Selenium is a powerful tool that can be leveraged in PowerShell to automate web browser interactions, allowing users to control and manipulate web applications programmatically.
# Example: Launching a Chrome browser and navigating to a webpage using Selenium in PowerShell
Add-Type -Path "path\to\Selenium.WebDriver.dll"
$driver = New-Object OpenQA.Selenium.Chrome.ChromeDriver
$driver.Navigate().GoToUrl("https://www.example.com")
Setting Up Your Environment
Installing PowerShell
To begin your journey with Selenium and PowerShell, ensure you have PowerShell installed on your machine. The installation process varies by operating system.
-
For Windows, PowerShell comes pre-installed. To check, simply search for "PowerShell" in your Start menu.
-
For macOS and Linux, you can install PowerShell via Homebrew or the package manager for your respective distribution. For example, on macOS, you can use:
brew install --cask powershell
Installing Selenium WebDriver for PowerShell
After ensuring PowerShell is installed, you need to install the Selenium WebDriver. This is typically done via NuGet, a package management tool for .NET.
- Open PowerShell as an administrator.
- Run the following command to install the Selenium module:
Install-Package Selenium.WebDriver
Choosing a Browser Driver
Selenium interacts with various web browsers through browser drivers. The most common driver is the ChromeDriver for Google Chrome, but there are drivers for Firefox (GeckoDriver), Edge, and Safari as well.
To set up ChromeDriver:
- Download it from the [official ChromeDriver download page](https://chromedriver.chromium.org/downloads).
- Ensure that the driver’s executable is accessible in your system's PATH. This allows PowerShell to locate it when executing commands.
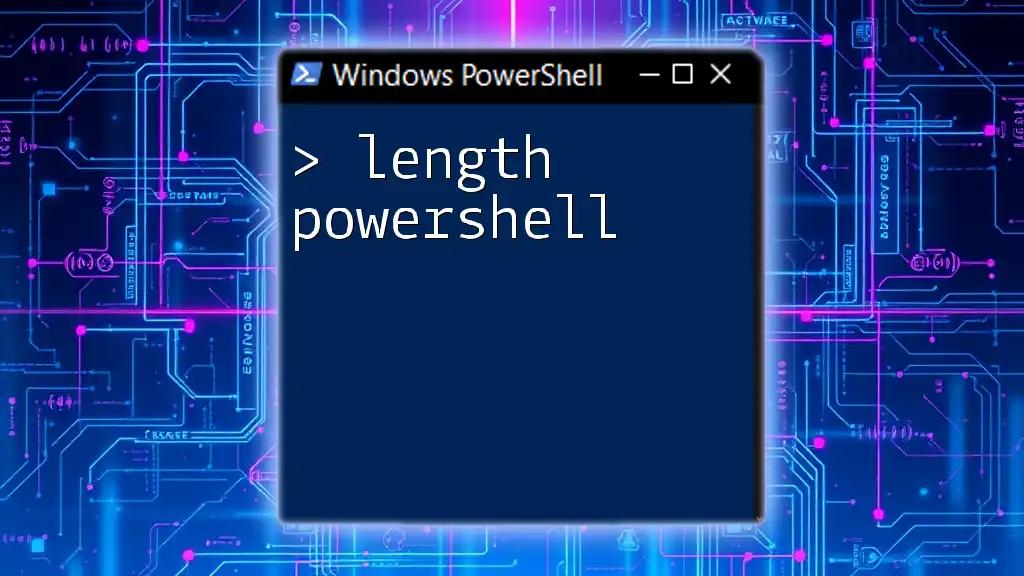
Writing Your First Selenium Script
Creating a New PowerShell Script
You can begin writing a PowerShell script using any text editor, but it is recommended to use an IDE or editor like Visual Studio Code for syntax highlighting and additional features.
Launching a Browser
Let’s start by launching a browser. Below is the example code to initialize and launch Google Chrome using Selenium:
# Import Selenium WebDriver
Add-Type -Path "C:\path\to\WebDriver.dll"
# Initialize Chrome Driver
$driver = New-Object OpenQA.Selenium.Chrome.ChromeDriver
Replace `"C:\path\to\WebDriver.dll"` with the actual path where you have installed the Selenium WebDriver.
Navigating to a Web Page
To navigate to a specific web page, use the `Navigate().GoToUrl()` method. Here’s how to navigate to example.com:
$driver.Navigate().GoToUrl("https://www.example.com")
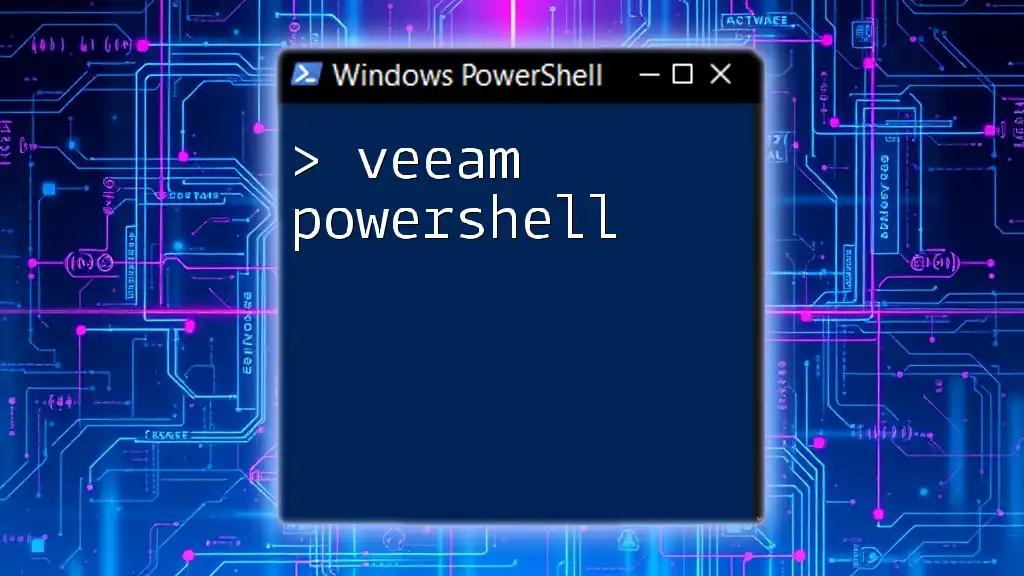
Interacting with Web Elements
Locating Elements on a Web Page
Selenium allows you to access elements on the page through various methods. Here are some commonly used methods to find elements:
- `FindElementById`
- `FindElementByName`
- `FindElementByXPath`
For instance, if you want to locate a submit button by its ID:
$button = $driver.FindElementById("submit-button")
Performing Actions on Elements
After locating elements, you can perform actions on them, such as clicking buttons or entering text. To click the button found in the previous code:
$button.Click()
Extracting Data from Web Elements
You can also retrieve text or attributes from web elements. For example, to extract a welcome message:
$text = $driver.FindElementById("welcome-message").Text
Write-Output $text
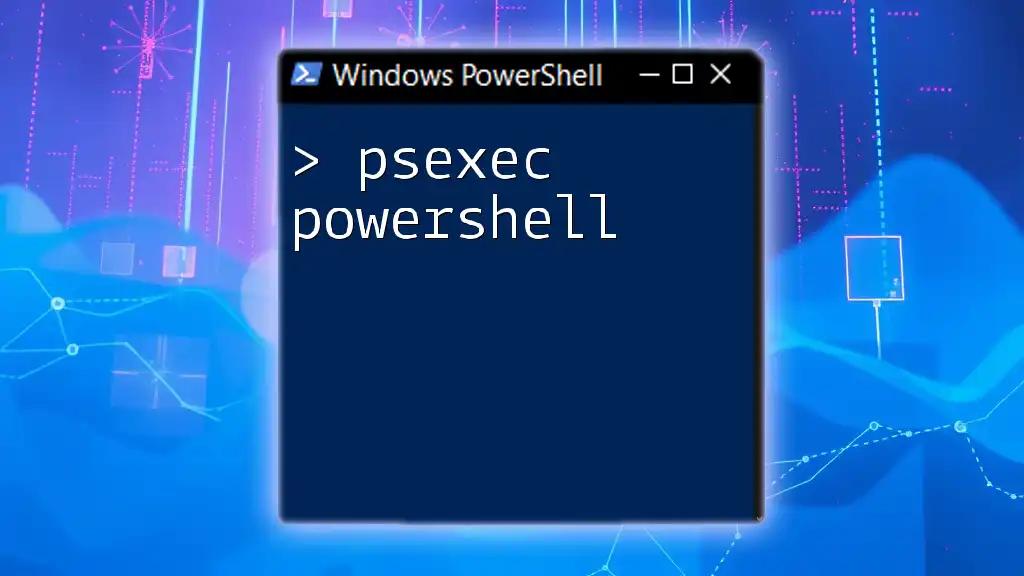
Advanced Selenium Techniques
Handling Alerts and Pop-ups
JavaScript alerts and other pop-ups can be managed through Selenium. To accept a simple alert:
$driver.SwitchTo().Alert().Accept()
Working with Frames and Windows
Switching between frames or handling multiple windows can be crucial for web automation. Use `SwitchTo().Frame()` or `SwitchTo().Window()` methods accordingly.
Executing JavaScript with PowerShell
Sometimes, you might need to run JavaScript for certain functionalities. To scroll the page down, for example:
$driver.ExecuteScript("window.scrollTo(0, 500);")
This powerful feature allows you to interact with web pages at a finer level.
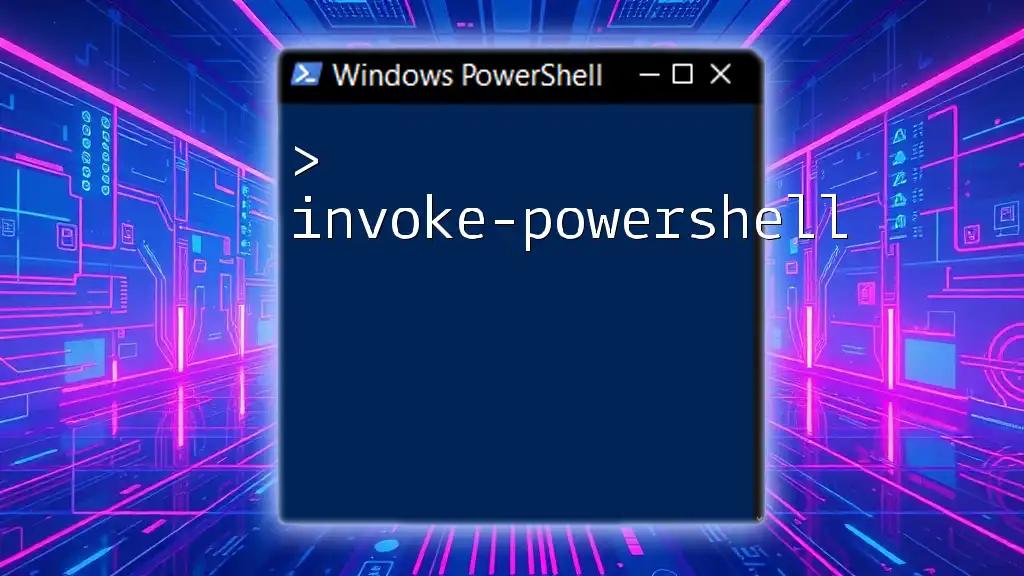
Error Handling and Debugging
Common Errors in Selenium Scripts
Selenium scripts may encounter various errors. Timed out exceptions or elements not found are among the most common. Understanding these errors is critical for effective debugging.
Best Practices for Debugging
Using try/catch blocks is an excellent way to handle exceptions in your scripts. This allows you to manage unexpected errors gracefully:
try {
$driver.Navigate().GoToUrl("https://www.example.com")
} catch {
Write-Output "An error occurred: $_"
}
By managing errors, your script remains robust and user-friendly.
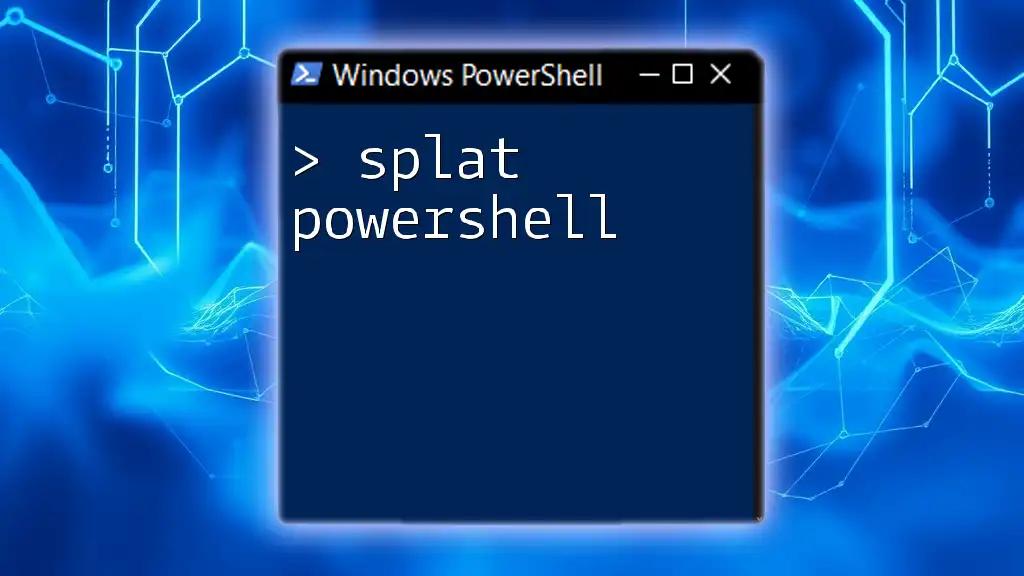
Closing the Browser and Cleaning Up
Quitting the WebDriver
Once your automation tasks are complete, you should properly close the browser to free up resources. To quit the WebDriver session:
$driver.Quit()
It is also good practice to call this method to ensure all instances are closed and resources are released.
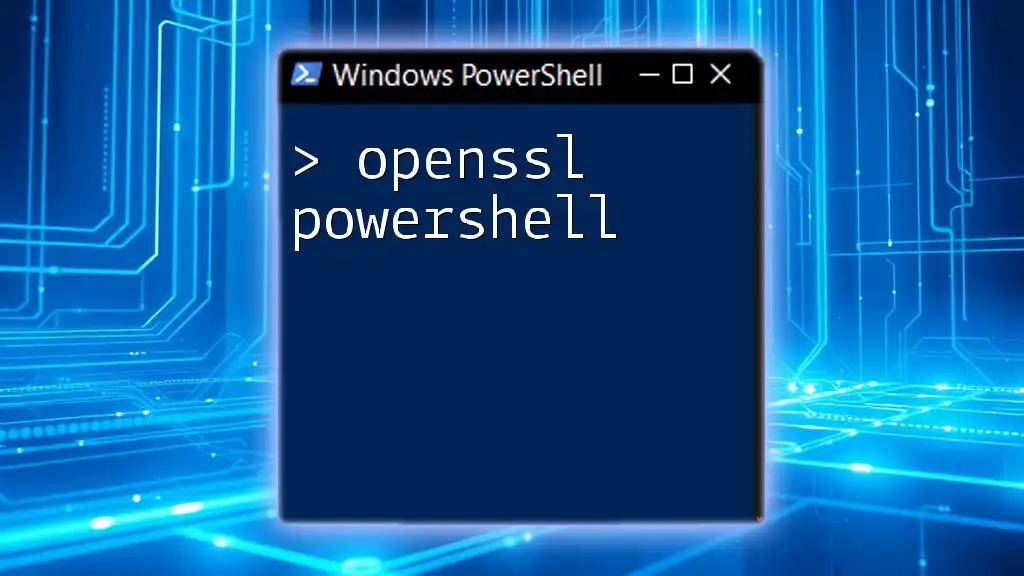
Example Projects and Use Cases
Web Scraping with Selenium and PowerShell
Web scraping involves extracting data from websites, which can be ethically questionable. However, when done responsibly, it can provide valuable insights. You can create a simple script to scrape data from a sample site by automating browsing actions and retrieving text elements.
Automating Form Submission
One of the most practical applications of Selenium is automating form submissions. By filling out forms programmatically, you can save time and reduce human error, especially for repetitive tasks such as testing or data entry.
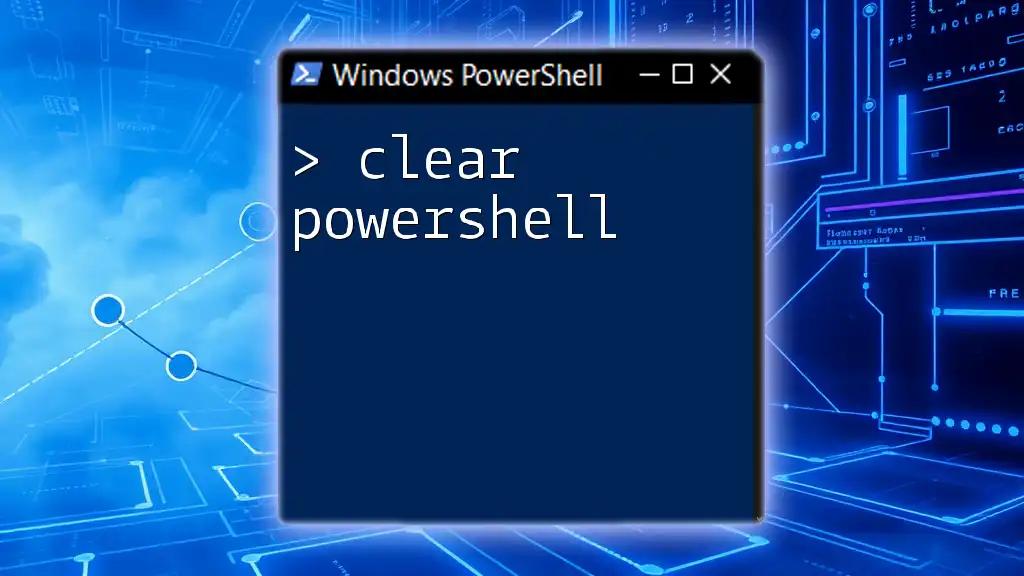
Conclusion
This comprehensive guide to Selenium with PowerShell has equipped you with the fundamental skills to automate web tasks effectively. From setting up your environment to handling complex web interactions, the possibilities are extensive. Keep exploring the rich capabilities of Selenium and PowerShell to unlock new levels of automation in your workflows.
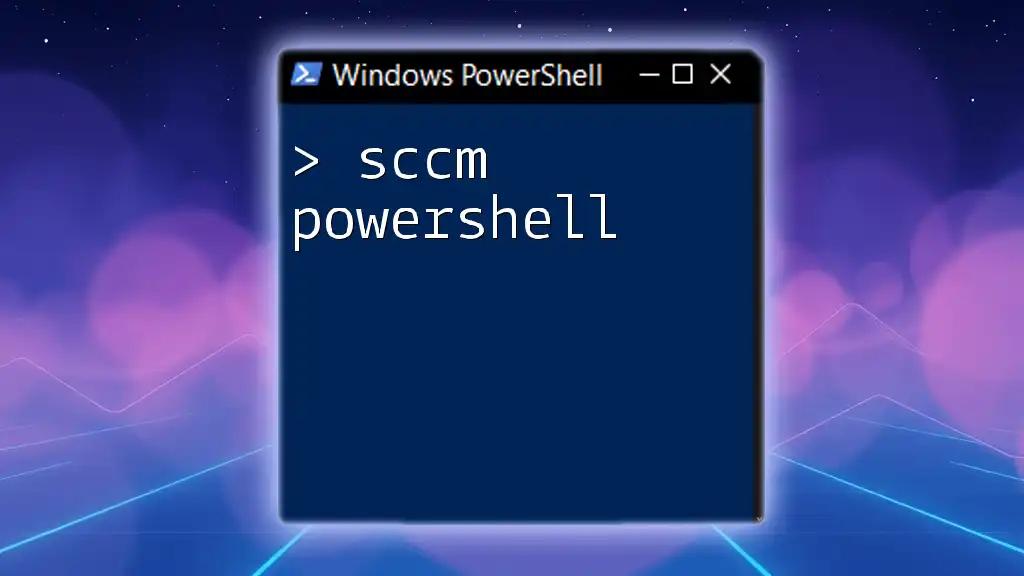
Resources
To further your understanding and mastery of Selenium and PowerShell, consider checking the following resources:
- Selenium Documentation: [Selenium HQ](https://www.selenium.dev/documentation/en/)
- PowerShell Documentation: [Microsoft Docs](https://docs.microsoft.com/en-us/powershell/)
- Community Forums: Engage with active forums like Stack Overflow and GitHub for community support and shared projects.
This guide serves as a solid foundation for anyone looking to dive into the world of automation with Selenium and PowerShell!