ServiceNow PowerShell refers to the integration of PowerShell scripting with ServiceNow's API to automate tasks and manage IT services efficiently.
Here’s a simple example to query incidents from ServiceNow using PowerShell:
$servicenowInstance = "https://yourinstance.service-now.com"
$user = "username"
$password = "password"
$uri = "$servicenowInstance/api/now/table/incident"
$credential = [System.Convert]::ToBase64String([System.Text.Encoding]::ASCII.GetBytes(("$user:$password")))
$response = Invoke-RestMethod -Uri $uri -Method Get -Headers @{Authorization=("Basic {0}" -f $credential)}
$response.result | Select-Object number, short_description
What is ServiceNow?
ServiceNow is a powerful cloud-based platform that specializes in automating IT service processes and enhancing the overall productivity of organizations. It provides a comprehensive suite of tools designed to streamline various IT operations, including incident management, problem management, change management, and configuration management. The platform is built on an intuitive interface that allows IT professionals to resolve issues swiftly, track service requests, and maintain service levels consistently.
Key features of ServiceNow include:
- ITSM (IT Service Management): Central to ServiceNow's functionality, enabling efficient management of IT services.
- Automation: Automates repetitive tasks, reducing the likelihood of human error.
- Customization: Offers extensive customization options to fit specific organizational needs.
The importance of ServiceNow in IT is profound, as it enables organizations to align IT services with business needs, ensuring quick delivery of applications and services.
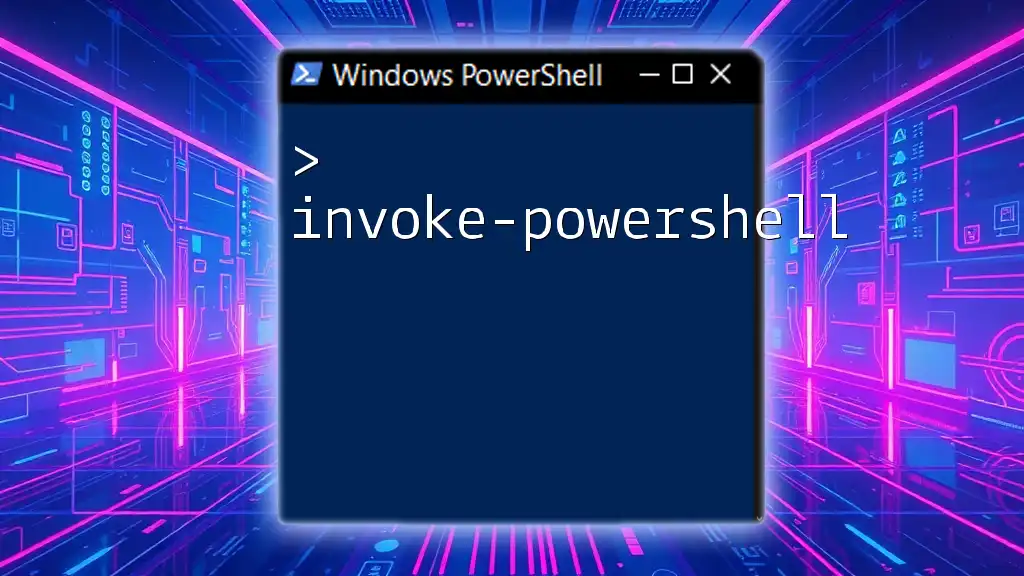
What is PowerShell?
PowerShell is a task automation and configuration management framework developed by Microsoft. It consists of a command-line shell and an associated scripting language. Over the years, PowerShell has evolved to become one of the essential tools in the IT professional's arsenal, notably because of its ability to interact seamlessly with various systems and applications.
The significance of PowerShell lies in its ability to automate complex processes through scripting. IT operations rely heavily on PowerShell to manage system configurations, retrieve data, and execute batch commands efficiently. Its integration with many applications also allows for effective management of resources across both local and remote systems.
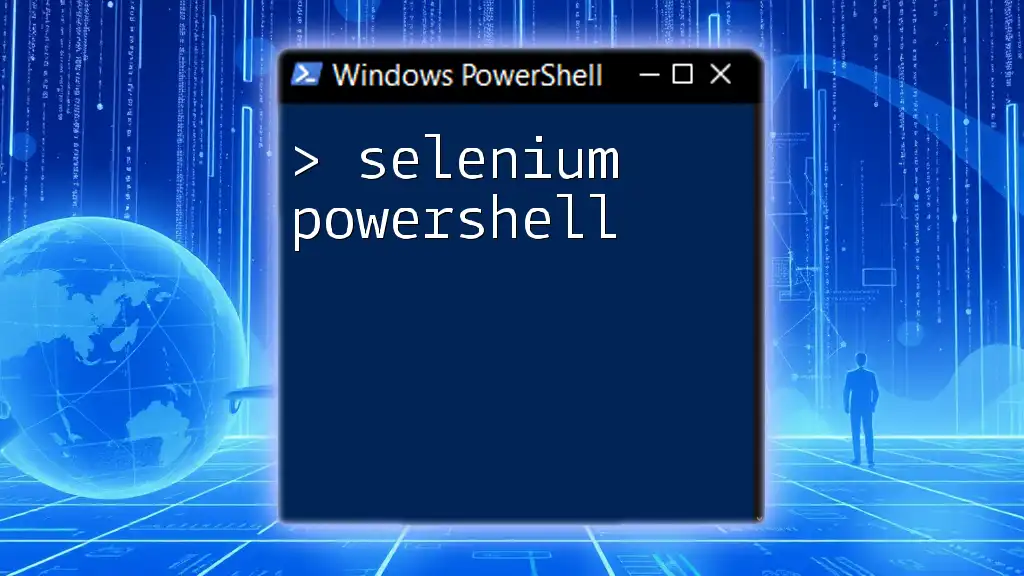
The Intersection of ServiceNow and PowerShell
Why Integrate PowerShell with ServiceNow?
Integrating PowerShell with ServiceNow offers a range of benefits that can significantly enhance IT operations:
- Automation: Automate routine tasks, such as creating or updating incidents, directly from PowerShell scripts.
- Streamlined Processes: Facilitate a more efficient workflow by executing multiple PowerShell commands that can trigger multiple actions in ServiceNow.
- Reduced Errors: Automating tasks minimizes human errors and improves the consistency of processes.
With the combination of ServiceNow's robust service management capabilities and PowerShell's flexibility, organizations can develop custom solutions that expedite operations.
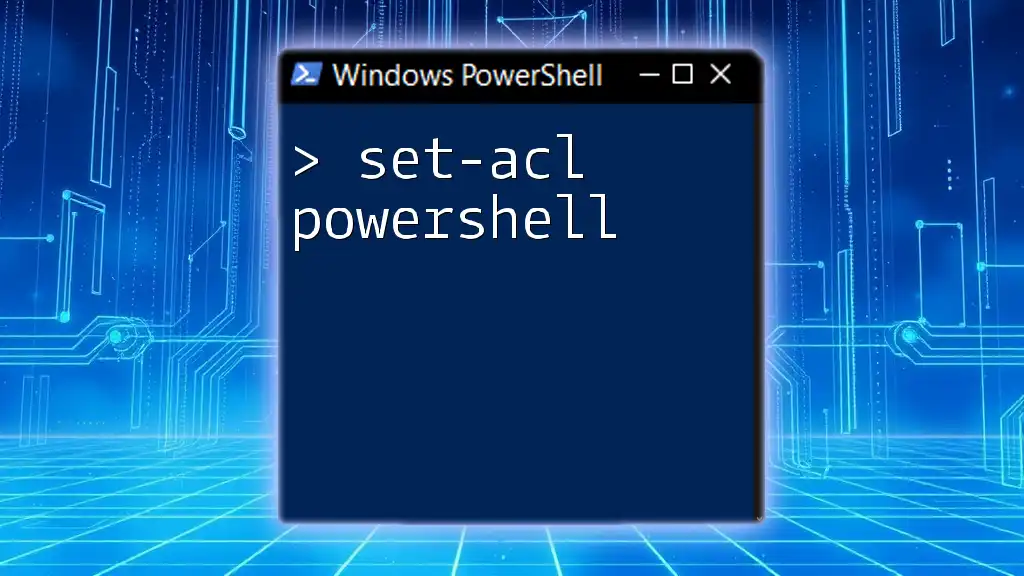
Setting Up Your Environment
Prerequisites
Before you can begin using PowerShell with ServiceNow, ensure that you have the necessary tools and software in place:
- ServiceNow Instance: Make sure you have access to a ServiceNow instance, preferably a developer instance for testing.
- PowerShell: Ensure that you have PowerShell installed on your operating system. PowerShell is natively installed on Windows; for Linux or Mac, it can be downloaded from the official Microsoft site.
Configuring ServiceNow API
To use PowerShell with ServiceNow, you need to set up API access:
- Understand the ServiceNow API: The ServiceNow REST API allows you to interact programmatically with the ServiceNow platform.
- Create a ServiceNow Developer Account: If you don’t have an account, sign up for a Developer account on the ServiceNow Developer site.
- Generate API Credentials: You must have appropriate user roles to access the API. Login to your ServiceNow instance and navigate to your user profile to access 'API Credentials' or create a new user specifically for API access with appropriate permissions.
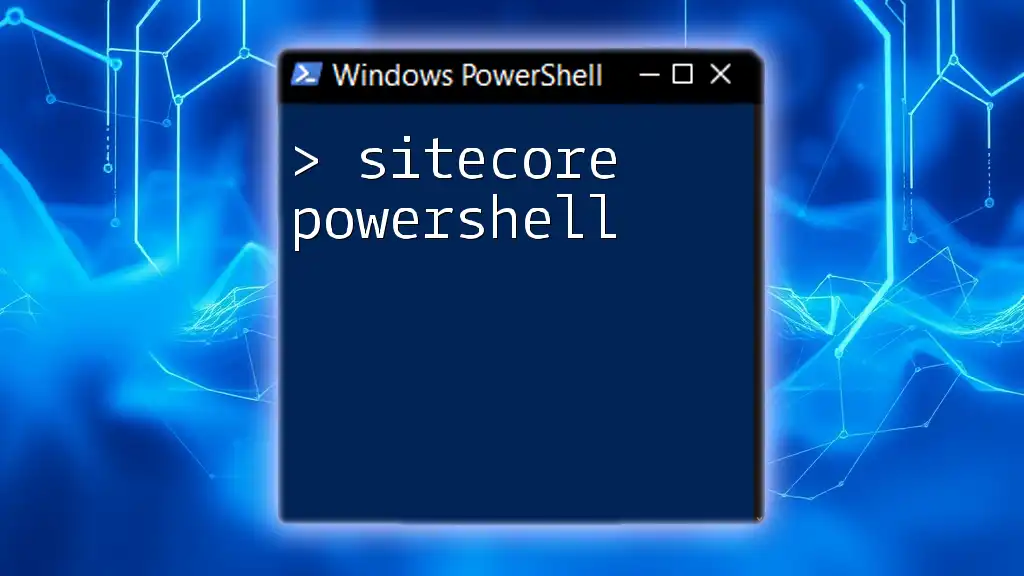
Basic PowerShell Commands for ServiceNow
Connecting to ServiceNow
To initiate a connection to ServiceNow using PowerShell, you can use the following script:
# Example connection script
$ServiceNowInstance = "https://yourinstance.service-now.com"
$User = "your_username"
$Pass = ConvertTo-SecureString "your_password" -AsPlainText -Force
$Credential = New-Object System.Management.Automation.PSCredential($User, $Pass)
$Session = New-PSSession -Uri $ServiceNowInstance -Credential $Credential -Authentication Basic
This script establishes a session with your ServiceNow instance using basic authentication. Ensure that you replace `"yourinstance.service-now.com"`, `"your_username"`, and `"your_password"` with your actual ServiceNow instance details.
Retrieving Data from ServiceNow
Using REST API to Query Incident Records
You can retrieve incident records from ServiceNow using the following command:
# Example command to get incidents
$Endpoint = "yourinstance.service-now.com/api/now/table/incident"
$Response = Invoke-RestMethod -Uri $Endpoint -Method Get -Credential $Credential
This command invokes a GET request to the incident table in your ServiceNow instance, returning all existing incidents. You can further manipulate or filter this response according to your needs.
Creating a New Incident in ServiceNow
If you want to automate the creation of a new incident in ServiceNow, you can use PowerShell as follows:
# Example command to create an incident
$NewIncident = @{
short_description = "Test Incident"
description = "This is a test incident created via PowerShell"
}
Invoke-RestMethod -Uri $Endpoint -Method Post -Credential $Credential -Body ($NewIncident | ConvertTo-Json) -ContentType "application/json"
In this example, a new incident is created with a short description and a detailed description. The body of the request is formatted in JSON.
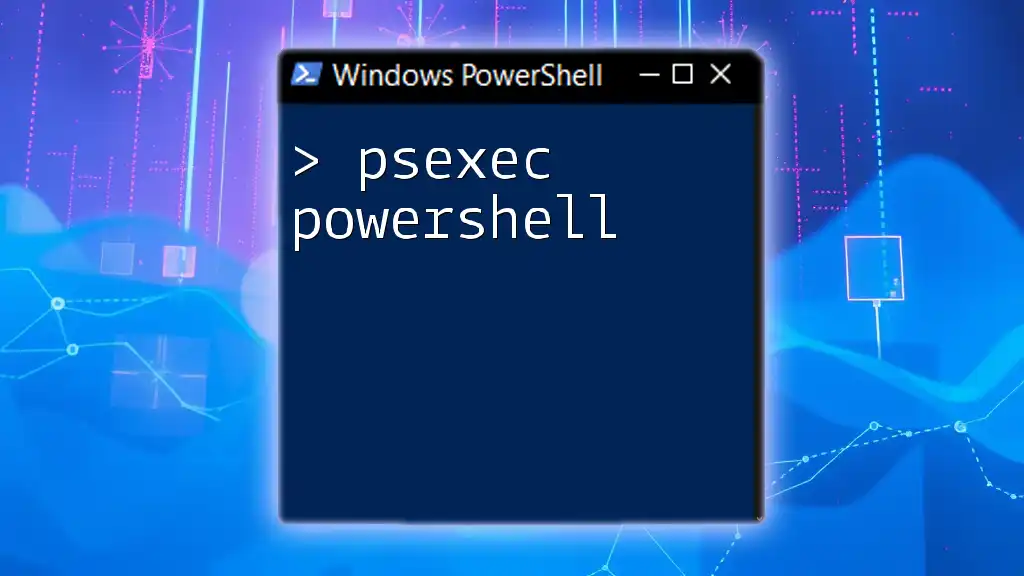
Working with ServiceNow Tables
Understanding ServiceNow Tables
ServiceNow uses a relational database structure where information is stored in tables. Common tables include Incident, Change, Problem, and Configuration Item (CI). Familiarize yourself with the table structure to efficiently manage data through PowerShell.
Fetching Specific Table Data
To fetch data from the Incident table and select specific fields, you can use:
# Example command to fetch specific fields from incidents
$Fields = "number,short_description"
$EncodedFields = [System.Web.HttpUtility]::UrlEncode($Fields)
$QueryEndpoint = "$Endpoint?sysparm_fields=$EncodedFields"
$Incidents = Invoke-RestMethod -Uri $QueryEndpoint -Method Get -Credential $Credential
This script retrieves incident records while specifically requesting the incident number and short description, thereby optimizing data handling.
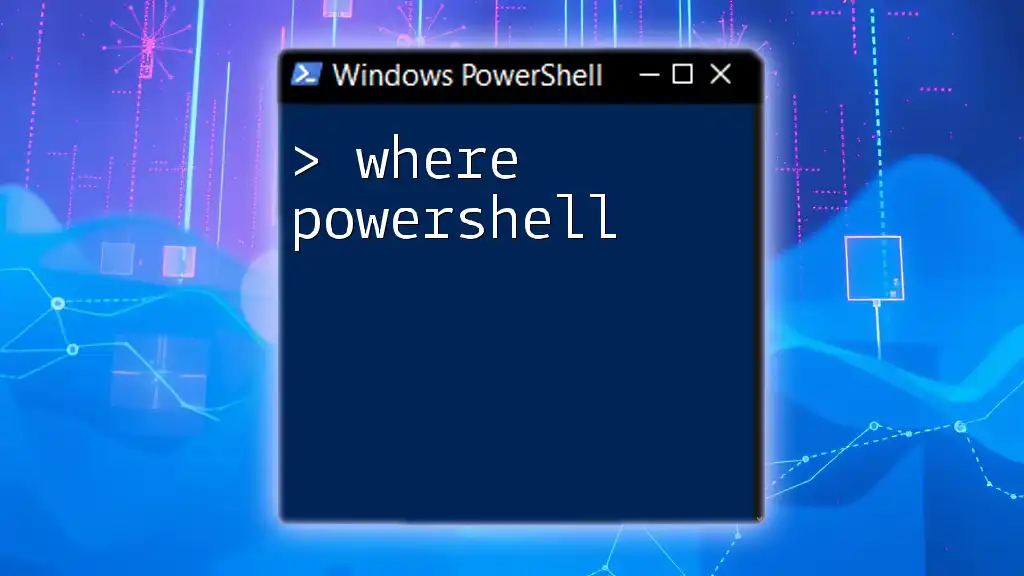
Advanced PowerShell Techniques
Error Handling and Debugging
Error handling is paramount when working with APIs. Implementing a Try-Catch block allows you to catch and manage errors effectively:
# Error handling example
try {
$Response = Invoke-RestMethod -Uri $Endpoint -Method Get -Credential $Credential
} catch {
Write-Host "An error occurred: $_"
}
This structure helps ensure your scripts run smoothly, and you receive relevant feedback regarding any issues.
Scheduling and Automation
You can utilize Windows Task Scheduler to run PowerShell scripts at specific intervals:
- Open Task Scheduler and create a new task.
- Set the trigger, choose “New,” and select a schedule that fits your needs.
- Under “Actions,” choose “Start a program” and enter `powershell.exe` in the Program/script field.
- Add your script path in the “Add arguments” field.
This setup allows the automation of ServiceNow tasks, such as regularly reviewing incidents.
Logging and Monitoring Script Outputs
Logging is crucial for tracking the execution of your scripts. You can implement simple logging functionalities within your PowerShell script as shown below:
# Example logging function
function Log-Output {
param (
[string]$Message
)
Add-Content -Path "C:\Logs\ServiceNow_PowerShell.log" -Value "$(Get-Date): $Message"
}
Logging important events helps in troubleshooting and maintaining transparency regarding script execution.
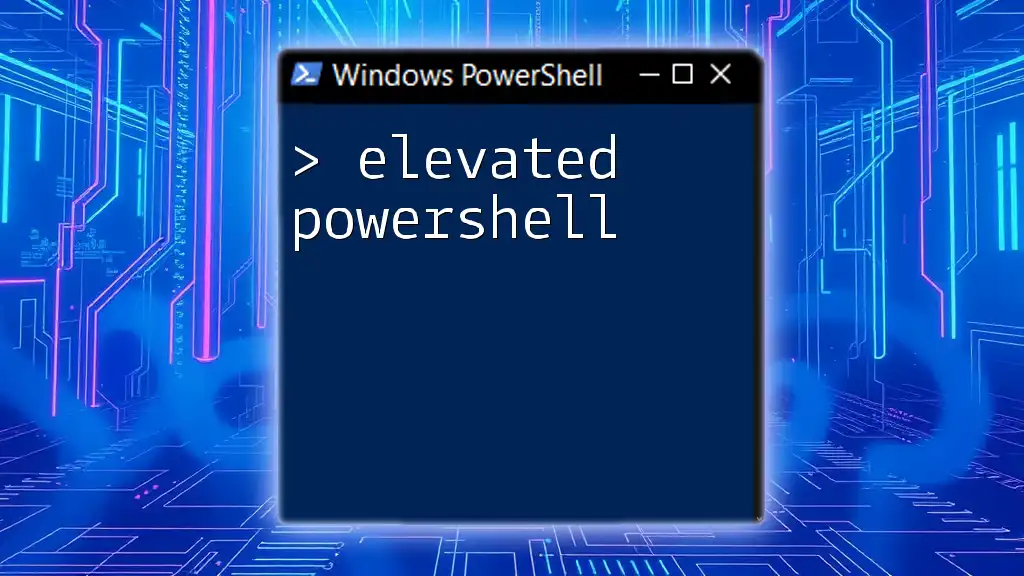
Best Practices
Security Considerations
When handling sensitive data and credentials, always prioritize security. Instead of using plain-text passwords in scripts, consider using OAuth or storing passwords securely in Windows Credential Manager.
Commenting and Documentation
Properly comment your code to enhance readability and maintainability. Documentation is essential for you or anyone else who may revisit the script in the future. Include a header at the start of your scripts detailing its functionalities, usage, and any dependencies.
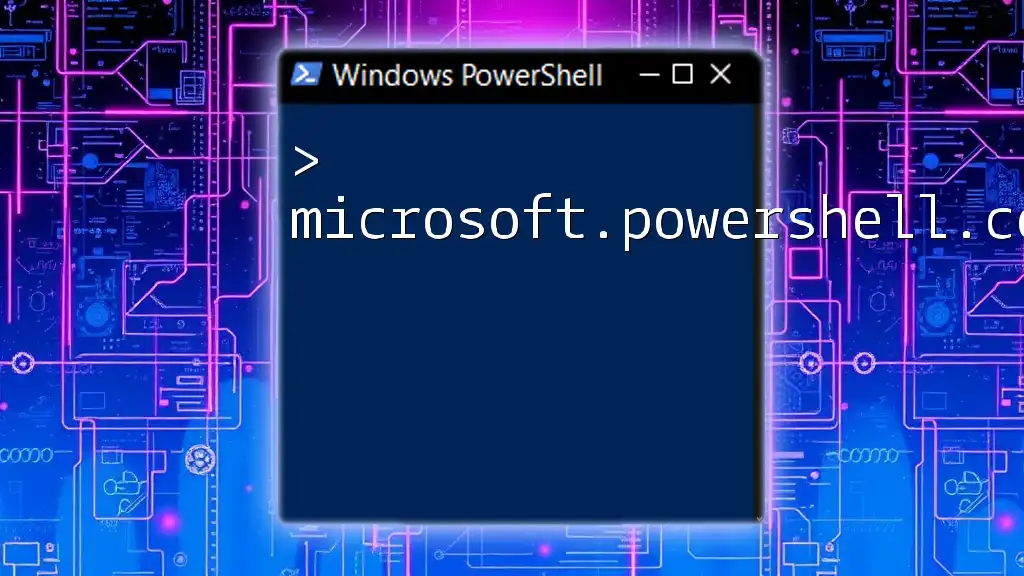
Conclusion
Integrating PowerShell with ServiceNow can significantly enhance your IT operations through automation and efficient data management. With the right setup and scripts, routine tasks can become streamlined, ensuring faster resolution and better service delivery. As you explore the capabilities of ServiceNow PowerShell, tailor your scripts according to your organization's needs, keeping security and best practices in mind.
Next Steps
Continue deepening your understanding of both PowerShell and ServiceNow by exploring additional resources, experimenting with various API calls, and engaging with communities that focus on automation and service management. The flexibility of PowerShell combined with the robust functionality of ServiceNow opens up an array of possibilities to enhance your IT workflows.