The `xcopy` command in PowerShell is a file management utility that allows users to copy files and directories from one location to another, even including subdirectories.
Here’s a code snippet using `xcopy` in PowerShell:
xcopy "C:\SourceFolder\*" "D:\DestinationFolder\" /E /I
Understanding xcopy
What is xcopy?
`xcopy` is an extended version of the basic `copy` command in Windows that enables users to copy files and directories, including subdirectories, from one location to another. Its enhanced functionality makes it an invaluable tool for system administrators and users tasked with managing file systems. Unlike the `copy` command, which can only handle files, `xcopy` can support more complex copying needs, such as selectively copying files based on criteria like timestamps.
Basic Syntax of xcopy
The basic syntax of the `xcopy` command is structured as follows:
xcopy [source] [destination] [options]
- source: The path to the file or folder you want to copy.
- destination: The path where you want to copy the file or folder.
- options: Various parameters that modify the behavior of the command.
Understanding this syntax is crucial as it forms the foundation upon which all `xcopy` operations are built.
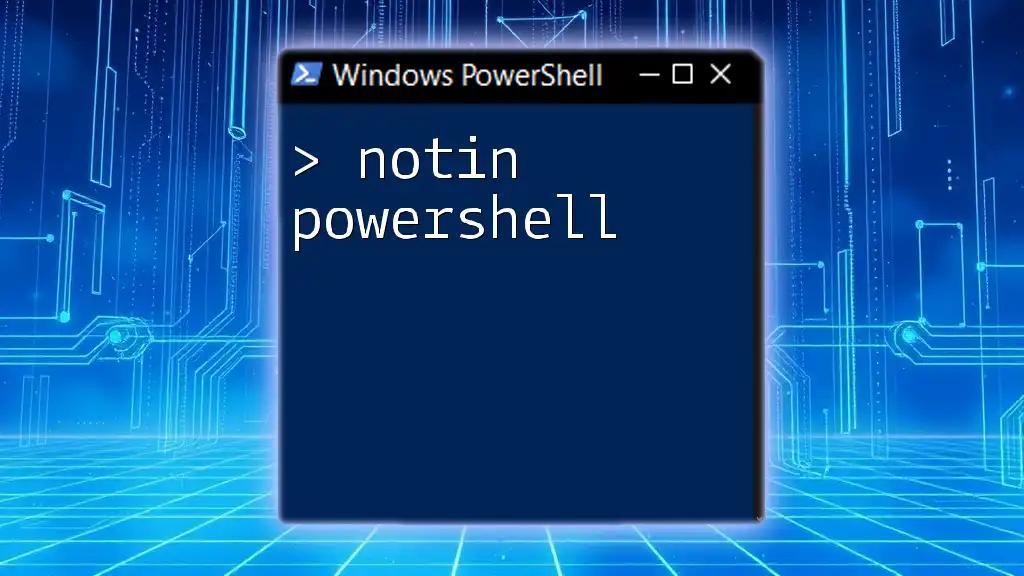
Using xcopy in PowerShell
Basic Usage of xcopy in PowerShell
In PowerShell, invoking `xcopy` is quite straightforward. One of the simplest implementations would be to copy files from one directory to another without any added parameters. Here’s an example:
xcopy C:\SourceFolder D:\TargetFolder
In this command, PowerShell will copy all files from `C:\SourceFolder` to `D:\TargetFolder`. If `D:\TargetFolder` doesn't exist, PowerShell will prompt you to confirm whether or not to create it.
Common Options and Their Uses
`xcopy` comes with several options that can dramatically alter its behavior and the results of your operations. Here, we will explore some of the most common options and their implications.
Overview of xcopy Options
-
/S: This option copies directories and subdirectories except for empty ones. It’s useful when you want a mirrored structure but do not want to clutter the destination with blank folders.
-
/E: Similar to `/S`, but this option also includes empty directories. If you need every folder to be mirrored, including those that are empty, this is the option for you.
-
/I: This option tells `xcopy` to assume the destination is a directory if you are copying multiple files and the destination does not exist. This can prevent unnecessary prompts and streamline your workflow.
-
/Y: By using this option, you can suppress prompts that request confirmation before overwriting a file. This is particularly useful for batch scripts or automated tasks.
Example Scenarios with Code Snippets
To illustrate the use of `xcopy` options, here are a few practical examples:
-
Example 1: Copy with subdirectories, excluding empty ones
xcopy C:\SourceFolder D:\TargetFolder /S
-
Example 2: Copy with all directories, including empty ones
xcopy C:\SourceFolder D:\TargetFolder /E
-
Example 3: Overwrite files without confirmation
xcopy C:\SourceFolder D:\TargetFolder /Y
Each of these snippets showcases how you can leverage options to tailor your `xcopy` commands to your specific needs.
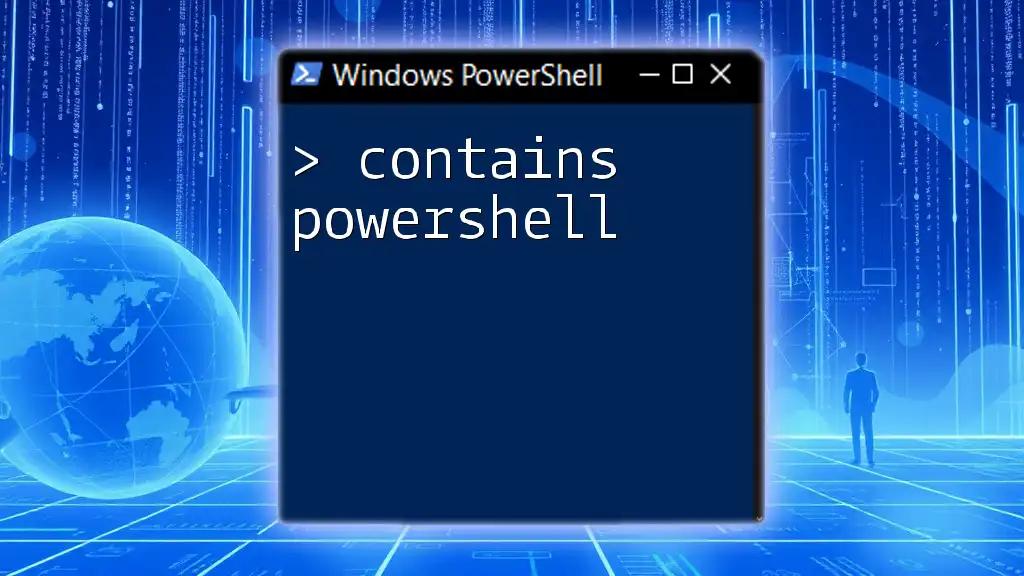
Advanced xcopy Techniques
Using xcopy with Variables
PowerShell allows for the use of variables, which can be particularly handy for frequently changing paths. By defining your source and destination in variables, you can create a more dynamic script:
$source = "C:\SourceFolder"
$destination = "D:\TargetFolder"
xcopy $source $destination /S
This flexibility makes it easy to modify just the variable values while maintaining a clean code structure.
Error Handling and Logging
When working with file copy commands, it's essential to be aware of potential errors that may occur, such as inadequate permissions or the target path being too long. Proper error handling is crucial to maintaining system integrity and data quality.
Understanding Potential Issues
Common errors include:
- Access Denied: Ensure you have appropriate privileges.
- Path Not Found: Double-check the source and destination paths.
Logging xcopy Operations
To track the outcomes of your copy operations, you can log the output. Capturing the results can be invaluable for troubleshooting and verification:
xcopy C:\SourceFolder D:\TargetFolder /S > C:\Logs\xcopy_log.txt
This command not only performs the copy operation but also captures the output in a log file, providing a record of what files were copied and any errors that may have occurred.

Alternatives to xcopy in PowerShell
Introduction to robocopy
While `xcopy` is a powerful tool, sometimes a more robust alternative like `robocopy` (Robust File Copy) is necessary. `robocopy` boasts several advantages over `xcopy`, such as:
- Resilient copying over network paths
- Multi-threaded capabilities for faster copying
- Extensive logging and error-handling features
In situations where you must ensure high reliability and comprehensive features, consider switching to `robocopy`.
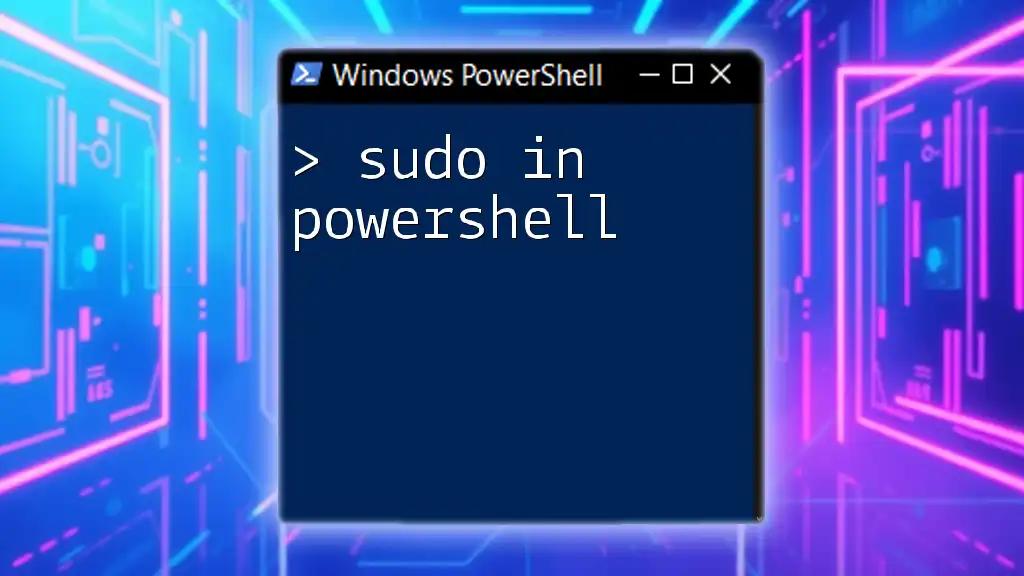
Best Practices for Using xcopy
Performance Considerations
To ensure you're using `xcopy` efficiently, consider the context of your file transfers. Using /J can significantly speed up the transfer of large files, as it will copy files using unbuffered I/O.
When to Choose xcopy over Other Commands
Choose `xcopy` when you need a simple, effective way to copy files and directories without the need for intricate options. It is suitable for straightforward tasks, particularly within scripts that do not require advanced operation features.
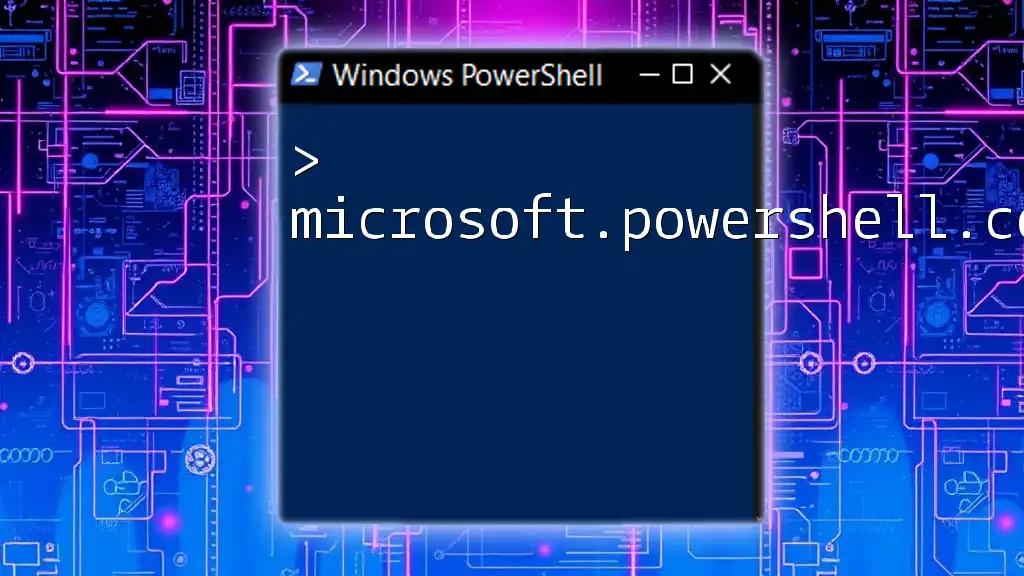
Conclusion
In summary, using xcopy in PowerShell provides a powerful means to manage file transfers effectively. From its basic syntax to advanced usage techniques, mastering `xcopy` can dramatically enhance your efficiency when working with files on Windows systems. Whether you are just getting started or looking to bolster your scripting capability, `xcopy` remains an essential command in the PowerShell arsenal.