In PowerShell, collections are versatile data structures that can store multiple items, enabling efficient data management and manipulation.
Here's a simple example of creating an array collection in PowerShell:
# Creating an array collection
$fruitCollection = @('Apple', 'Banana', 'Cherry')
# Displaying the collection
$fruitCollection
What Are Collections?
Collections in PowerShell refer to data structures that store and manage multiple values. They play a crucial role in scripting, allowing users to group data together, manipulate it efficiently, and perform complex operations on these data sets. By utilizing collections properly, you can enhance the readability and effectiveness of your scripts.
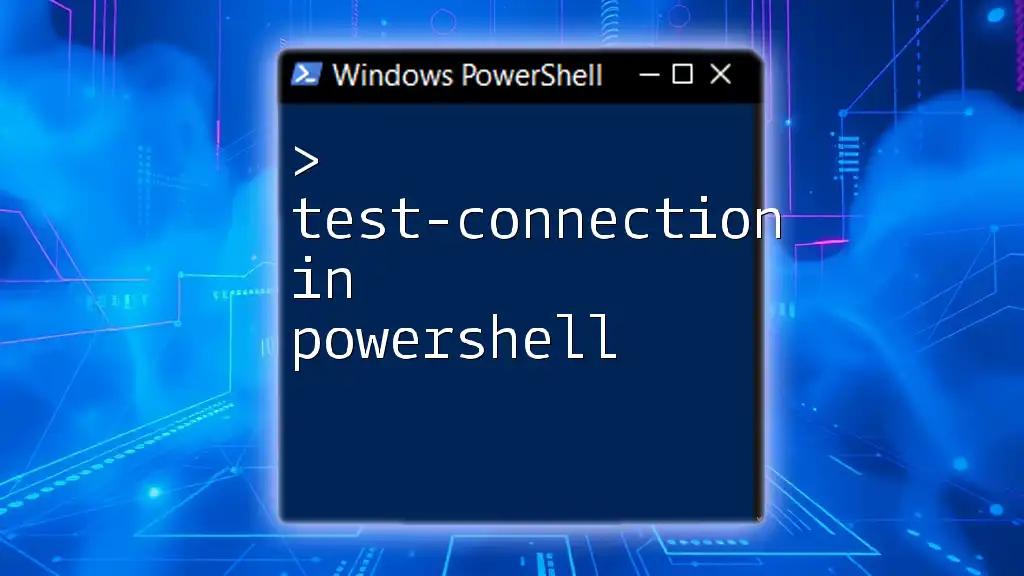
Types of Collections
Arrays
Definition and Characteristics
Arrays are one of the most basic forms of collections in PowerShell. An array holds a fixed-size sequential collection of items of the same type. Unlike some other programming languages, PowerShell arrays can hold items of different data types.
Creating and Using Arrays
Creating an array in PowerShell is straightforward. You can do this using the `@()` syntax.
Example Code Snippet:
$myArray = @(1, 2, 3, 4)
In this example, `@()` denotes that you're creating an array, and you can include any number of comma-separated values.
Common Array Methods
You can easily manipulate arrays through various methods.
-
Adding elements: You can append elements using the `+=` operator.
Example Code Snippet:
$myArray += 5 # Add an element
-
Accessing elements: Elements in an array are accessed using zero-based indexing.
Example Code Snippet:
$secondElement = $myArray[1] # Access the second element
Hash Tables
Definition and Characteristics
Hash tables are another powerful collection type in PowerShell, representing a collection of key-value pairs. This structure makes it easy to look up data by keys, providing fast access to value information.
Creating and Using Hash Tables
You create a hash table using the `@{}` syntax.
Example Code Snippet:
$myHashTable = @{
'Name' = 'John'
'Age' = 30
}
This snippet creates a hash table with keys `Name` and `Age`, each associated with their respective values.
Common Hash Table Methods
Hash tables allow for dynamic resizing and easy data manipulation.
-
Adding key-value pairs: You can add new pairs effortlessly.
Example Code Snippet:
$myHashTable['Location'] = 'USA' # Add new key-value pair
-
Accessing values: Fetching a value is simple: just use the key.
Example Code Snippet:
$age = $myHashTable['Age'] # Access the value associated with 'Age'
ArrayLists
Definition and Characteristics
An ArrayList is a collection that can hold an assortment of elements, with a key feature being its dynamic resizing capability. Unlike static arrays, it can grow as you add data.
Creating and Using ArrayLists
To create an ArrayList, utilize the `New-Object` cmdlet:
Example Code Snippet:
$myArrayList = New-Object System.Collections.ArrayList
$myArrayList.Add("Item1")
This creates an ArrayList and adds an item.
Common ArrayList Methods
ArrayLists provide several useful methods to manipulate data.
-
Inserting and Removing items: You can insert or remove items easily.
Example Code Snippet:
$myArrayList.RemoveAt(0) # Remove the first item

Working with Collections
Iterating through Collections
Using loops is one of the primary methods of iterating through collections.
Using Loops
A `foreach` loop works effectively here, as shown below:
Example Code Snippet:
foreach ($item in $myArray) {
Write-Output $item
}
This loop prints each element in `$myArray`, demonstrating how to access items seamlessly.
Filtering Collections
Efficiency in working with data can be achieved through filtering. The `Where-Object` cmdlet is invaluable for this purpose.
Example Code Snippet:
$filteredArray = $myArray | Where-Object {$_ -gt 2}
In this example, the filter returns only items greater than 2, showcasing how to manage collections based on conditions.
Sorting Collections
Using Sort-Object
Organizing data is essential, and the `Sort-Object` cmdlet simplifies this task.
Example Code Snippet:
$sortedArray = $myArray | Sort-Object
This command sorts the array in ascending order, making data easier to read and analyze.
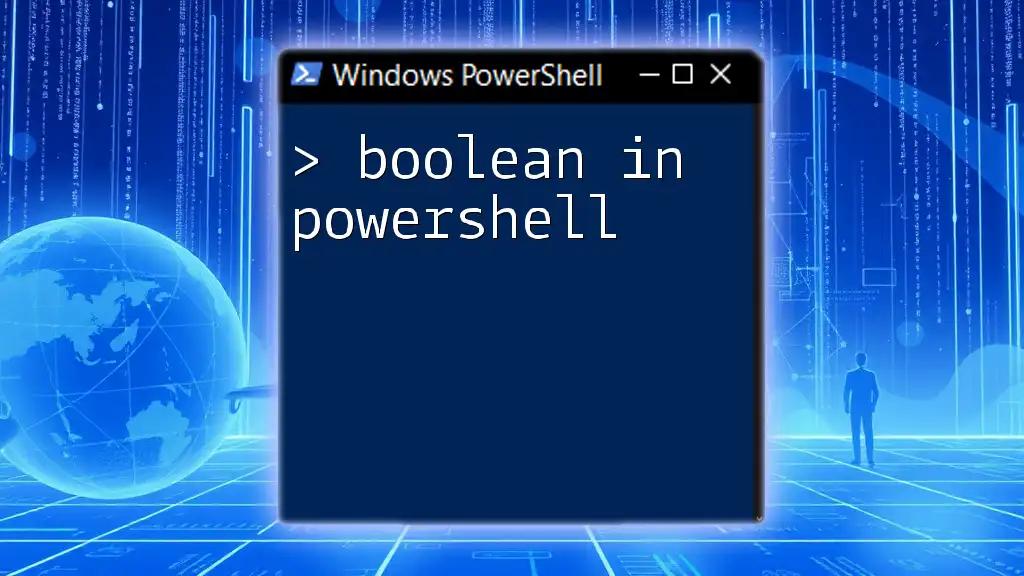
Advanced Collection Techniques
Nested Collections
Understanding and Using Nested Arrays
Collections can be nested, allowing for complex data structures. For instance, you can have an array of arrays.
Example Code Snippet:
$nestedArray = @(@(1,2,3), @(4,5,6))
Accessing nested array elements requires two indices.
Converting Collections
Converting Arrays to Hash Tables
You may find the need to convert an array into a hash table for better organization and accessibility of data.
Example Code Snippet:
$convertedHashTable = @{}
foreach ($item in $myArray) {
$convertedHashTable[$item] = $item * 2
}
In this snippet, each element of `$myArray` is used as a key, with values double the key's value.
Using Cmdlets for Conversion
PowerShell provides cmdlets like `ConvertTo-Json` for easily transforming collections into JSON format for API interactions or external processing.
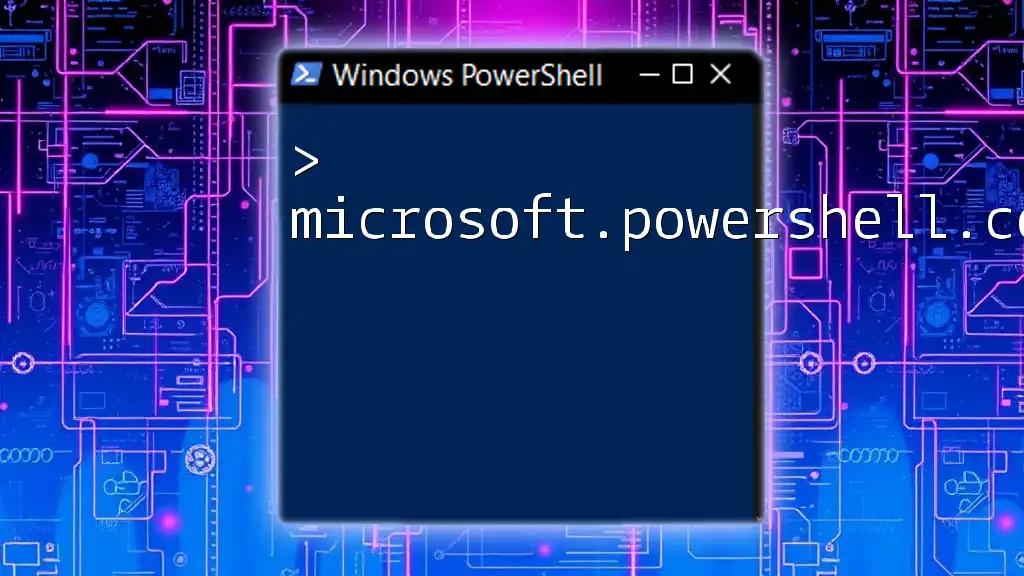
Practical Applications of Collections
Collections in PowerShell serve numerous practical applications, particularly in automation tasks. They excel in scenarios such as:
- Data manipulation: Easily modify data elements in batches.
- Reporting: Group and summarize data for reports dynamically.
These capabilities streamline the scripting process, making your code more efficient and powerful.
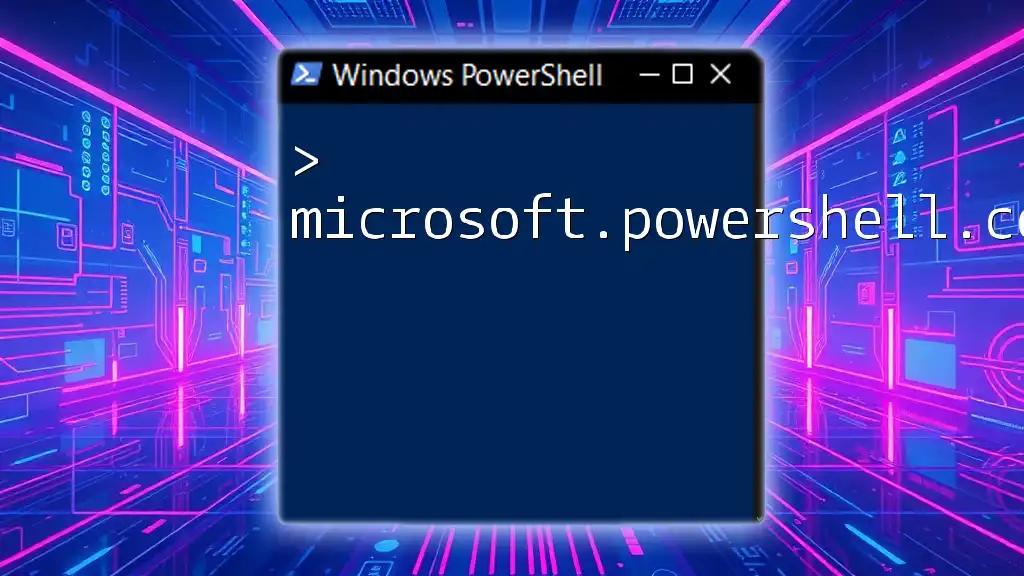
Best Practices
To maximize the effectiveness of collections in PowerShell, consider the following best practices:
- Use the appropriate collection type based on your use case.
- Regularly comment on your code to clarify data manipulations for future reference.
- Test your collections thoroughly, especially when filtering or converting, to avoid data loss.
Awareness of common pitfalls, such as treating arrays like hash tables, can also prevent bugs and improve your overall coding experience.
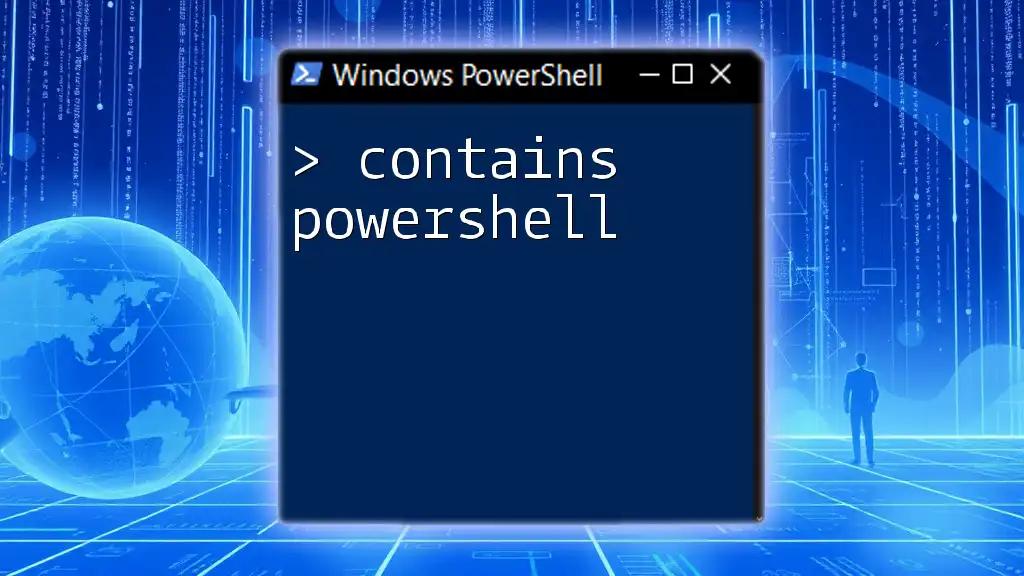
Conclusion
Understanding collections in PowerShell is essential for anyone looking to automate tasks efficiently. By mastering the various types of collections and how to manipulate them, you can significantly enhance the effectiveness of your scripts and achieve more complex results with ease. Embrace these techniques, and watch your PowerShell skills flourish!
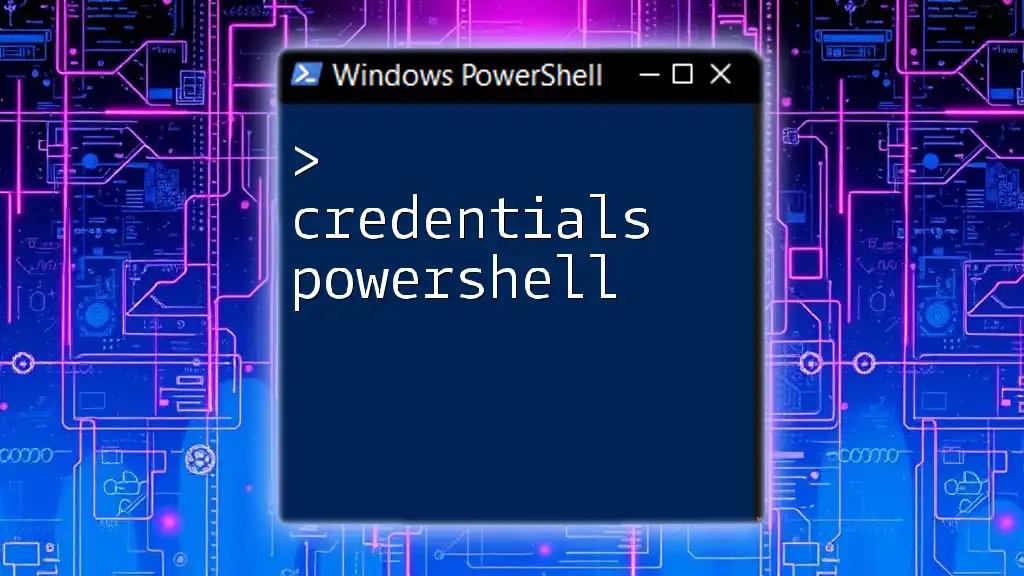
Additional Resources
To further deepen your understanding of collections in PowerShell, consider exploring the official PowerShell documentation and engaged community forums. They provide actionable insights, diverse coding styles, and advanced use cases that can be immensely beneficial in your learning journey.