The `New-WebServiceProxy` cmdlet in PowerShell 7 allows users to create a proxy object for a web service, enabling easy access to its methods and properties.
Here’s a simple example of how to use it:
$proxy = New-WebServiceProxy -Uri "http://www.example.com/service.asmx?WSDL"
Understanding Web Services
What is a Web Service?
A web service is a standardized way of integrating web-based applications using open standards over an internet protocol backbone. These services can be broadly classified into two types: SOAP (Simple Object Access Protocol) and REST (Representational State Transfer).
- SOAP: A protocol for exchanging structured information in the implementation of web services.
- REST: An architectural style that uses standard HTTP methods for communication and is typically easier to consume.
Benefits of Using Web Services
Web services enable interoperability, allowing different systems to communicate regardless of platform or language. Additionally, they support reusability of code, giving developers the ability to compose complex operations using existing services. Lastly, they provide easy access to a vast array of APIs, enhancing the capabilities of applications.
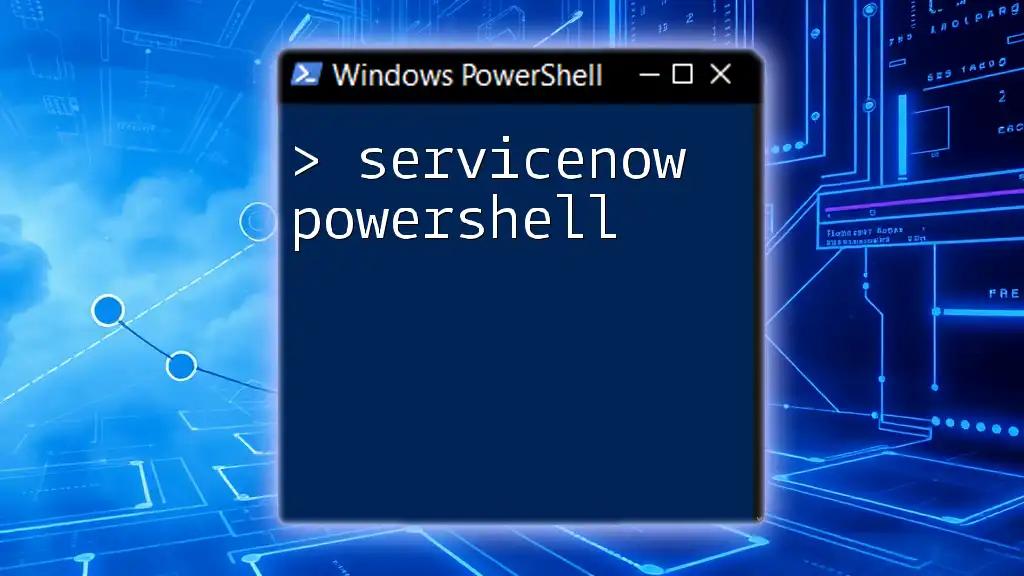
Getting Started with New-WebServiceProxy
Prerequisites
Before you start, ensure you have PowerShell 7 installed, and you possess a basic understanding of PowerShell commands. You will also need access to a web service with a defined WSDL (Web Services Description Language).
Syntax of New-WebServiceProxy
The syntax of the New-WebServiceProxy command is both straightforward and flexible, allowing various options to customize your service interaction:
New-WebServiceProxy -Uri <Uri> [-Credential <PSCredential>] [-UseDefaultCredential] [-Namespace <String>] [-OutVariable <String>] [-PassThru] [-Asynchronous] [-Comment <String>]
- Uri: Specifies the location of the WSDL file.
- Credential: Allows the use of specified authentication credentials.
- Namespace: Used for organizing related types in a PowerShell session.
- OutVariable: Stores the output in a specified variable for later use.
Common Parameters Explained
Understanding the parameters is essential for effective usage:
-
Uri: The Uniform Resource Identifier that points to the web service's WSDL. For example:
$wsdl = "http://www.example.com/service?wsdl"
-
Credential: This allows you to specify credentials for a web service that requires authentication. Use the Get-Credential cmdlet to securely input your username and password.
-
Namespace: By defining a namespace, you can prevent naming conflicts between multiple services or methods. This is useful in larger scripts or when consuming multiple web services.
-
OutVariable: Assigning an output variable can help you keep track of the service proxy, which is later useful for making method calls.
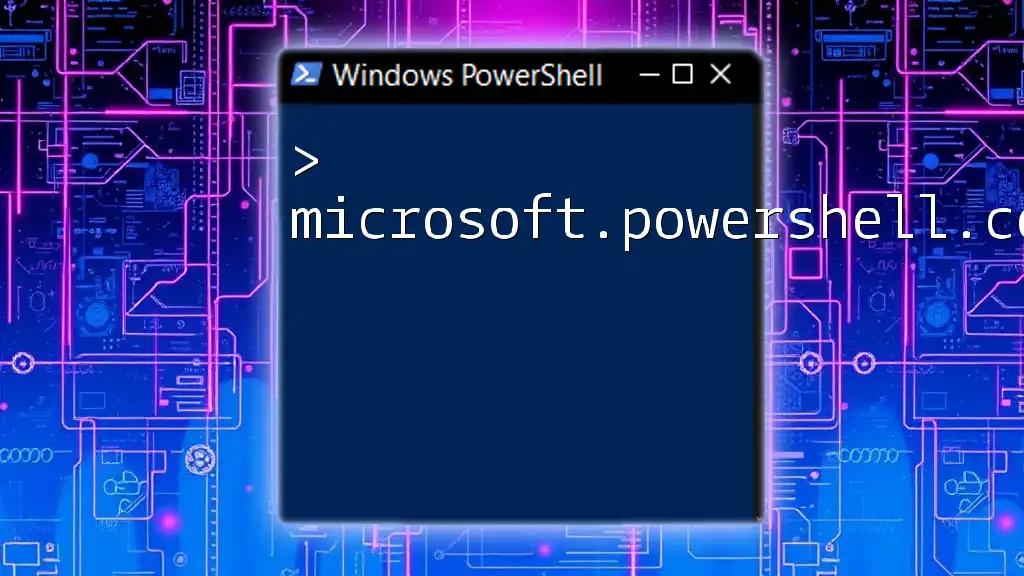
Creating a Web Service Proxy
Step-by-Step Guide
Step 1: Fetching the WSDL
Before you can use New-WebServiceProxy, you need the WSDL file. This file tells PowerShell how to interact with the web service.
Step 2: Running New-WebServiceProxy
To create a proxy object that you can use to call methods, utilize the following command:
$proxy = New-WebServiceProxy -Uri $wsdl
Step 3: Calling a Web Service Method
Once you have your proxy, you can invoke methods defined in the WSDL:
$result = $proxy.MethodName($parameter1, $parameter2)
Step 4: Error Handling
When working with web services, errors can occur. Use a try-catch block to handle exceptions gracefully:
try {
$result = $proxy.MethodName()
} catch {
Write-Error "An error occurred: $_"
}
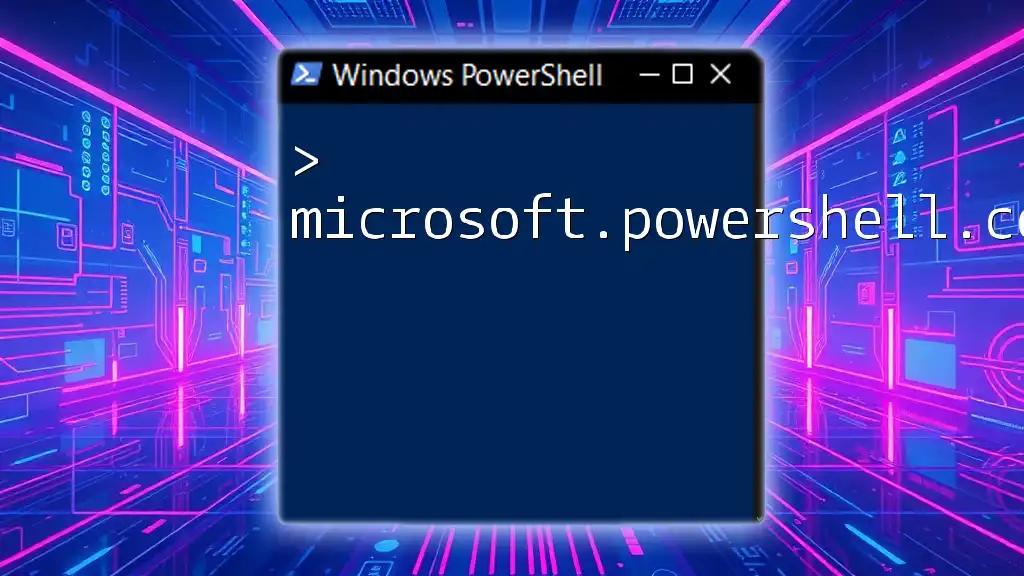
Practical Examples
Example 1: Consuming a SOAP Web Service
In this example, we will interact with a SOAP web service. First, define the WSDL URL and then create the proxy:
$wsdl = "http://example.com/service?wsdl"
$service = New-WebServiceProxy -Uri $wsdl
$response = $service.SomeMethodName('parameter')
After calling the method, it's crucial to analyze the response to ensure it meets your expectations. You can log or output the result with:
Write-Output $response
Example 2: Consuming a REST Web Service
For REST-based services, New-WebServiceProxy is not suitable. Instead, you can leverage the Invoke-RestMethod cmdlet. Here's a straightforward example of calling an API endpoint:
$response = Invoke-RestMethod -Uri "http://example.com/api/resource" -Method Get
Write-Output $response
The response, often in JSON format, can be handled easily in PowerShell. For instance, you can convert it to a PowerShell object for further manipulation.
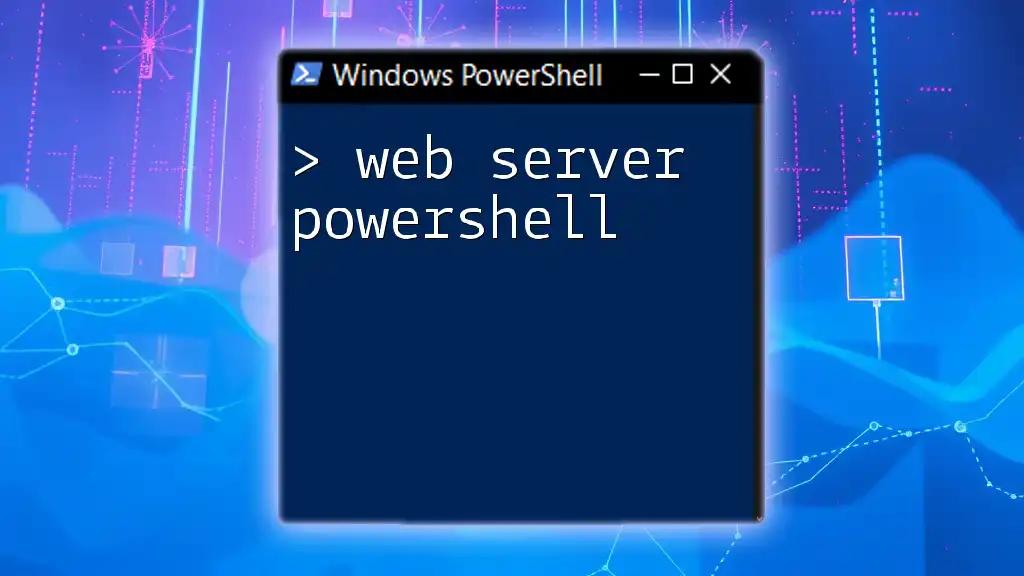
Advanced Usage
Customizing the Web Service Proxy
Once you've established a proxy, you might want to customize its behavior. This includes setting specific namespaces to keep your workspace organized or modifying parameters to fine-tune how your commands execute.
Incorporating Error Handling
Incorporating error handling into your script is crucial. A well-structured error management system can help detect issues early and provide meaningful feedback to the user. Always use Try-Catch to manage exceptions effectively when invoking web services.
Performance Considerations
When working with web services that may involve session management, consider using persistent sessions where appropriate, especially if you are frequently calling a service that maintains state. Optimize performance further by caching responses or reusing service proxy instances when possible.
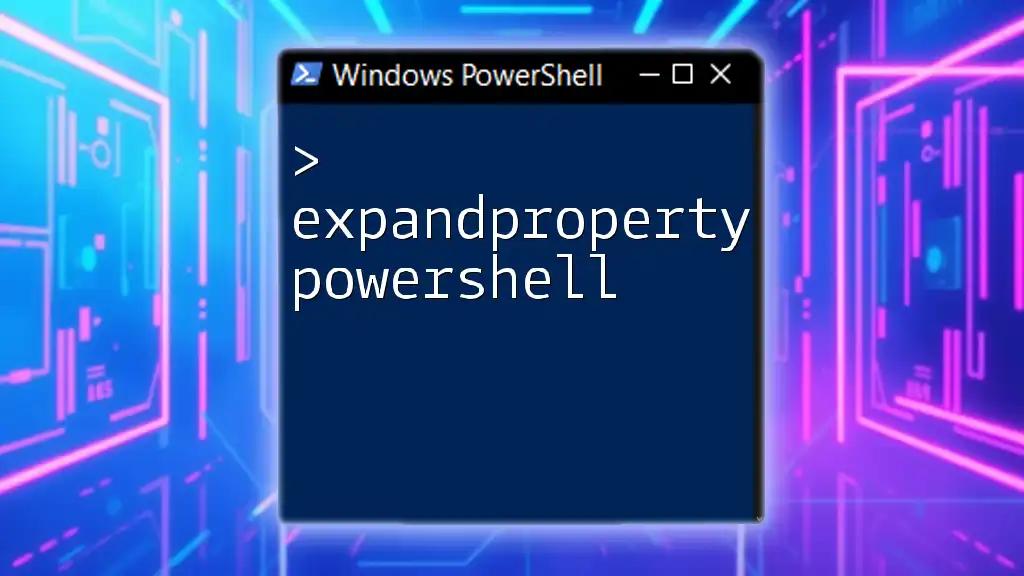
Troubleshooting Common Issues
Common Errors and Solutions
When working with New-WebServiceProxy, you might encounter connectivity issues or receive "method not found" errors. Verify that the WSDL is accessible and ensure the method names and parameters match the ones defined in the WSDL.
Debugging Techniques
Utilize PowerShell's built-in debugging tools to step through your script. The Set-PSBreakpoint cmdlet lets you pause execution and examine values, making it easier to diagnose issues in real time.
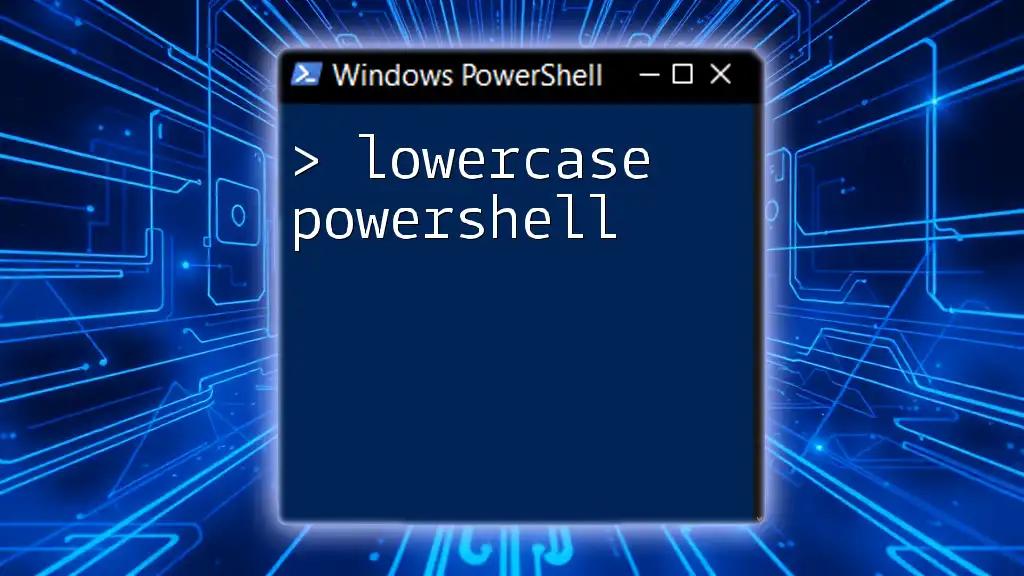
Conclusion
Recap of Key Points
Navigating New-WebServiceProxy in PowerShell 7 opens up a world of possibilities for consuming web services. From setting up your proxy and invoking methods to handling responses and errors, you have everything you need to start harnessing the power of web services effectively.
Where to Learn More
For those eager to expand their knowledge, explore the official Microsoft documentation on PowerShell and web services. Various forums and online communities also provide excellent resources for learning and troubleshooting.
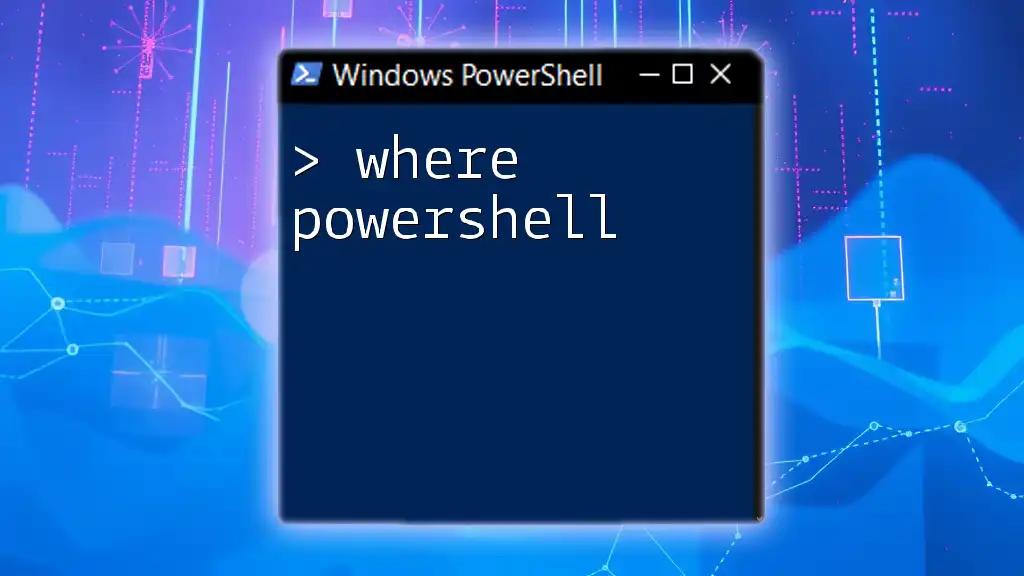
Call to Action
Start practicing with New-WebServiceProxy today; experiment with different web services and enhance your PowerShell skills. Join our learning platform for more tutorials, hands-on training, and to connect with fellow learners!