In PowerShell, classes are blueprints for creating objects, allowing you to define properties and methods that encapsulate data and behavior.
class Greeting {
[string]$Message
Greeting([string]$msg) {
$this.Message = $msg
}
[void]SayHello() {
Write-Host $this.Message
}
}
$greet = [Greeting]::new("Hello, World!")
$greet.SayHello()
What Are Classes in PowerShell?
Classes in PowerShell are fundamental structures that define objects, encapsulating data (properties) and functionality (methods) in a single package. Understanding classes is pivotal for leveraging the true power of PowerShell's object-oriented capabilities.
Classes vs. Objects: A class is a blueprint or template from which objects are created. Objects are specific instances of a class and contain real values, whereas classes define properties and behaviors without actual data.
Basic Concepts
When working with classes, it's essential to grasp three primary concepts:
- Properties: Attributes that hold data for the objects. For instance, a class representing a person might have properties like `Name` and `Age`.
- Methods: Functions or actions that the objects can perform. For example, a `GetDetails` method could return a formatted string of the person’s name and age.
- Inheritance: The ability to create new classes based on existing ones, allowing for code reusability and the establishment of hierarchical relationships.
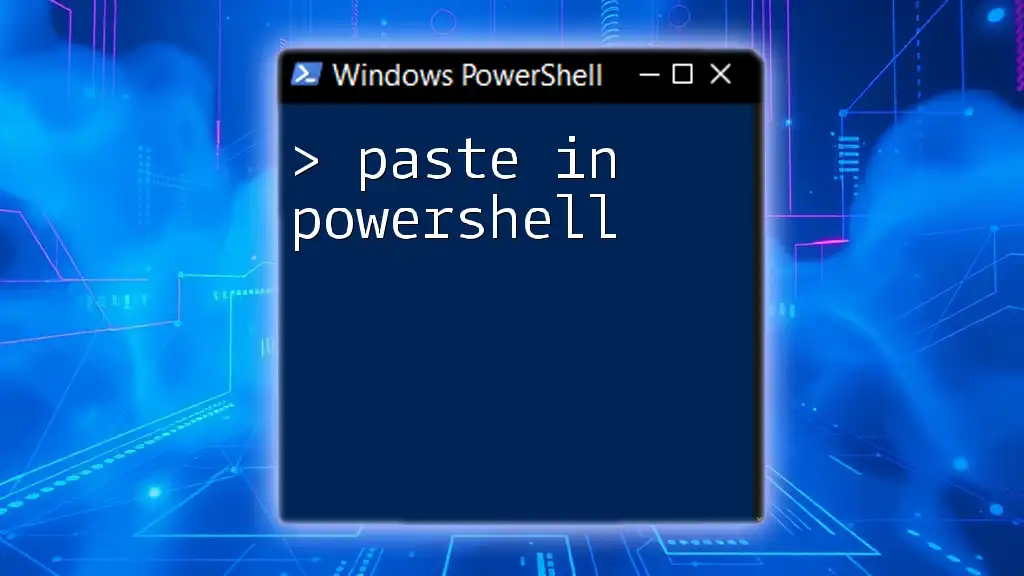
Why Use Classes in PowerShell?
Utilizing classes in PowerShell introduces several advantages that enhance coding practices:
- Object-Oriented Programming: Classes enable you to use an object-oriented programming paradigm, allowing for better data management and modular design.
- Code Reusability: Once defined, classes can be reused throughout your PowerShell scripts, reducing redundancy and errors.
- Improved Readability: Well-structured classes improve code readability, making it easier for developers to understand the logic and flow of the scripts.
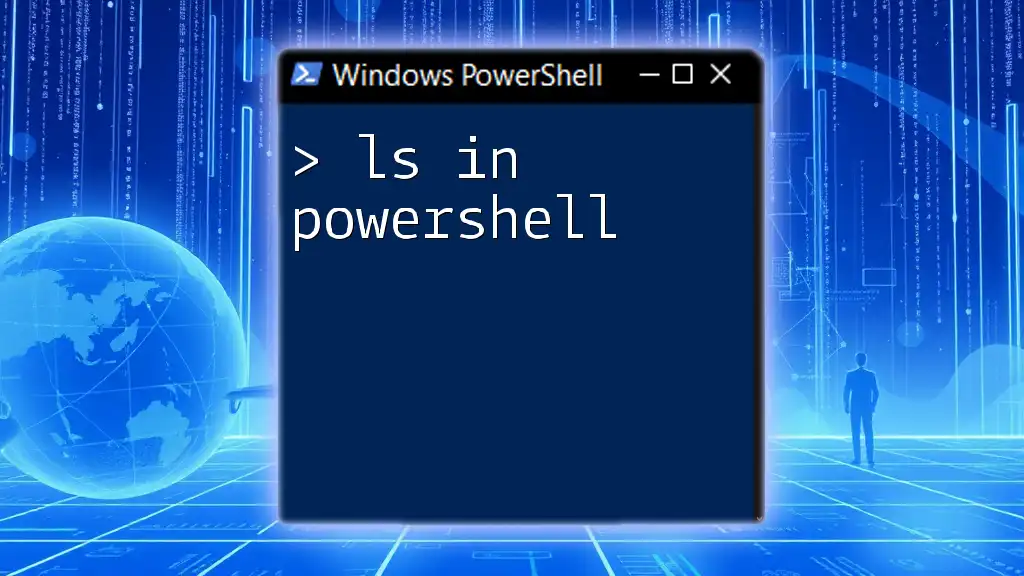
Creating a Class in PowerShell
Creating a class in PowerShell is straightforward. The basic structure is as follows:
class ClassName {
# Properties and methods go here
}
Example: Creating a Simple Class
Here is a simple demonstration of creating a `Person` class:
class Person {
[string]$Name
[int]$Age
Person([string]$name, [int]$age) {
$this.Name = $name
$this.Age = $age
}
[string] GetDetails() {
return "Name: $($this.Name), Age: $($this.Age)"
}
}
In this example, the `Person` class has two properties—`Name` and `Age`. The constructor initializes these properties, while the `GetDetails` method returns a summary of the object's data.
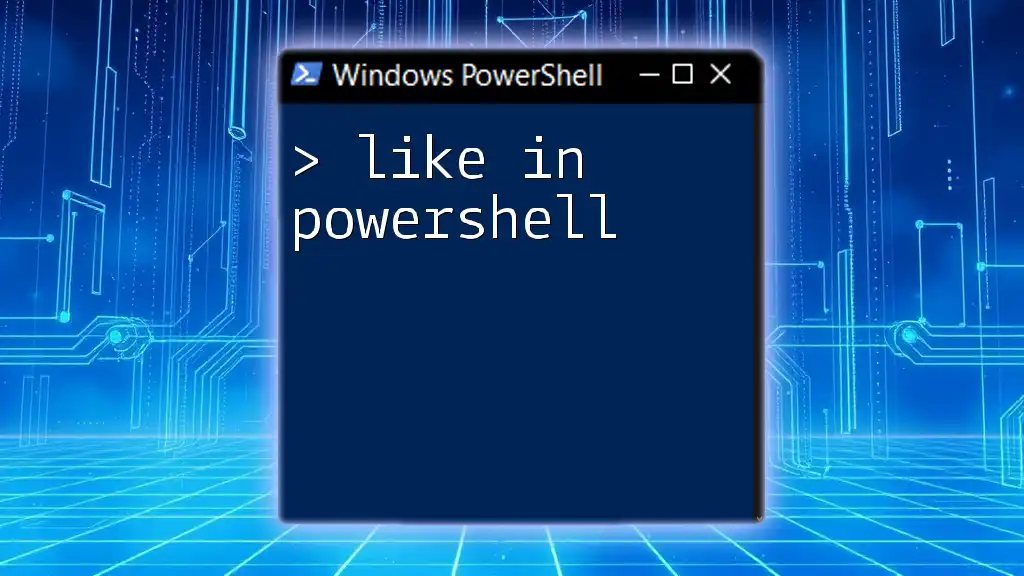
Creating and Using Objects from Classes
Instantiating Objects
Creating an object from a class is performed using the `new` method. For instance:
$person1 = [Person]::new("Alice", 30)
This line instantiates a new `Person` object, `person1`, with the name "Alice" and age 30.
Accessing Properties and Methods
Once an object is created, you can easily access its properties and call its methods:
To access a property, use:
$person1.Name # Output: Alice
To invoke a method, use:
$person1.GetDetails() # Output: Name: Alice, Age: 30
This illustrates how simple it is to interact with instances of your classes.
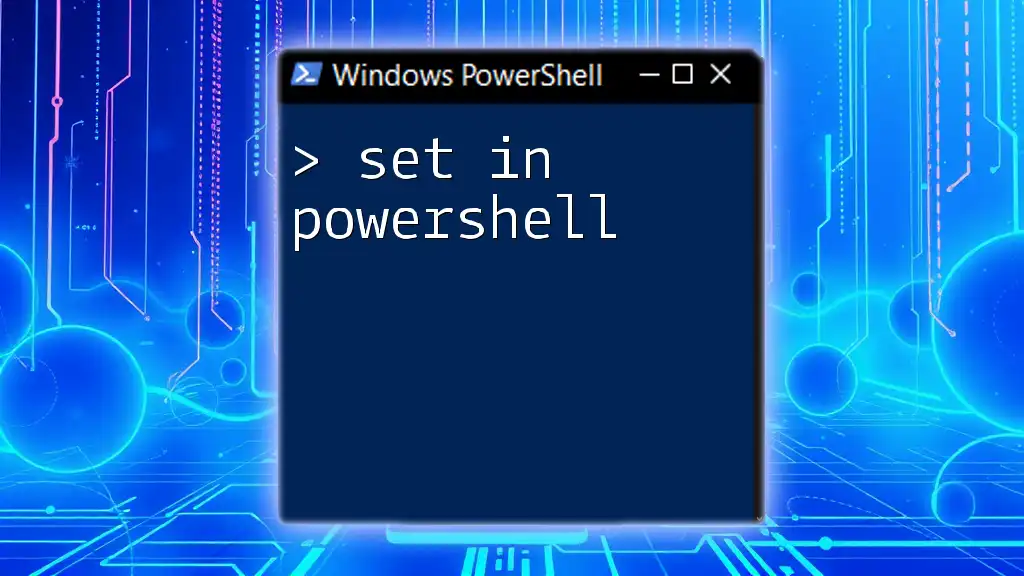
Class Inheritance in PowerShell
What Is Inheritance?
Inheritance allows one class (derived class) to inherit the properties and methods of another (base class). This fosters a cleaner design and code reuse.
Creating a Derived Class
Consider the following example that demonstrates class inheritance:
class Employee : Person {
[string]$Position
Employee([string]$name, [int]$age, [string]$position) : base($name, $age) {
$this.Position = $position
}
[string] GetDetails() {
return "Name: $($this.Name), Age: $($this.Age), Position: $($this.Position)"
}
}
In this example, `Employee` inherits from the `Person` class, gaining access to its properties and methods. The `GetDetails` method is overridden to include the position of the employee, showcasing polymorphism.
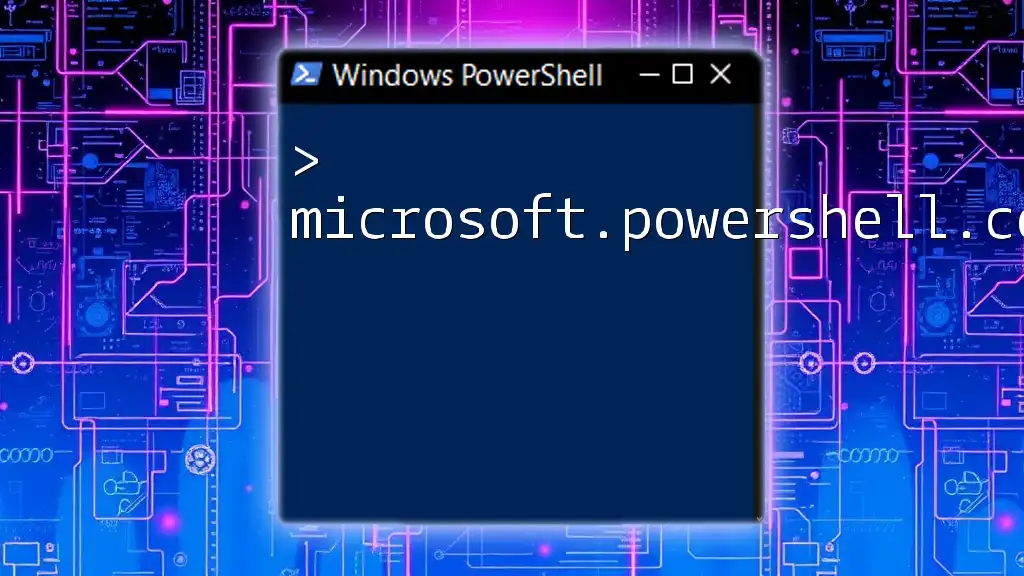
Understanding Properties and Methods
Properties
Properties are defined within a class to hold data associated with an object. You can specify data types to enforce type safety.
Methods
Methods define behaviors for the objects. They can accept parameters, which allow you to pass data to be processed.
Encapsulation: This principle ensures that object data is protected and only accessible through methods, maintaining integrity and security.
Static Members
Static properties and methods are accessible without creating instances of a class. Here’s how you can define a static method:
class MathOperations {
static [int] Add([int]$a, [int]$b) {
return $a + $b
}
}
You can call the static method directly:
$sum = [MathOperations]::Add(2, 3) # Output: 5
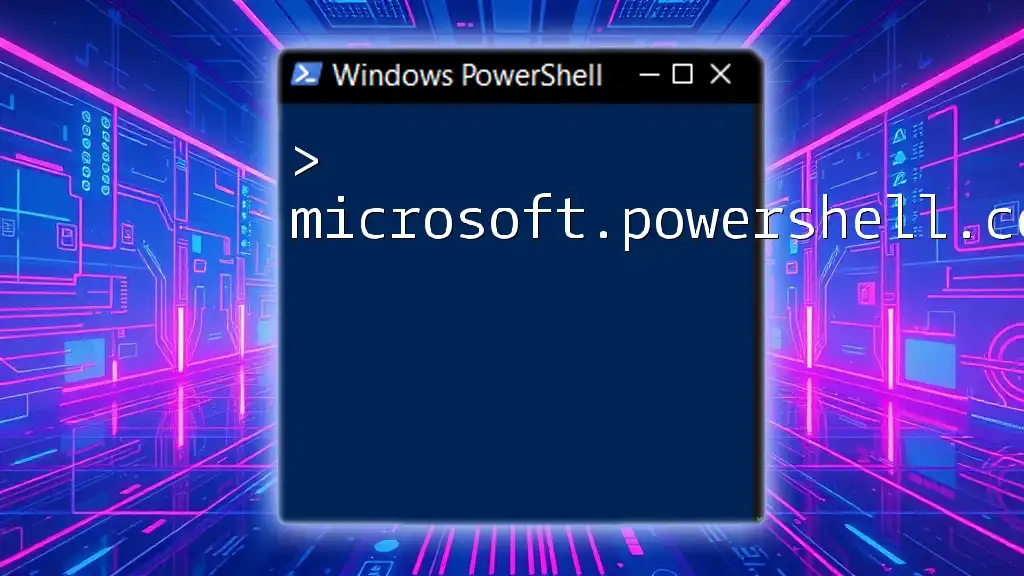
Advanced Class Features
Interfaces
Interfaces define a contract of methods that implementing classes must have. Here’s an example:
interface IShape {
[double] CalculateArea()
}
Classes that implement this interface must provide a definition for the `CalculateArea` method.
Abstract Classes
An abstract class cannot be instantiated directly and typically includes abstract methods that must be defined in derived classes. Here's an example:
abstract class Shape {
[double] CalculateArea()
}
Practical Applications of Classes in PowerShell
Classes can be applied in various scenarios, such as:
- Automation Scripts: Simplifying complex scripts by grouping related functions into classes.
- Configuration Management: Encapsulating configuration settings in objects for easier management.
- Reusable Modules: Packaging classes as modules to share across multiple scripts.
Sample Project
An example project could involve creating a user management system where `User`, `Admin`, and `Guest` classes inherit from a base `User` class, encapsulating common attributes and behaviors.
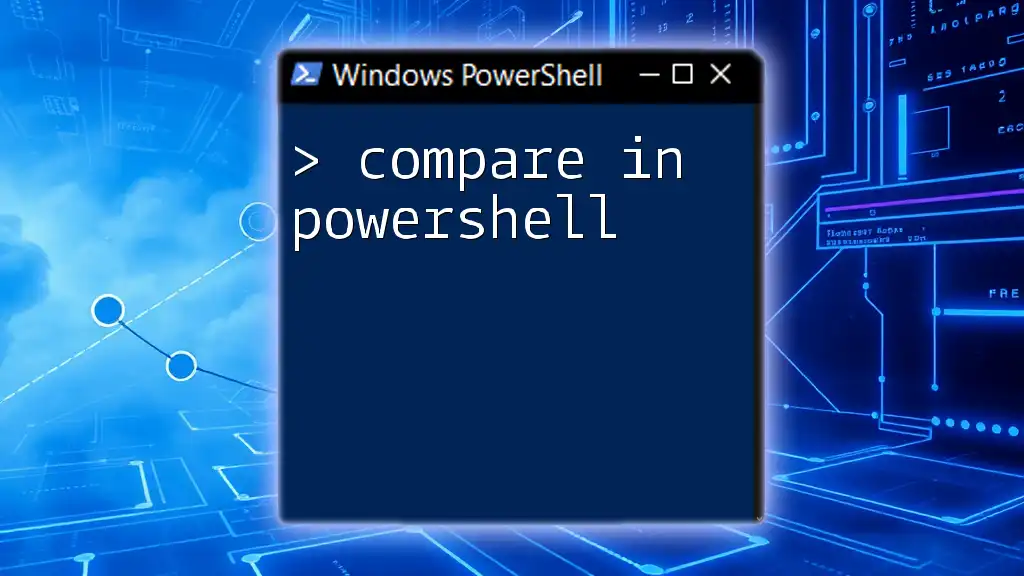
Best Practices for Using Classes in PowerShell
Naming Conventions
When naming classes, use PascalCase (e.g., `Person`, `Employee`) for clarity and consistency. Method names should also follow this convention.
Documentation and Commenting
Documenting your code with comments is essential for maintenance. Use comments to explain complex logic or to provide context for specific code sections.
For example:
# This method returns the details of the person
[string] GetDetails() {
return "Name: $($this.Name), Age: $($this.Age)"
}
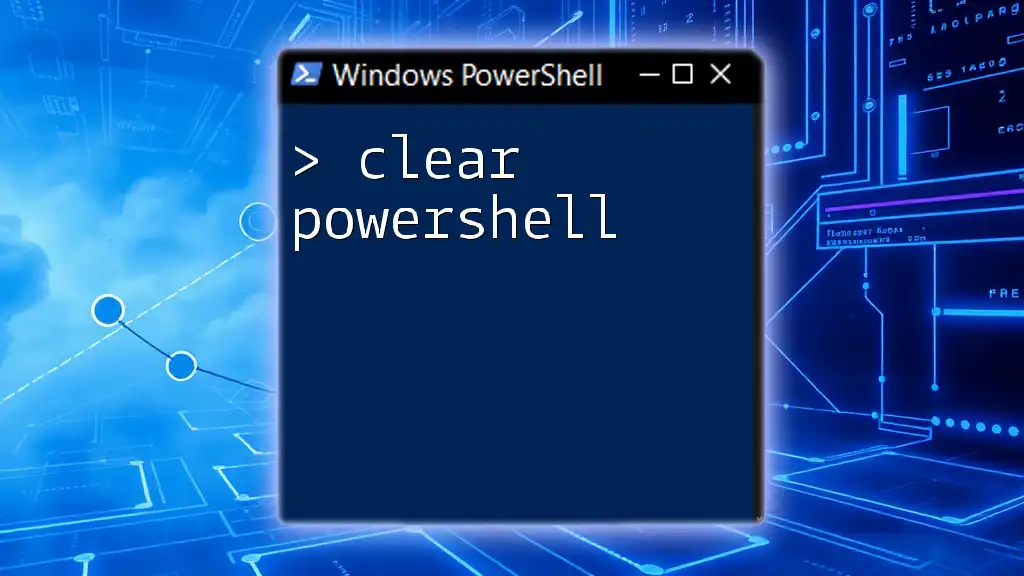
Conclusion
Understanding classes in PowerShell expands your scripting capabilities and introduces powerful object-oriented programming concepts into your workflows. By leveraging classes, you can create cleaner, more maintainable, and reusable scripts, enhancing your productivity and the quality of your code.
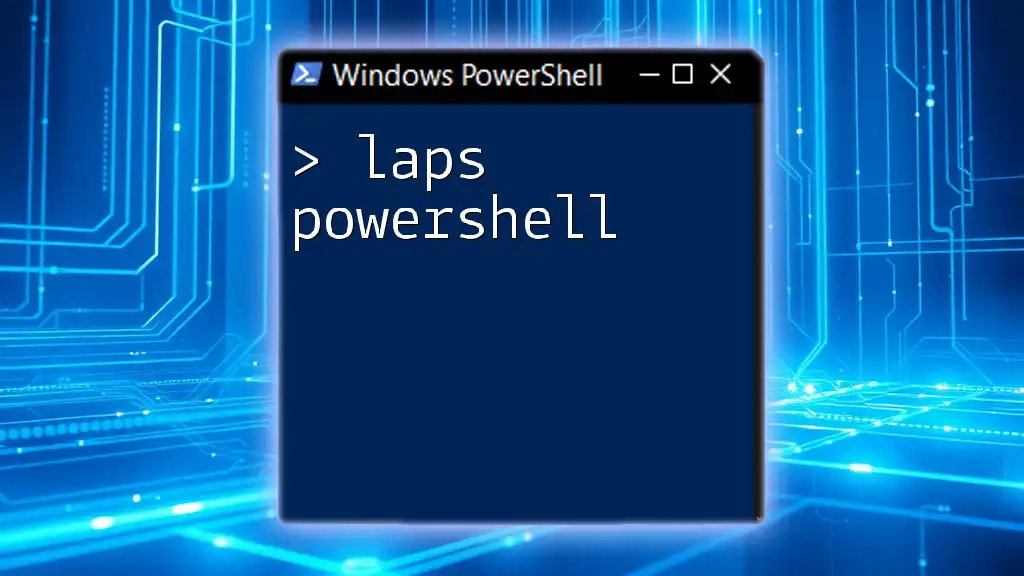
Additional Resources
To further deepen your understanding of classes in PowerShell, consider exploring the following resources:
- Official Microsoft documentation on PowerShell classes
- PowerShell community forums and websites
- Books focusing on advanced PowerShell scripting techniques
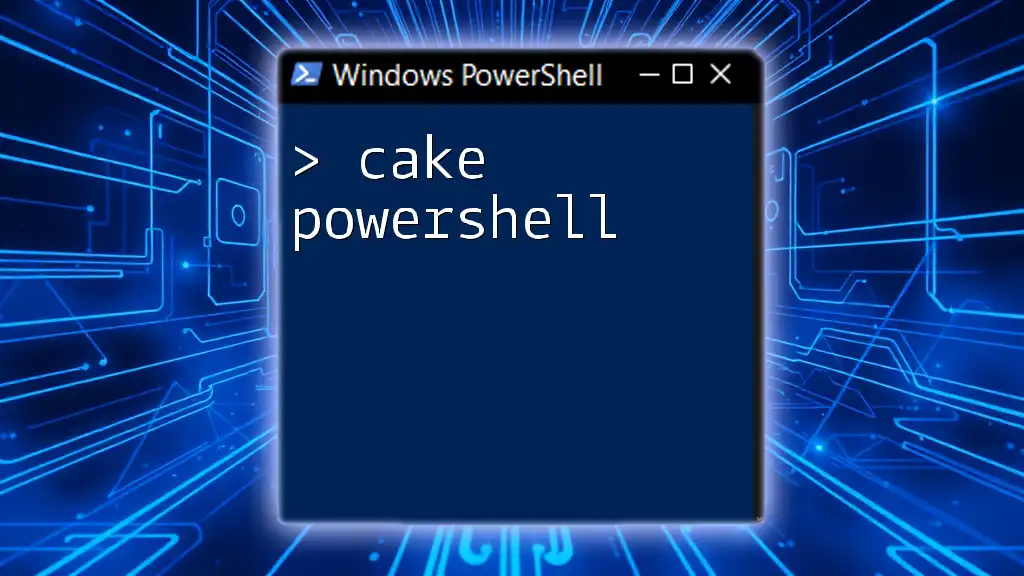
Call to Action
Enhance your PowerShell skills by subscribing to our teaching services for more in-depth tutorials! What class-based projects have you worked on? Share your experiences or questions in the comments below!