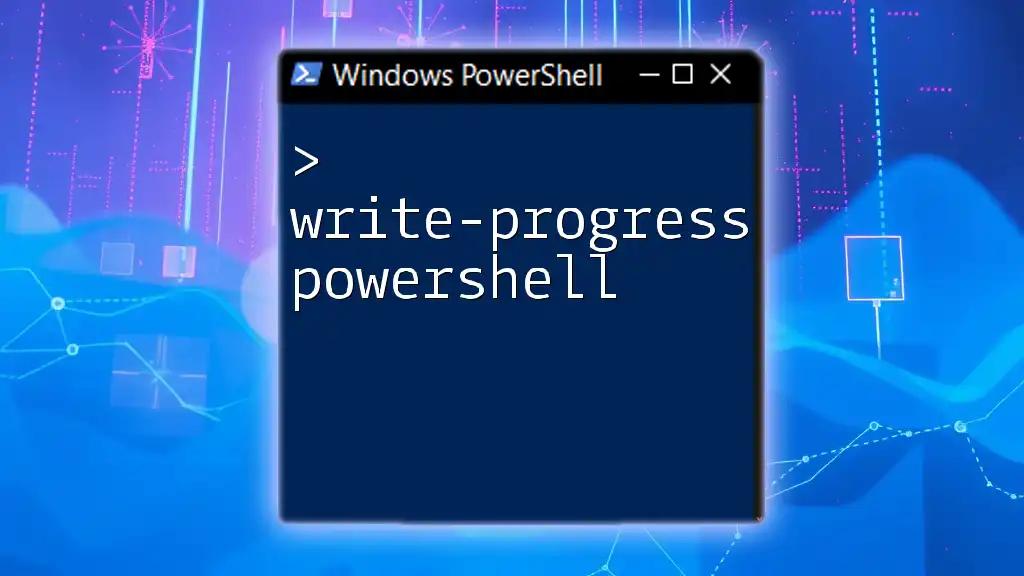
Why Use Progress Bars in PowerShell Scripts?
Using progress bars in your PowerShell scripts is pivotal for several reasons:
- User Engagement: It allows users to see real-time progress, making scripts feel responsive rather than static.
- Error Handling: Progress bars can also aid in identifying where a script may be taking longer than expected, making troubleshooting easier.
- Professionalism: Implementing visual elements like progress bars can make your scripts appear more polished and user-friendly.
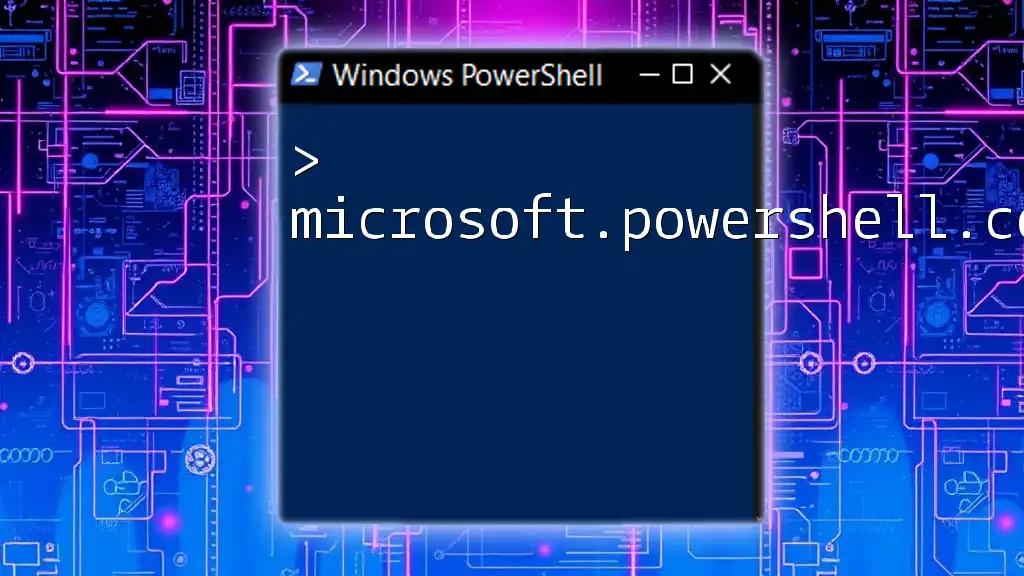
Understanding the Basics of PowerShell Progress Bars
PowerShell Cmdlets for Progress Bars
One of the primary cmdlets used for creating a progress bar in PowerShell is the `Write-Progress` cmdlet. This cmdlet displays the progress of a running task in a console window.
Syntax and Usage
The syntax of `Write-Progress` is straightforward, enabling users to customize the progress message and percentage completion easily. Here’s a basic example:
Write-Progress -Activity "Copying Files" -Status "File 1 of 10" -PercentComplete 10
Key Parameters of the Write-Progress Cmdlet
Understanding the key parameters of `Write-Progress` will allow you to fully utilize its capabilities:
- `-Activity`: A string that describes the overall activity. This should be high-level and provide context for what the user is doing.
- `-Status`: A string that gives a brief description of the current status—this could indicate what file is currently being processed or a similar operation.
- `-PercentComplete`: A numeric value between `0` and `100` that indicates the completion percentage. This is crucial for giving a sense of how close the task is to finishing.
- `-CurrentOperation`: A string that details the current operation being processed. Think of this as a way to present what the user should focus on right now.
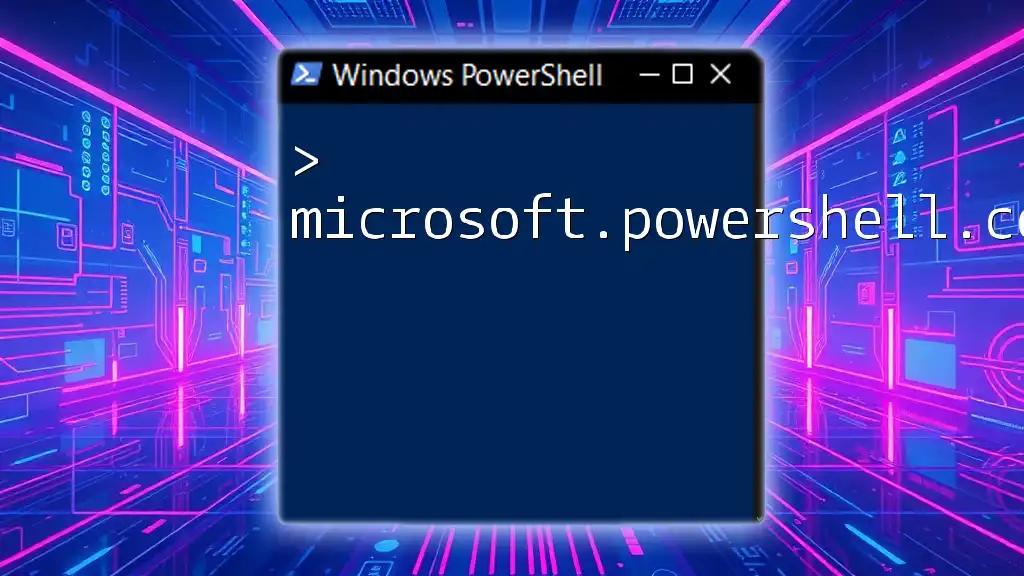
Creating a Simple Progress Bar in PowerShell
Example: A Basic File Copy Script
Let’s walk through creating a basic file copying script that uses a progress bar to inform the user of its progress:
$files = Get-ChildItem "C:\Source"
$totalFiles = $files.Count
for ($i = 0; $i -lt $totalFiles; $i++) {
$file = $files[$i]
Copy-Item $file.FullName "C:\Destination\$($file.Name)"
$percentComplete = [math]::Round((($i + 1) / $totalFiles) * 100)
Write-Progress -Activity "Copying Files" -Status "$($file.Name) copied." -PercentComplete $percentComplete
}
In this script:
- We gather all files from a source directory.
- We then loop through each file, copying it to a destination directory.
- After each copy operation, we calculate the completion percentage and update the progress bar using `Write-Progress`.
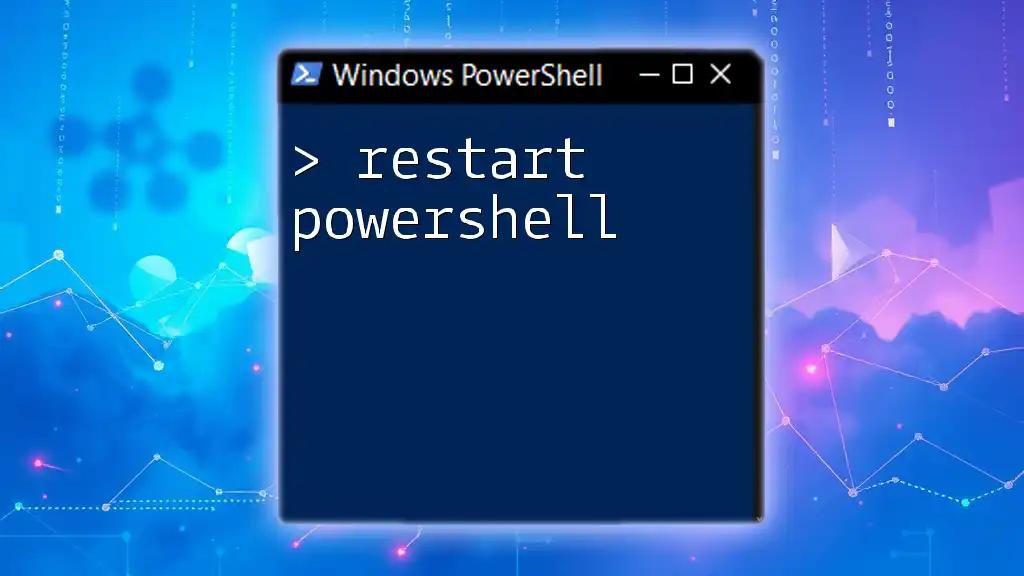
Advanced Progress Bar Features
Customizing the Progress Bar Appearance
PowerShell allows you to customize the appearance of your progress bars, making them more visually appealing. You can change the colors of the progress bar using the `-ForegroundColor` and `-BackgroundColor` parameters.
Write-Progress -Activity "Processing Data" -Status "Working" -PercentComplete 50 -ForegroundColor Green -BackgroundColor Black
Adding Multi-Level Progress Bars
Sometimes, a single progress bar isn’t enough, especially in scripts that include nested tasks. In such cases, you can use multi-level progress bars.
Let’s look at an example where we have multiple main processes, each with its own set of operations:
for ($i = 0; $i -lt 3; $i++) {
Write-Progress -Activity "Main Process" -Status "Step $($i + 1) of 3" -PercentComplete (($i + 1) * (100/3))
for ($j = 0; $j -lt 3; $j++) {
Start-Sleep -Seconds 1
Write-Progress -Activity "Main Process" -Status "Step $($i + 1) of 3" -CurrentOperation "Inner Process $($j + 1) of 3" -PercentComplete (($i * 100/3) + (($j + 1) * (100/(3 * 3))))
}
}
In this example:
- The outer loop represents a main process, while the inner loop indicates multiple tasks within that process.
- Each layer of the progress bar is updated independently, allowing for a detailed, granular view of progress.
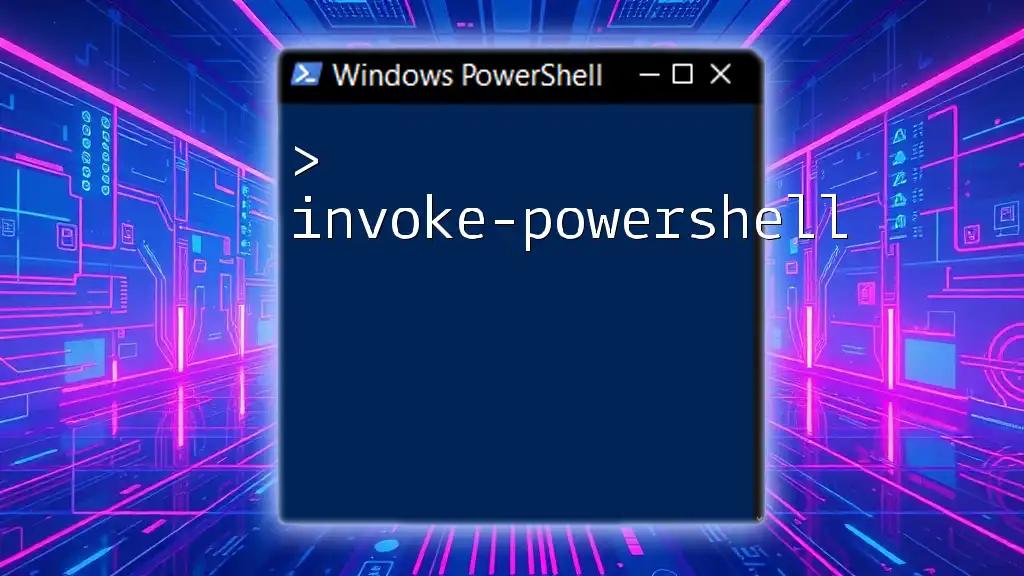
Best Practices for Using Progress Bars
Keeping Progress Bars Informative
It's essential to maintain clarity in your status messages. Users should have a solid understanding of what is currently happening, which can be achieved by using descriptive messages that provide context.
Balancing Progress Updates with Performance
While updates are essential, frequent updates can sometimes bog down performance. Implement a reasonable delay between updates, especially for operations that complete quickly. This not only increases the effectiveness of the progress bar but also can improve overall script performance.
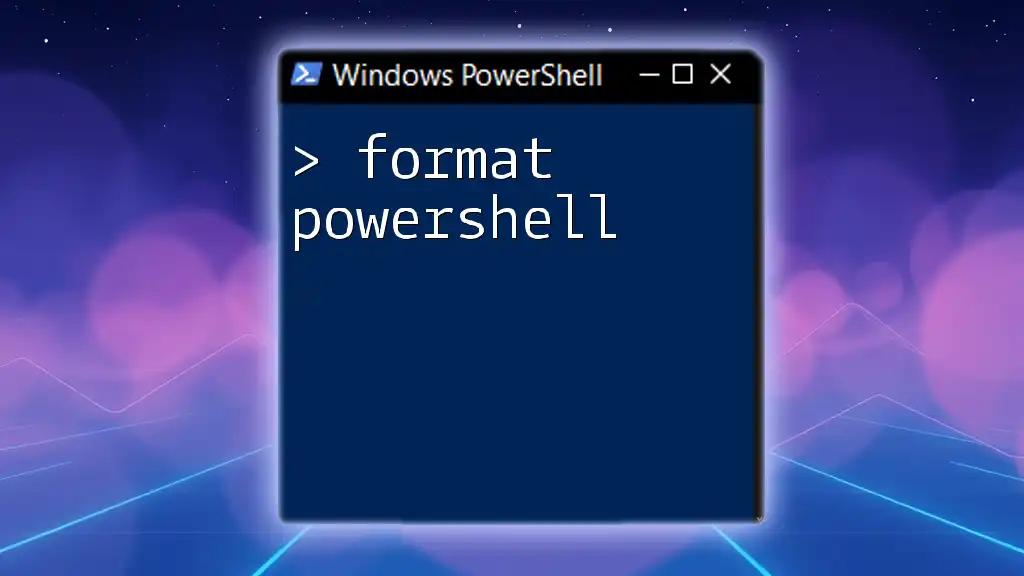
Sample Use Cases of PowerShell Progress Bars
Use Case 1: Data Import Scripts
In scripts that import large datasets, a progress bar can keep users informed about how much data has been processed. This visibility is crucial for users, especially if they are importing thousands of rows or more.
Use Case 2: Maintenance/Backup Tasks
For backup tasks that may take a long time to complete, implementing a progress bar is equally important. It gives users confidence that the task is in progress and reassures them when they see a visual indicator of completion.
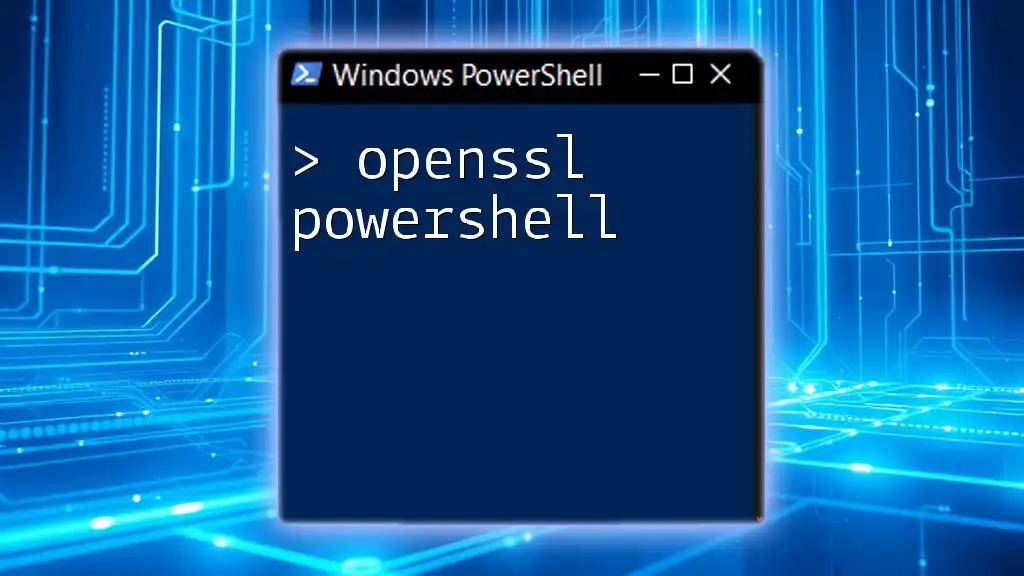
Common Issues and Troubleshooting
Problems with Display/Functionality
Sometimes, progress bars may not display correctly, particularly in different console types or environments. If you encounter display issues, try using other visual feedback mechanisms or validating your coding environment settings.
Debugging Progress Bar Scripts
Common mistakes might include missing parameters or incorrect percentages being passed to `Write-Progress`. Carefully review your script for errors, and consider adding debug statements to identify where issues might be occurring.
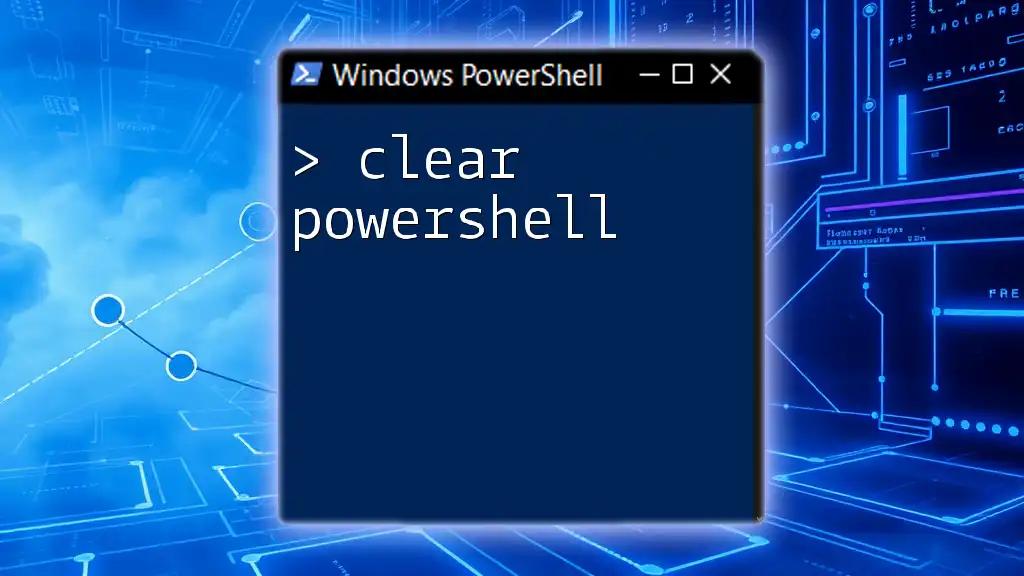
Conclusion
Incorporating a progress bar in PowerShell scripts not only improves user interaction but also instills a sense of control and awareness over script operations. Practice creating your own progress bars and explore their varying functionalities. As you become more comfortable with `Write-Progress`, you'll find it an indispensable tool in your PowerShell scripting toolkit. For additional tips and tricks on PowerShell, consider joining our community and stay updated!