To unblock a downloaded file in PowerShell, you can use the `Unblock-File` cmdlet, which removes the security warning associated with files downloaded from the internet.
Unblock-File -Path "C:\path\to\your\file.txt"
Understanding File Blocking in Windows
What is File Blocking?
File blocking refers to a security feature in Windows that protects users from potentially harmful files, particularly those downloaded from the internet. When a file is downloaded, Windows may mark it as unsafe, preventing accidental execution or usage. This is commonly seen as files from the internet are tagged to indicate their origin, commonly referred to as "blocked" files. Recognizing these blocked files helps users avoid actions that might expose them to risks, such as malware or unwanted software.
Why Use PowerShell to Unblock Files?
Using PowerShell provides a powerful alternative to traditional file unblocking methods, such as right-clicking a file and selecting "Unblock" from the properties menu. Here are several reasons to prefer PowerShell:
- Automation: PowerShell allows for efficient handling of bulk operations, saving time and effort.
- Scripting: You can create scripts to perform file unblocking tasks repeatedly, which is especially useful in environments where many files require unblocking regularly.
- Remote Management: PowerShell can be used to unblock files on remote systems, which is invaluable for system administrators.
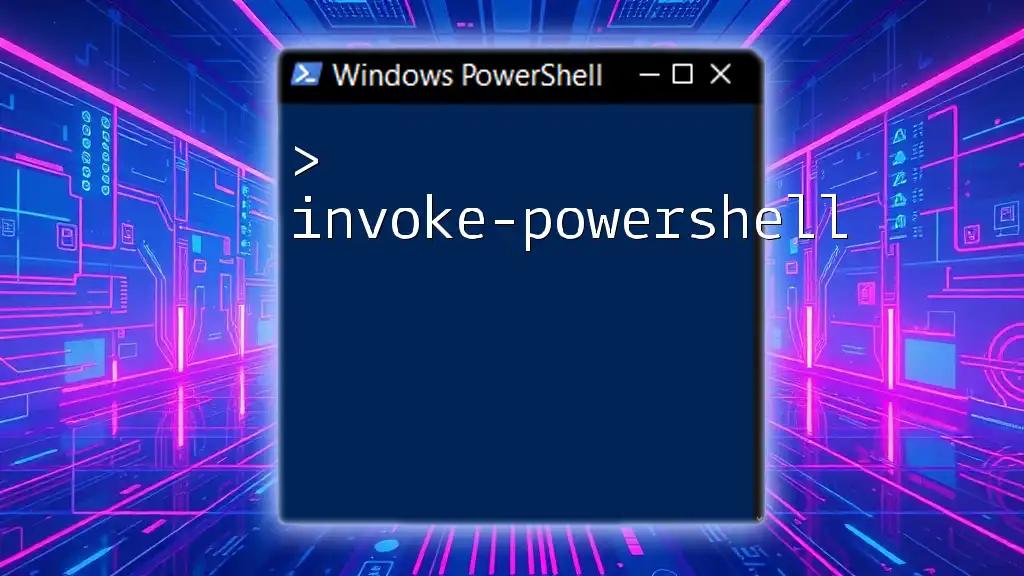
How File Blocking Works in Windows
The Zone Identifier
The Zone Identifier is an NTFS alternate data stream that Windows attaches to files to indicate their origin. This attribute tells the operating system whether the file was downloaded from the internet, providing a security layer before executing these files. In order to see the Zone Identifier for a file, users can run the following command in PowerShell:
Get-Item "C:\Path\To\Your\File.txt" -Stream Zone.Identifier
If the file has been blocked, the information will display, confirming its status.
File Extension Considerations
Certain file types are more likely to be blocked by Windows, such as executables (.exe) and compressed files (.zip). However, not all files are automatically blocked. Files that Windows deems safe (based on type or source) may bypass this protection altogether. Understanding which types of files are subject to blocking can help users anticipate the need for unblocking.
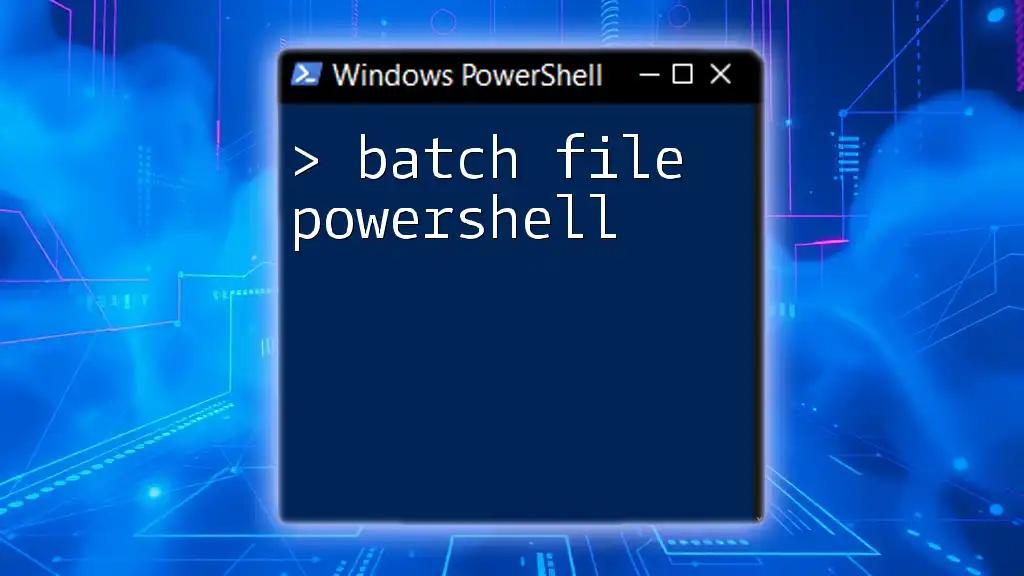
Using PowerShell to Unblock Files
The `Unblock-File` Cmdlet
The `Unblock-File` cmdlet is a simple yet effective PowerShell command used for unblocking files. This command targets the Zone Identifier of the file, removing the blocking tag and allowing the user to access the file freely. The syntax is straightforward:
Unblock-File -Path <filepath>
Step-by-Step Instructions to Unblock a File
Unblocking a Single File
To unblock a single file, simply execute the command as follows:
Unblock-File -Path "C:\Path\To\Your\File.txt"
After running this command, the file will no longer be blocked, allowing you to open or run the file without issues. Always check if the file is from a trusted source to avoid potential risks.
Unblocking Multiple Files
PowerShell makes it easy to unblock multiple files at once, which can be particularly beneficial if you’ve downloaded a batch of files. Here’s how to do it using wildcards:
Unblock-File -Path "C:\Path\To\Your\*.txt"
For a more advanced scenario, suppose you want to unblock all text files in a specific directory, you can use a loop with `Get-ChildItem`:
Get-ChildItem "C:\Path\To\Your\Folder" -Filter *.txt | Unblock-File
This command retrieves all the `.txt` files in the specified directory and unblocks each one seamlessly.
Unblocking Files from Specific Locations
Unblocking Files in a Specific Folder
If you need to unblock all files within a specific folder, simply run the following command:
Get-ChildItem "C:\Path\To\Your\Folder" | Unblock-File
This command iterates through every file in the designated folder and unblocks them effectively.
Unblocking Files Recursively
To unblock files in subdirectories of a specified folder, use the `-Recurse` flag with `Get-ChildItem`:
Get-ChildItem "C:\Path\To\Your\Folder" -Recurse | Unblock-File
This command allows you to unblock all applicable files not just in the folder, but also in any subfolders, ensuring comprehensive unblocking.
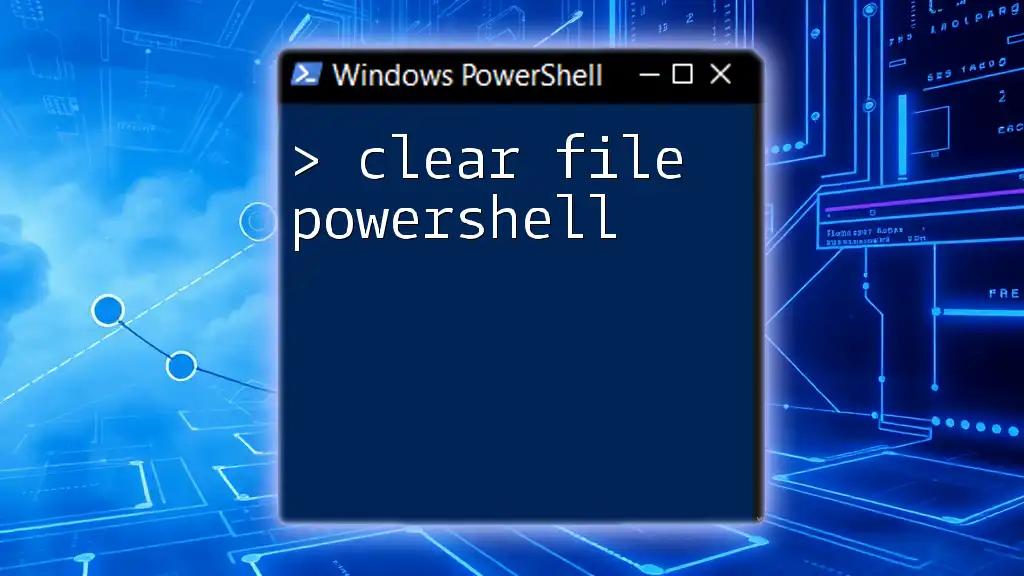
Verification After Unblocking
Confirming File Status
Once you have unblocked files, it's wise to confirm their status. You can check whether a file remains blocked by using the following command:
Get-Item "C:\Path\To\Your\File.txt" | Select-Object -ExpandProperty AlternateDataStream
If the file is unblocked, the Zone Identifier will not appear.
Common Issues and Troubleshooting
While using `Unblock-File`, you may encounter various error messages, such as permission issues or file not found errors. If you run into problems, consider the following troubleshooting steps:
- Ensure you have the necessary permissions to unblock the file.
- Confirm that the file path is accurate and the file exists at that location.
- If the file resides on a networked drive, check for connectivity issues or access rights.
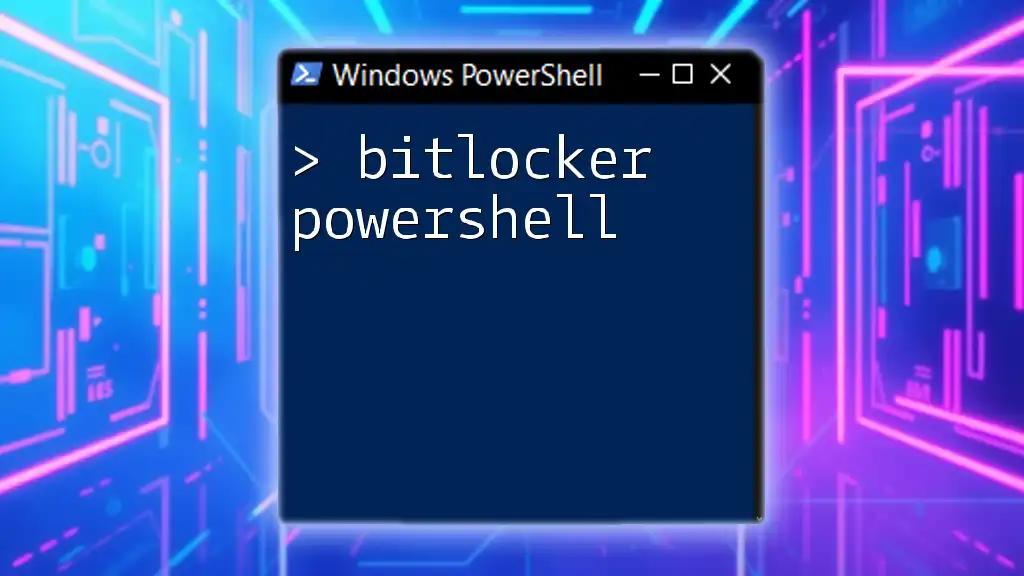
Best Practices for Using PowerShell to Unblock Files
Safety Considerations
Before unblocking any file, always verify its source. Files from untrusted or unknown origins can harbor malware or undesirable software. Being cautious even when unblocking files with PowerShell will help minimize risks.
Efficient PowerShell Scripting
To save time on recurring tasks, consider automating your file unblocking processes by creating scripts. Below is a simple script template that unblocks files in a given directory:
$path = "C:\Path\To\Your\Folder"
Get-ChildItem $path -Recurse | Unblock-File
Using such scripts helps automate your workflow, enhancing productivity and streamlining operations.
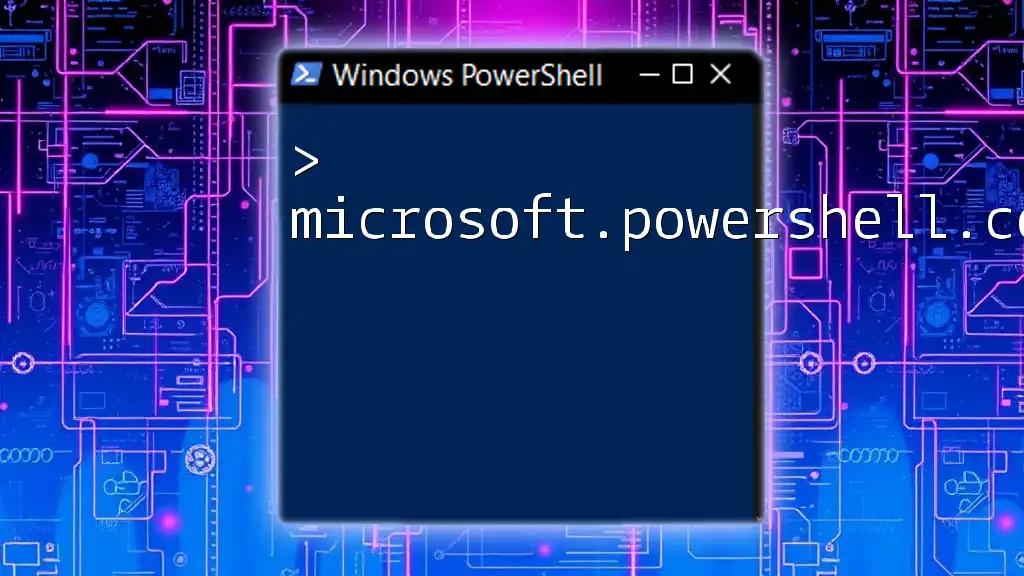
Conclusion
Recap of Key Takeaways
In summary, understanding how to effectively unblock file PowerShell commands can significantly improve your workflow while maintaining security. Leveraging PowerShell’s capabilities not only saves time but also enhances your ability to manage files efficiently.
Encouragement to Practice
Feel free to experiment with these commands to enhance your PowerShell skills and take full advantage of its automation features.
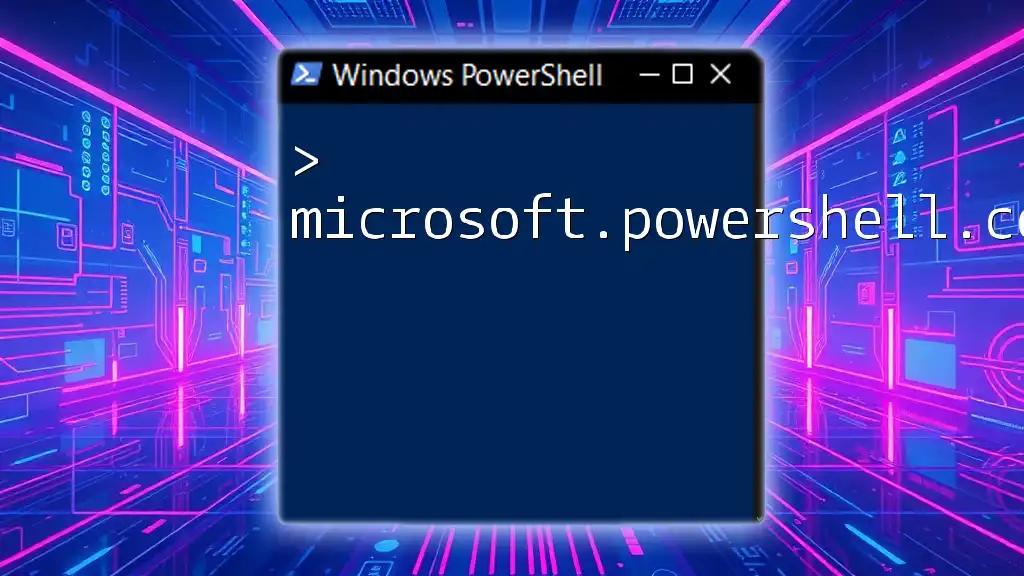
Additional Resources
Learning PowerShell
To deepen your understanding of PowerShell, consider enrolling in online courses or tutorials available on various educational platforms.
Community Support
Joining forums and online communities provides great resources for troubleshooting and learning from others. Engage with fellow users to share experiences and solutions regarding PowerShell commands and file management.