Batch files can serve as a bridge to execute PowerShell commands seamlessly, allowing users to automate tasks and streamline workflows efficiently. Here’s a simple code snippet that demonstrates running a PowerShell command from a batch file:
@echo off
powershell -command "Write-Host 'Hello, World!'"
What are Batch Files?
Batch files, often with the `.bat` extension, are simple text files that contain a series of commands executed by the Windows Command Prompt. Their primary purpose is to automate repetitive tasks. Common uses include automating software installations, running diagnostics, and executing maintenance scripts.
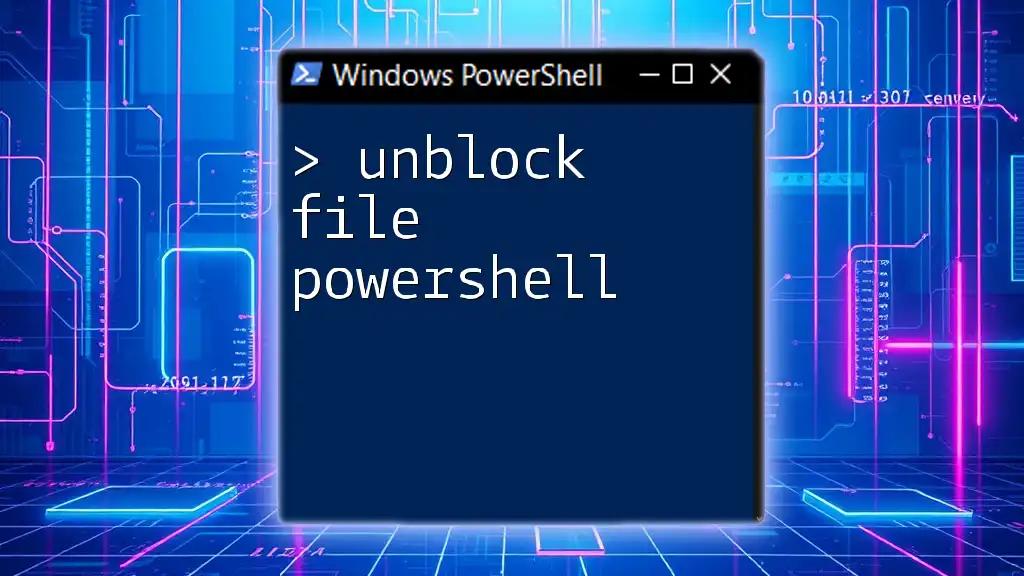
Understanding PowerShell
PowerShell is a powerful scripting language and command-line shell designed for system administration and automation. Unlike traditional command shells, PowerShell enables users to perform more complex tasks by leveraging .NET framework capabilities, making it highly versatile for managing system configurations.

Why Use PowerShell from Batch Files?
Integrating PowerShell with batch files opens up a world of possibilities. Batch files can be used to leverage PowerShell's powerful cmdlets and features without needing to learn an entirely new syntax. This combination is particularly beneficial for users transitioning from traditional scripting to PowerShell scripting. Real-world applications include automating system updates, managing Active Directory, and deploying software across many machines.
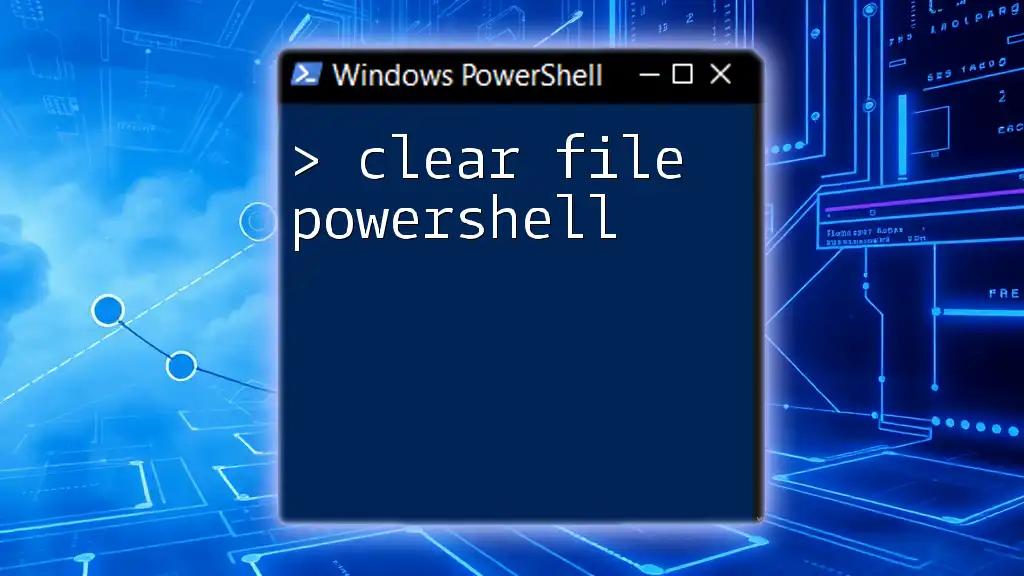
Creating a Basic Batch File to Run PowerShell Scripts
Setting Up Your Environment
Before creating batch files to execute PowerShell scripts, ensure you have the required tools:
- A text editor (like Notepad or Visual Studio Code).
- Access to the Windows Command Prompt with administrative privileges, if necessary.
Creating a Simple Batch File
To create a batch file, simply open your text editor, type the commands, and save the file with a `.bat` extension. Here's an example of a basic batch file that calls PowerShell:
@echo off
powershell -ExecutionPolicy Bypass -File "C:\Path\To\YourScript.ps1"
Explanation of the Code:
- `@echo off` hides the command execution for cleaner output.
- `powershell -ExecutionPolicy Bypass` allows the script to run regardless of the current execution policy set in PowerShell, which is crucial when executing scripts that are not digitally signed.
- `-File "C:\Path\To\YourScript.ps1"` specifies the path to the PowerShell script you wish to execute.
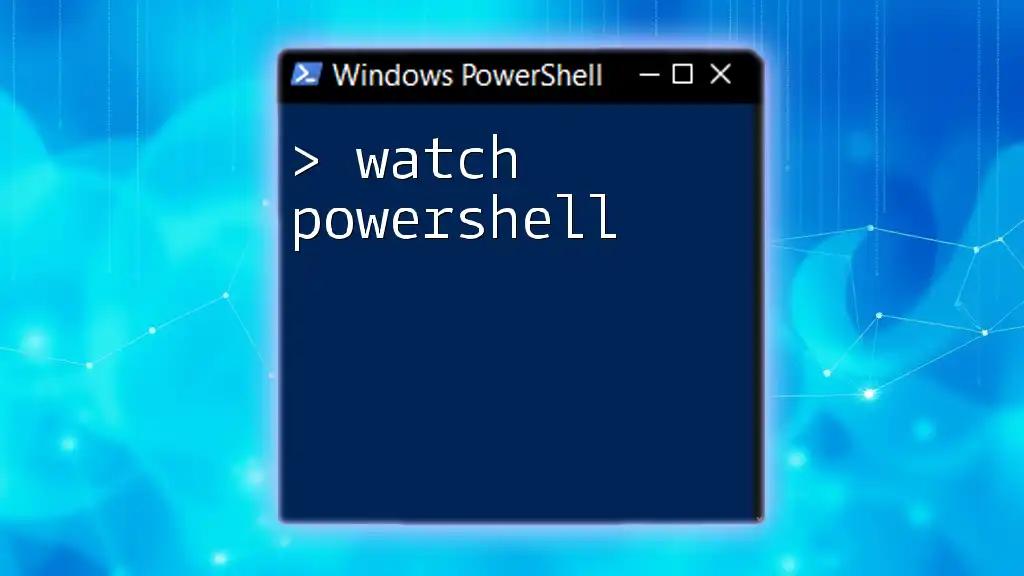
How to Execute a PowerShell Script from a Batch File
Using PowerShell Command in Batch Files
To call a PowerShell script from a batch file, you need to invoke the `powershell` command and specify the script file.
Example: Executing a PowerShell Script
Here’s a straightforward example of executing a PowerShell script from a batch file:
@echo off
powershell -File "C:\Path\To\YourScript.ps1"
The key point to note is that if PowerShell's execution policy is restrictive, your script may not run. You can temporarily bypass this with the `-ExecutionPolicy Bypass` option, as shown previously.
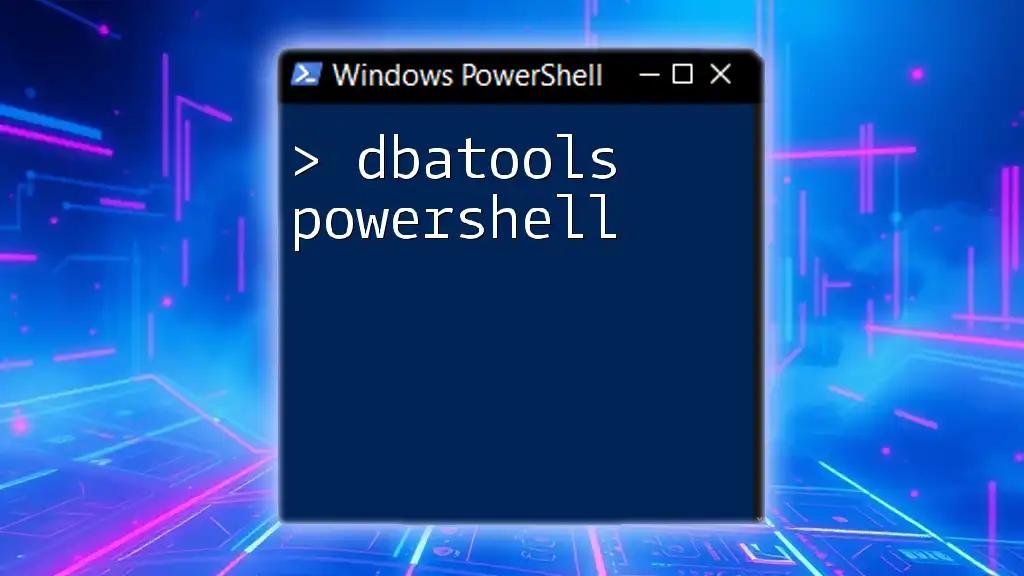
Passing Arguments from Batch Files to PowerShell Scripts
Why Pass Arguments?
Enabling your scripts to accept parameters increases flexibility and allows for dynamic operations based on the input you provide.
How to Pass Parameters
To pass parameters from your batch file to a PowerShell script, define the parameters in the PowerShell script and reference them in the batch file.
Example of Parameterized Batch File:
@echo off
set param1=value1
powershell -File "C:\Path\To\YourScript.ps1" -param1 %param1%
In this example:
- `set param1=value1` defines a variable that can be used as an argument.
- `-param1 %param1%` passes this value into the PowerShell script.

Error Handling and Debugging in PowerShell Scripts Called from Batch Files
Common Errors When Running PowerShell from Batch
When executing PowerShell scripts via batch files, common pitfalls include:
- Execution Policy Issues: If the policy is too strict, scripts may be blocked.
- Path Errors: Ensure the script paths are correctly specified.
Debugging Tips
To effectively troubleshoot your scripts, incorporate error handling within PowerShell:
try {
# Your code here
} catch {
Write-Host "Error: $_"
}
Using `try-catch` blocks allows you to capture and output specific errors, preventing crashes.
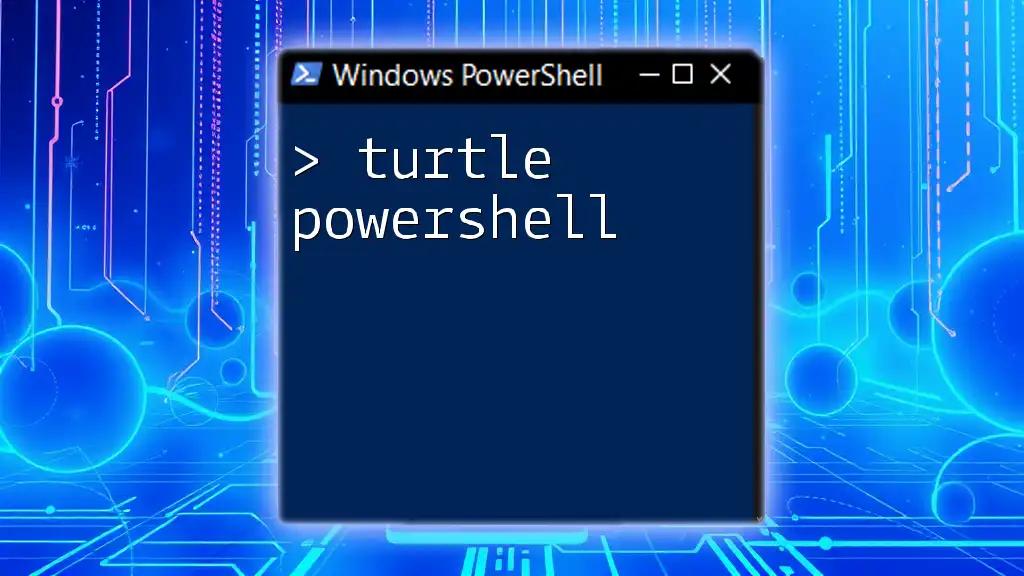
Converting Existing Scripts: From Batch to PowerShell
Why Consider Migration?
Migrating from batch to PowerShell can streamline processes and leverage more advanced capabilities offered by PowerShell, such as object-oriented programming and built-in cmdlets.
How to Convert a Batch File to PowerShell
Here’s a fundamental conversion example. Consider the following batch file code:
@echo off
echo Hello, World!
The equivalent PowerShell code would be:
Write-Host "Hello, World!"
The conversion involves understanding the differences and adapting the syntax accordingly.
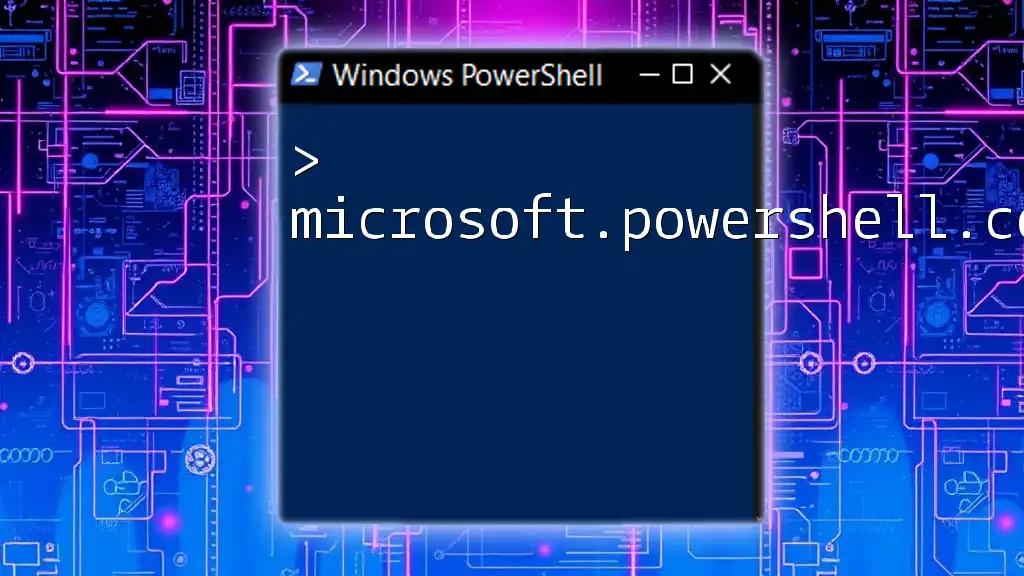
Advanced Techniques: Running Multiple PowerShell Scripts from Batch Files
Batch Execution of Multiple Scripts
You can execute multiple PowerShell scripts sequentially by placing additional `powershell` commands in your batch file:
@echo off
powershell -File "C:\Path\To\FirstScript.ps1"
powershell -File "C:\Path\To\SecondScript.ps1"
This code segment sequentially runs two PowerShell scripts one after the other.
Running PowerShell Script with Conditional Logic
You can add conditional execution within your batch file using `if` statements. Here’s a practical example:
@echo off
if exist "C:\Path\To\YourScript.ps1" (
powershell -File "C:\Path\To\YourScript.ps1"
) else (
echo Script not found!
)
This ensures that the script only runs if it exists at the specified location, providing error feedback when it does not.
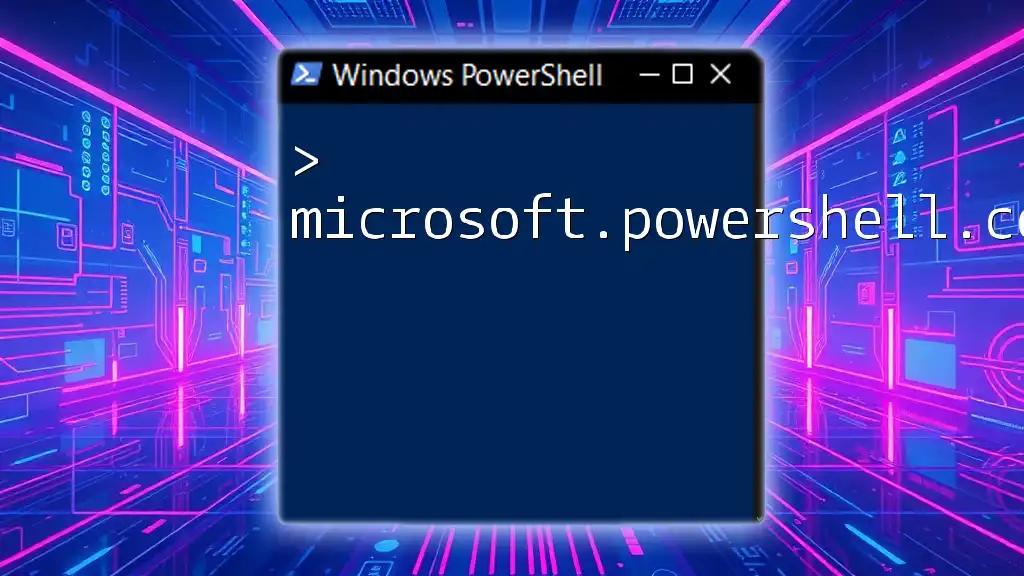
Conclusion: Mastering PowerShell through Batch Files
Exploring the integration of batch file PowerShell can significantly enhance your automation capabilities. By mastering this technique, you can efficiently automate tasks, streamline operations, and gradually transition to more advanced PowerShell scripting. Make sure to experiment with the concepts discussed, and seek resources to expand your knowledge and expertise further.
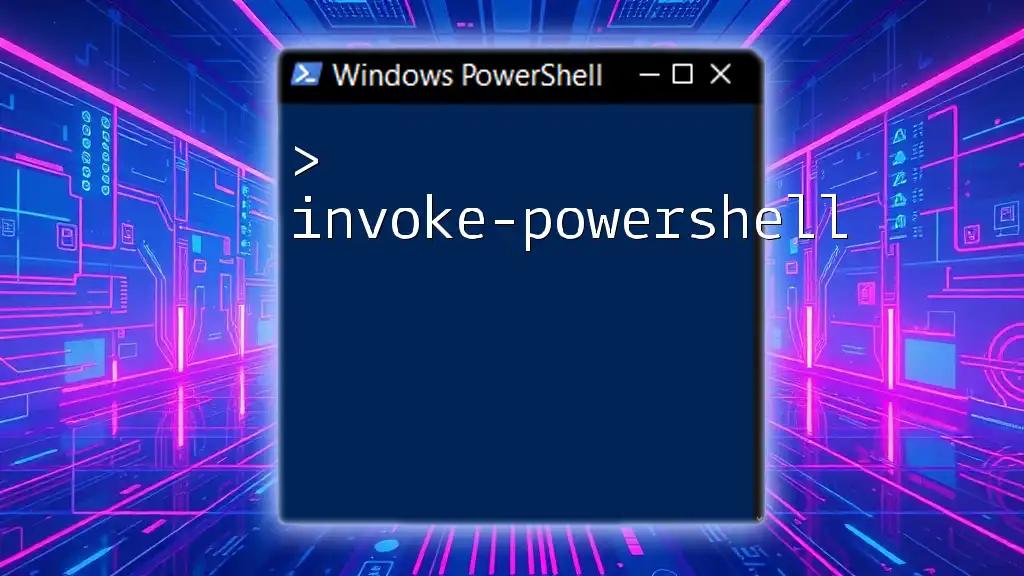
FAQ Section
- How do I create a PowerShell Batch File?
- Can I run a PowerShell script silently?
- What should I do if my PowerShell scripts won't execute from Batch?
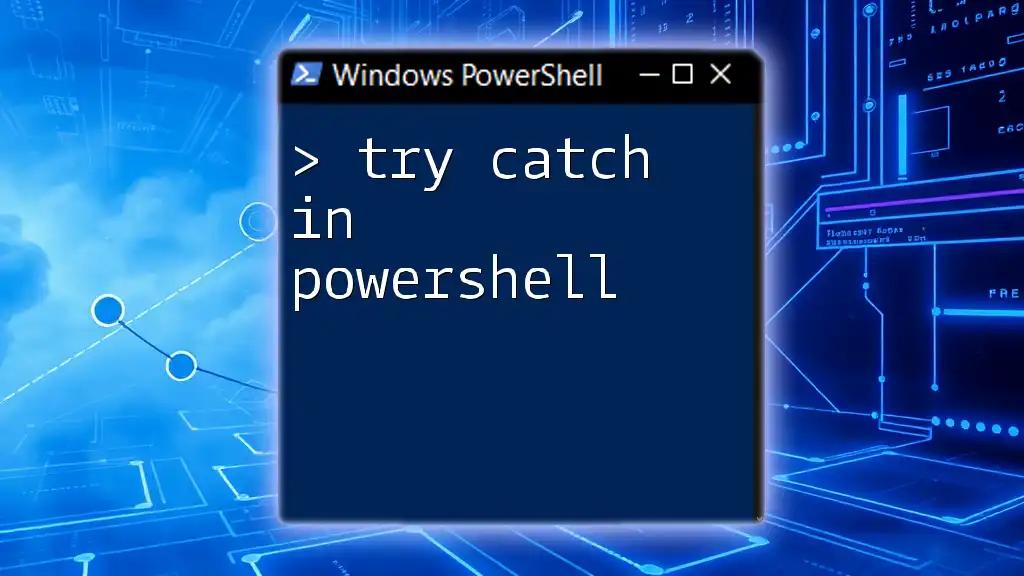
Call to Action
Take the plunge! Experiment with creating and executing your PowerShell scripts from batch files. Sign up for our course or newsletter to continue your learning journey in mastering PowerShell and scripting automation!