In PowerShell, `null` represents a lack of value or an absence of an object, and it is often used to signify that a variable has not been assigned any data.
$variable = $null
if ($variable -eq $null) {
Write-Host 'The variable is null.'
}
Understanding Null in PowerShell
What Does Null Mean?
Null is a fundamental concept in programming that represents a lack of value. In PowerShell, understanding null is crucial because it can affect the behavior of scripts and functions. A variable that is null essentially means it does not point to any object in memory, which can lead to unexpected results if not properly handled.
PowerShell `$null`
In PowerShell, `$null` is a special variable that is used to denote the absence of a value. It is important to understand that `$null` is not the same as a blank string or an empty collection — it specifically represents nothing. This distinction is vital to prevent logic errors in your scripts.
Here’s a simple code example illustrating how `$null` behaves:
$example = $null
Write-Output "The value is: $example" # output: The value is:
As shown, when we attempt to print the value stored in `$example`, it yields no output because it is null.

Checking for Null in PowerShell
Common Scenarios for Null Checks
You may frequently find situations in scripting where you need to determine if a variable is null. Examples include:
- When initializing data from a database and checking for null values that may indicate missing information.
- Handling optional parameters in functions where a null value might imply that no data has been provided.
By systematically checking for null values, you can avoid potential errors that arise from attempting operations on variables that do not hold any data.
How to Check if a Variable is Null or Empty
Using `if` Statements
A straightforward way to check if a variable is null is by using an `if` statement. The syntax is simple and allows for clear logic in your scripts.
if ($example -eq $null) {
Write-Output "The variable is null."
}
In this case, if `$example` is indeed null, the condition will evaluate to true, and the output will confirm that the variable is null.
Using the `IsNull` Function
You can create custom functions to encapsulate the logic for checking for null. This promotes code reuse and clarity in your scripts.
function Is-Null {
param ($var)
return $var -eq $null
}
You can then use this function in your scripts to quickly check for null values.
PowerShell Null Check
Using `Test-Path`
The `Test-Path` cmdlet is typically used to determine if a file or a directory exists, but it can also be leveraged to check for null values.
if (-not (Test-Path $example)) {
Write-Output "The variable is null or does not exist."
}
This method is particularly useful when your variable might represent a file path and you want to ensure it points to something valid.
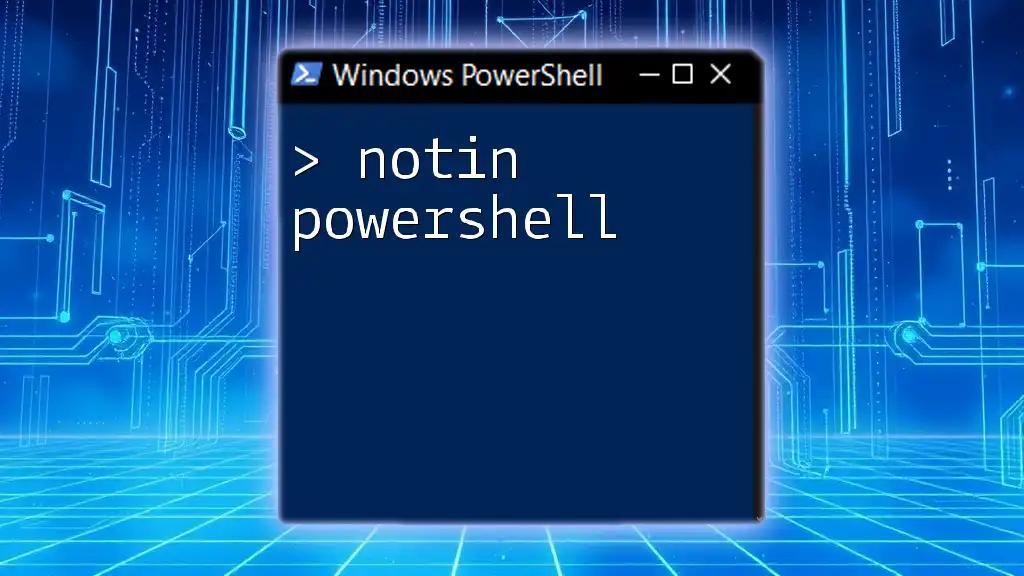
Managing Null Values in PowerShell
Setting a Variable to Null
Sometimes, it’s necessary to explicitly set a variable to null. Doing so can reset its state, especially when reusing variables throughout code.
$example = "Hello, World!"
$example = $null # Now $example is null
This can be helpful for managing state in longer scripts where you may want to clear variable values at certain points in execution.
PowerShell If Statements: Checking for Not Null
Using `-ne` Operator
To check if a variable is not null, you can use the `-ne` operator. This can be useful for validation checks before running further commands.
if ($example -ne $null) {
Write-Output "The variable is not null."
}
This structure ensures that any subsequent code depending on the presence of a value only executes when the variable isn’t null.
Using `Where-Object` for Filtering
If you’re working with collections, it’s common to filter out null values using `Where-Object`. This is crucial for ensuring that operations only apply to valid data.
$array = @(1, $null, 2, $null, 3)
$notNullItems = $array | Where-Object { $_ -ne $null }
Write-Output $notNullItems # Output: 1, 2, 3
Here, the filtered array only includes items that are not null, making it easier to work with the remaining data.

Further Examples and Use Cases
Real-World Applications of Null Checks
Effective null checks are essential in various situations, particularly in automation scripts where data is retrieved from APIs or databases. For instance, if your script retrieves user records, a null check on the results can help you handle scenarios where records might be missing.
Avoiding Null Reference Errors
To ward off null reference errors, adopt a disciplined approach to checking for null at the start of your functions, especially when dealing with input parameters. Implementing such checks ensures your scripts run smoothly without unexpected interruptions.
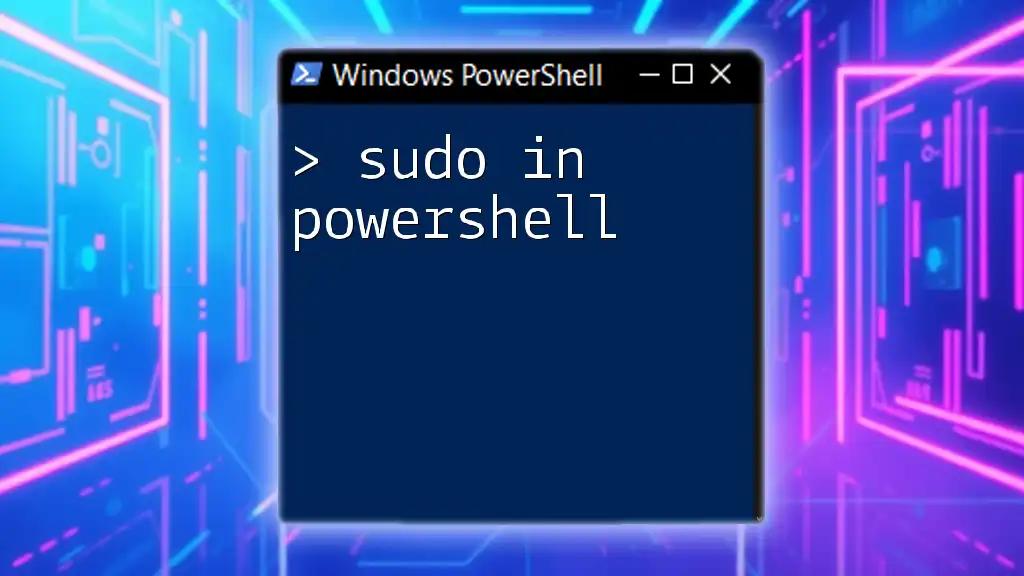
Conclusion and Best Practices
Quick Recap of Key Takeaways
Understanding null in PowerShell is not just an abstract concept; it is crucial for writing reliable and error-free scripts. Knowing how to effectively check for null values allows you to build robust solutions that can gracefully handle missing data.
Learning More about PowerShell
To enhance your PowerShell skills, seek out additional resources such as documentation, online courses, and community forums. Regular practice will aid in solidifying your understanding of null handling and other scripting techniques, empowering you to tackle more complex PowerShell tasks confidently.