The `Write-Progress` cmdlet in PowerShell is used to display a progress bar in the command line, indicating the status of a long-running operation.
Here’s a simple code snippet to demonstrate its usage:
for ($i = 1; $i -le 100; $i++) {
Write-Progress -PercentComplete $i -Status "Processing..." -CurrentOperation "Step $i"
Start-Sleep -Milliseconds 50
}
Understanding Write-Progress
What is Write-Progress?
`Write-Progress` is a cmdlet in PowerShell that provides a way to display progress information to the user when executing scripts or long-running commands. This cmdlet plays a vital role in enhancing the user experience by offering visual feedback during script execution. When scripts take time to complete—like batch processing tasks, file transfers, or data migrations—users appreciate knowing the status of ongoing operations.
Key Features of Write-Progress
- Visual Feedback: It allows the script to present a progress bar with real-time updates, indicating how much of the task is complete.
- Customizability: The appearance of the progress bar can be tailored to meet specific user needs, making it easier to understand what is happening at any moment.
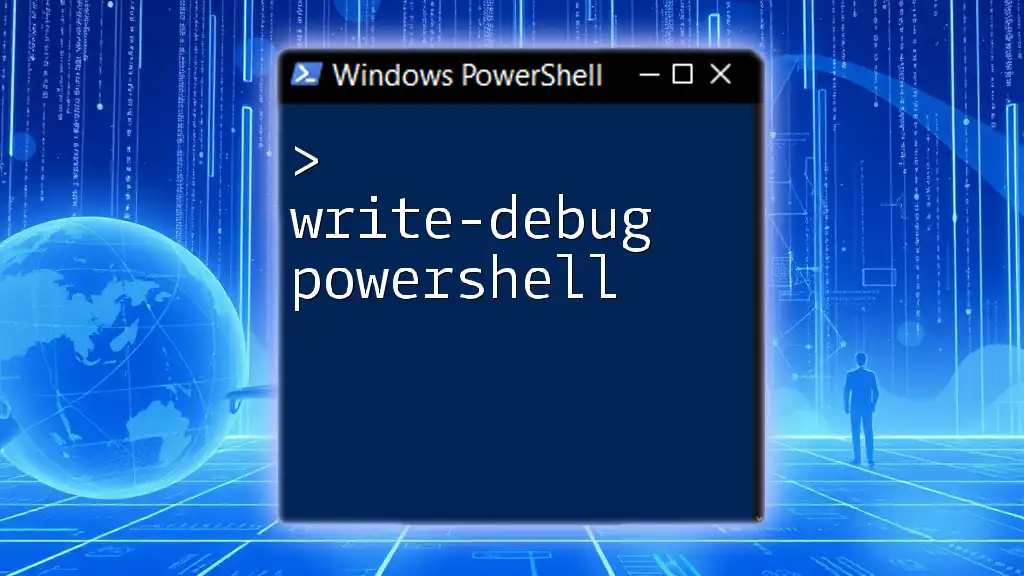
Syntax of Write-Progress
Basic Syntax Breakdown
The basic structure of the `Write-Progress` cmdlet is simple yet powerful. Here’s the foundational syntax:
Write-Progress -Activity "Activity Name" -Status "Current Status" -PercentComplete 0-100
- Activity: This parameter allows you to specify what overarching task is currently being executed.
- Status: Here, you provide additional context about the current state of the task, adding clarity.
- PercentComplete: This numeric value, ranging from 0 to 100, indicates the completion percentage of the currently tracked activity.
Additional Parameters
`Write-Progress` also accepts several other parameters that can enhance its functionality:
- -Id: You can use this parameter to identify multiple active progress indicators, allowing scripts to display information for different tasks simultaneously.
- -CurrentOperation: This parameter specifies the exact operation in progress, giving the user a clearer picture of what’s happening.
- -SecondsRemaining: This optional parameter provides an estimate of how long the current task is expected to take.
Here’s an example that showcases several parameters being used together:
Write-Progress -Id 1 -Activity "Copying Files" -Status "Copying Document.txt" -PercentComplete 50 -CurrentOperation "Document.txt"
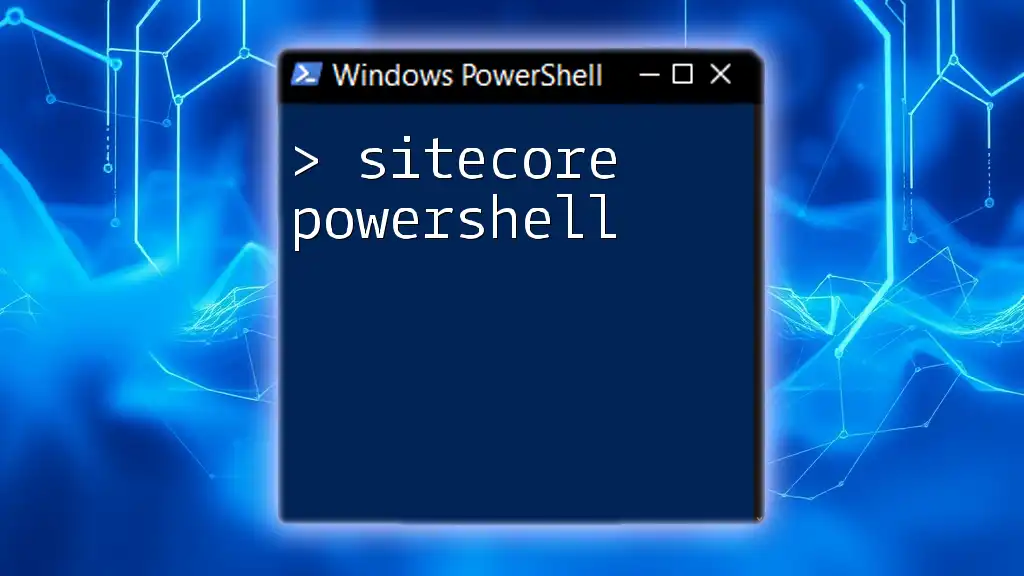
How to Use Write-Progress in Your Scripts
Simple Example
To illustrate the use of `Write-Progress`, consider the following straightforward loop that processes items. This demo updates the progress bar as each item is processed:
for ($i = 1; $i -le 10; $i++) {
Write-Progress -Activity "Processing Items" -Status "Processing item $i" -PercentComplete (($i / 10) * 100)
Start-Sleep -Seconds 1 # Simulates a time-consuming operation
}
In this example, as the loop iterates over a ten-item list, the progress bar updates every second, letting users know which item is currently being processed and how far along the operation is.
Advanced Example
Using Write-Progress with Data Operations
For a more complex scenario, consider a script that copies files from one directory to another. The usage of `Write-Progress` here not only informs users which file is being copied but also updates the overall progress percentage:
$files = Get-ChildItem "C:\Source"
$totalFiles = $files.Count
for ($i = 0; $i -lt $totalFiles; $i++) {
$file = $files[$i]
# Here, we perform the copy operation
Copy-Item $file.FullName "C:\Destination\$($file.Name)"
Write-Progress -Activity "Copying Files" -Status "Copying $($file.Name)" -PercentComplete (($i / $totalFiles) * 100)
}
In this example, as files are copied, users see real-time updates about the specific file being processed and what percentage of the total task is complete.
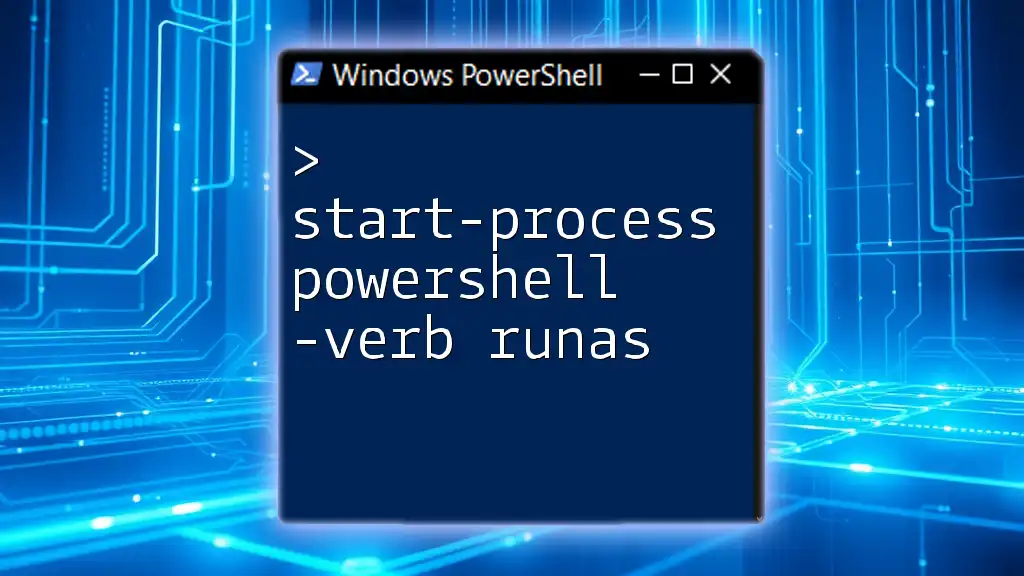
Practical Use Cases for Write-Progress
Batch Processing Tasks
When performing batch operations, such as processing data records or migrating databases, `Write-Progress` can be invaluable. It provides users with a clear indication of how far along the script is, ultimately enhancing the overall experience.
Long-Running Scripts
In any scenario where a PowerShell script takes a considerable amount of time to execute, it is crucial to give users constant feedback. Using `Write-Progress` transforms the user experience from waiting in uncertainty to actively engaging with the script's progress.
Contextual Progress Information
Advanced use of `Write-Progress` facilitates the monitoring of multiple operations within a single script. For instance, if you were running several tasks sequentially, incorporating unique `Write-Progress` messages for each could help users keep track of everything that is happening.
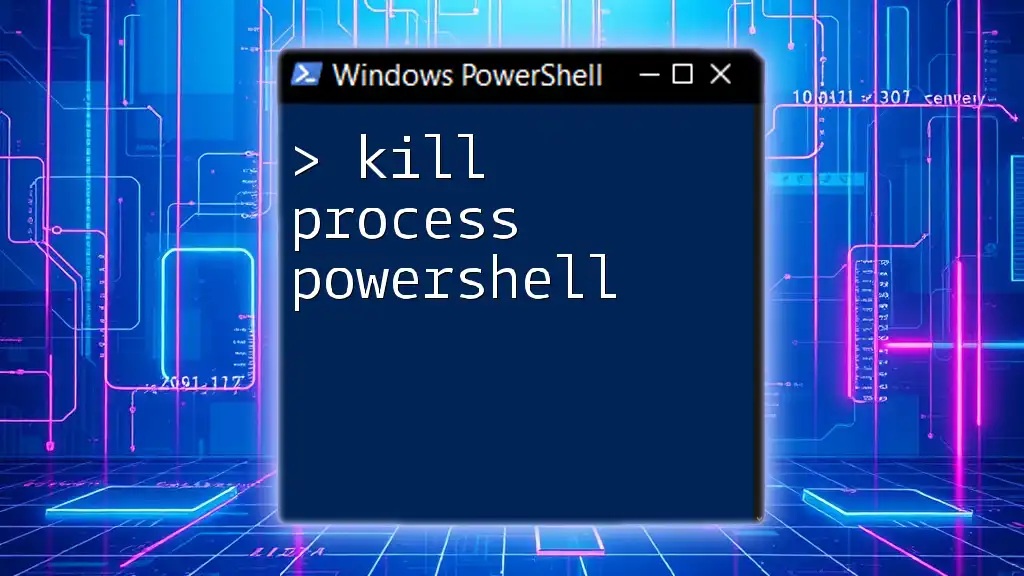
Best Practices for Using Write-Progress
Consistency and Clarity
When utilizing `Write-Progress`, it’s critical to maintain consistency. Ensure that the parameters you update are relevant and clearly convey the current state of the operation. This helps users understand what to expect and enables them to assess performance effectively.
Testing and Validation
Before deploying scripts that use `Write-Progress`, conduct thorough testing. Validate that each progress message updates correctly and accurately reflects the operation's status. This validation is vital for maintaining user trust.
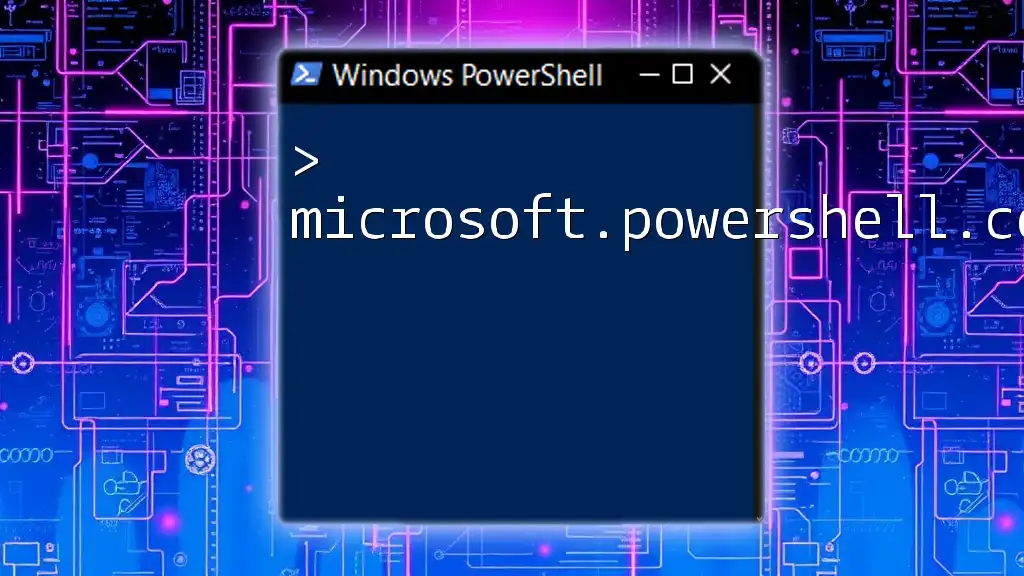
Debugging Progress Issues
Common Problems
Sometimes, scripts may experience issues where the progress display does not appear correctly. Common reasons for this include missing parameters or logical errors in the script logic causing the progress bar to halt.
Troubleshooting Steps
To troubleshoot progress display issues, review the parameters being used in `Write-Progress`. Ensure that the logic related to the completion percentage and other parameters is functioning as expected.
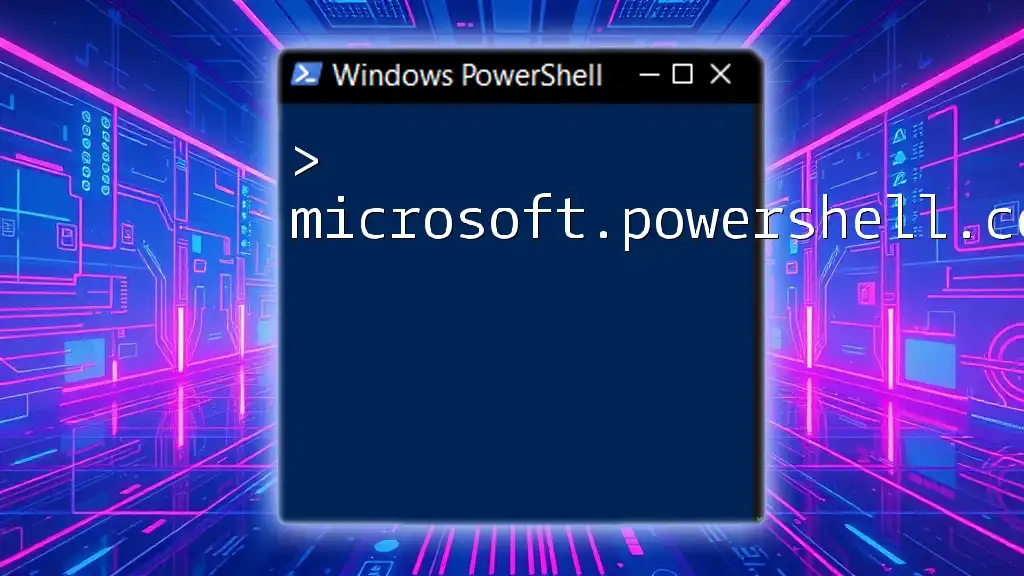
Conclusion
Integrating `Write-Progress` into your PowerShell scripts is an effective way to provide visual feedback during lengthy operations and significantly enhance user experience. By employing this cmdlet, you facilitate clearer communication through real-time progress updates, giving users a pleasant interaction with your scripts.
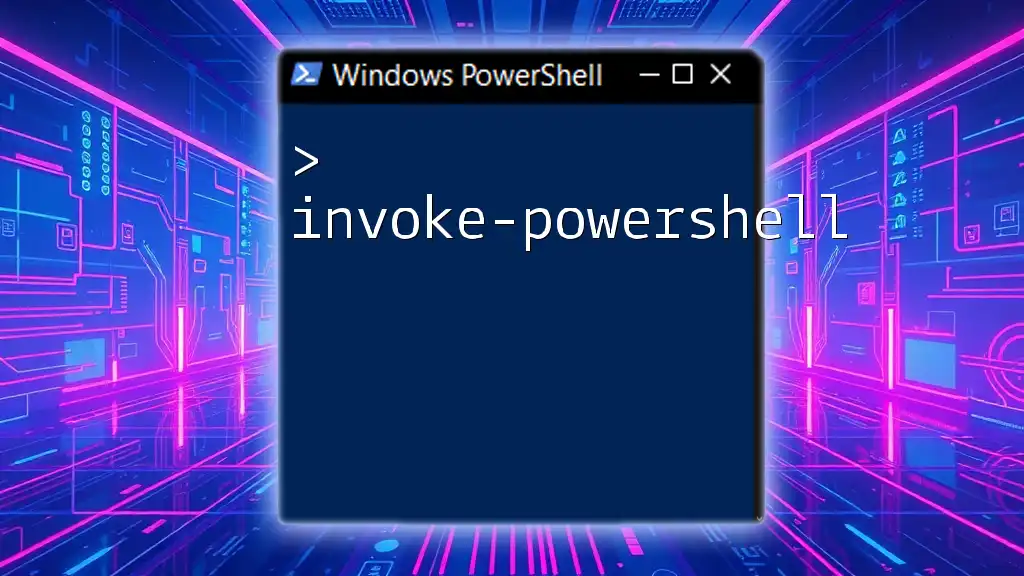
Further Resources
Documentation Links
For more details, check the official PowerShell documentation on `Write-Progress`. This resource provides comprehensive guidance and additional context.
Community Forums
Engagement with PowerShell community forums can also provide peer insights, troubleshooting tips, and shared experiences to enrich your understanding of using `Write-Progress`.
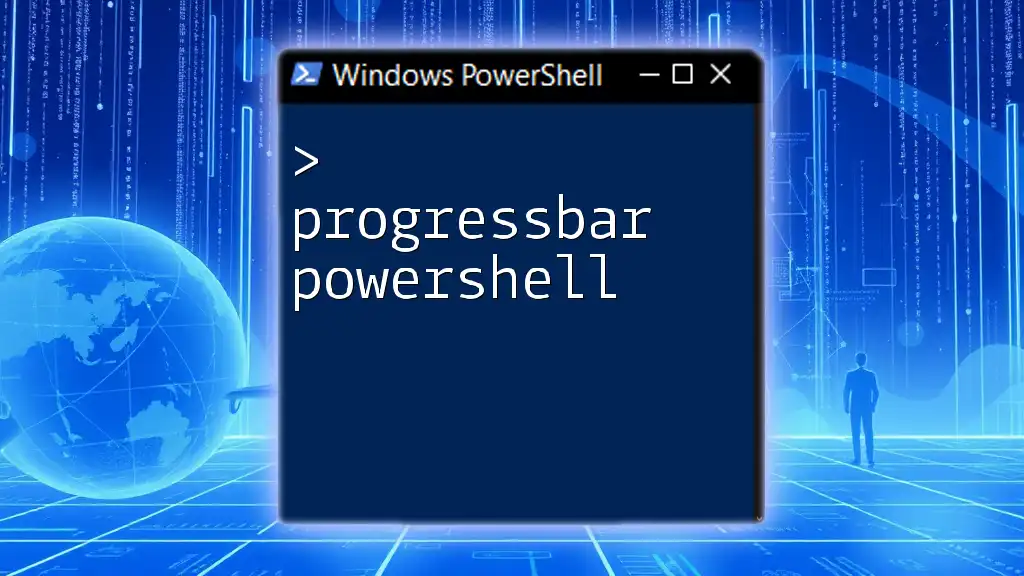
Call to Action
Challenge yourself to implement `Write-Progress` in your next PowerShell script. Observe how this simple enhancement can make a significant difference in user experience and script effectiveness. Share your experiences as you explore this useful cmdlet!