In PowerShell, comments are created using the `#` symbol, allowing you to add notes or explanations within your scripts without affecting their execution.
# This is a comment in PowerShell
Write-Host 'Hello, World!'
What are Comments in PowerShell?
In PowerShell, comments are annotations included in your scripts that are not executed as part of the code. They serve several essential purposes, primarily enhancing the readability and maintainability of scripts. When others (or future you) review the code, comments provide context and explanations that help clarify the intent behind certain commands or logic.
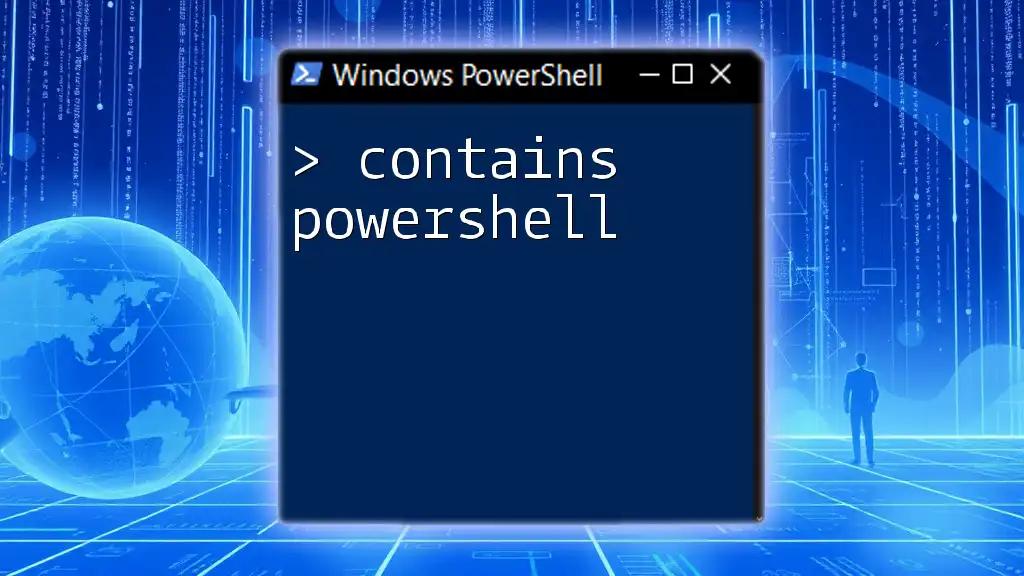
Types of Comments in PowerShell
Single-Line Comments
Single-line comments in PowerShell are initiated with the `#` symbol. Anything following this symbol on that line will be treated as a comment. This format is particularly useful for adding brief notes about specific lines or commands.
Example:
# This is a single-line comment
Get-Process # This command retrieves a list of processes
Best Practices for Single-Line Comments
- Keep them relevant and concise: Ensure that comments are directly related to the code they describe, avoiding unnecessary repetition.
- Avoid obvious statements: If the code is self-explanatory, a comment might not add value. For example, writing “incrementing counter” next to a command like `$counter++` is redundant.
Multi-Line Comments
Multi-line comments are denoted by `<#` to start the comment and `#>` to end it. This is particularly useful when you need to provide longer explanations that span multiple lines, making it easier to articulate complex logic or detailed documentation.
Example:
<#
This is a multi-line comment.
It can span multiple lines, helping to explain complex logic.
#>
Get-Service
Best Practices for Multi-Line Comments
- Use multi-line comments when you need to provide elaborate explanations or document specific logic that may not be clear at a glance.
- Assess when to use this format versus single-line comments, ensuring you choose the most effective method to communicate your intent.
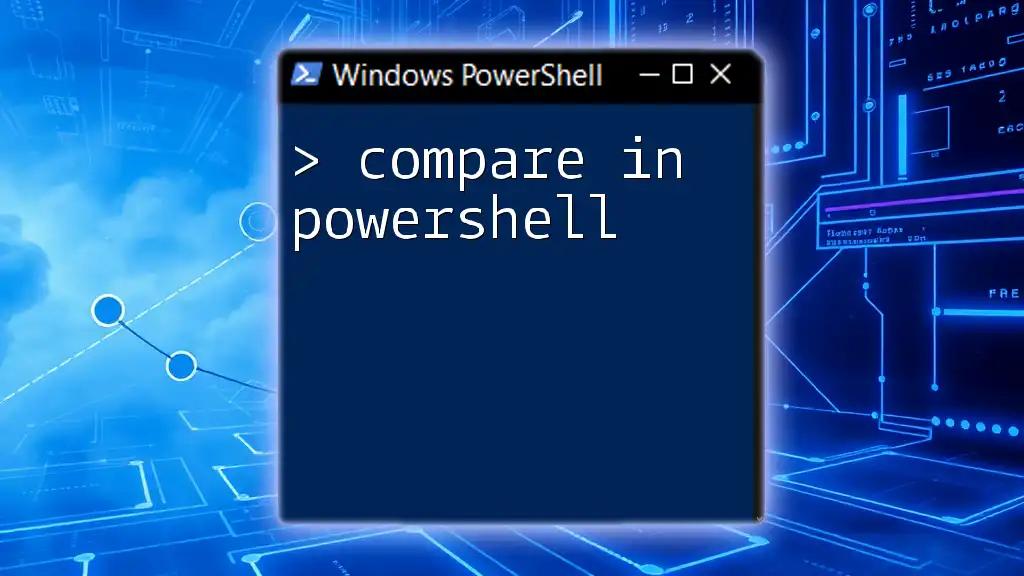
How to Write Effective Comments
Clarity and Purpose
When writing comments, clarity is crucial. Comments should convey the purpose and context of the code clearly. For instance, instead of saying:
# This command does something
You could express:
# Fetching current user information from Active Directory
Get-ADUser -Identity $username
This not only clarifies what the command does but also indicates its significance in the overall script.
Avoiding Over-Commenting
While comments are beneficial, it is essential to find a balance. Over-commenting can lead to clutter, making the code harder to read. Consider this when you find yourself commenting on nearly every line. Instead, focus on explaining the intent of sections or complex logic rather than stating the obvious.
Consistency
Maintaining a uniform commenting style across your scripts is essential, particularly in a collaborative environment. Consider establishing a simple style guide for comments. This guide could outline:
- How to mark sections of the script.
- The use of capitalization or punctuation.
- Guidelines on when to use comments and when to let the code speak for itself.
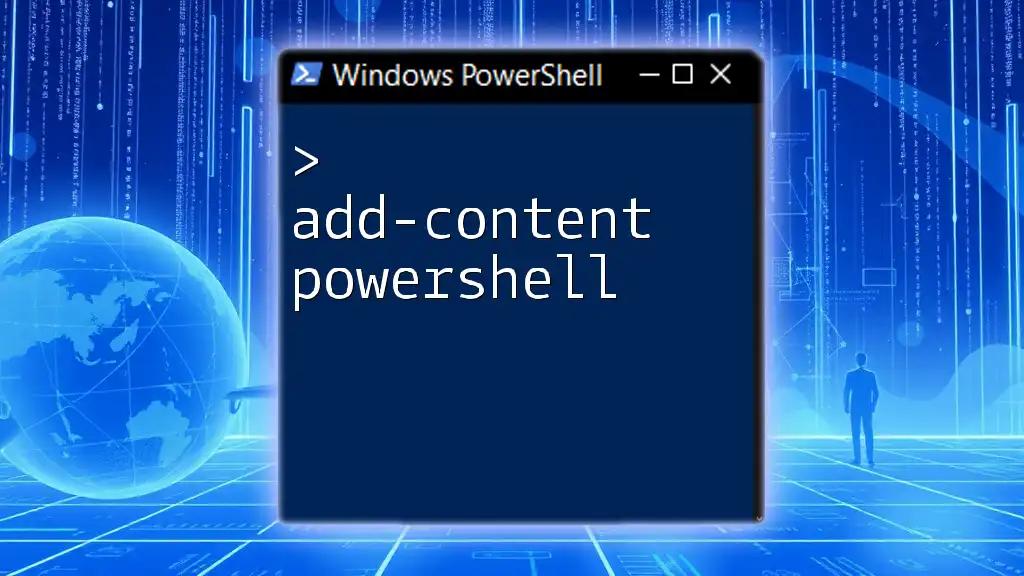
Practical Examples of Using Comments
Effective commenting becomes particularly evident in more complex scripts. Consider the following example where the comments clearly articulate the script's intent:
# This script backs up user files to a remote server
# Define variables for source and destination
$source = "C:\Users\Public\Documents"
$destination = "\\RemoteServer\Backup"
# Copy files to backup location
Copy-Item -Path $source -Destination $destination -Recurse
In this script, comments succinctly explain the intent behind each section, aiding understanding without getting in the way of the code.
Documenting Functions
Comments play a crucial role in documenting functions. When defining a function, utilize comments to describe its purpose and the parameters it takes. Here's an example:
function Backup-Files {
<#
.SYNOPSIS
Backs up specified files to a remote location.
.PARAMETER Source
The source directory to copy files from.
.PARAMETER Destination
The destination directory to copy files to.
#>
param(
[string]$Source,
[string]$Destination
)
# Copy files from source to destination
Copy-Item -Path $Source -Destination $Destination -Recurse
}
In this example, the use of a multi-line comment at the beginning serves as documentation, clearly outlining what the function does and what parameters it accepts, making it easier for users to understand how to use the function correctly.
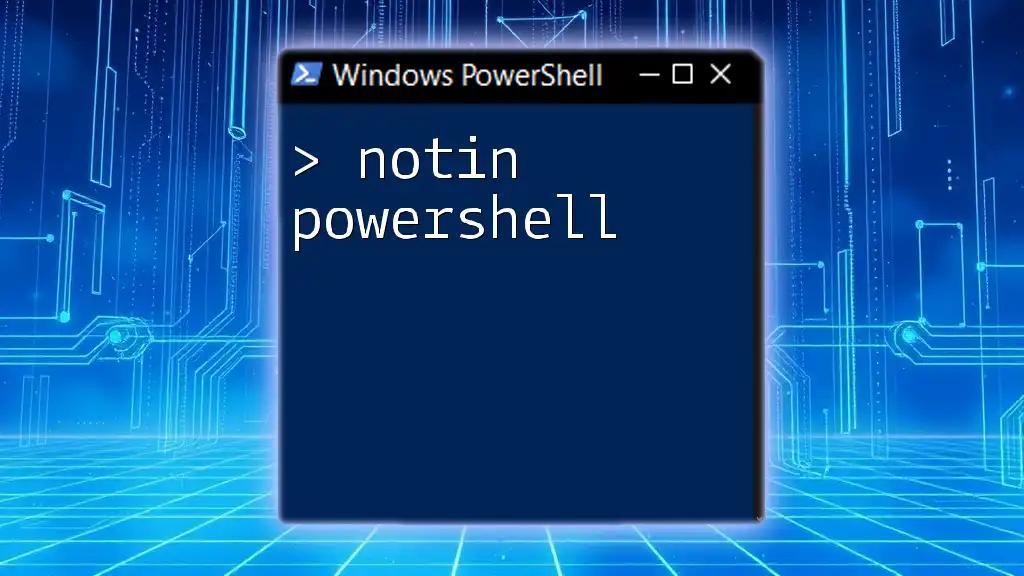
Common Mistakes to Avoid
One common mistake is commenting out code instead of properly removing it. This can lead to confusion, especially for team members who come across the commented-out code and wonder about its relevance. In general, if a code segment is no longer needed, it's often best to delete it rather than comment it out.
Another pitfall is relying on comments to explain poorly written code. While comments can help clarify the intent, it's often more effective to improve the code’s clarity instead of attempting to explain it away with comments.
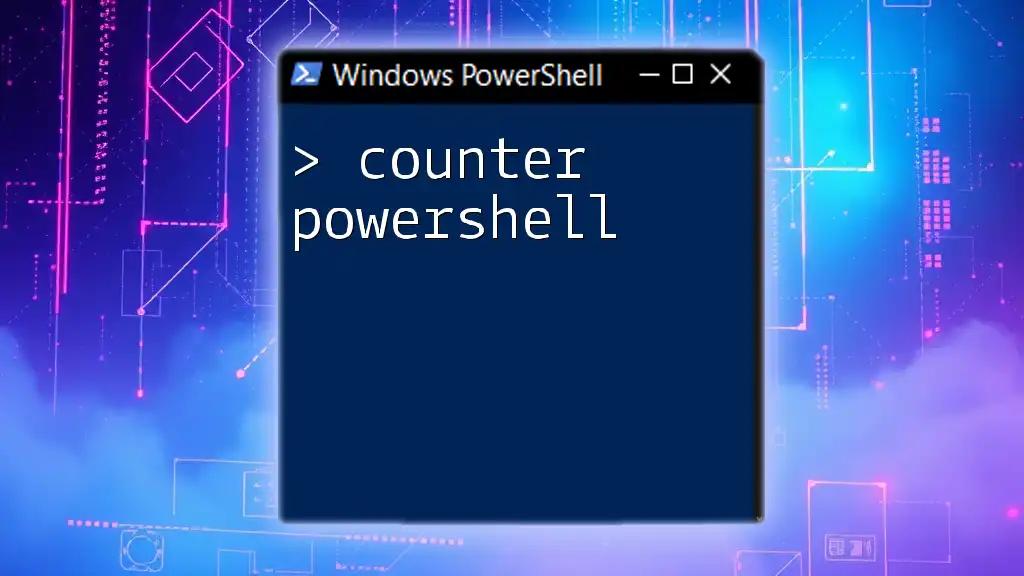
Tools and Resources
Utilizing tools like the PowerShell Integrated Scripting Environment (ISE) can assist you in adding and editing comments conveniently. Within ISE, you can leverage features like syntax highlighting, which helps distinguish between code and comments, enhancing your editing experience further.
If you are looking to deepen your understanding of PowerShell comments and scripting in general, consider exploring recommended resources such as:
- Books on PowerShell programming.
- Online courses tailored to PowerShell scripting.
- Community forums where you can share tips and learn from others.
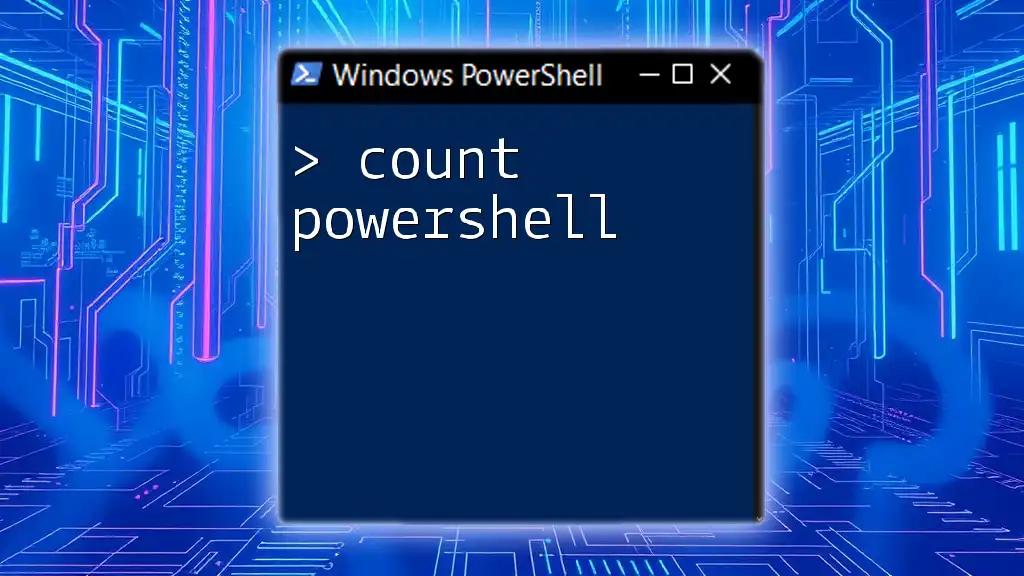
Conclusion
In summary, commenting in PowerShell scripts is not just a good practice—it's a necessity for ensuring that your scripts remain understandable and maintainable over time. By effectively using both single-line and multi-line comments, you can significantly improve code readability. Moreover, adhering to best practices ensures clarity while avoiding the pitfalls of over-commenting or lack of consistency.
As you try out these techniques, remember that practice makes perfect. Start integrating these strategies into your own PowerShell scripts, and soon you'll see how well-placed comments can enhance your coding experience.

FAQs
What’s the best way to comment complex scripts?
The best approach involves using multi-line comments for elaborate explanations while utilizing single-line comments for brief, contextual notes.
Are there any limitations to the comment syntax in PowerShell?
While the comment syntax is straightforward, be mindful of context and structure to prevent misunderstandings.
Can comments affect script performance?
No, comments do not affect performance as they are ignored during execution. However, keep in mind that excessive commenting can make code harder to read and maintain.