In PowerShell, a "for loop" is used to execute a block of code a specified number of times, iterating over a range of values or an array.
for ($i = 0; $i -lt 5; $i++) { Write-Host "Iteration $i" }
Understanding Loops in PowerShell
What is a Loop?
In programming, a loop is a fundamental concept that allows you to execute a block of code repeatedly until a specified condition is met. Loops are vital in automating repetitive tasks, making your scripts more efficient and concise. In PowerShell, there are several types of loops, including the for loop, while loop, do-while loop, and foreach loop. Each has its particular use case and advantages.
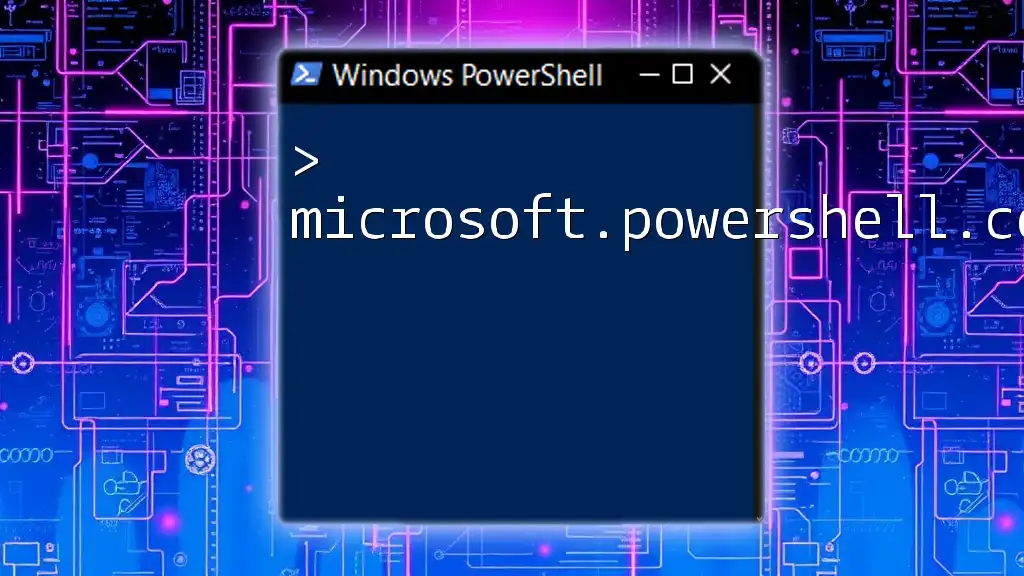
PowerShell For Loops: An Overview
What is a For Loop?
A for loop is a control flow statement designed to execute a set of instructions multiple times in a controlled manner. It is particularly useful when the number of iterations is known beforehand. Understanding how to implement a for loop in PowerShell will significantly enhance your scripting capabilities, enabling you to automate complex workflows easily.
Syntax of a PowerShell For Loop
The syntax of a for loop in PowerShell follows a specific structure. It consists of three main components: initialization, condition, and increment. Here is a basic format:
for ($i = 0; $i -lt 10; $i++) {
# Code to execute
}
- Initialization: This part initializes the loop control variable (in this case, `$i`).
- Condition: The loop continues executing as long as this condition is true (`$i -lt 10`).
- Increment: This updates the loop control variable after each iteration (`$i++` means increment `$i` by 1).
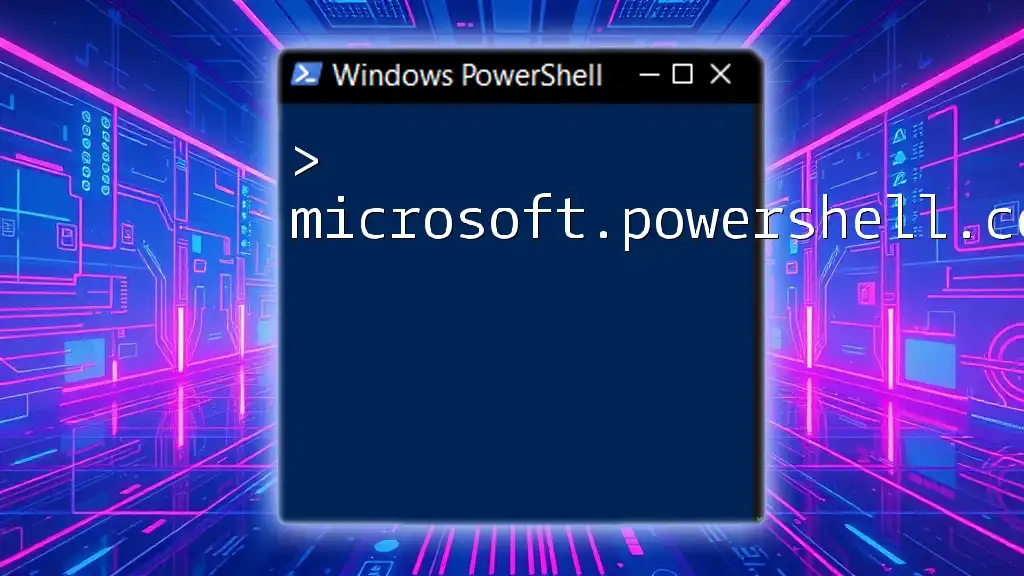
Practical Examples of PowerShell For Loops
Example 1: Basic For Loop
To illustrate how a for loop functions, let's implement a basic example that prints the numbers 1 through 10.
for ($i = 1; $i -le 10; $i++) {
Write-Host $i
}
In this example, the loop starts with `$i` initialized to 1 and runs until `$i` is less than or equal to 10. Each number is printed to the console with `Write-Host`, resulting in a list of numbers from 1 to 10.
Example 2: Looping Through an Array
Another common use case for for loops is to iterate over an array. This example demonstrates how to print the elements of an array.
$array = @( 'Apple', 'Banana', 'Cherry' )
for ($i = 0; $i -lt $array.Length; $i++) {
Write-Host $array[$i]
}
Here, `$array` contains three fruits. The loop iterates from index 0 to the length of the array, printing each fruit. This syntax makes it easy to work with data collections, allowing you to access individual elements efficiently.
Example 3: Using a For Loop to Perform Calculations
Let’s consider a scenario where we want to calculate the square of numbers from 1 to 5. The loop executes a set of calculations, demonstrating how for loops can be used in arithmetic operations.
for ($i = 1; $i -le 5; $i++) {
$square = $i * $i
Write-Host "The square of $i is $square"
}
In this code snippet, each iteration calculates the square of `$i` and outputs it using `Write-Host`. The expected output will display squares from 1 to 5:
The square of 1 is 1
The square of 2 is 4
The square of 3 is 9
The square of 4 is 16
The square of 5 is 25
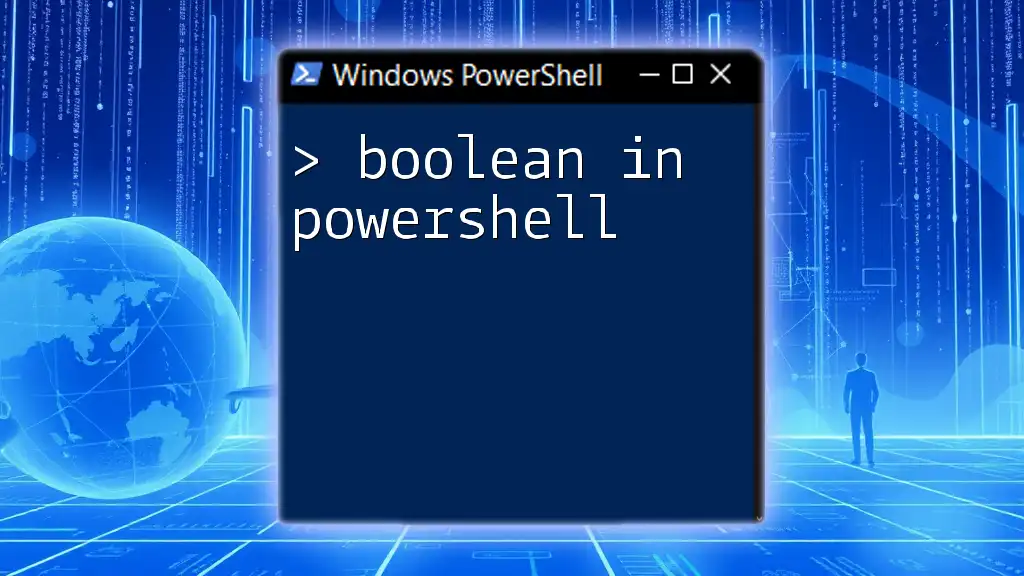
Combining For Loops with Conditional Statements
Using If Statements in For Loops
Enhancing a for loop with conditional statements allows for more complex logic. By adding an `if` statement within a loop, you can execute certain actions based on specific conditions.
For example, let's print only the even numbers from 1 to 10.
for ($i = 1; $i -le 10; $i++) {
if ($i % 2 -eq 0) {
Write-Host "$i is even"
}
}
In this snippet, the condition `$i % 2 -eq 0` checks if `$i` is even. If true, it prints that `$i` is even. This method effectively demonstrates how combining loops and conditionals can create more dynamic scripts.
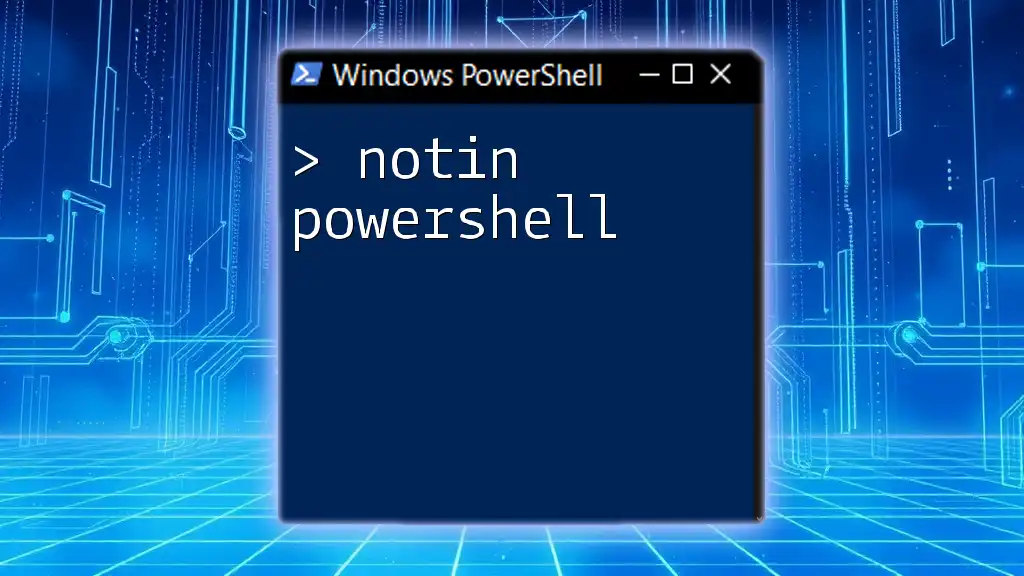
Best Practices for Using For Loops in PowerShell Scripts
When writing PowerShell loop scripts, there are several best practices to keep in mind:
- Clarity and Readability: Always aim to write code that is easy to understand. Use meaningful variable names to clarify their purpose.
- Avoiding Infinite Loops: Ensure that your loop condition will eventually become false; otherwise, you may end up in an infinite loop. For example, changing the increment or misplacing conditions can create unintended behavior.
- Optimization: Evaluate whether a for loop is the best choice for your task. Sometimes, a `foreach` loop may be more appropriate for iterating through collections.
- Commenting Your Code: Including comments helps clarify your logic, especially in complex loops.
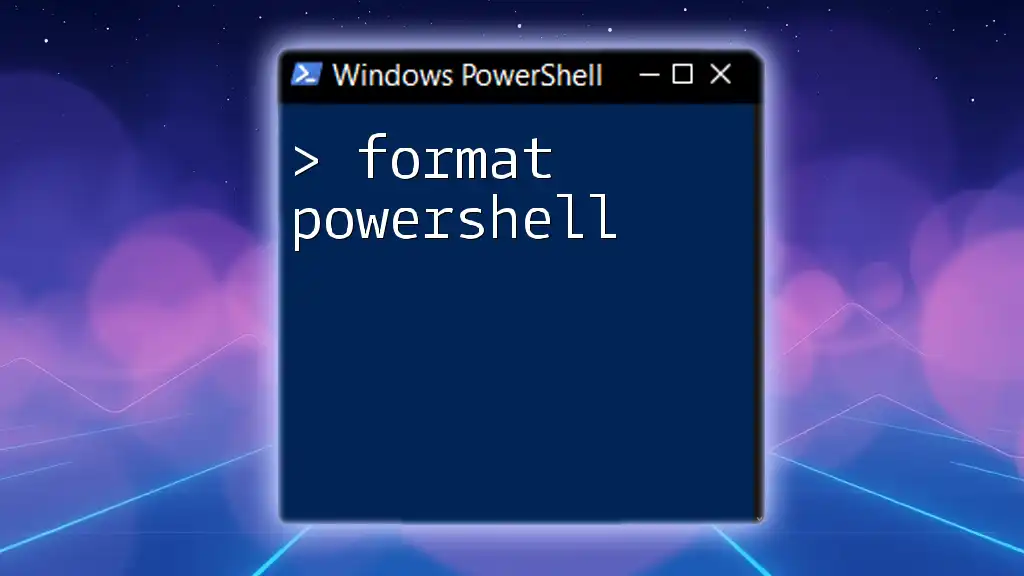
Conclusion
Mastering the for loop in PowerShell is essential for automating repetitive tasks and improving your scripting efficiency. By understanding its syntax, exploring practical examples, and implementing best practices, you can write more effective and powerful PowerShell scripts. As you expand your knowledge, continue to experiment with different types of loops to unlock additional capabilities that will enhance your scripting prowess.
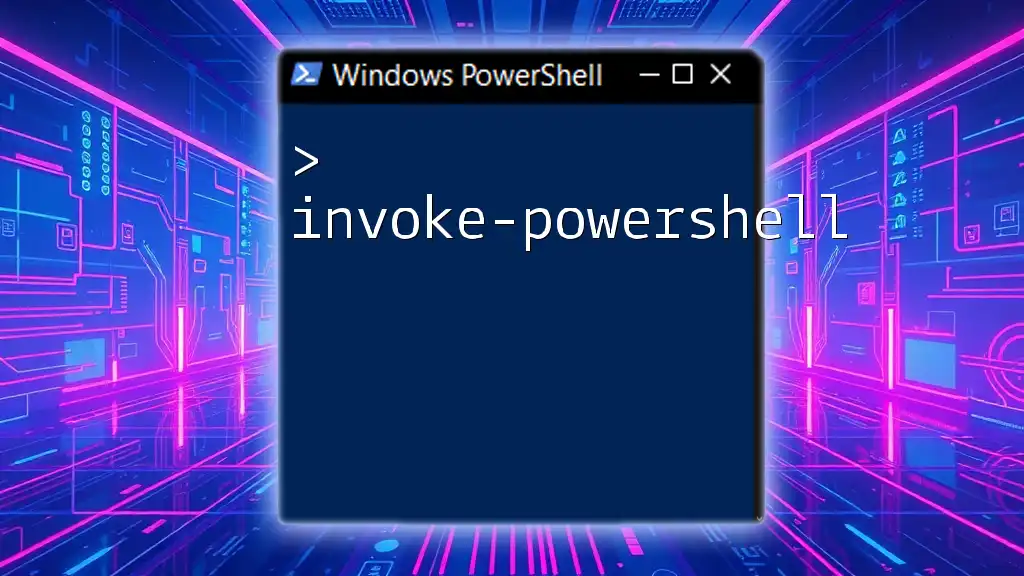
Call to Action
Share your experience with for loops in PowerShell or pose any questions you may have. Engaging with the community can foster further learning and improvement. Check out additional resources or related articles for deeper insights and advanced techniques in PowerShell scripting.