In PowerShell, the `echo` command is used to display the value of a variable, allowing you to easily output information to the console. Here's how you can do it:
$greeting = 'Hello, World!'
echo $greeting
What is a Variable in PowerShell?
Definition of Variables
In PowerShell, a variable is essentially a storage location that can hold data. This data can be of different types, such as strings (text), integers (numbers), or even more complex types like arrays and objects. Variables are integral to scripting as they allow you to manipulate and store data dynamically.
The syntax for defining a variable in PowerShell is straightforward. You start with a dollar sign (`$`), followed by the variable name. Here’s how you would define a simple variable:
$greeting = "Hello, PowerShell!"
Types of Variables
PowerShell supports several types of variables. Here are a few common types:
-
String: A series of characters enclosed in quotes. For example:
$name = "Alice"
-
Integer: A whole number without any decimal point. For example:
$age = 30
-
Array: A collection of items. Arrays can hold multiple values:
$fruits = @("Apple", "Banana", "Cherry")
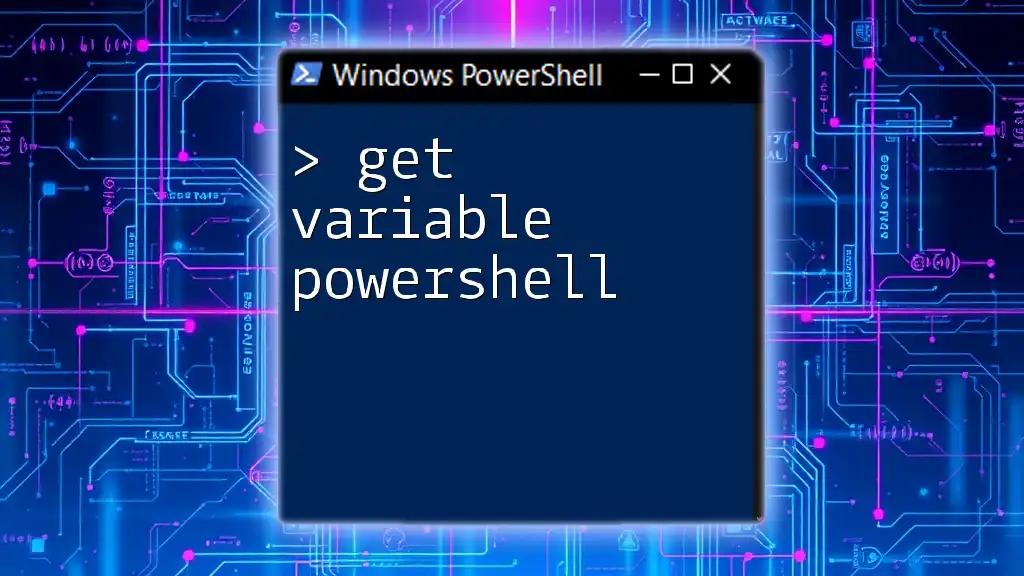
The Echo Command in PowerShell
Understanding "Echo"
The `echo` command in PowerShell is an alias for `Write-Output`. It is primarily used to display output to the console. Understanding how to use `echo` is essential for scripting, as it allows you to provide feedback or display results dynamically.
Basic Usage of the Echo Command
Using `echo` is incredibly simple. You can use it to output strings directly. For example:
echo "Hello, World!"
This will display the message "Hello, World!" in the PowerShell console.
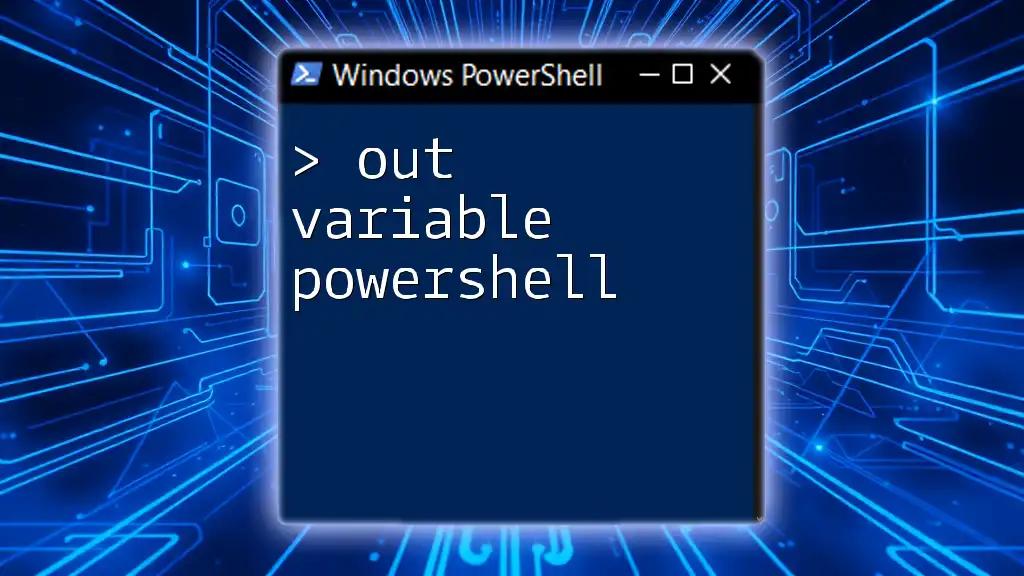
How to Echo a Variable in PowerShell
Assigning a Value to a Variable
The first step is to assign a value to a variable. For example:
$greeting = "Welcome to PowerShell!"
In this case, `$greeting` now holds the string "Welcome to PowerShell!".
Using Echo to Display a Variable
Once you have a variable defined, you can easily use the `echo` command to display its contents:
echo $greeting
Running this will output: `Welcome to PowerShell!`.
Demonstrating Echo with Different Variable Types
String Variables
Let’s take a string variable and display it using `echo`:
$name = "Alice"
echo "Hello, $name!"
This will output: `Hello, Alice!`.
Integer Variables
You can also echo an integer variable. Consider the following example:
$age = 30
echo "You are $age years old."
The output here will be: `You are 30 years old.`
Arrays
Echoing an array variable requires a slightly different approach to ensure that the output is properly formatted. Here’s how to echo the values of an array:
$fruits = @("Apple", "Orange", "Banana")
echo "Fruits: $($fruits -join ', ')"
The command will output: `Fruits: Apple, Orange, Banana`.
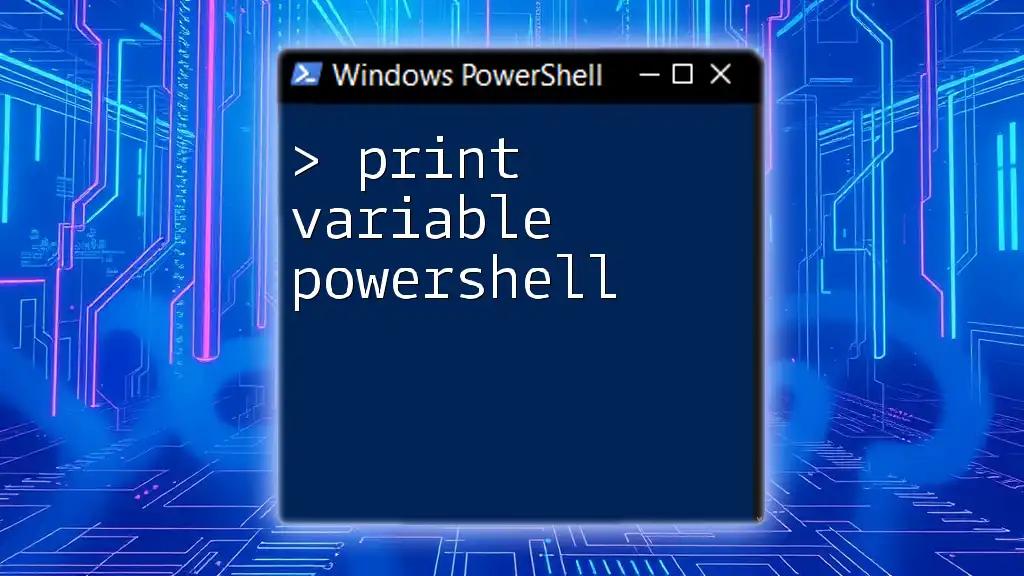
Best Practices for Echoing Variables in PowerShell
Using Subexpressions
When you need to perform operations within an echo statement, using subexpressions can be vital. Subexpressions allow you to calculate or manipulate values right within your output. For example:
$value = 10
echo "The double of $value is $($value * 2)."
The output will be: `The double of 10 is 20.`
Quoting and Handling Special Characters
It’s crucial to quote strings correctly, particularly if your variable holds paths or string literals that may contain special characters. Here's an example:
$path = "C:\Users\Alice\Documents\"
echo "Your path is: $path"
The output will show the correct file path.
Combining Strings and Variables
Combining strings and variables effectively can help format your output more elegantly. You can use string interpolation or traditional concatenation. Here’s an example:
$firstName = "John"
$lastName = "Doe"
echo "Full Name: $firstName $lastName"
This line will result in: `Full Name: John Doe`.
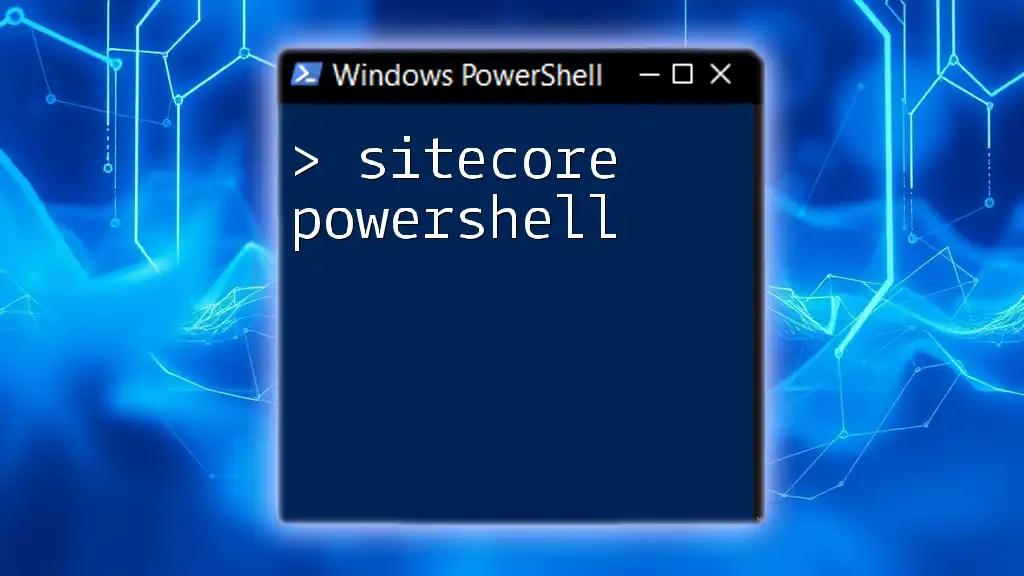
Common Pitfalls When Echoing Variables
Forgetting to Use `$` for Variables
One common mistake is forgetting to use the `$` sign before a variable name. Here's an example of what not to do:
echo "Your name is name." # Incorrect
In this case, `name` was intended to be a variable but was not defined with `$`. The correct version should be:
$name = "Alice"
echo "Your name is $name." # Correct
Output Formatting Issues
Improper formatting can lead to confusion. Always ensure you’re spacing your strings correctly and using quotes when necessary. Not paying attention to these details can make your outputs less readable.
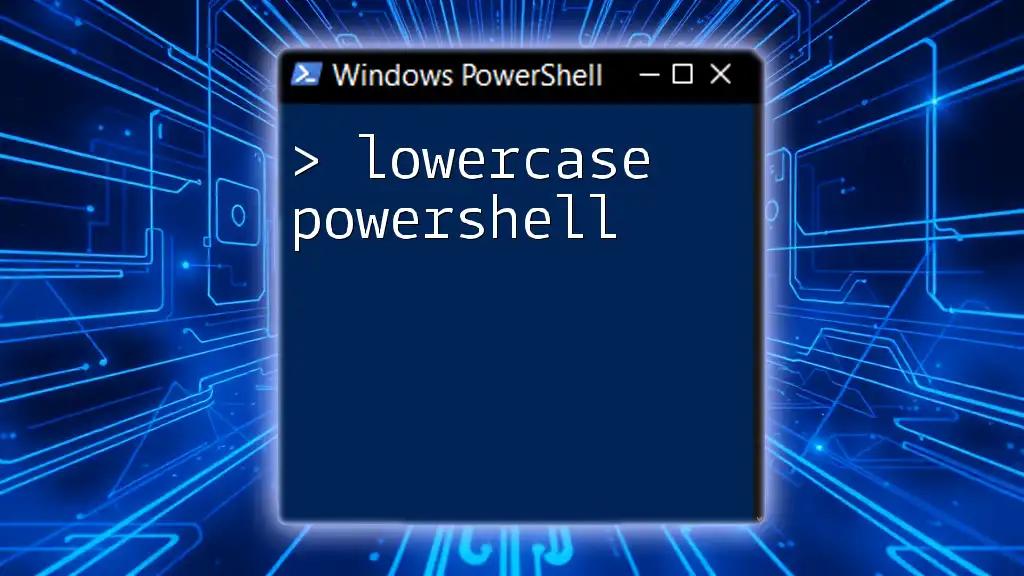
Conclusion
In conclusion, mastering how to echo variables in PowerShell is a fundamental skill that can significantly enhance your scripting capabilities. Utilizing the `echo` command effectively will allow you to deliver dynamic and informative outputs tailored to your needs.
Practice with various data types, operations, and outputs to become proficient in using PowerShell to its fullest. Each step you take will build your confidence and capabilities in automation and scripting.
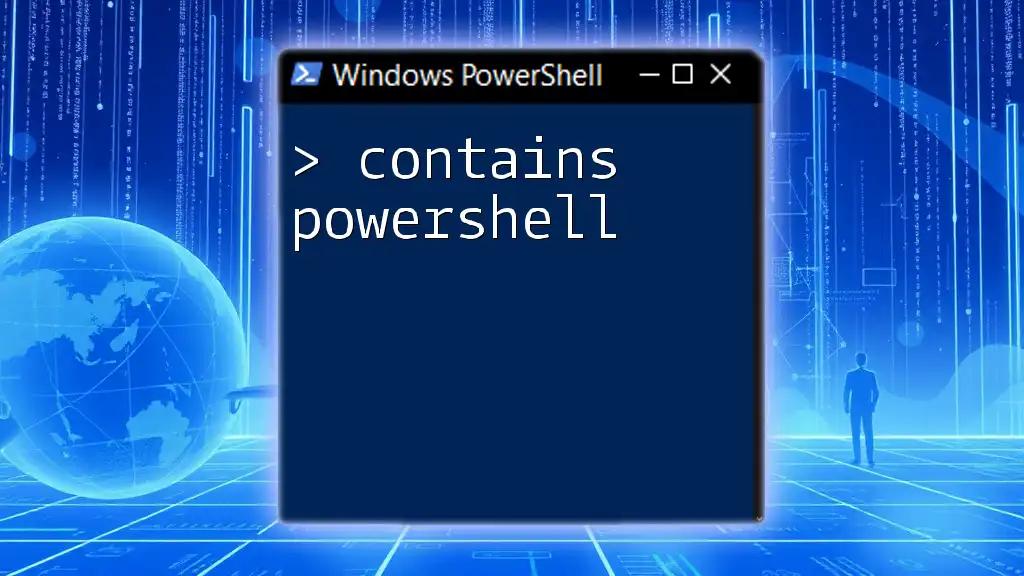
Additional Resources
For further learning, consult the official PowerShell documentation and explore more advanced scripting techniques that can take your PowerShell skills to the next level. Happy scripting!