The "Start-Process" command in PowerShell allows you to initiate a new process or application directly from the command line.
Here's a code snippet to illustrate how to use it:
Start-Process notepad.exe
Understanding PowerShell Tasks
What are PowerShell Tasks?
PowerShell tasks are automated commands or scripts that enable users to perform various operations on a Windows system efficiently. Tasks can range from starting applications to managing system services or executing scheduled jobs. They are a fundamental aspect of PowerShell's ability to streamline repetitive actions and enhance productivity.
For example, launching an application like Notepad or restarting a service such as Windows Update can be automated using PowerShell tasks, allowing IT professionals to save time and reduce human error.
The Importance of Tasks in Automation
Automation plays a crucial role in IT and development environments. By using PowerShell to automate tasks, users can:
- Increase operational efficiency
- Reduce the risk of errors
- Ensure consistency in task execution
- Free up time for more critical activities
With the power of PowerShell, you can automate tedious tasks, enabling a smoother workflow and allowing you to focus on strategic initiatives.
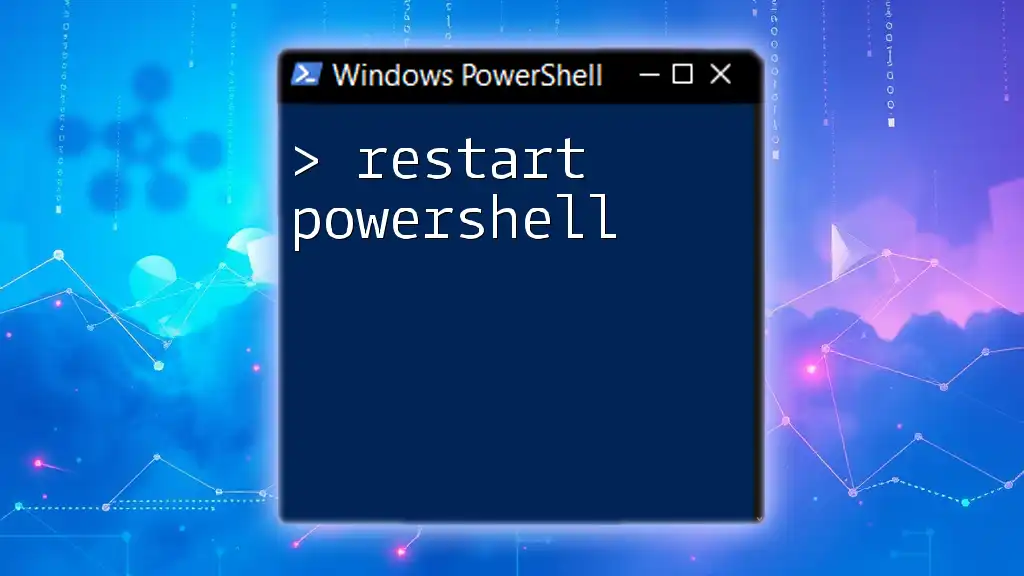
Starting Tasks with PowerShell
The `Start-Process` Cmdlet
A fundamental way to start a task in PowerShell is by using the `Start-Process` cmdlet. This cmdlet allows you to run executable files, scripts, or any other command line-based utilities.
Syntax of `Start-Process` is straightforward:
Start-Process -FilePath "path_to_executable"
For example, to start Notepad, you would use the following command:
Start-Process "notepad.exe"
The `Start-Process` cmdlet also comes with various parameters that can enhance its functionality. For instance, you can specify the working directory, pass arguments, or run the process in elevated mode. Here’s a brief rundown:
- -ArgumentList: Pass parameters to the process.
- -WorkingDirectory: Set the current directory for the process.
- -Credential: Run the process under a specified user account.
Starting Services Using PowerShell
Using `Start-Service`
Windows services are critical components of the operating system. You can easily manage these services using PowerShell. The `Start-Service` cmdlet is designed specifically for this purpose.
The syntax for `Start-Service` is simple:
Start-Service -Name "service_name"
To start a specific service, such as the Windows Update service (`wuauserv`), you can use:
Start-Service -Name "wuauserv"
Using `Start-Service` helps to ensure that essential services are running, and it can be vital for maintaining system performance and reliability.
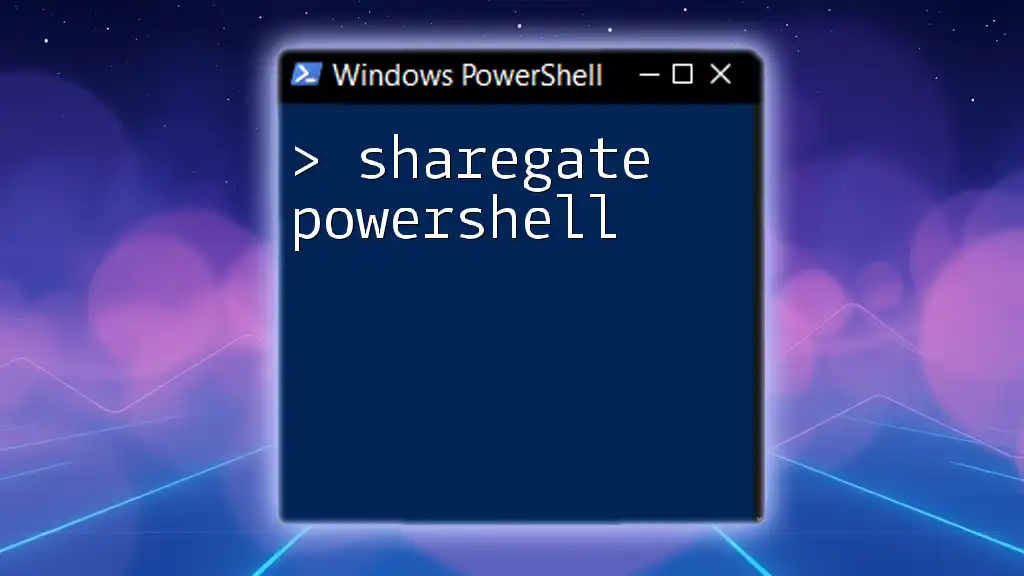
Running Scheduled Tasks from PowerShell
Understanding Scheduled Tasks
Scheduled Tasks are automated jobs that can run at specified times or under specific conditions, making them an indispensable part of system administration. PowerShell provides powerful tools to manage these tasks seamlessly.
Using PowerShell to Start a Scheduled Task
The `Start-ScheduledTask` Cmdlet
When you want to start a previously defined scheduled task using PowerShell, the `Start-ScheduledTask` cmdlet is what you need.
The basic syntax is as follows:
Start-ScheduledTask -TaskName "task_name"
For example, if you have a scheduled task named "MyScheduledTask," you can initiate it like this:
Start-ScheduledTask -TaskName "MyScheduledTask"
This command efficiently triggers the execution of the specified task—no need to navigate through the Task Scheduler GUI.
Checking the Status of Scheduled Tasks
Using `Get-ScheduledTask`
To manage scheduled tasks properly, it’s essential to check their status. The `Get-ScheduledTask` cmdlet allows you to retrieve information about all scheduled tasks or specific ones based on various criteria.
For instance, to check the status of "MyScheduledTask," you can use:
Get-ScheduledTask | Where-Object {$_.TaskName -eq "MyScheduledTask"}
This command filters the scheduled tasks, allowing you to examine their properties and confirm whether the task is running, ready, or disabled.
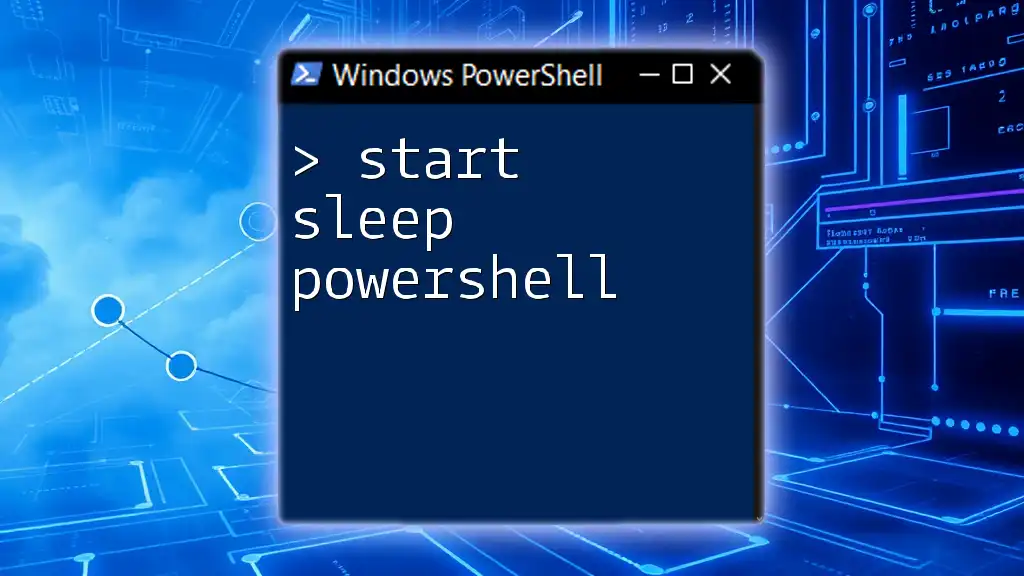
Advanced Usage of Starting Tasks
Using Scripts to Start Multiple Tasks
In a dynamic environment, you might need to start several tasks simultaneously. PowerShell scripting enables you to do this efficiently. Here’s a simple script that starts multiple scheduled tasks:
$tasks = @("Task1", "Task2", "Task3")
foreach ($task in $tasks) {
Start-ScheduledTask -TaskName $task
}
This script stores the task names in an array and then iteratively starts each task. This method not only saves time but also reduces potential errors that can occur when starting tasks individually.
Error Handling When Starting Tasks
Error handling should always be part of your script to ensure robust automation. Utilizing try-catch blocks can help manage exceptions gracefully.
Here’s an example of how to implement error handling when starting a scheduled task:
try {
Start-ScheduledTask -TaskName "InvalidTask"
} catch {
Write-Host "An error occurred: $_"
}
This snippet attempts to start an invalid task and captures any errors, providing feedback instead of terminating the script unexpectedly.
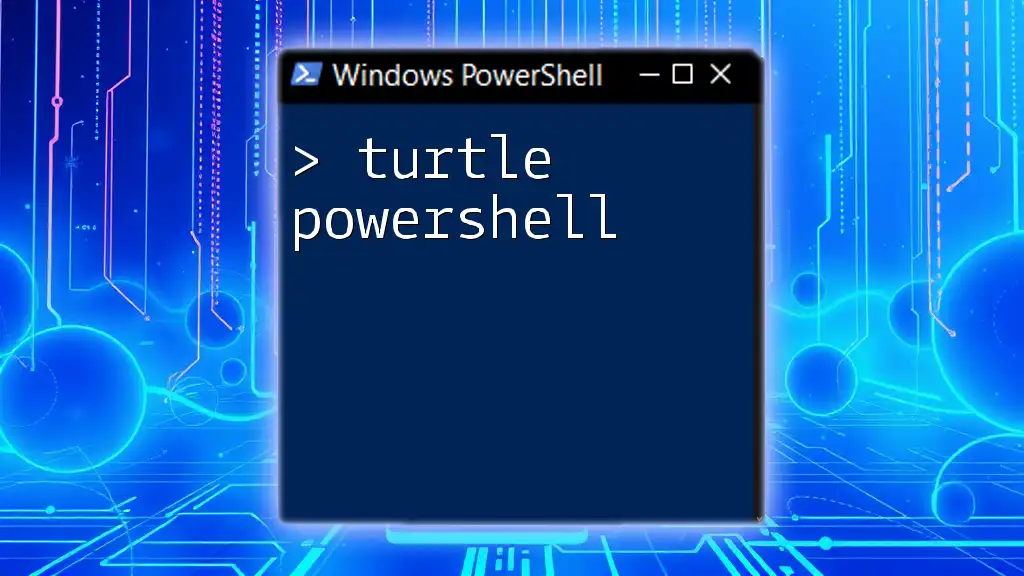
Troubleshooting Common Issues
Common Errors When Starting Tasks
When working with PowerShell to start tasks, you may encounter common issues, such as:
- Task names that do not exist
- Lack of permissions to execute a task
- Incorrect cmdlet usage
To troubleshoot these problems, it's essential to verify the task's existence and your user permissions.
Verifying Task Execution
After initiating a task, confirming that it has started successfully is crucial. You can achieve this by checking if the relevant process is running. For instance, to verify if Notepad is running, you can use:
Get-Process | Where-Object {$_.Name -eq "notepad"}
This command filters the process list to check for the Notepad process, ensuring that your command to start the task was successful.
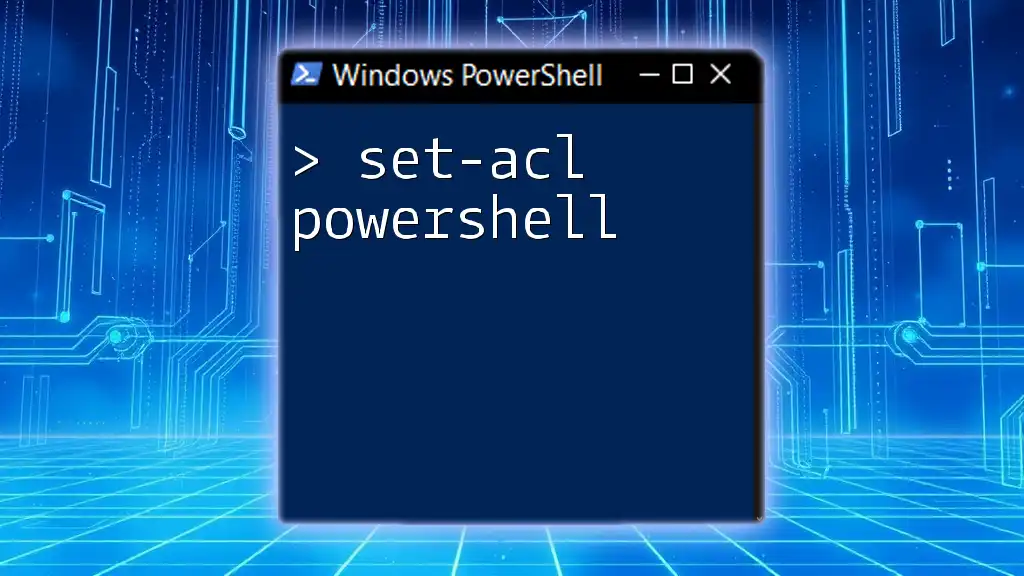
Conclusion
Starting tasks using PowerShell is an essential skill that empowers users to automate their workflows and streamline system management. By leveraging cmdlets like `Start-Process`, `Start-Service`, and `Start-ScheduledTask`, you can efficiently manage your tasks while minimizing errors and maximizing productivity.
Experiment with these commands and explore the vast possibilities PowerShell offers for task automation! Whether you're an IT professional or a developer, mastering these skills can significantly enhance your ability to deliver efficient solutions.