To terminate a running process in PowerShell, you can use the `Stop-Process` cmdlet followed by the process name or ID.
Here’s a code snippet to illustrate this:
Stop-Process -Name "processName" -Force
Replace `"processName"` with the actual name of the process you want to kill.
Understanding Processes in Windows
What Is a Process?
A process is essentially an instance of a running application or program on your computer. Each process operates independently in its own environment and can include not only applications you use daily but also background tasks that keep your operating system functioning. Understanding how processes work is crucial for effective system management and optimization.
Why You Might Need to Kill a Process
There are various scenarios in which you may need to kill a process:
- Unresponsive Applications: Sometimes, apps freeze and do not respond to user input.
- Resource Hogging: Certain applications may consume excessive CPU or memory, slowing down your system.
- Testing: Developers often need to terminate processes during testing cycles when changes are made.
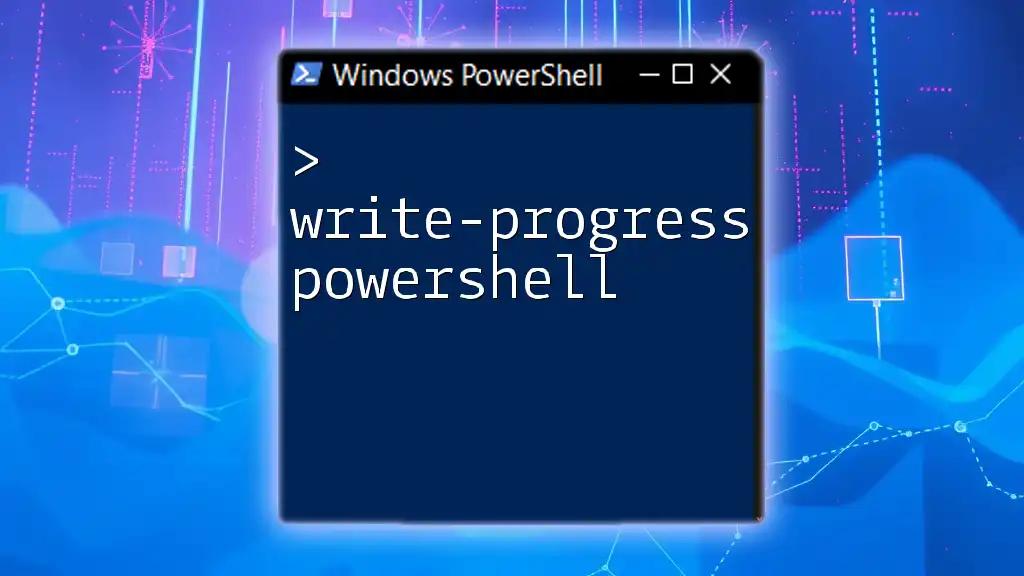
The PowerShell Kill Command
Introduction to the `Stop-Process` Cmdlet
PowerShell provides a variety of commands for managing system processes, with the `Stop-Process` cmdlet being the most prevalent for killing processes.
The basic syntax for using this cmdlet is:
Stop-Process -Id <ProcessId>
or
Stop-Process -Name <ProcessName>
By utilizing either of these syntaxes, you can efficiently terminate unwanted processes in your system.

Killing Processes by Name
How to Kill a Process Using PowerShell by Name
To kill a process by its name, you would use the `Stop-Process -Name` command followed by the specific name of the process.
Example: To kill a Notepad process, the command would look like this:
Stop-Process -Name "notepad"
This command will seamlessly terminate all instances of Notepad running on your machine.
Killing All Processes by Name
PowerShell allows you to use wildcards when specifying process names. This is particularly useful for terminating multiple instances or similar processes.
Example: To kill all instances of Google Chrome, you can issue the command:
Stop-Process -Name "chrome*" -Force
The `-Force` parameter ensures that even stubborn processes are terminated.
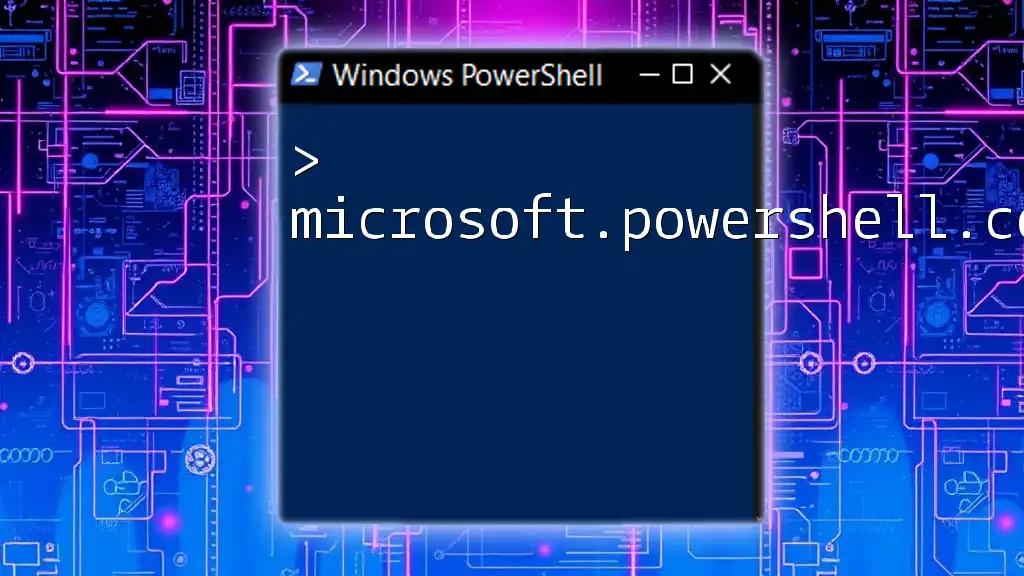
Killing Processes by ID
Using PowerShell to Kill Process by ID
Sometimes, you may want to kill a specific instance of a process, especially if multiple instances are running. To obtain the Process ID (PID), you can use the `Get-Process` command followed by filtering parameters.
Example: First, retrieve the PID for Notepad:
$processId = (Get-Process -Name "notepad").Id
Stop-Process -Id $processId
Here, `$processId` captures the ID of Notepad, which is then used to terminate that particular instance.
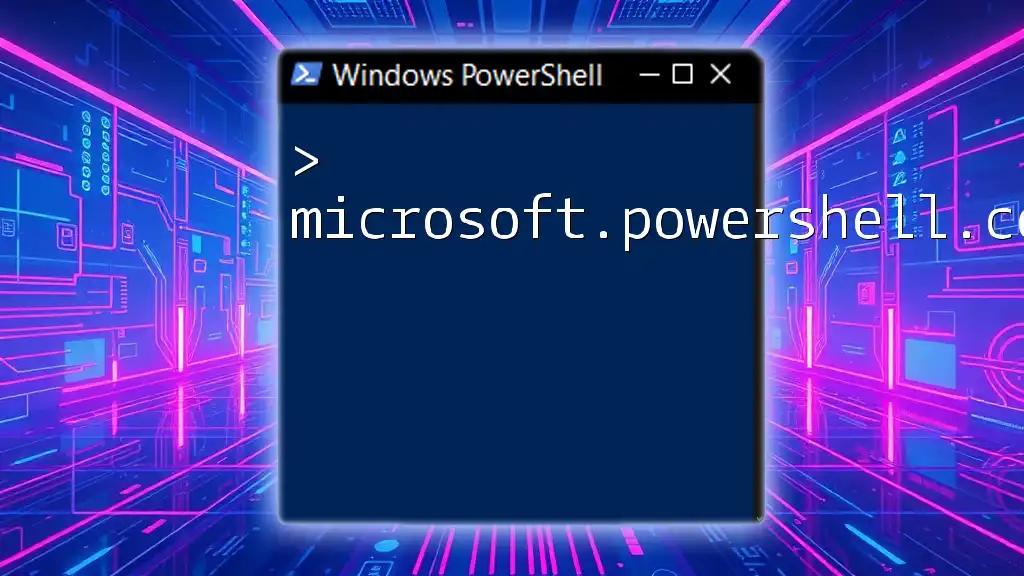
Forcefully Killing Processes
Understanding Process Force Killing
Using the `-Force` parameter allows you to terminate processes that may not respond to regular kill commands. It’s crucial to wield this power with caution, as forcefully killing a process can lead to data loss or corruption.
Examples of Force Killing a Process
When you find that a process isn't responding despite your initial attempt to stop it, you can invoke the `-Force` parameter.
Example: To forcefully kill a Notepad process, execute:
Stop-Process -Name "notepad" -Force
This command forces all instances of Notepad to terminate, regardless of their state.
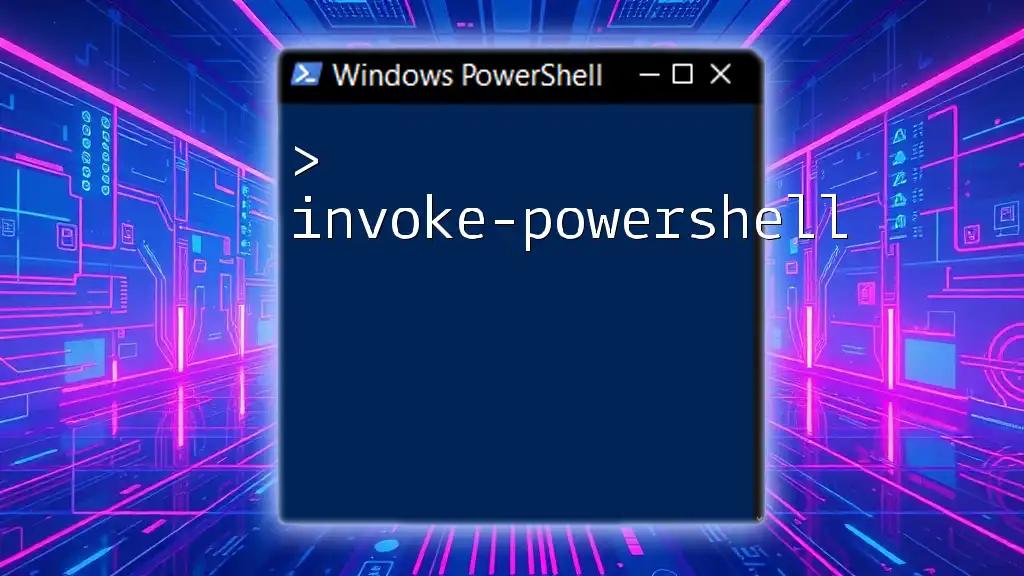
Killing Multiple Processes
A Simple Way to Kill Multiple Processes
If you need to kill several processes simultaneously, leveraging an array with the `Stop-Process` cmdlet can save you time.
Example: To kill both Notepad and Chrome processes, you can prepare an array:
$processesToKill = "notepad", "chrome"
Stop-Process -Name $processesToKill
Here, all specified processes are terminated in one go.
A More Advanced Approach: Using a Loop
For more complex scenarios, such as when checking process statuses before terminating them, a loop can be beneficial.
Example: Using a loop to kill several processes:
$processesToKill = @("notepad", "chrome")
foreach ($process in $processesToKill) {
Stop-Process -Name $process -Force
}
This method iterates through the array, applying the `Stop-Process` cmdlet to each named process.
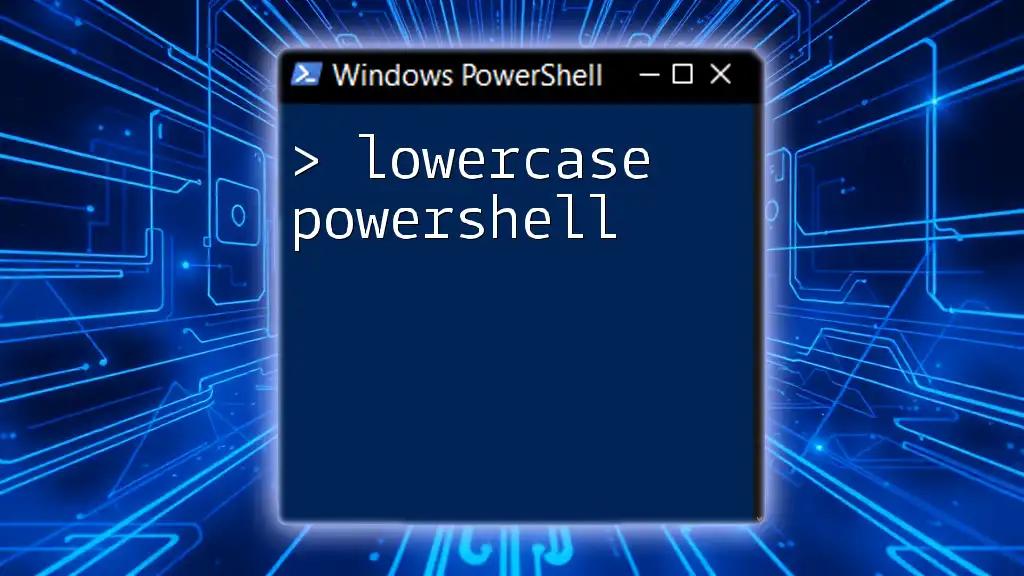
Utilizing PowerShell Task Kill Alternatives
Overview of `taskkill` Command
In addition to `Stop-Process`, Windows offers the `taskkill` command as part of the Command Prompt. While both serve similar functions, `taskkill` is more familiar to many users who have worked with the command line.
Examples Using `taskkill`
You can execute the `taskkill` command directly inside PowerShell to kill processes as well.
Example: To kill Notepad using `taskkill`, use:
taskkill /IM notepad.exe /F
This command directly targets the application's executable name, allowing for versatile process termination.
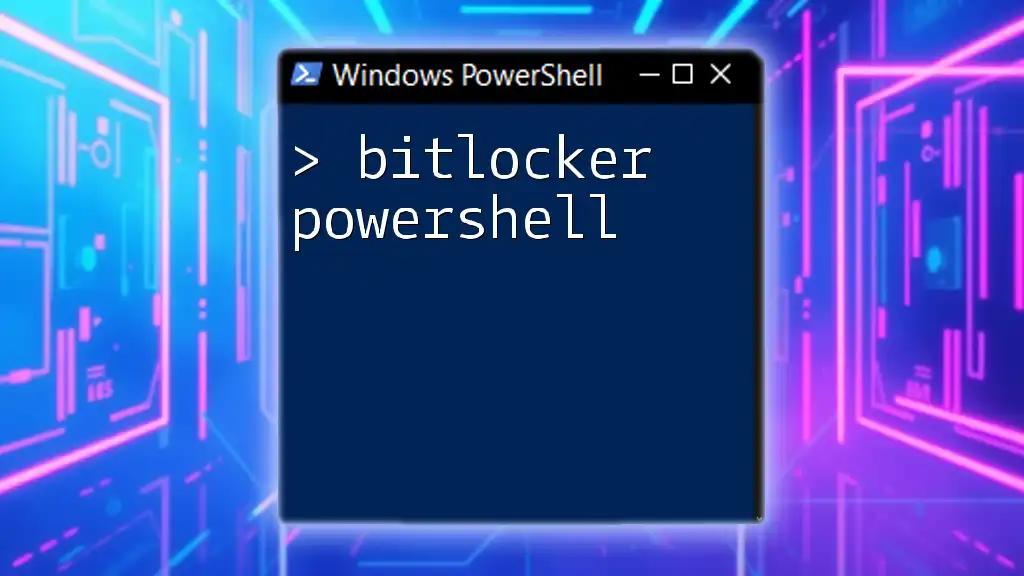
Best Practices for Killing Processes
Safety Measures
Before terminating any process, it's crucial to assess the impact of your actions. Make sure you fully understand what each process does and how killing it may affect your system.
Commands to Confirm Process Before Killing
A wise practice is to verify the process you're about to terminate using the `Get-Process` command as follows:
Get-Process -Name "notepad"
This command displays detailed information about the specific process, helping you make well-informed decisions.

Troubleshooting Common Issues
Common Errors When Killing Processes
You may encounter several common errors, such as “Process not found” or “Access denied.” These often stem from either targeting an incorrect process name or lacking the necessary user permissions.
Solutions to Common Problems
If your user account lacks permissions, consider running PowerShell as an Administrator. To do this, right-click the PowerShell icon and select Run as Administrator. This elevates your command privileges and may solve access-related issues.
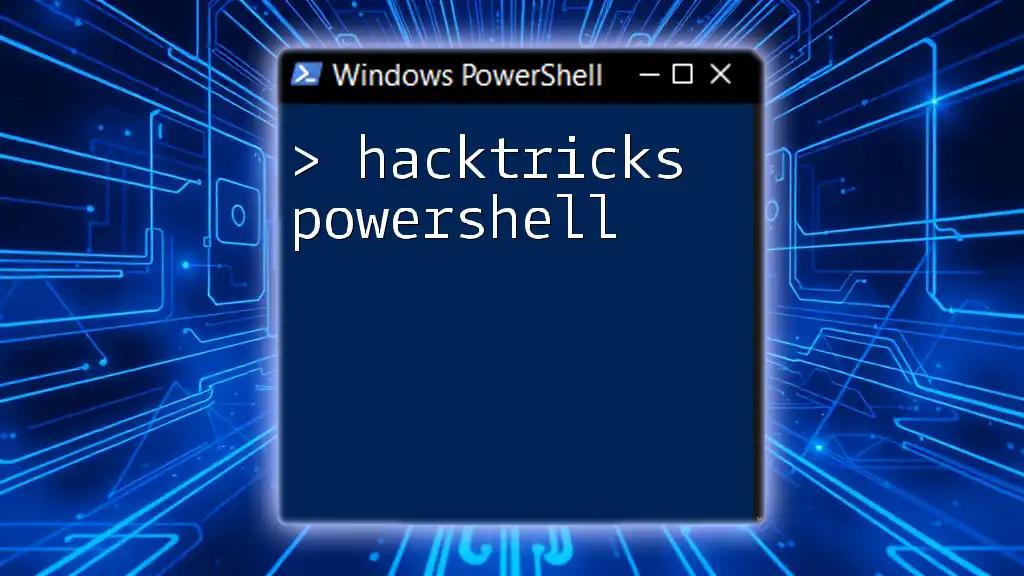
Conclusion
Mastering how to kill processes using PowerShell can dramatically improve system management and operation efficiency. Whether you find yourself needing to stop an unresponsive application or terminate resource-heavy processes, understanding the various methods of process termination in PowerShell allows you to keep your machine running smoothly.

Call to Action
If you found this guide helpful, be sure to subscribe for more insights and tutorials on PowerShell. Share your experiences and learnings about using PowerShell commands with your peers!
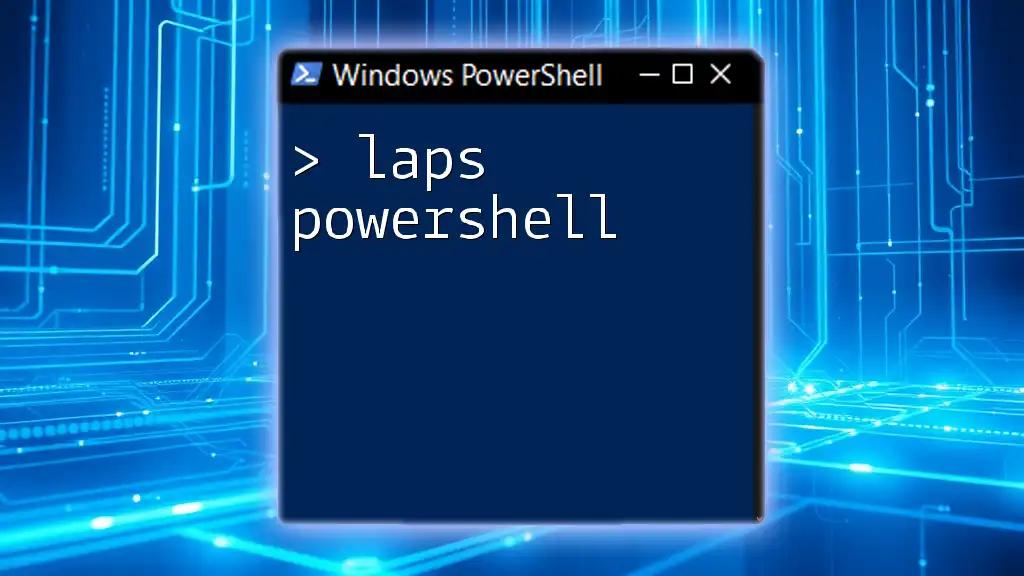
Additional Resources
For further exploration, check out the official Microsoft documentation on PowerShell and additional resources available on PowerShell scripting courses. These materials can deepen your understanding and expand your PowerShell skills to new heights.