The `Start-Sleep` command in PowerShell is used to pause the script's execution for a specified amount of time, allowing you to control the timing of your commands.
Here’s a code snippet demonstrating its usage:
Start-Sleep -Seconds 5
Write-Host 'Hello, World!'
Understanding the Wait Command
The wait command in PowerShell refers to the ability to delay the execution of commands in your scripts. This can be particularly useful in various scenarios, such as waiting for a task to complete, pacing script execution, or allowing time for user interactions. Understanding how this command works is essential for efficient script automation.
Why Use the Wait Command?
Control over timing is crucial in scripting and automation. Here are a few scenarios where implementing a wait command is beneficial:
- Chaining Command Execution: Sometimes, you may need to wait for a process to complete before performing subsequent actions. Failing to add a wait might lead to errors or unforeseen behavior.
- User Feedback: Introducing pauses allows the user to read output or provide input. This ensures that the script doesn’t rush through prompts and helps in understanding the results.
- Resource Management: In some cases, waiting can help to avoid resource contention issues. For example, when interacting with databases or APIs, introducing a wait can minimize the chances of running into throttling issues.

Basic Syntax of the Wait Command
The most common way to implement pauses in PowerShell scripts is through the Start-Sleep command, which is a built-in cmdlet designed to halt execution for a specified duration.
Syntax Breakdown
The general syntax for the wait command in PowerShell using Start-Sleep is:
Start-Sleep -Seconds <time>
- The `-Seconds` parameter allows you to specify how long the script should wait.
- `<time>` represents the duration in seconds.
Example: Simple Usage of Start-Sleep
Here's a basic example illustrating how to use the wait command:
Start-Sleep -Seconds 5
This line will pause the execution of the script for five seconds.
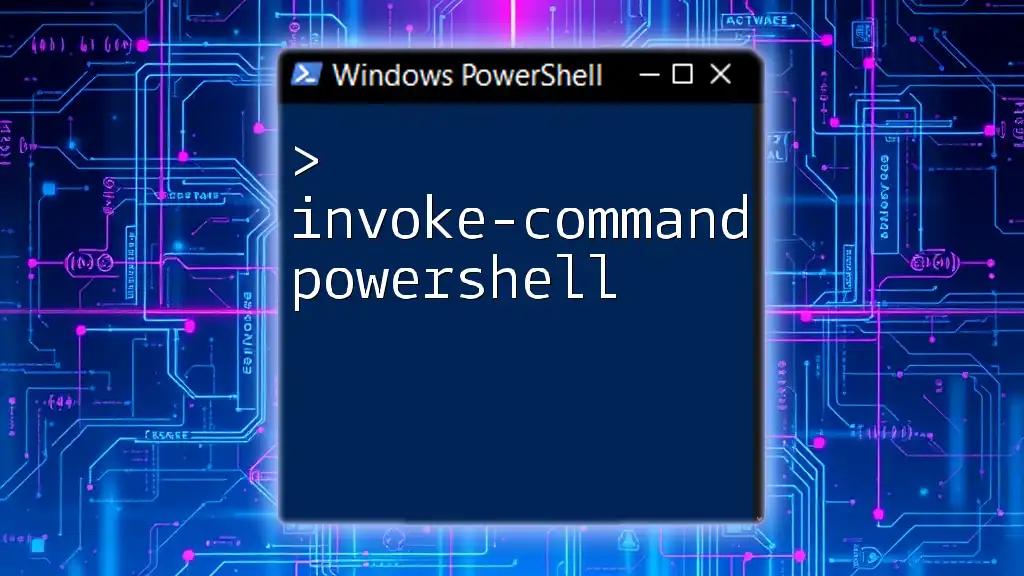
Using the Wait Command in Practice
Setting a Wait Timer
In practical scenarios, you can easily introduce a wait timer in your scripts. For example, consider the following script snippet, which demonstrates a ten-second delay before executing the next command:
Write-Host "Waiting for 10 seconds..."
Start-Sleep -Seconds 10
Write-Host "Continuing script execution."
In this example:
- The script informs the user that a delay is occurring.
- After a ten-second pause, it indicates that continuation is happening.
Practical Applications of Wait Time
Automated Tasks: Introduce wait commands in automated scripts, such as deployment or data processing tasks, where timing impacts the success of subsequent operations.
User Prompts: Utilize pauses to enhance user experience. For instance, allowing users time to read or respond to prompts during a script run.
Process Monitoring: In automated scripts that deal with external processes, such as data imports, using wait commands to pause execution until a process completes can stabilize outcomes.

Advanced Uses of the Wait Command
Wait for a Specific Event
The wait command in PowerShell can also be used in combination with other cmdlets to manage execution more finely. Consider the scenario where you want to wait for a specific process to complete before moving on:
$process = Start-Process notepad -PassThru
$process.WaitForExit()
Write-Host "Notepad has exited."
In this example:
- `Start-Process` is used to initiate Notepad.
- The script pauses until Notepad is closed because of the `WaitForExit()` method, ensuring that the following command only executes after the notepad process is complete.
Conditional Waiting
For more complex logic, you can implement loops to create a conditional wait. For example, you might want to wait until a specific file appears in the directory:
while (-not (Test-Path "C:\temp\myfile.txt")) {
Write-Host "Waiting for file..."
Start-Sleep -Seconds 5
}
Write-Host "File found!"
This loop continuously checks for the existence of "myfile.txt". If the file doesn’t exist, it pauses for five seconds and checks again. This ensures that the script won’t proceed until the required file is available.
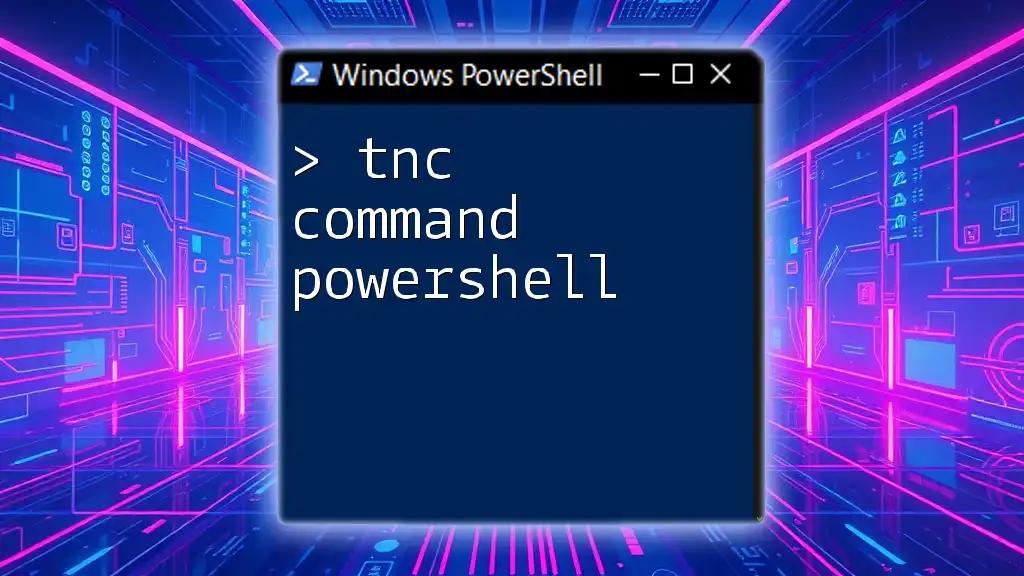
Performance Considerations
Choosing the Right Wait Time
When using the wait command in PowerShell, it's vital to determine appropriate wait times. Long wait durations can slow down script execution considerably, while too-short waits can lead to failures if the desired condition hasn’t been met yet. Always aim for a balance between performance and reliability.

Best Practices for Using the Wait Command
Tips for Effective Use
- Minimal Wait Times: Keep wait times as short as possible to maintain responsiveness in your scripts.
- Conditional Waits over Fixed Waits: Whenever feasible, implement conditional waits that only pause when necessary, rather than using fixed static wait times.
- Documentation: Clearly document wait commands in your scripts to ensure that their purpose and need are understandable, facilitating easier debugging or enhancements in the future.
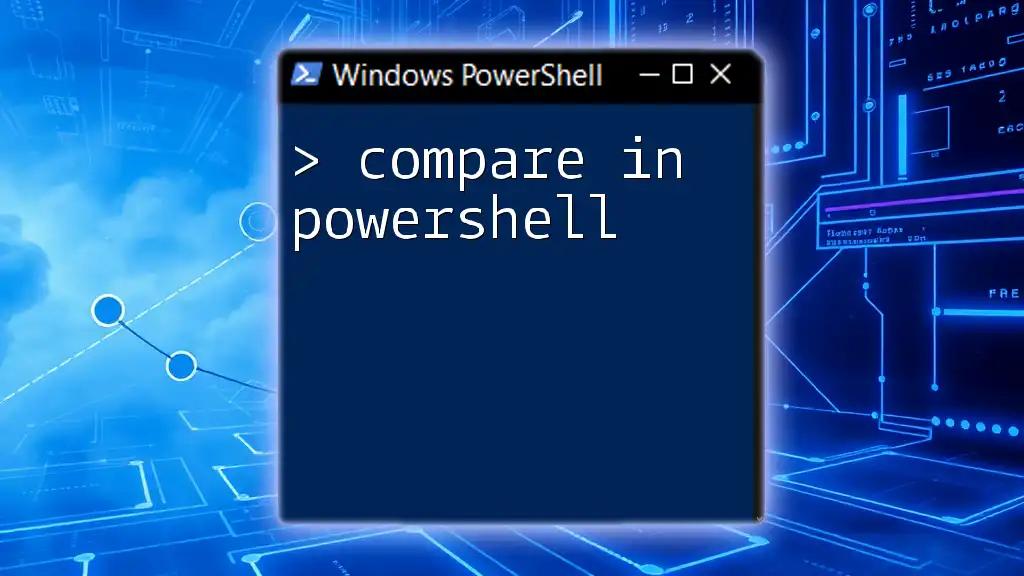
Conclusion
The wait command in PowerShell is a powerful tool that enhances the functionality and reliability of scripts. By mastering this command, you can greatly improve the control you have over your automation processes. It’s not just about pausing; it’s about making sure everything flows seamlessly, leading to efficient task execution.

Additional Resources
For further learning, consider exploring the official PowerShell documentation, where you can find more in-depth information on cmdlets and best practices for scripting and automation. Engaging with community forums and tutorial offerings can also bolster your understanding and practical skills.
Call to Action
If you're eager to deepen your knowledge and improve your scripting abilities, join our courses to explore the intricacies of PowerShell and unlock new automation possibilities.