In PowerShell, you can retrieve the value of a variable by simply using its name, which allows you to access or manipulate the stored data in your script. Here's a code snippet that demonstrates how to declare and get the value of a variable:
$greeting = 'Hello, World!'
Write-Host $greeting
Exploring the Get-Variable Cmdlet
What is Get-Variable?
The `Get-Variable` cmdlet in PowerShell is a powerful tool that allows you to retrieve variable information in your current session. When you work with PowerShell scripts, variables play a crucial role in storing data, making `Get-Variable` essential for managing and monitoring these variables effectively.
Basic Syntax of Get-Variable
The basic syntax for using `Get-Variable` is as follows:
Get-Variable [-Name <String[]>] [-Scope <String>] [-Force] [<CommonParameters>]
Understanding this syntax is fundamental to utilizing this cmdlet effectively. Here’s a breakdown of the primary parameters:
- -Name: Specifies the name of the variable or variables you wish to retrieve.
- -Scope: Determines the scope from which to retrieve the variable, which can be useful in complex scripts.
- -Force: Ignores the restrictions imposed by the scope.
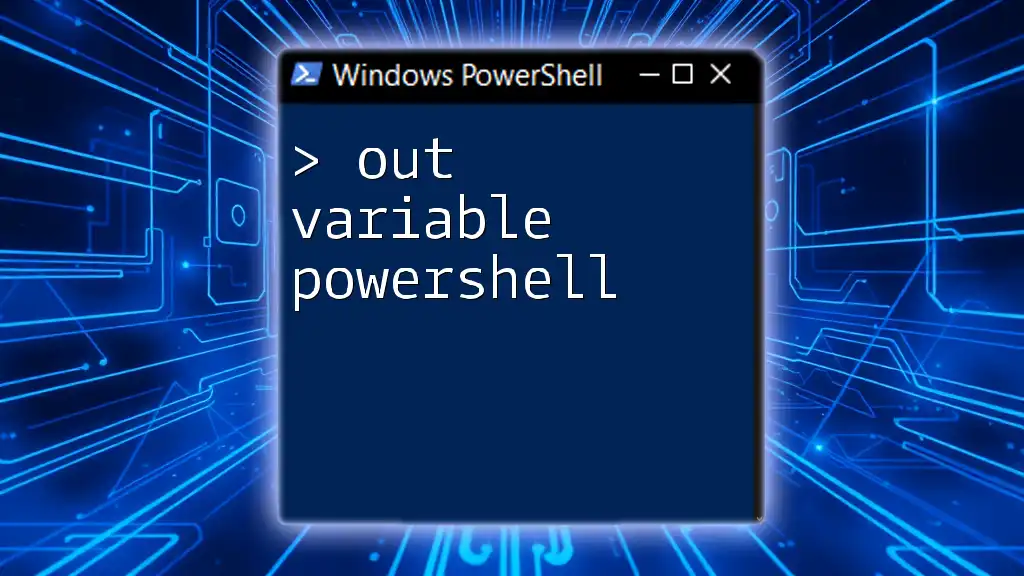
Using Get-Variable in PowerShell
Retrieving All Variables
To get started, you might want to retrieve all variables currently defined in your PowerShell session. Simply use:
Get-Variable
This command will list all existing variables, along with their values and their associated scopes. The output format will include the variable names across the top, followed by their values and other metadata in rows beneath. This can be incredibly useful for debugging and validation purposes.
Filtering Variables
Using the -Name Parameter
If you are interested in a specific variable, you can use the `-Name` parameter to filter by name:
Get-Variable -Name "myVariable"
This command will return only the variable named `myVariable`. If the variable exists, you'll see its value and properties; if it doesn't, PowerShell will simply return nothing. This targeted approach is efficient for large scripts where tracking numerous variables can become cumbersome.
Using Wildcards
You might also want to filter variables using wildcards, allowing you to match multiple variables with similar names:
Get-Variable -Name "var*"
In this case, `Get-Variable` will return all variables whose names begin with "var". This feature is especially helpful when dealing with arrays or collections of variables that share a naming convention.
Inspecting Variable Values
When you want to inspect the actual value of a variable, it's as simple as wrapping the `Get-Variable` access in a variable assignment:
$value = Get-Variable -Name "myVariable"
$value.Value
Here, you retrieve `myVariable` and then access its `.Value` property. This approach is vital when you need to work with variable values directly, perhaps for further processing or conditional operations.
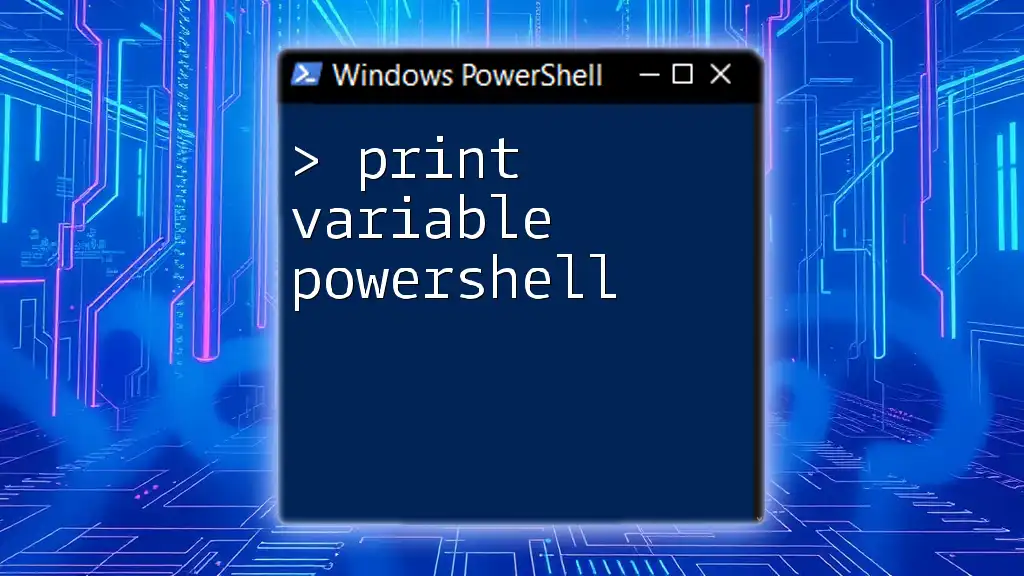
Using Scopes with Get-Variable
Understanding Scopes
In PowerShell, understanding variable scope is crucial. Scope refers to the visibility of a variable within different parts of your script or session. The primary scopes are:
- Global: Accessible from anywhere in your session.
- Local: Available only within the function or script it was declared.
- Script: Available throughout the script but not outside of it.
- Private: Accessible only within the script or function where it's defined.
Retrieving Scoped Variables
You can use `Get-Variable` to access variables in different scopes effectively. For instance, if you need to retrieve global variables, you would use:
Get-Variable -Scope Global
This command will list all variables that are accessible globally, which is particularly useful when you’ve been working with multiple scripts that might interact with the same variables.
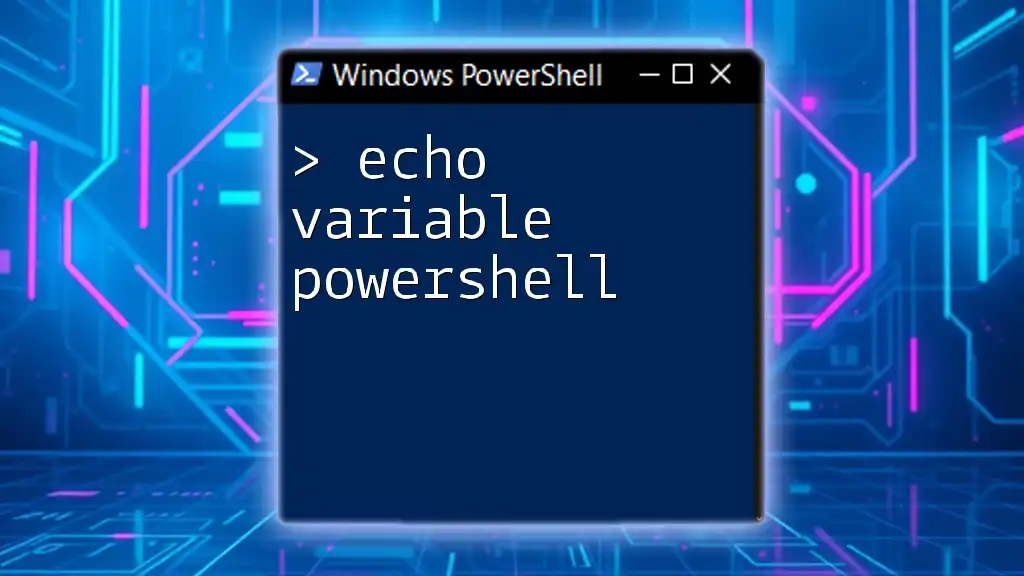
Practical Examples
Example 1: Listing all variables in your script
A great practice is to list all defined variables for quick reference in your script:
# This script lists all defined variables for quick reference
Get-Variable
Utilizing this command helps you quickly audit what variables are available and can assist in troubleshooting issues related to variable management.
Example 2: Monitoring Variable Changes
If you're monitoring changes in a variable, you can store its value and retrieve it later to track modifications, like so:
$myVar = 'Initial Value'
$myVar = 'Updated Value'
Get-Variable -Name 'myVar'
The code snippet above shows how to assign and then change the value of `myVar`. Running `Get-Variable` afterward would indicate the current state, which is valuable for understanding how data flows throughout your script.
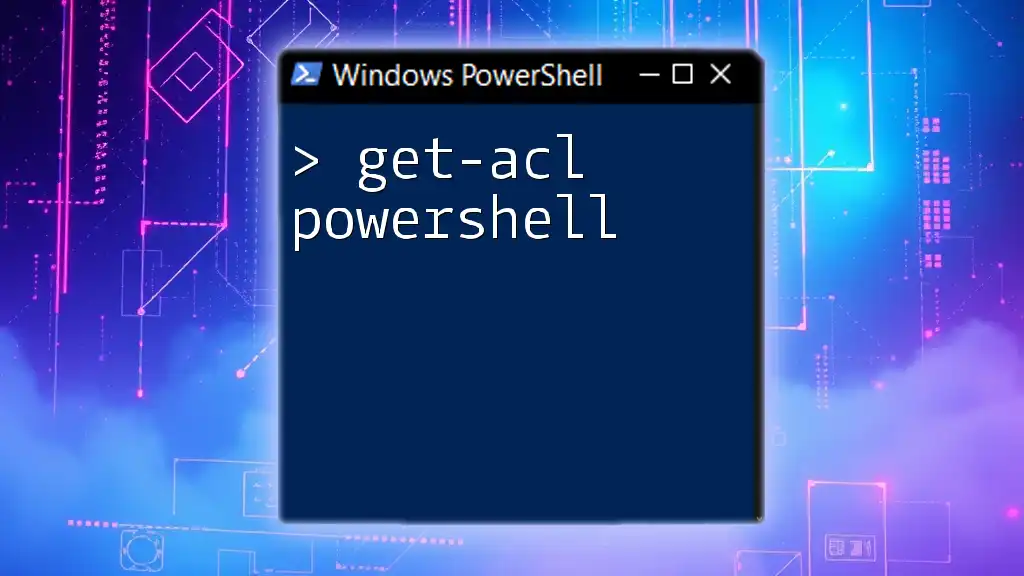
Best Practices with Variables in PowerShell
Naming Conventions
Establishing clear naming conventions for your variables is key. Use descriptive names that reflect the data held in the variable, making your scripts more readable and maintainable.
Scope Awareness
Be aware of the scope in which you're defining your variables. Using scope thoughtfully can prevent variable name conflicts and improve the predictability of your scripts. Always check if a variable exists in the desired scope before creating a new one.
Clearing Variables
To maintain a clean environment and prevent memory issues, remove or clear variables when they are no longer needed:
Remove-Variable -Name 'myVar'
This command is a simple way to declutter your session, ensuring that old or unused variables do not interfere with your current operations.
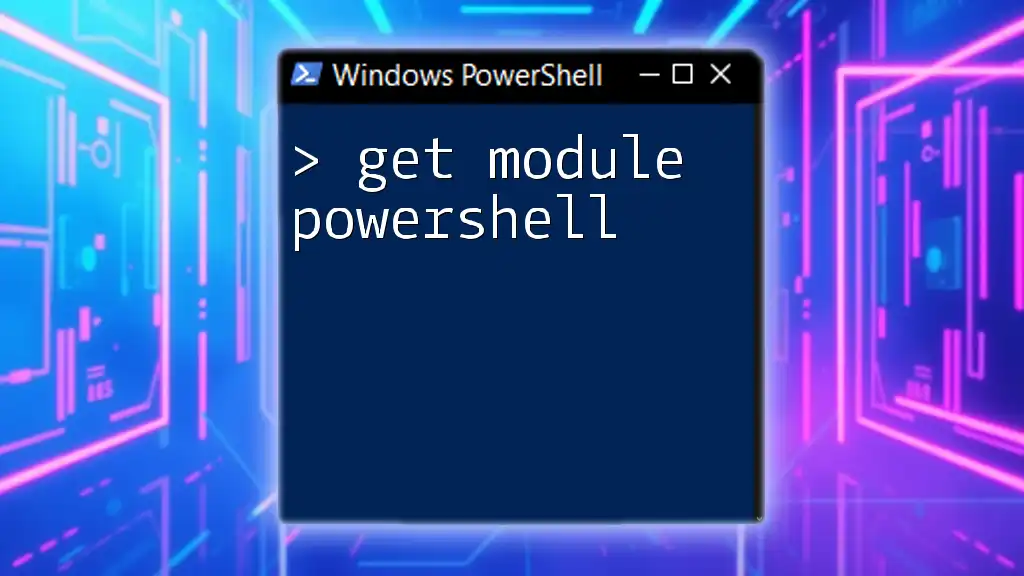
Conclusion
By mastering the `Get-Variable` cmdlet, you're effectively enhancing your PowerShell fluency and script management capabilities. The ability to easily retrieve, access, and manage your variables will significantly streamline your coding process. Don’t forget to practice these techniques regularly, as familiarity will drive your success in scripting with PowerShell.
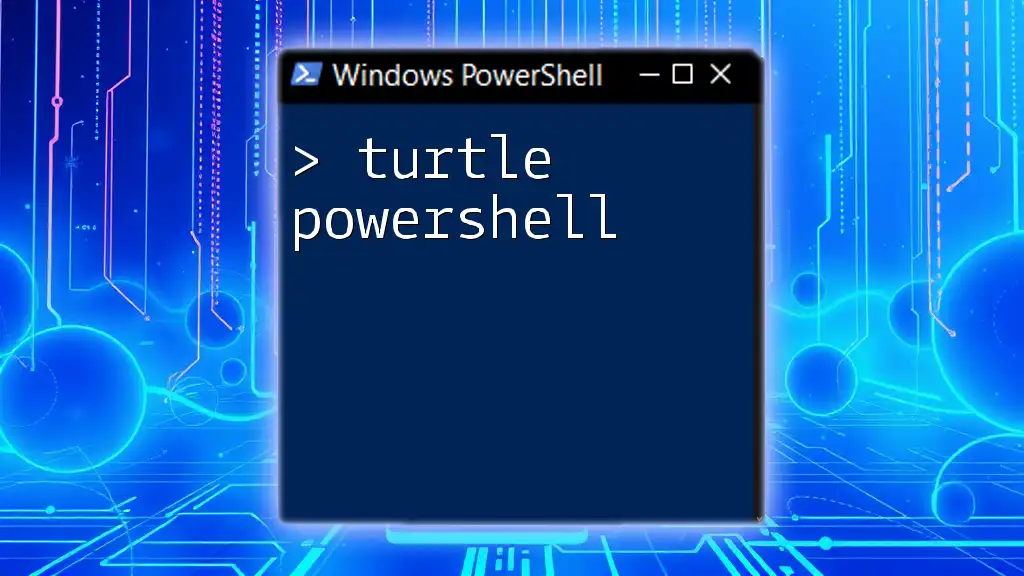
Call to Action
Join our community today for more PowerShell insights and tutorials! Feel free to leave comments or questions about `Get-Variable` or any other PowerShell topics you wish to explore further. Your engagement is valuable to us!