In PowerShell, a dictionary (or hashtable) is a collection of key-value pairs that allows for efficient data retrieval and manipulation.
Here’s a simple example of creating and accessing a hashtable in PowerShell:
$dictionary = @{"Name"="John"; "Age"=30; "City"="New York"}
Write-Host "Name: $($dictionary['Name']), Age: $($dictionary['Age']), City: $($dictionary['City'])"
What is a Dictionary in PowerShell?
A dictionary in PowerShell is a collection of key-value pairs where each key is unique. This data structure provides a fast way to retrieve, update, and manage large sets of data. Unlike arrays, which are indexed by numeric positions, dictionaries allow for a more flexible and descriptive way to work with data, making them ideal for various scripting scenarios.
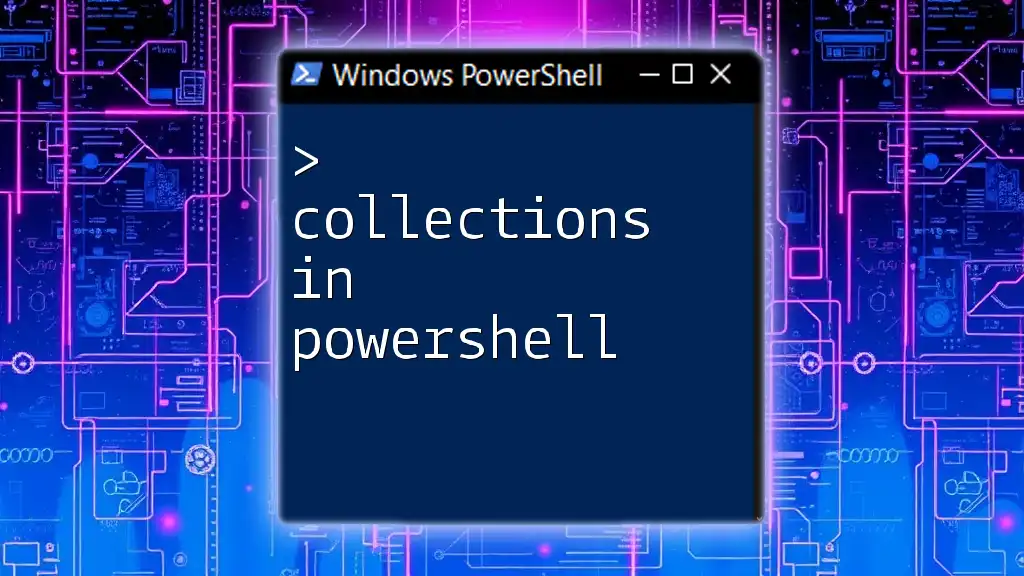
Why Use Dictionaries?
Using dictionaries can significantly enhance both the performance and readability of your scripts. They enable you to organize data in a way that makes sense contextually—allowing you to access values quickly without searching through a list of items. This efficiency is particularly beneficial when dealing with large datasets or complex scripts where clarity is key.
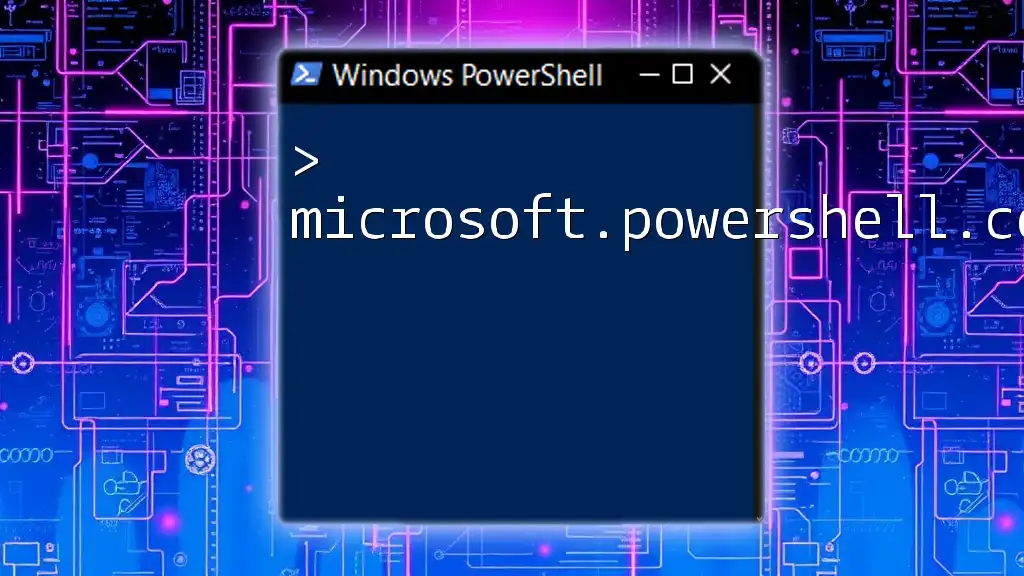
Understanding Dictionaries
Definition of a Dictionary
A dictionary, sometimes referred to as an associative array or hash table, consists of two main components: keys and values. Each key serves as a unique identifier for its corresponding value. Because keys must be unique, a dictionary prevents duplicate entries, ensuring that you can efficiently look up data.
Types of Dictionaries in PowerShell
Standard Dictionary: This is the default type of dictionary in PowerShell that allows you to store key-value pairs.
Ordered Dictionary: This variation retains the order in which items were added, making it useful when the sequence of data matters.
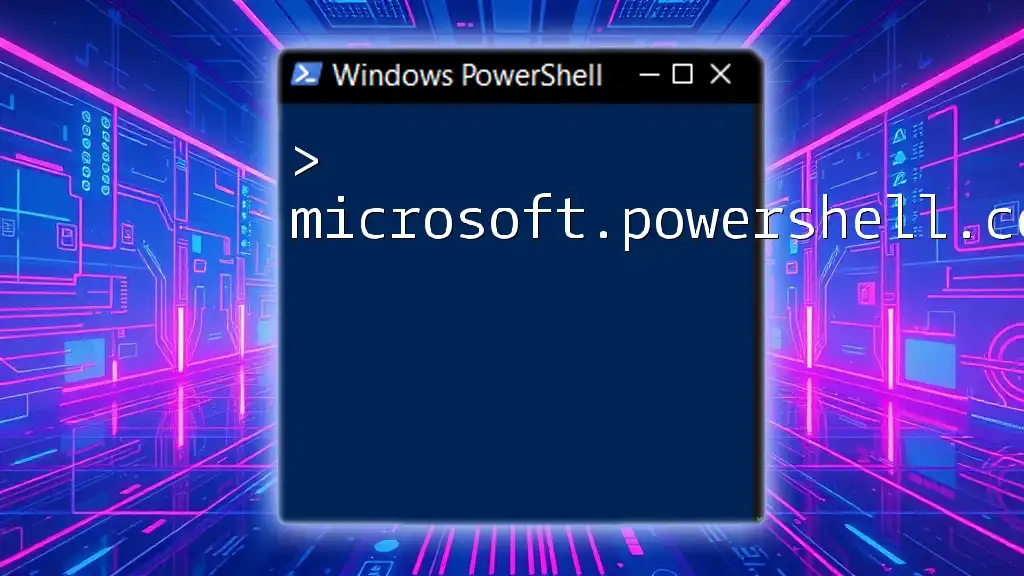
Creating a Dictionary in PowerShell
Syntax for Creating a Dictionary
Creating a simple dictionary in PowerShell can be done using `@{}` syntax:
$dictionary = @{}
Example: Simple Dictionary Initialization
Here’s how you can initialize a basic dictionary with a few key-value pairs:
$myDict = @{'Name'='John'; 'Age'=30; 'Country'='USA'}
Adding Key-Value Pairs
You can easily add new items to your dictionary:
$myDict['City'] = 'New York'
This operation shows how flexible dictionaries can be, allowing you to add or modify data dynamically.
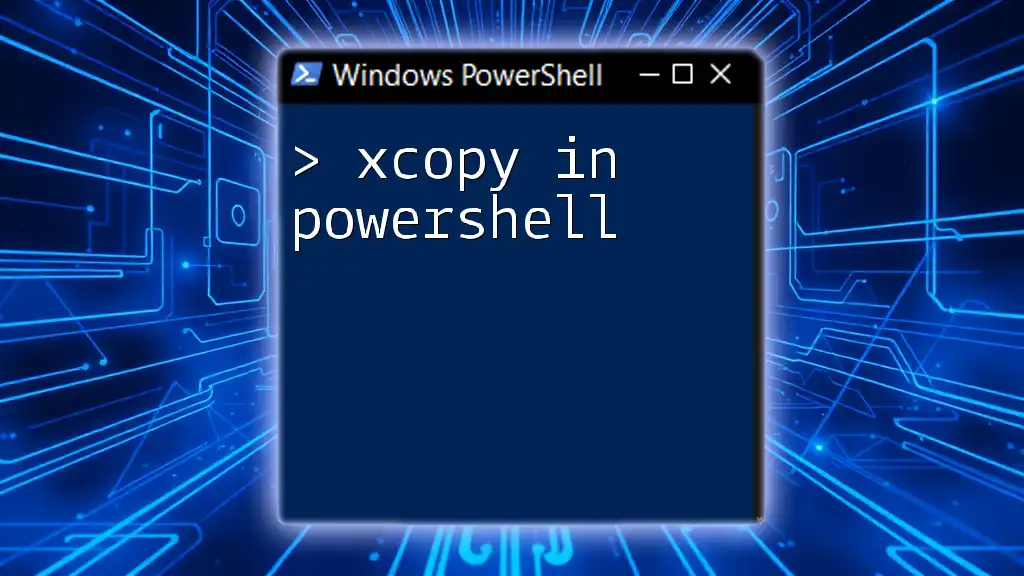
Accessing Data in a Dictionary
Retrieving Values
To retrieve a value based on its key, you can use the following syntax:
$value = $myDict['Name']
Example: Accessing and Using Retrieved Data
You can output the retrieved value like this:
Write-Host "User Name: $value"
Checking for Existence of Keys
Dictionaries provide a method to check if a key exists:
if ($myDict.ContainsKey('Age')) {
Write-Host "Age exists!"
}
This ensures that you can safely perform operations without encountering key-not-found errors.
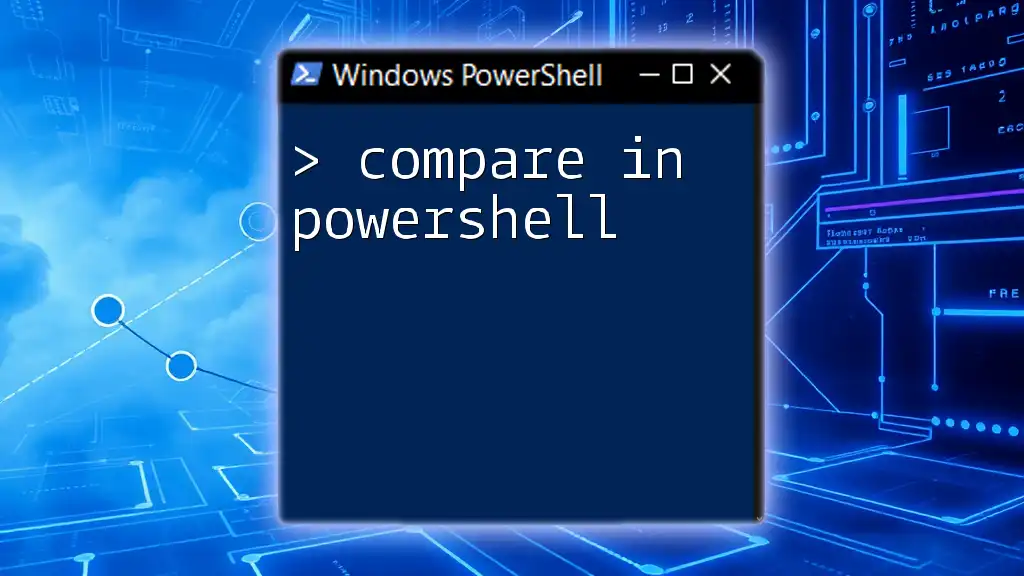
Modifying a Dictionary
Updating Values
Updating a value in a dictionary is straightforward. For instance, if you want to change the age:
$myDict['Age'] = 31
Removing Key-Value Pairs
To remove an entry, you simply call the `Remove` method:
$myDict.Remove('City')
Example: Verifying Removal
You can verify that the key has been removed:
if (-not $myDict.ContainsKey('City')) {
Write-Host "City key has been removed."
}
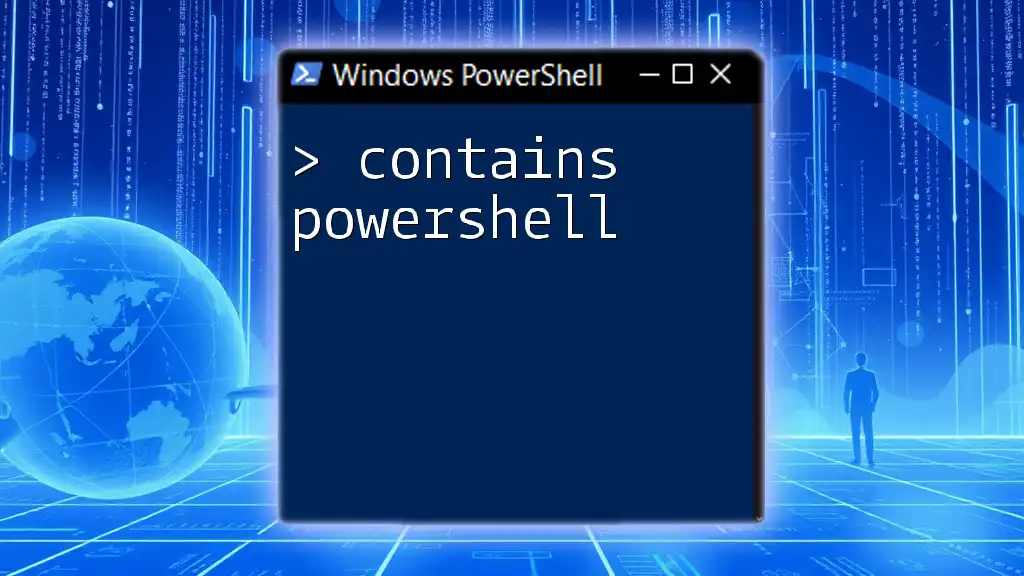
Iterating Over a Dictionary
Looping Through Keys and Values
You can loop through the keys in a dictionary efficiently by using a `foreach` loop:
foreach ($key in $myDict.Keys) {
Write-Host "$key: $($myDict[$key])"
}
Example: Displaying All Key-Value Pairs
This loop easily allows you to display each key with its corresponding value.
Using ForEach-Object
Another method to iterate through a dictionary is using the `ForEach-Object` cmdlet:
$myDict.GetEnumerator() | ForEach-Object {
Write-Host "$($_.Key): $($_.Value)"
}
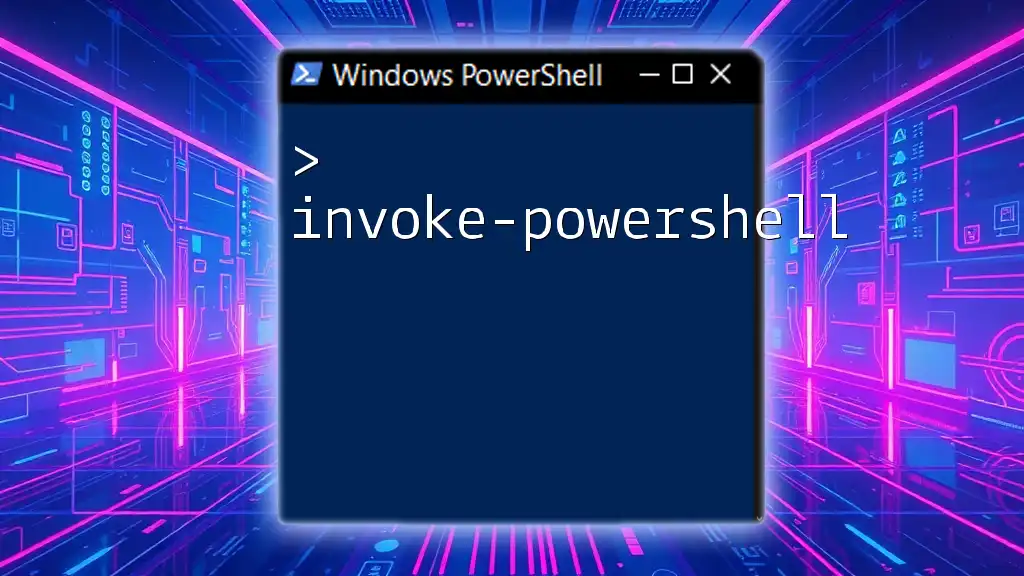
Advanced Dictionary Operations
Merging Dictionaries
You can combine two dictionaries into one. Here’s how you can achieve that:
$anotherDict = @{'State'='NY'; 'Year'=2023}
$mergedDict = @{}
$myDict.GetEnumerator() | ForEach-Object { $mergedDict[$_.Key] = $_.Value }
$anotherDict.GetEnumerator() | ForEach-Object { $mergedDict[$_.Key] = $_.Value }
This creates a merged dictionary containing entries from both source dictionaries.
Sorting a Dictionary
You might wish to sort a dictionary based on either keys or values. For example, to sort by keys:
$sortedDict = $myDict.GetEnumerator() | Sort-Object Key
This operation provides an ordered list, making it easier to manipulate or display.
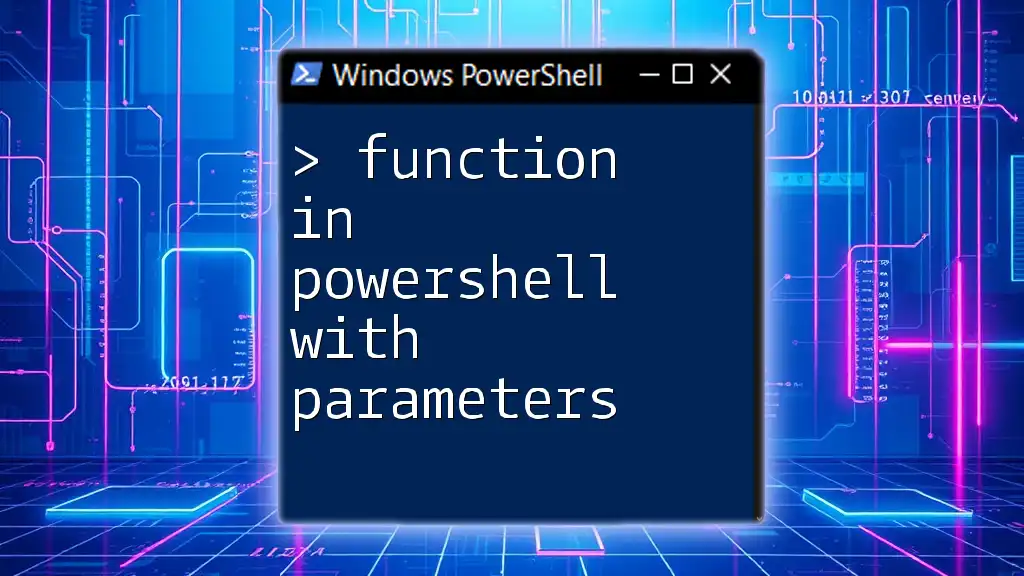
Best Practices for Using Dictionaries in PowerShell
When to Use Dictionaries vs Arrays
Dictionaries are favored when you need to look up items by a specific identifier, while arrays are best suited for ordered lists of items. Using dictionaries for associative storage helps keep your data organized and accessible.
Performance Considerations
In many scenarios, dictionaries offer significant performance advantages—especially for lookups. While arrays may involve searching through index positions, dictionaries allow for O(1) average time complexity for key lookups, making them more efficient in scripting environments.
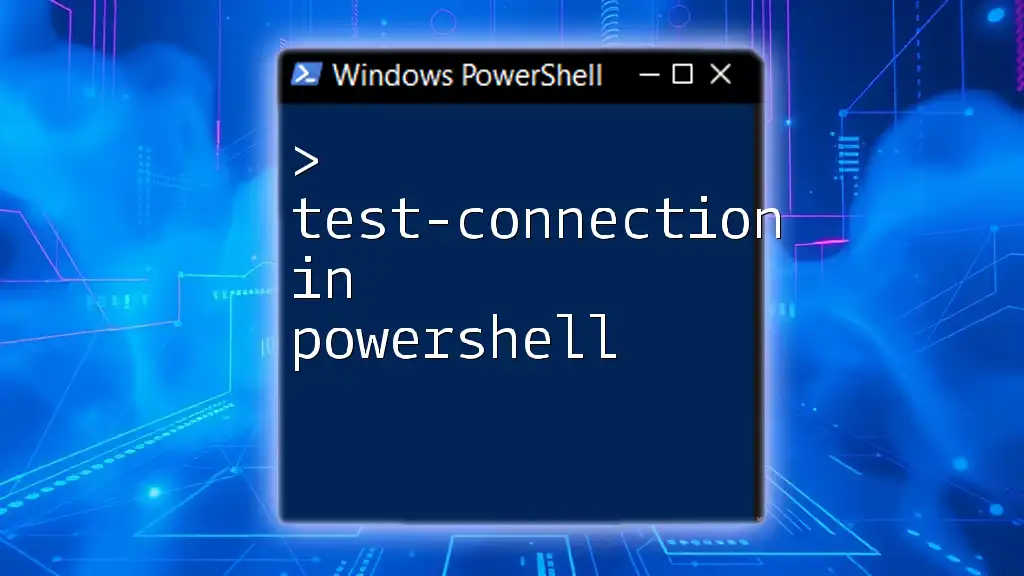
Conclusion
Understanding how to effectively use a dictionary in PowerShell opens up a world of possibilities in your scripting. With their unique capabilities, dictionaries can lead to cleaner, more understandable, and faster scripts. By practicing the examples provided, you will master this powerful data structure in no time.
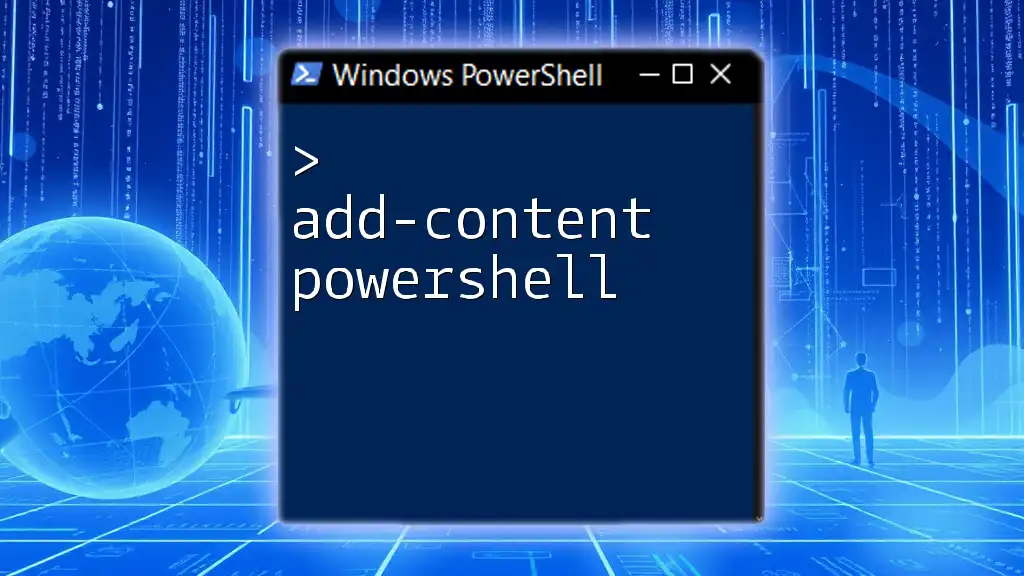
Call to Action
Don’t forget to subscribe for more PowerShell tips and tricks, and feel free to explore related articles to elevate your scripting skills further.
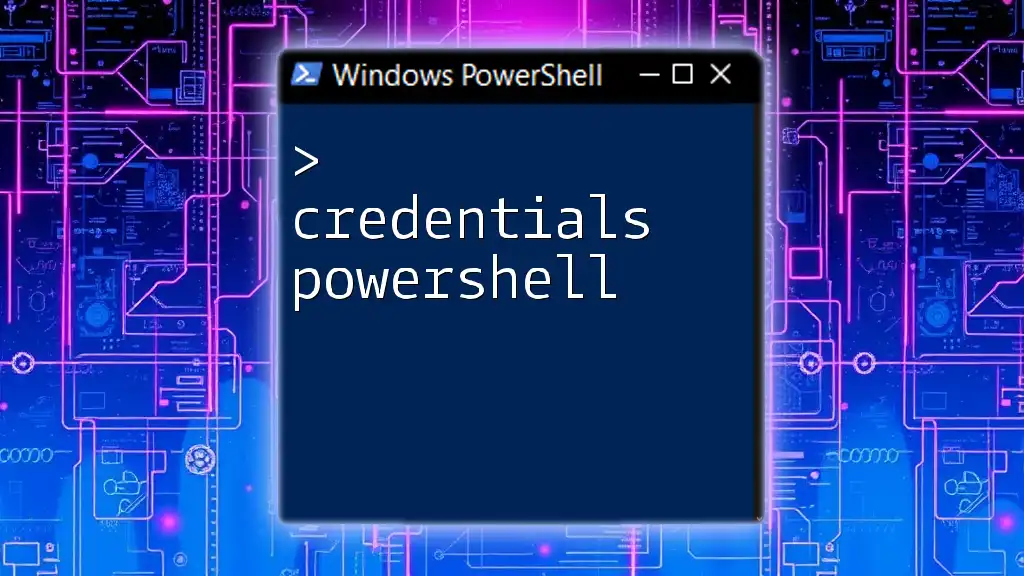
Additional Resources
For more in-depth knowledge, check the official PowerShell documentation on data structures or consider enrolling in recommended books or courses that focus on advanced PowerShell techniques.