In PowerShell, the `-eq` operator is used to compare two values for equality, returning `$true` if they are equal and `$false` if they are not.
Here’s a simple example using the `-eq` operator in PowerShell:
$number = 5
if ($number -eq 5) {
Write-Host 'The number is five.'
} else {
Write-Host 'The number is not five.'
}
Understanding PowerShell Equality
What is Equality in PowerShell?
In programming, equality refers to the concept of determining whether two values are the same. In PowerShell, understanding equality is crucial for effective scripting, as it allows you to make decisions based on whether conditions are met. There are two main types of equality to consider: value equality and reference equality. Value equality checks if the actual values of two variables are the same, while reference equality checks if two variables point to the same object in memory.
PowerShell Comparison Operators
PowerShell provides a variety of comparison operators that allow you to evaluate conditions in scripts. One of the key operators is `-eq`, which is used specifically for equality comparisons. Other comparison operators include:
- `-ne`: Not equal
- `-lt`: Less than
- `-gt`: Greater than
These operators enable developers to create complex decision-making logic in their scripts.
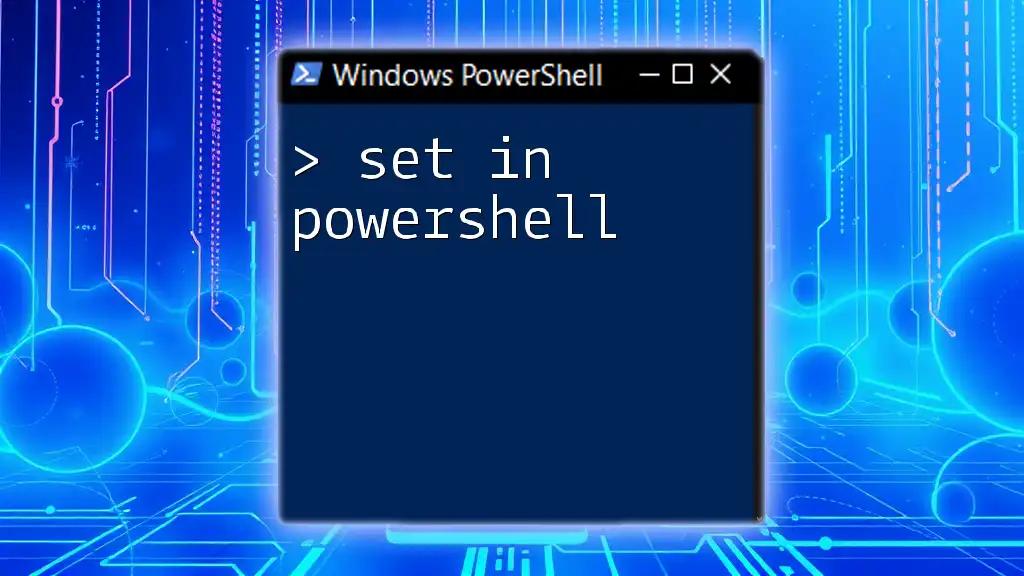
The `-eq` Operator in PowerShell
Syntax of the `-eq` Operator
The syntax for using the `-eq` operator is straightforward. The basic form looks like this:
$result = $value1 -eq $value2
In this example, if `$value1` is equal to `$value2`, the variable `$result` will be set to `True`. If they are not equal, it will be `False`. Understanding this syntax is key to using the operator effectively in various scenarios.
When to Use the `-eq` Operator
The `-eq` operator is particularly useful in numerous scripting scenarios. It is most appropriate when you need to compare values directly, such as integers, strings, and objects. While you should instinctively use `-eq` for equality checks, it is crucial to differentiate it from other operators such as `-ne`, which checks for inequality, and operators like `-lt` and `-gt`, which deal with numerical comparisons.

Practical Examples of Using the `-eq` Operator
Basic Example
A simple usage of the `-eq` operator can be demonstrated with numeric values:
$a = 10
$b = 10
if ($a -eq $b) {
"They are equal"
}
In this example, since both `$a` and `$b` hold the same value, the output will be "They are equal". This straightforward check forms the foundation of many conditional checks in PowerShell scripts.
Using `-eq` with Strings
String comparisons in PowerShell can be affected by case sensitivity. Consider the following example:
$string1 = "Hello"
$string2 = "hello"
if ($string1 -eq $string2) {
"Strings are equal"
} else {
"Strings are not equal"
}
In this case, the output will be "Strings are not equal" because PowerShell treats string literals as case-sensitive by default. This is an important point to remember, especially when comparing user input or working with string data.
Comparing Arrays with the `-eq` Operator
PowerShell also allows for equality checks between arrays, although the behavior may differ from scalar values. Here’s an example:
$array1 = 1, 2, 3
$array2 = 1, 2, 3
if ($array1 -eq $array2) {
"Arrays are equal"
} else {
"Arrays are not equal"
}
In this scenario, you might expect the arrays to be considered equal since they contain the same elements. However, PowerShell does not treat them as equal in this context because they are distinct objects. To compare the contents of arrays, you may need to use the `Compare-Object` cmdlet or other methods that focus on the value within the arrays.
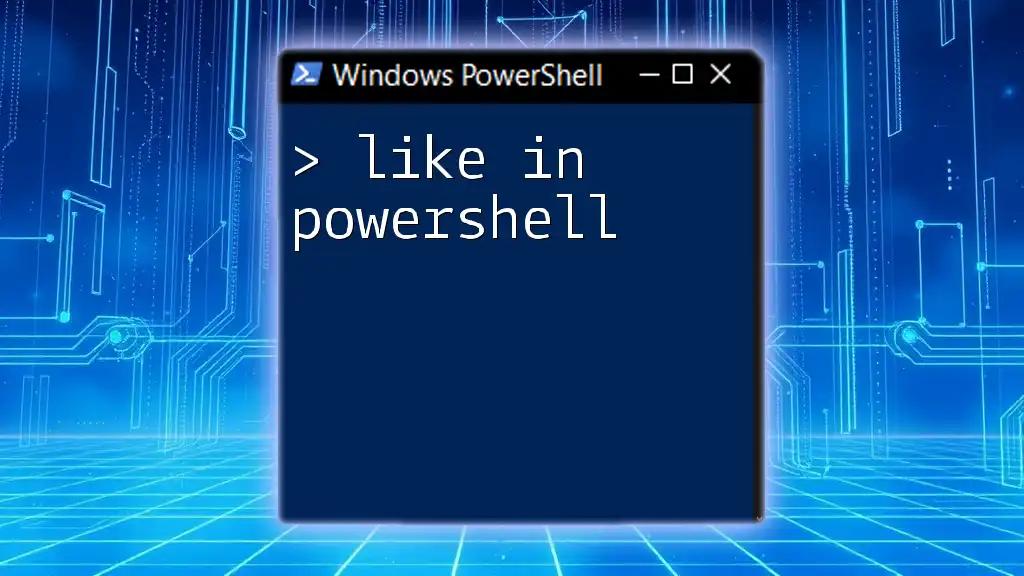
Advanced Usage of the `-eq` Operator
Using `-eq` in Conditional Statements
The `-eq` operator is particularly effective in conditional statements, such as `if` statements. Here’s an example that applies the operator to user inputs:
$userInput = Read-Host "Enter a number"
if ($userInput -eq "5") {
"You entered five!"
}
In this instance, the user's input is checked against the string value `"5"`. This approach can help guide script flows based on user decisions, making your scripts interactive and dynamic.
Using `-eq` in Loops
The `-eq` operator can also enhance looping structures, enabling checks within iterations. For example:
$numbers = 1..10
foreach ($number in $numbers) {
if ($number -eq 5) {
"Found five!"
}
}
In this case, when the loop encounters the number `5`, the output will read "Found five!". This illustrates how `-eq` can facilitate targeted processing in standard loops.
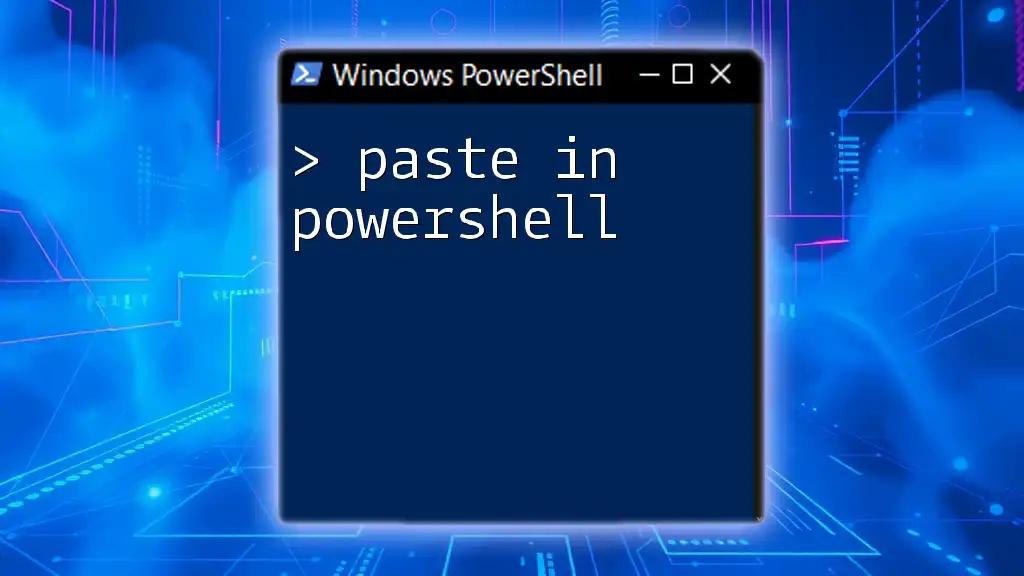
Common Pitfalls and Troubleshooting
Type Casting and the `-eq` Operator
Implicit and explicit type casting issues can arise when using the `-eq` operator. If the types of the variables differ, it can lead to unexpected behaviors. Here is an example of such a case:
$num = 5
if ($num -eq "5") {
"Types match"
}
Despite the differences in type (integer vs. string), PowerShell will evaluate this as true due to its intelligent type handling. Nonetheless, it is essential to be aware of how types are evaluated to avoid confusion.
Null Values and Equality Checks
Handling null values is critical when performing equality checks. Consider the following scenario:
$value = $null
if ($value -eq $null) {
"Value is null"
}
In this case, the script correctly identifies that `$value` is indeed null, demonstrating the need for careful null handling in your scripts, especially when operating on data that may contain uninitialized or empty values.
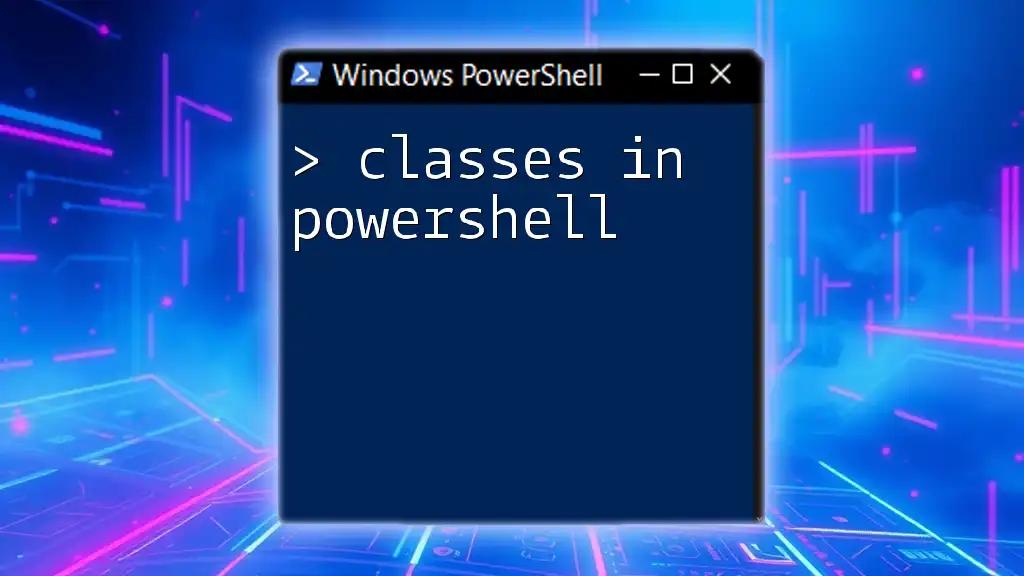
Conclusion
Understanding the `eq in PowerShell` is essential for effective scripting and automation. Its applications range from basic comparisons to complex conditional logic in loops and user input scenarios. By mastering the `-eq` operator, you can enhance your scripting capabilities and improve the control flow in your PowerShell projects.
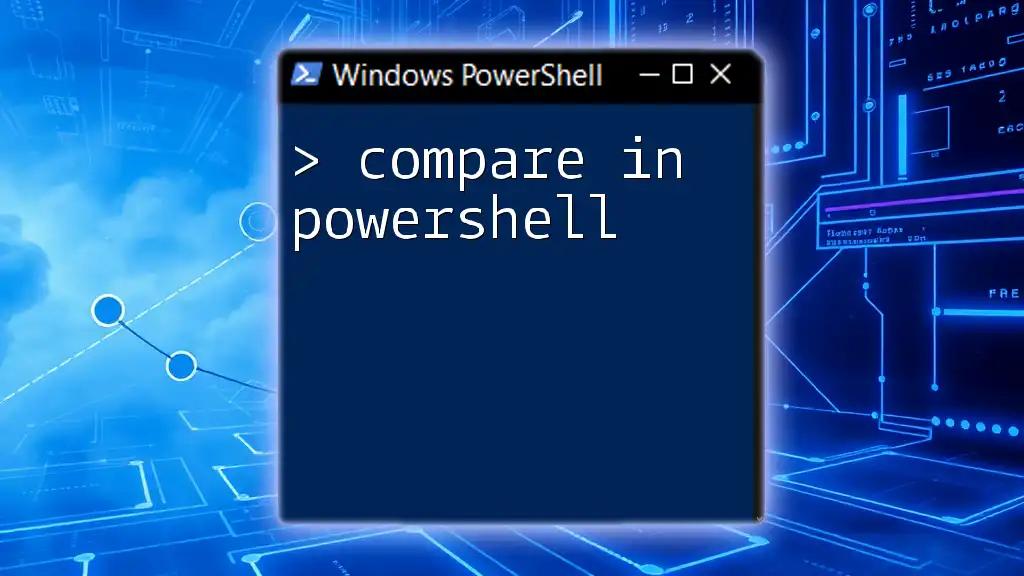
Additional Resources
To further develop your skills in PowerShell, consider exploring recommended books, online courses, and the official PowerShell documentation. Engaging with community forums can also provide invaluable support and insights into best practices and troubleshooting techniques.