To start a process with elevated privileges using PowerShell, you can utilize the `Start-Process` cmdlet with the `-Credential` parameter for the `runas` functionality.
Here's a code snippet for your reference:
$cred = Get-Credential
Start-Process "notepad.exe" -Credential $cred
Understanding Start-Process
What is Start-Process?
The Start-Process cmdlet in PowerShell is a robust command designed to initiate processes on your local computer. It allows users to run executables, open documents with their associated applications, and even execute scripts.
One of the primary advantages of using Start-Process is its ability to run processes independently of the PowerShell session that started them. This means you can continue using your PowerShell session while the initiated process runs in the background.
Syntax of Start-Process
The syntax of Start-Process is straightforward but powerful. Here is a breakdown of its essential components:
Start-Process -FilePath <string> [-ArgumentList <string[]>] [-WorkingDirectory <string>] [-NoNewWindow] [-Wait]
- -FilePath: The path to the executable or script you wish to run.
- -ArgumentList: Optional parameters that can be passed to the executable.
- -WorkingDirectory: Specifies the directory in which to run the process.
- -NoNewWindow: If this parameter is specified, the process will be started in the current console window rather than a new one.
- -Wait: Indicates that the command will pause the execution of the script until the launched process has completed.

Running a Process with Elevated Permissions
What is RunAs?
RunAs is a Windows feature that allows users to run applications with different permissions than the user's current session. Typically, this is used for running applications with administrative privileges, which is essential for many administrative tasks in system management.
Understanding when to use RunAs is crucial, as it ensures that you can execute processes that may change system settings, install software, or perform actions restricted to standard user profiles.
Using Start-Process with RunAs
The Basic Command
Integrating RunAs with Start-Process allows you to run processes under a different user context seamlessly. Here’s a basic example of executing a command as a different user:
Start-Process "powershell.exe" -ArgumentList "-Command", "Get-Process" -Credential (Get-Credential)
In this example, a PowerShell command is initiated to list the processes running on the system. The -Credential parameter prompts for a username and password, effectively allowing you to authenticate as a different user.
Examples of Running Different Applications
One of the most common needs is to run applications with administrative rights. Here’s how to open Notepad as an Administrator:
Start-Process "notepad.exe" -Verb RunAs
This command prompts the User Account Control (UAC) to elevate Notepad's permissions, enabling you to perform actions that require administrative access.
Another frequent scenario is executing a PowerShell script with elevated permissions:
Start-Process "powershell.exe" -ArgumentList "-File 'C:\Path\To\YourScript.ps1'" -Verb RunAs
This command lets you run a specific script as an administrator, ensuring that it has the right permissions to execute.
Handling UAC Prompts
Understanding User Account Control (UAC)
User Account Control (UAC) is a security feature in Windows designed to prevent unauthorized changes to the operating system. While UAC increases security, it can often become an obstacle when you attempt to run applications as an administrator.
Suppressing UAC Prompts
Managing UAC prompts can enhance productivity. One way to minimize UAC prompts is to use Task Scheduler to create a task that runs with elevated permissions. Execute the following command to do this:
schtasks.exe /create /tn "RunAsElevatedTask" /tr "powershell.exe -File 'C:\Path\To\YourScript.ps1'" /sc onlogon /rl highest
This command schedules a task to run your PowerShell script automatically when you log on, alleviating the need for constant UAC prompts during execution.

Tips and Best Practices
Security Considerations
When using RunAs and handling credentials, it's essential to prioritize security. Avoid hardcoding sensitive information or passwords directly into your scripts. Instead, encourage the use of Get-Credential to input credentials securely at runtime.
Error Handling
While working with Start-Process, it's not uncommon to encounter errors, especially if the command or file paths are incorrect. Effective error handling enhances the robustness of your scripts. Here’s an example of error handling that captures and displays errors appropriately:
try {
Start-Process "notepad.exe" -Verb RunAs
} catch {
Write-Host "An error occurred: $_"
}
This snippet will alert you if there’s an issue starting Notepad, providing insight into what went wrong.
Performance Considerations
Using RunAs can introduce some overhead, especially when launching resource-intensive applications. It's crucial to be mindful of how this impacts performance. Utilizing the -Wait parameter allows you to control the flow of subsequent commands until the initiated process has completed execution.
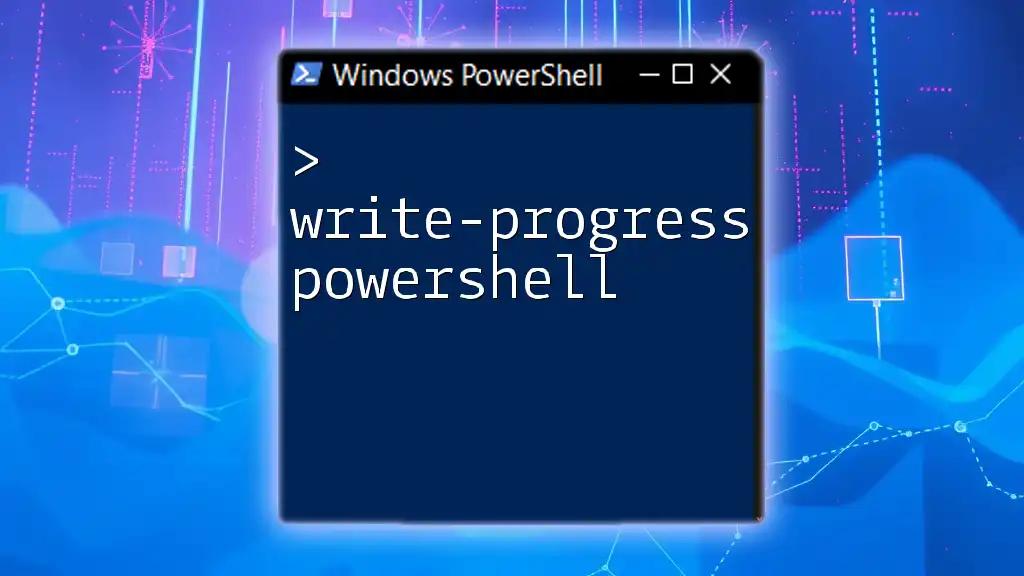
Advanced Examples
Running Scripts in Different Environments
PowerShell can run in various environments, including PowerShell ISE and PowerShell Core. When launching scripts across these environments, you maintain the same syntax for execution. Here's an example:
Start-Process "powershell.exe" -ArgumentList "-File 'C:\Path\To\YourScript.ps1'" -Verb RunAs -WorkingDirectory "C:\Path\To\"
This command ensures that the script runs in the context of the specified working directory.
Automating Routine Tasks
Automation is key to effective system management. You can use Start-Process to execute multiple commands or scripts. Here’s how you could automate routine tasks efficiently:
$tasks = @("Task1", "Task2", "Task3")
foreach ($task in $tasks) {
Start-Process "powershell.exe" -ArgumentList "-Command", $task -Verb RunAs
}
In this script, a series of tasks is run, all elevated, streamlining your daily processes.

Conclusion
Mastering the Start-Process PowerShell RunAs capability empowers you to efficiently manage processes within your Windows environment. By understanding how to start processes with different user privileges, handle UAC prompts, and implement error handling, you equip yourself with critical skills for effective system administration.
As you experiment with the examples provided, you will become more adept at leveraging PowerShell for your administration tasks. The capability to run processes with elevated permissions is essential, and with practice, you'll find your ability to use these tools will enhance your productivity significantly.

Resources
- For further readings and references, you can refer to the official Microsoft documentation on PowerShell and the Start-Process cmdlet.
- Join community forums and discussion platforms to enhance your skills further and seek clarification on any queries you may have regarding PowerShell commands.