The `Write-Debug` cmdlet in PowerShell is used to output debug messages that can help in tracking the flow of a script during execution when debugging is enabled.
Here's a code snippet demonstrating its use:
Write-Debug 'This is a debug message.'
To see the output, you need to enable debug messages by running your script with the `-Debug` switch.
What is Write-Debug?
`Write-Debug` is a cmdlet in PowerShell specifically designed to help developers debug their scripts and functions by providing debug messages during execution. Debugging is a critical aspect of script development, as it allows you to track down and resolve issues quickly. It's a lightweight tool that allows for better visibility into your code without cluttering your output with print statements or errors.
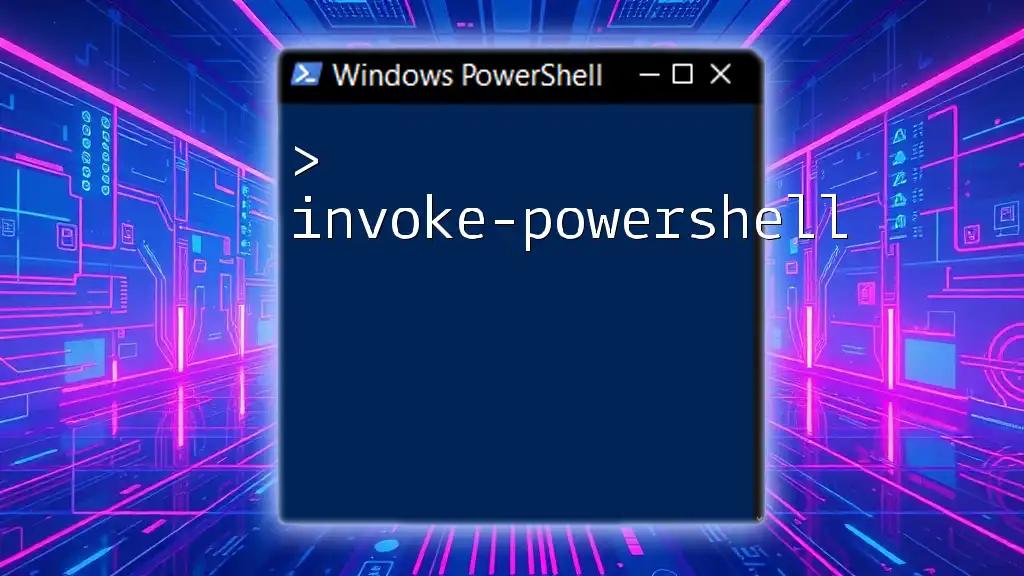
Why Use Write-Debug?
Using `Write-Debug` can significantly enhance your development process for several reasons:
- Clarity in Development: It provides a clear and concise way to output messages from your scripts, which can be invaluable when you’re trying to understand what is happening at various points in your code.
- Non-Intrusive: Unlike using `Write-Host` or `Write-Output`, `Write-Debug` does not affect the actual output of the script unless debugging is enabled, keeping user outputs clean.
- Dynamic Control: Being able to enable or disable debug messages at runtime means you can easily toggle these messages based on your current needs.
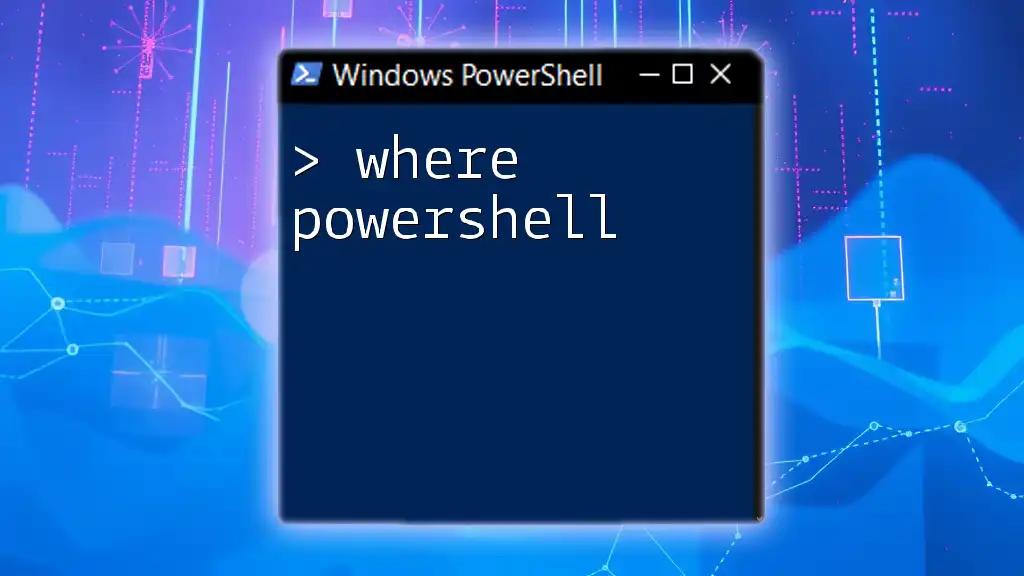
Understanding Write-Debug Syntax
Basic Syntax Overview
The basic syntax for the `Write-Debug` cmdlet is simple. To write a debug message, you can use:
Write-Debug "This is a debug message"
This command sends a message to the debug output stream, which will only be displayed if `DebugPreference` is set to "Continue."
The Importance of the `-Message` Parameter
Although the `Write-Debug` cmdlet can be used without any parameters, using the `-Message` parameter gives you clearer and more defined messages. For example:
Write-Debug -Message "Variable value: $myVariable"
This explicit structure helps maintain better readability in your debugging outputs.
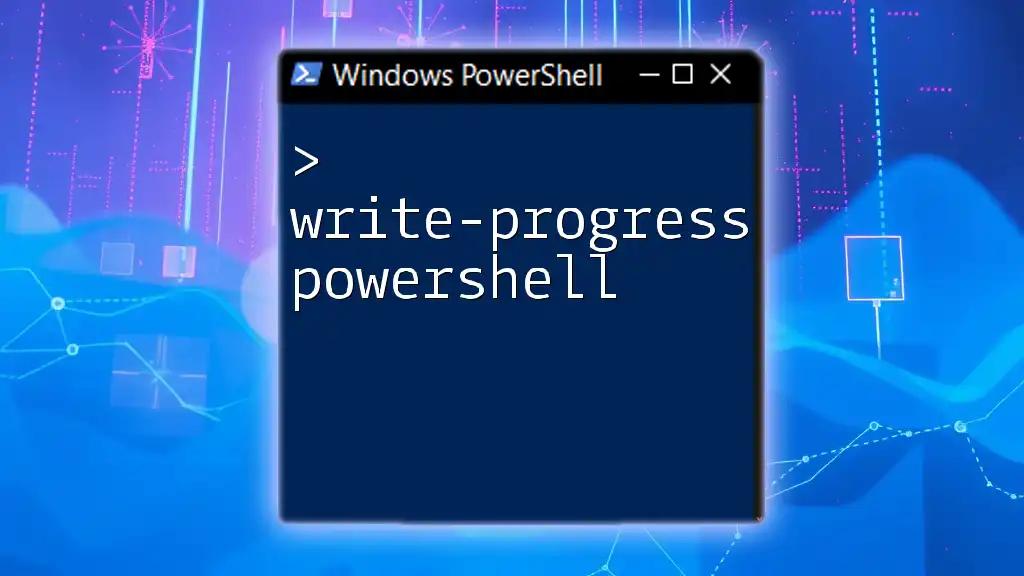
Configuring Debugging in PowerShell
Enabling Debugging Messages
To see the debug output messages while your script executes, you need to set the `$DebugPreference` variable to "Continue". You can do this by running:
$DebugPreference = "Continue"
This action instructs PowerShell to display all debug messages generated by `Write-Debug`.
Understanding DebugPreference Levels
`DebugPreference` can take on several values, each affecting how debug messages are handled:
- SilentlyContinue: Suppresses all debug output.
- Continue: Displays debug messages.
- Inquire: Prompts before displaying each debug message.
- Stop: Stops script execution upon encountering debug messages.
To change the `DebugPreference`, you can simply assign it as shown:
$DebugPreference = "Inquire"
This asks for confirmation before displaying each debug message, which can be helpful in lengthy scripts where you want control over what gets reported.
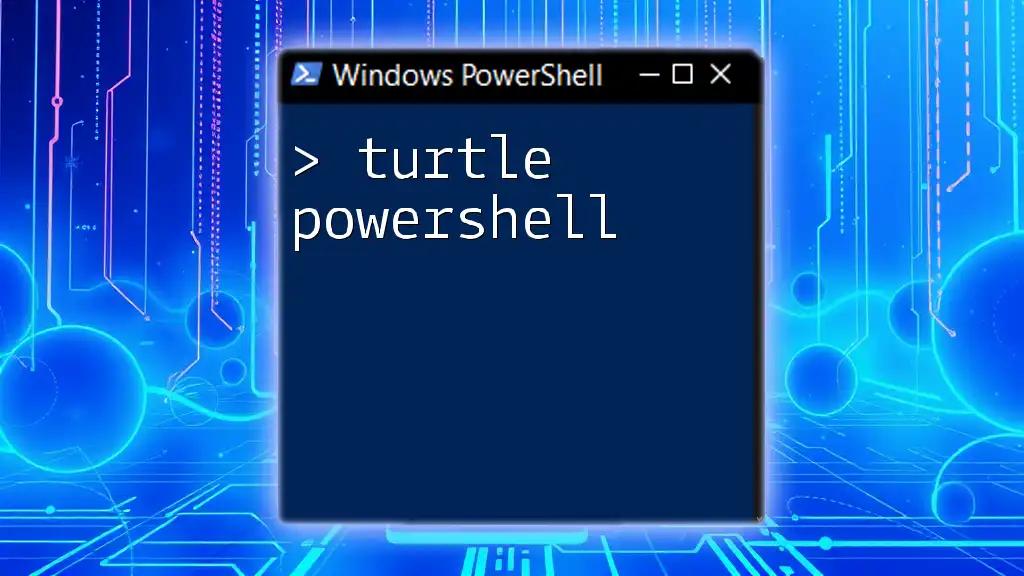
Utilizing Write-Debug in Scripts
Inserting Write-Debug in Your Scripts
When writing scripts, the placement of the `Write-Debug` statements can significantly affect your debugging process. It’s best to place them at critical points in your script, such as before or after significant actions or conditional checks. Here’s a simple example of a script leveraging `Write-Debug`:
function Test-Debug {
$myVariable = "Hello, PowerShell!"
Write-Debug "Debugging myVariable: $myVariable"
}
In this example, `Write-Debug` provides insight into the value of `$myVariable`.
Real-World Use Cases
`Write-Debug` is particularly useful in situations involving loops or conditional statements. For instance, when iterating over a collection, adding debug messages can help you track progress and find potential issues:
foreach ($item in $items) {
Write-Debug "Processing item: $item"
}
Here, each iteration logs the current item being processed, making it much easier to identify where something might be going wrong.
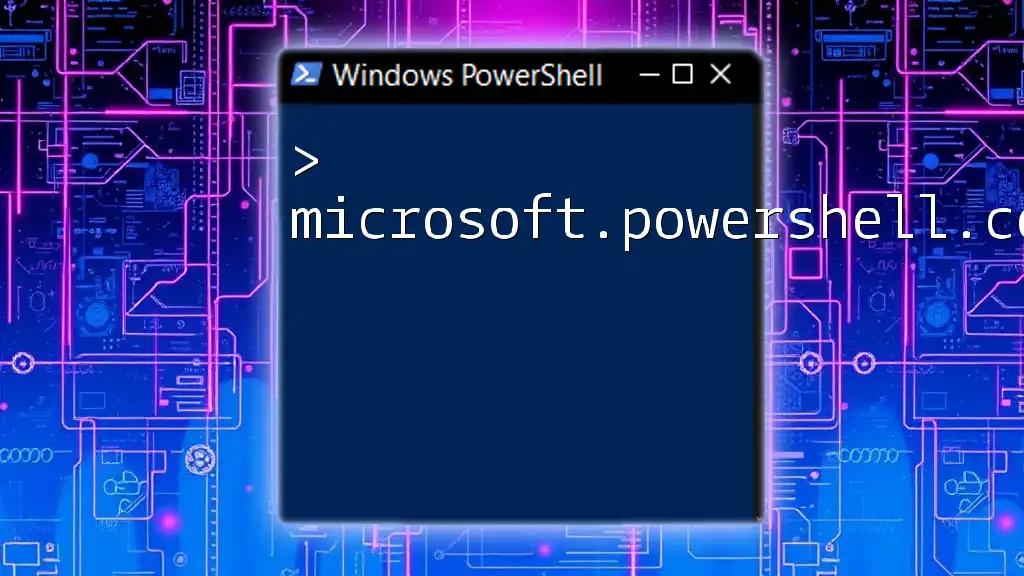
Handling Output from Write-Debug
Viewing Debug Outputs
To see the messages generated by `Write-Debug`, ensure your `$DebugPreference` is set appropriately. When enabled, you will see the debug messages directly in the console where your script is executing.
Redirecting Debug Outputs
If you want to keep a record of your debug messages or analyze them later, you can redirect the output to a file using `Start-Transcript`. Here’s an example:
Start-Transcript -Path "C:\Logs\debug.log"
Write-Debug "This will go to the log file"
Stop-Transcript
This technique allows you to maintain a persistent log of your debug sessions for future reference.
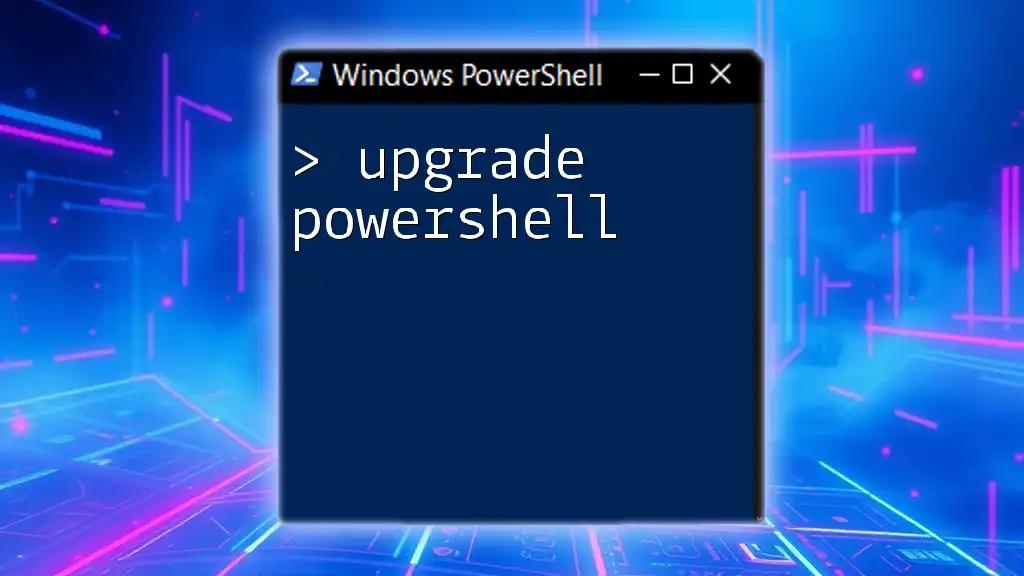
Common Mistakes and Best Practices with Write-Debug
Common Mistakes When Using Write-Debug
Frequently, users forget to change the `$DebugPreference` variable. Another common mistake is neglecting to format debug messages clearly, leading to confusion during troubleshooting. Always ensure you’re using a consistent and descriptive message format.
Best Practices for Effective Debugging
To maximize the utility of `Write-Debug`, consider the following best practices:
- Use concise messages: Craft short, yet informative messages that can be quickly understood.
- Employ variable values: Include variable values within your debug messages to provide context.
- Leverage conditional debugging: Use `if` statements around `Write-Debug` calls to limit output based on certain conditions, reducing clutter.
Implementing these strategies will make your debugging process more efficient and effective.
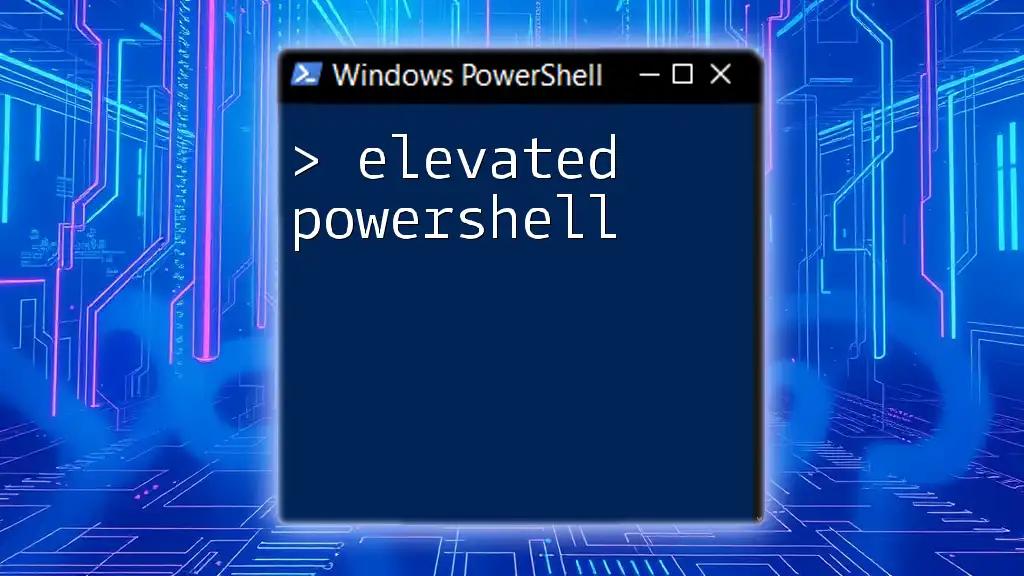
Conclusion
In summary, `Write-Debug` is an invaluable tool in your PowerShell arsenal, greatly enhancing the debugging process. By allowing developers to output meaningful debug messages without affecting the main output, it provides clarity and control during script development. Adopting its use and following the best practices discussed can lead to cleaner, more maintainable scripts and a smoother troubleshooting experience.
Additional Resources
For further learning, consider diving into PowerShell documentation or exploring online forums and tutorials dedicated to exploring deeper functionalities and best practices. Continue experimenting with `Write-Debug`, and you’ll soon find it to be an essential part of your PowerShell toolkit.