A web server in PowerShell can be quickly set up to host web applications or serve static content using simple commands.
Here’s a basic example of how to start a simple HTTP server using PowerShell:
# Start a simple HTTP server on port 8080
Start-Process powershell -ArgumentList "-NoProfile", "-Command", "while ($true) { Start-ListeningHttpServer -Port 8080 }"
This command initiates a loop that runs a simple HTTP server on port 8080, allowing you to serve content directly from your PowerShell environment.
Understanding Web Server Basics
Types of Web Servers
IIS (Internet Information Services)
IIS is Microsoft's web server platform, widely used in enterprise environments. PowerShell seamlessly integrates with IIS, allowing administrators to perform various tasks efficiently through scripting. Familiarity with its components, such as application pools and sites, facilitates management tasks.
Apache HTTP Server
Apache is an open-source web server that supports a wide range of configurations and features. While it's more commonly associated with Linux environments, it can also be run on Windows. Understanding how PowerShell interacts with Apache through command-line operations extends its utility beyond IIS.
Key Features of Web Servers
Hosting Websites
Web servers provide the backbone for hosting websites, whether via shared hosting, VPS, or dedicated servers. Shared hosting offers cost-effective solutions for small projects, while VPS and dedicated hosting are tailored for larger applications requiring more resources.
Handling Requests
Web servers operate by processing HTTP requests and returning responses. Understanding how this process works can help you configure your web server optimally for performance and security.
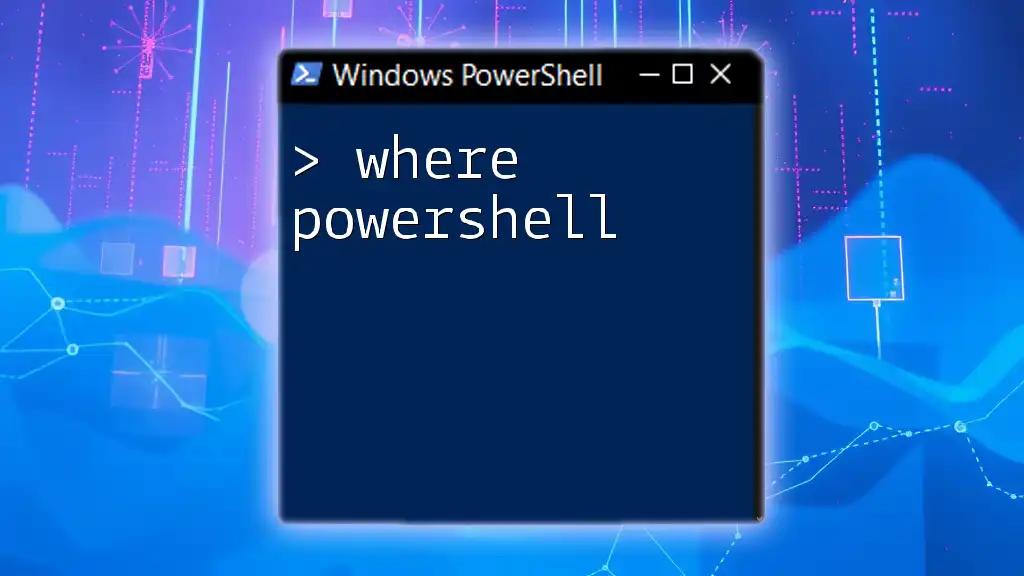
Setting Up PowerShell for Web Server Management
Prerequisites
Installing PowerShell
To begin, ensure you have PowerShell installed. You can download it from the official Microsoft website. It's essential to use the latest version to leverage new features and security improvements.
PowerShell Modules for Web Servers
For effective IIS management, you should leverage the `WebAdministration` module. This module contains a collection of cmdlets specifically designed for managing IIS, and you can import it using the following command:
Import-Module WebAdministration
Configuring PowerShell Remoting
Why Use Remoting?
PowerShell remoting allows you to execute commands on remote machines. This feature is vital when managing multiple web servers or handling servers that do not require physical access.
Setting Up Remoting
To enable PowerShell remoting, execute the following command in an elevated PowerShell session:
Enable-PSRemoting -Force
This command sets up the necessary configurations for remote management, ensuring that your servers can be controlled from a single interface.
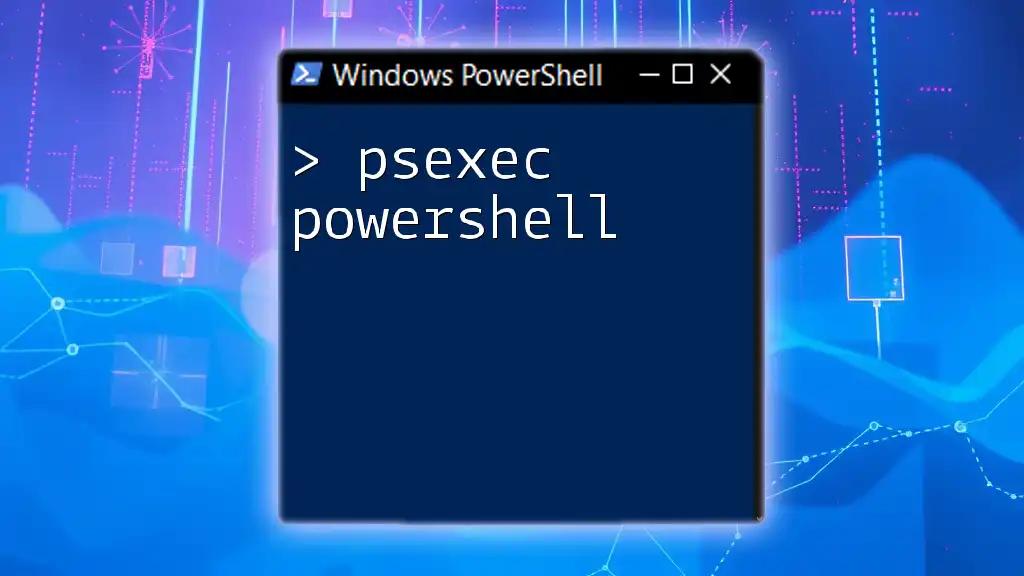
Using PowerShell with IIS
Basic IIS Management Commands
Starting and Stopping the IIS Service
Managing the IIS service can be done through PowerShell. To stop or start the service, use the following commands:
Stop-Service W3SVC
Start-Service W3SVC
These commands enable you to quickly stop or restart the web server as needed, which is particularly useful during maintenance.
Creating and Managing Websites
Creating a new website in IIS through PowerShell is straightforward. For example, to create a site named "MySite" that listens on port 80, run:
New-WebSite -Name "MySite" -Port 80 -PhysicalPath "C:\inetpub\MySite"
To view all websites, you can use:
Get-WebSite
And to remove a website, the command is:
Remove-WebSite -Name "MySite"
These commands empower you to manage your web properties effectively.
Managing Application Pools
Creating an Application Pool
Application pools are critical for managing multiple websites on the same server. You can create one using:
New-WebAppPool -Name "MyAppPool"
Starting and Stopping Application Pools
To manage the state of your application pools, use:
Start-WebAppPool -Name "MyAppPool"
Stop-WebAppPool -Name "MyAppPool"
Understanding application pools is essential for optimizing your web server's performance and resource allocation.
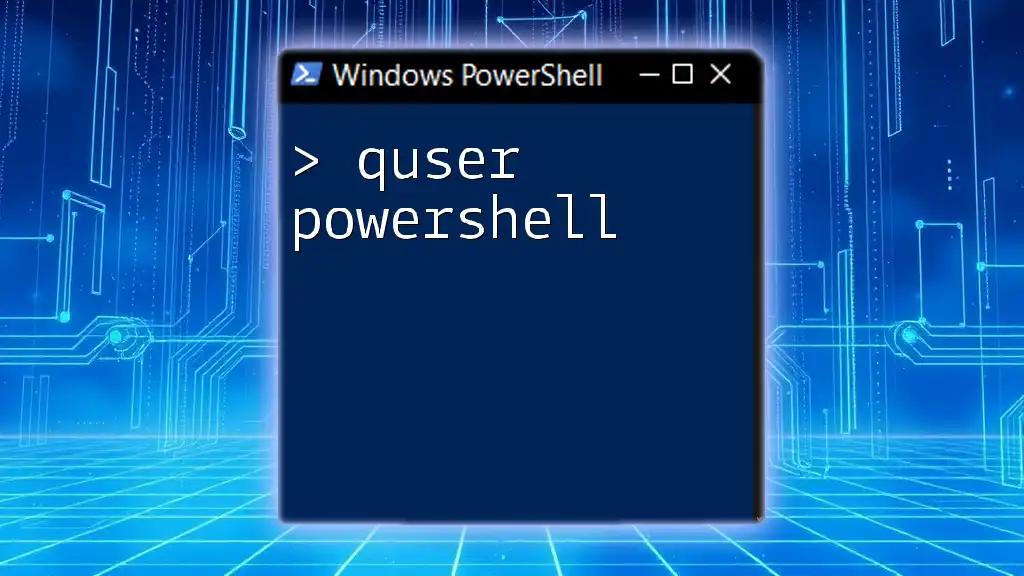
Advanced PowerShell Techniques for IIS
Scripting Batch Tasks
Creating a PowerShell Script to Back Up IIS Configurations
Automation simplifies management tasks. Here's a script to back up IIS configurations:
Export-WebApp -Name "MySite" -Path "C:\Backup\MySiteBackup.zip"
This command captures the current state of your website, preserving it for future reference.
Automating Website Deployment
With PowerShell, you can automate the deployment of multiple websites. Scripting removes manual effort, reduces errors, and saves significant time, especially when managing numerous sites.
Monitoring IIS Performance
Using Get-WebSiteStatistics
Monitoring website performance is crucial. Use the following command to check the state of your application pool:
Get-WebSite -Name "MySite" | Get-WebAppPoolState
This command provides insights into how well your website is performing and helps identify potential issues before they escalate.
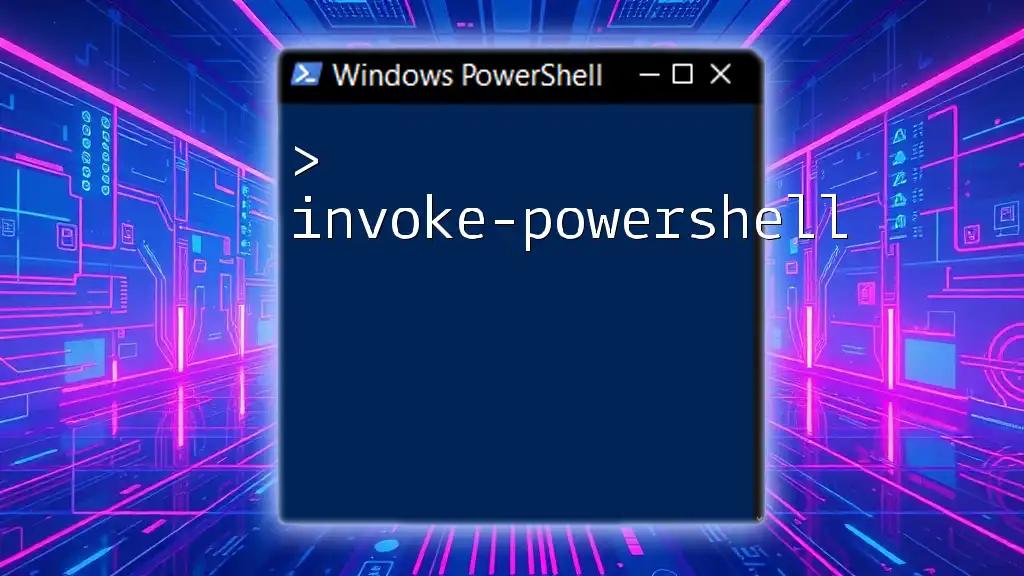
Using PowerShell with Apache
Installing Apache and PowerShell Tools
Installing Apache on Windows
Installing Apache on Windows is the first step to integrating it with PowerShell. Ensure that the installation path is added to your system's environment variables for easy access.
Managing Apache Configuration with PowerShell
Editing Configuration Files
You can modify Apache settings directly using PowerShell. For example, to edit the `httpd.conf` file, use:
Set-Content -Path "C:\Apache24\conf\httpd.conf" -Value "Updated Configuration"
This command allows for live configuration changes, ensuring that your web server adapts to new requirements promptly.
Restarting the Apache Service
After making configuration changes, the service must be restarted:
Stop-Service -Name "apache2.4"
Start-Service -Name "apache2.4"
Being familiar with these commands is essential for maintaining a smooth operational flow.
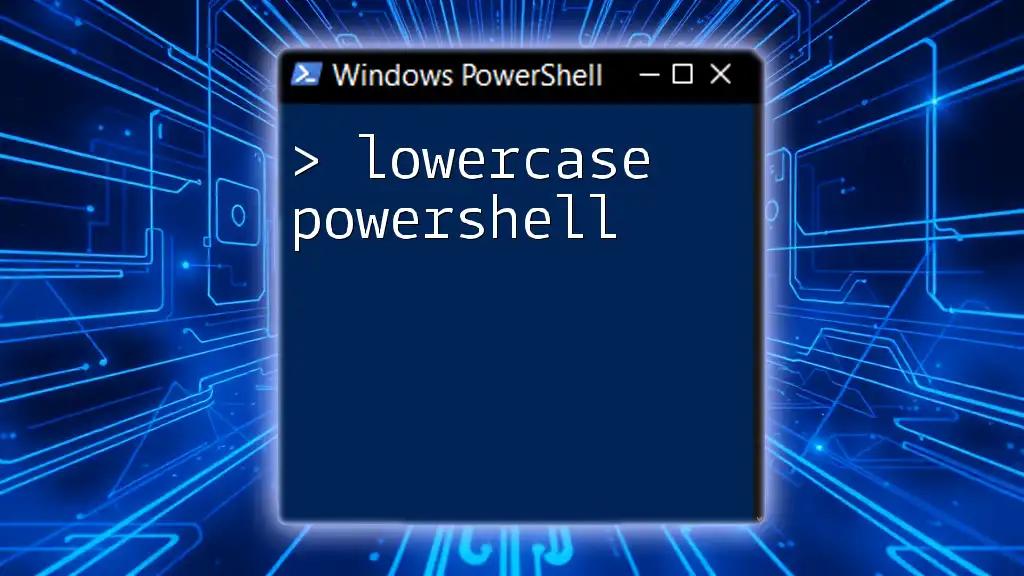
Troubleshooting and Common Issues
Common Problems with Web Servers
Website Not Responding
If a website is down, using PowerShell can provide valuable diagnostics. Leverage commands like `Test-Connection` to check server reachability:
Test-Connection -ComputerName "MyWebsite.com"
This command helps determine whether network issues are preventing access.
Debugging PowerShell Scripts
Using Write-Debug and Write-Host
During development, outputting debug information can help identify issues. Use `Write-Debug` to print debug messages within your scripts, ensuring you understand what's happening at each step.
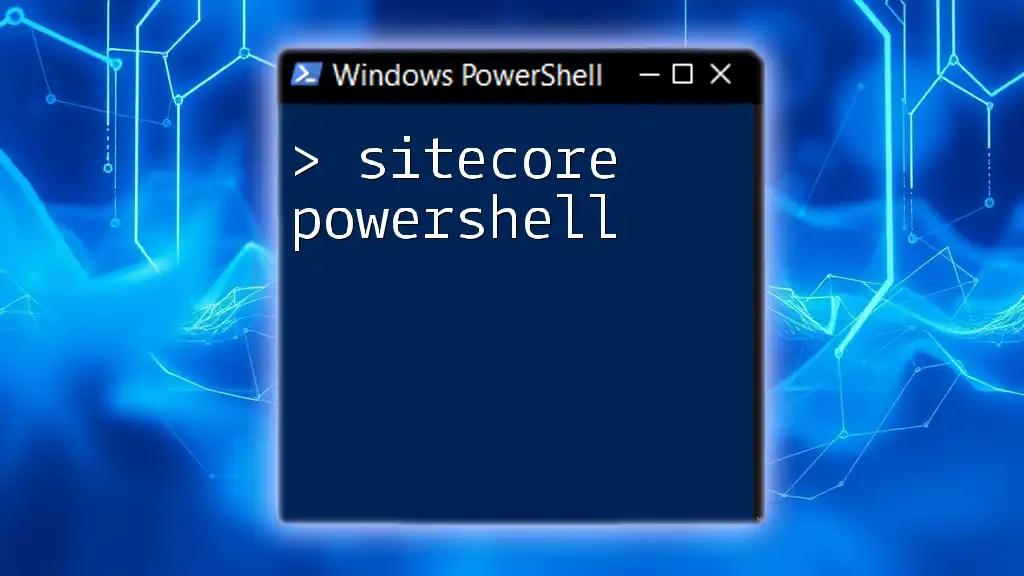
Conclusion
In summary, PowerShell offers powerful capabilities for managing web servers, specifically with IIS and Apache. With the right preparations and commands, you can automate, optimize, and troubleshoot your web server environment efficiently. As you delve deeper into PowerShell, the potential to enhance server management becomes boundless. By practicing these techniques and engaging with the community, you can master web server management through PowerShell.