The `Tail` command in PowerShell is used to display the last few lines of a file, allowing users to monitor log files in real-time, similar to the Unix `tail` command.
Here’s a code snippet to demonstrate how to tail a log file using PowerShell:
Get-Content "C:\path\to\your\logfile.log" -Tail 10 -Wait
Understanding the Tail Command in PowerShell
What is the Tail Command?
The tail command originated from Unix/Linux systems, providing users with the ability to view the last few lines of a text file. It is particularly useful for monitoring log files in real-time—an essential practice for system administrators and developers. In PowerShell, there isn't a dedicated `tail` command, but the functionality is effectively realized through the `Get-Content` cmdlet.
The PowerShell Equivalent of Tail
Although PowerShell does not have a built-in `tail` command, you can achieve similar results using `Get-Content`, which retrieves content from a file. The command, combined with specific parameters, allows you to tail a file by viewing its latest entries, particularly useful for logs that are rapidly updated.
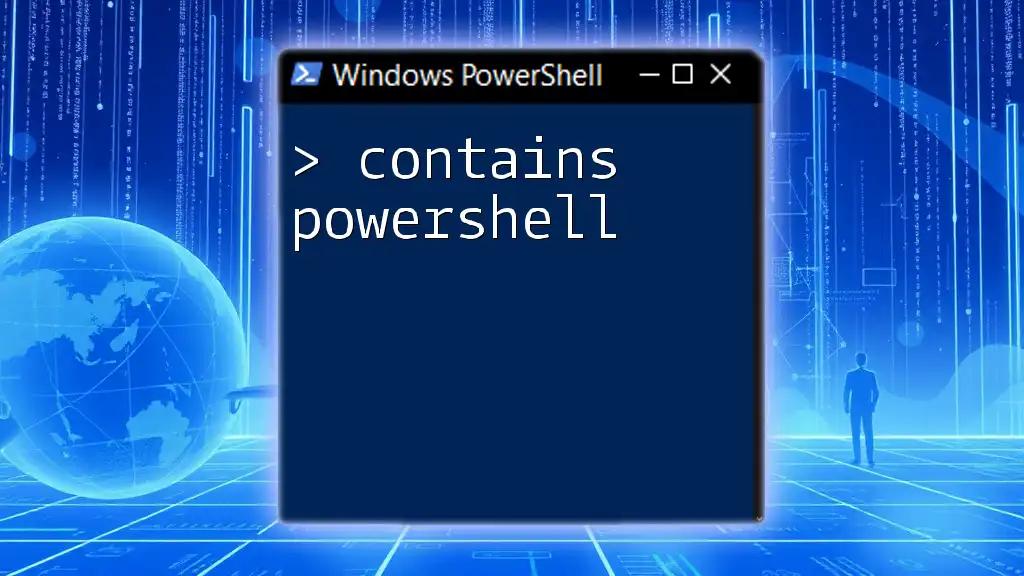
Tail Log PowerShell: Basic Usage
How to Tail a Log File in PowerShell
To tail a log file in PowerShell, you can use the `Get-Content` cmdlet with the `-Tail` and `-Wait` parameters. Here’s how:
Get-Content -Path "C:\path\to\your\logfile.log" -Tail 10 -Wait
- `-Path` specifies the location of the log file.
- `-Tail 10` lets you view the last 10 lines of the file.
- `-Wait` keeps the command running, allowing you to see new log entries as they are written.
This command is crucial for real-time logging, enabling you to stay updated without constantly reopening the file.
Tail File PowerShell: Example Scenarios
Monitoring Application Logs
Consider an application that writes logs for troubleshooting. You can monitor these logs continuously with:
Get-Content -Path "C:\Logs\Application.log" -Tail 20 -Wait
This command helps developers quickly identify issues, as they can instantly see error messages or critical log entries without having to reload the log file.
Tail Logs during System Diagnostics
PowerShell also allows monitoring of system events, which can be essential during troubleshooting:
Get-Content -Path "C:\Windows\System32\winevt\Logs\Application.evtx" | Select-Object -Last 20
Utilizing the `Select-Object -Last 20` command allows you to obtain the most recent entries from an event log file.
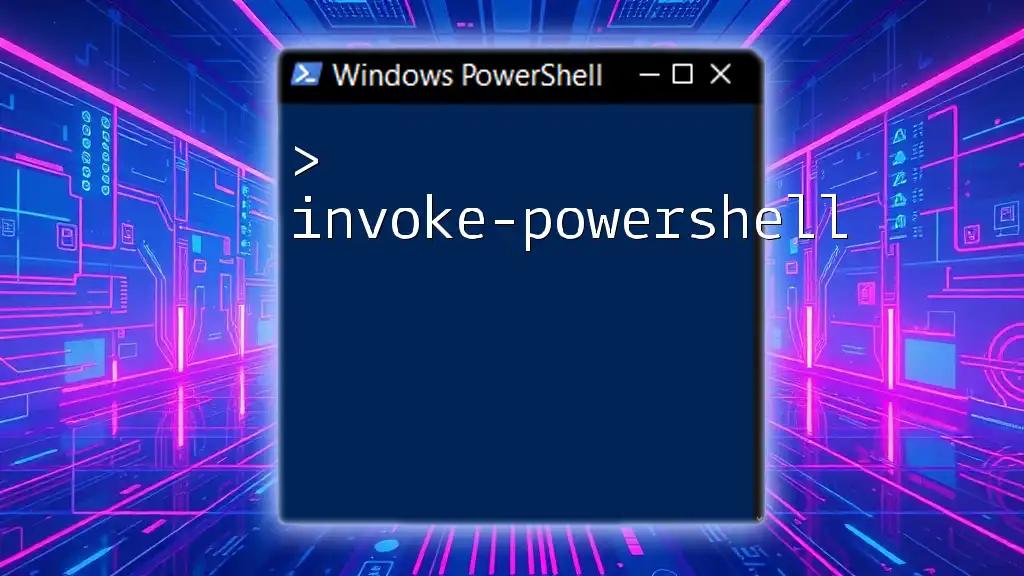
Advanced Use Cases for PowerShell Tail
Filtering Content While Tailing Logs
You can refine your log monitoring experience by filtering specific terms, such as errors. For instance:
Get-Content -Path "C:\path\to\your\logfile.log" -Tail 10 -Wait | Where-Object { $_ -match "Error" }
This command enables you to focus on error messages, thereby enhancing the efficiency of your diagnostics.
Using Wildcards with Tail File PowerShell
If you are dealing with multiple log files, you can utilize wildcards to tail several logs at once:
Get-Content -Path "C:\logs\*.log" -Tail 5 -Wait
This command makes monitoring easier when working with applications that generate multiple log files, allowing for a centralized view of all relevant logs.
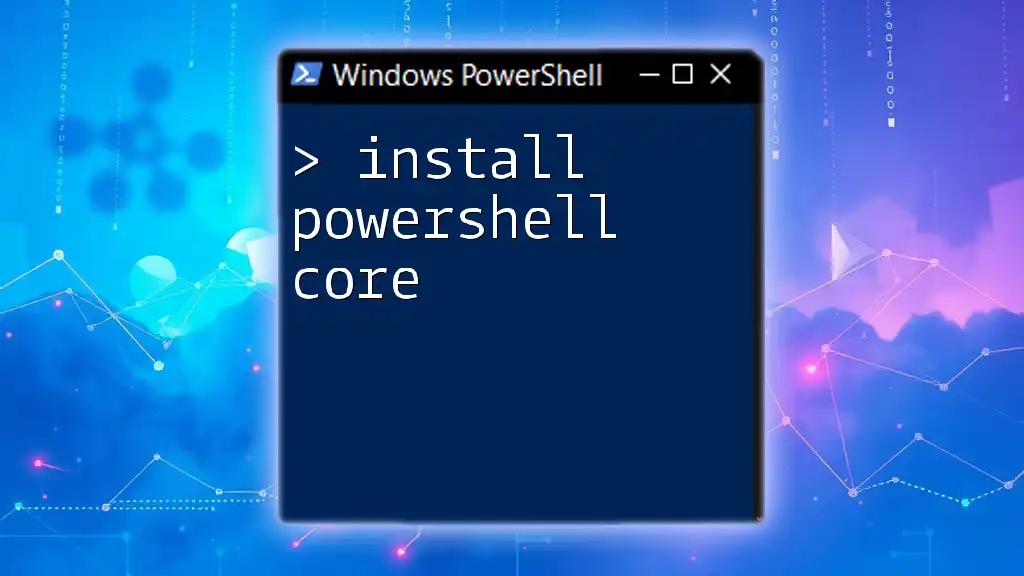
Using Windows PowerShell Tail in Scripts
Automating Log Tailing
You can automate your log monitoring by creating a PowerShell script. Here's a simple example:
$logPath = "C:\path\to\your\logfile.log"
Get-Content -Path $logPath -Tail 10 -Wait | Out-File -FilePath "C:\path\to\output.log" -Append
This script continuously tails the specified log file and appends the output to `output.log`. Automating log monitoring significantly reduces manual efforts and improves response time to critical log events.
Scheduling Tail Commands with Task Scheduler
If you want to regularly monitor logs without manual intervention, consider scheduling your PowerShell script using Windows Task Scheduler. This allows you to run your monitoring script at a set time or interval, ensuring you never miss out on important log entries.
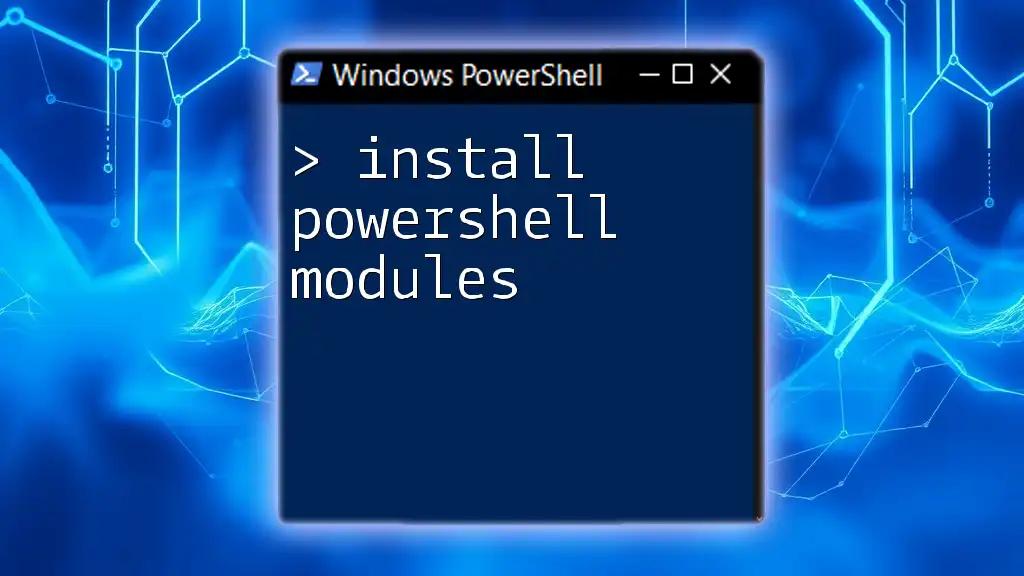
Best Practices for Tailing Logs in PowerShell
Performance Considerations
When tailing logs, be aware of performance implications, especially with large log files. To optimize performance:
- Use the `-Tail` parameter to limit the amount of data being processed.
- Consider rotating log files to prevent any single log file from consuming excessive resources.
Security and Permissions
Always understand file permissions when accessing log files, as security is paramount. Here are a few guidelines:
- Ensure your scripts run with the necessary permissions to access the log files.
- Regularly review who has access to log files, as they may contain sensitive information.

Conclusion
By mastering the `tail log powershell` functionality, you can greatly enhance your ability to monitor and analyze log files efficiently. Whether you're troubleshooting an application or diagnosing system issues, PowerShell provides the tools needed to keep track of logs in real time. Use the techniques outlined in this guide to improve your log management practices and take full advantage of PowerShell's capabilities.
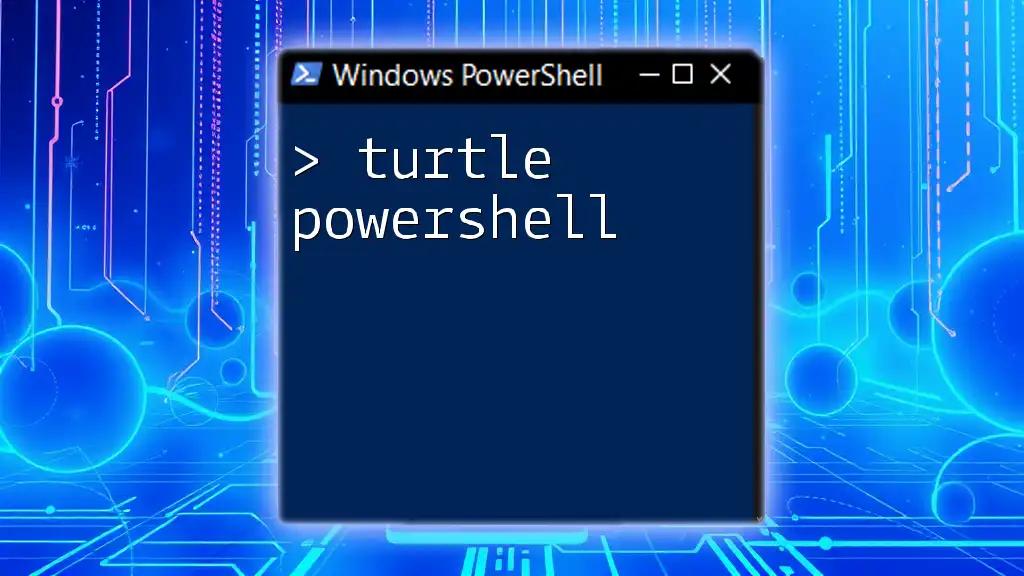
Additional Resources
Further Reading and Learning
For more in-depth understanding, refer to the official PowerShell documentation. Online courses and PowerShell user communities can also provide valuable knowledge and support as you dive deeper into PowerShell scripting.
Community and Support
Engage with PowerShell communities through forums or social media platforms. Sharing your experiences and learning from others can significantly enhance your proficiency and help you stay updated with the latest best practices and techniques.