The "Clear-File" action in PowerShell is accomplished by using the `Clear-Content` cmdlet, which effectively empties the contents of a specified file without deleting the file itself.
Clear-Content -Path 'C:\path\to\your\file.txt'
Understanding the Basics of PowerShell
PowerShell is a powerful scripting language and command-line shell that enables you to automate system management tasks. Understanding file management in PowerShell is crucial for efficient operations, as it encompasses various tasks such as creating, reading, modifying, and deleting files. Being adept at handling files allows system administrators and users to maintain systems effectively and enhance productivity.
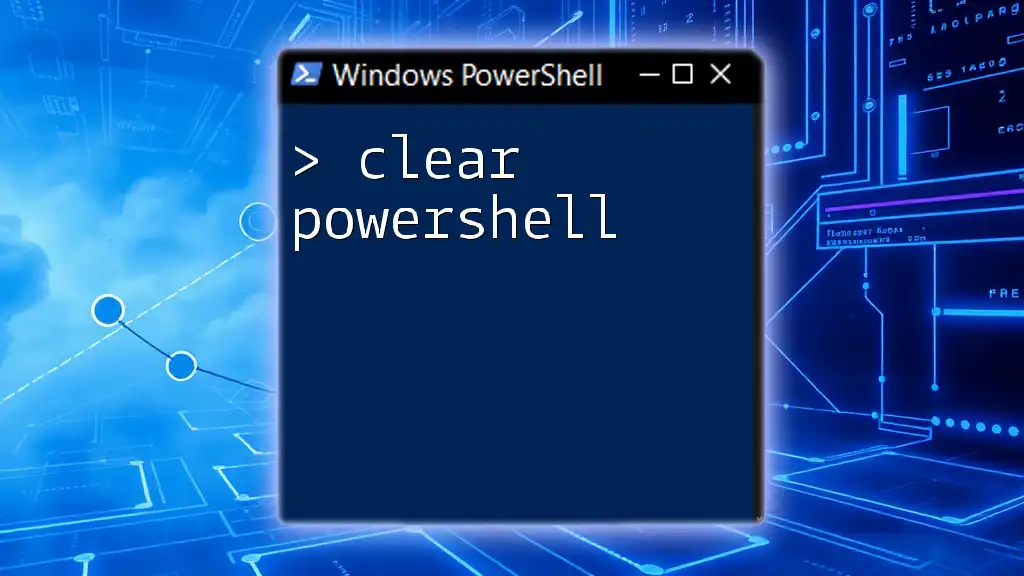
Clearing File Contents in PowerShell
When it comes to file management, it's essential to differentiate between clearing a file's contents and deleting the file entirely. Clearing a file removes its data but retains the file for future use. This capability is particularly useful for systems that need to maintain existing file structures while refreshing their contents.
Using the `Clear-Content` Command
One of the simplest ways to clear the contents of a file in PowerShell is by using the `Clear-Content` cmdlet. This command is designed to remove data from a specified file without affecting its properties or file structure.
Syntax:
Clear-Content -Path "C:\path\to\your\file.txt"
Example: To clear the content of a specific log file, use the following command:
Clear-Content -Path "C:\Logs\SystemLog.txt"
This command will successfully empty the `SystemLog.txt` file while keeping the file itself intact.
Using Redirection Operators
Another effective way to clear a file's contents is through the use of redirection operators. These operators can be leveraged to manipulate the output and thereby adjust file contents.
Clearing a File with Output Redirection
You can use the output redirection operator `>` to clear a file efficiently. Essentially, you’re instructing PowerShell to write nothing into the file, thus clearing it.
Syntax:
"" > "C:\path\to\your\file.txt"
Example: To clear a text file, you can execute:
"" > "C:\Logs\ImportantNotes.txt"
This command replaces the contents of `ImportantNotes.txt` with nothing, effectively clearing it.
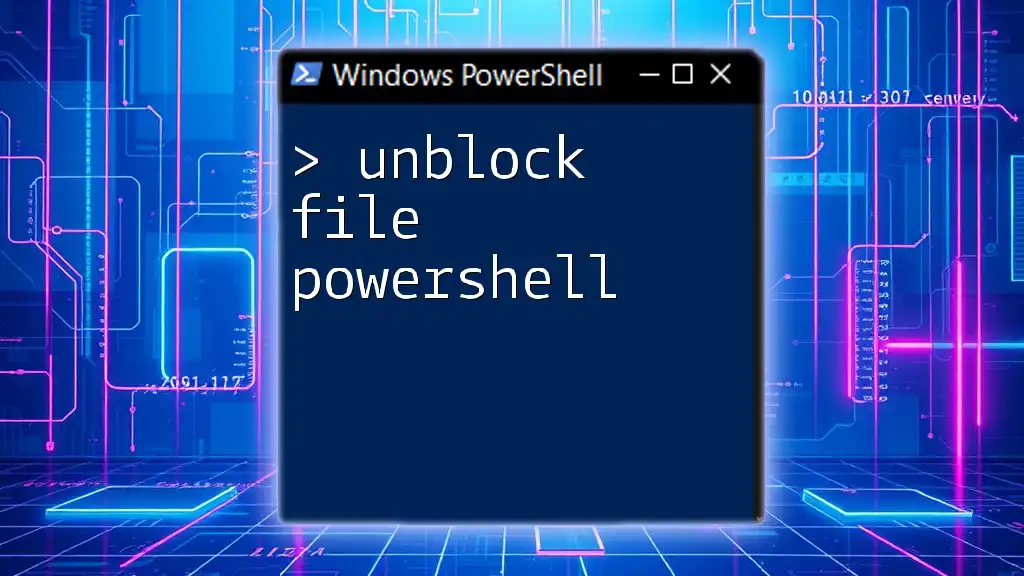
Practical Applications of Clearing File Contents
There are various scenarios in which clearing file contents is beneficial, particularly with log files and temporary data.
Managing Log Files
Log files often grow substantially over time, making them cumbersome if left unchecked. Periodically clearing these files helps manage disk space and maintains operational efficiency.
For instance, to clear a specific error log, you can simply run:
Clear-Content -Path "C:\Logs\ErrorLog.txt"
This action ensures your error log remains clear and manageable without deleting the file itself.
Handling Temporary Files
Temporary files can accumulate quickly and take up valuable storage space. One way to free this space is by regularly clearing these files.
For example, if you wish to clear the contents of all files in a temporary directory, you could use:
Get-ChildItem -Path "C:\Temp\*" | ForEach-Object { Clear-Content -Path $_.FullName }
This script efficiently clears all files located in the `C:\Temp\` directory.
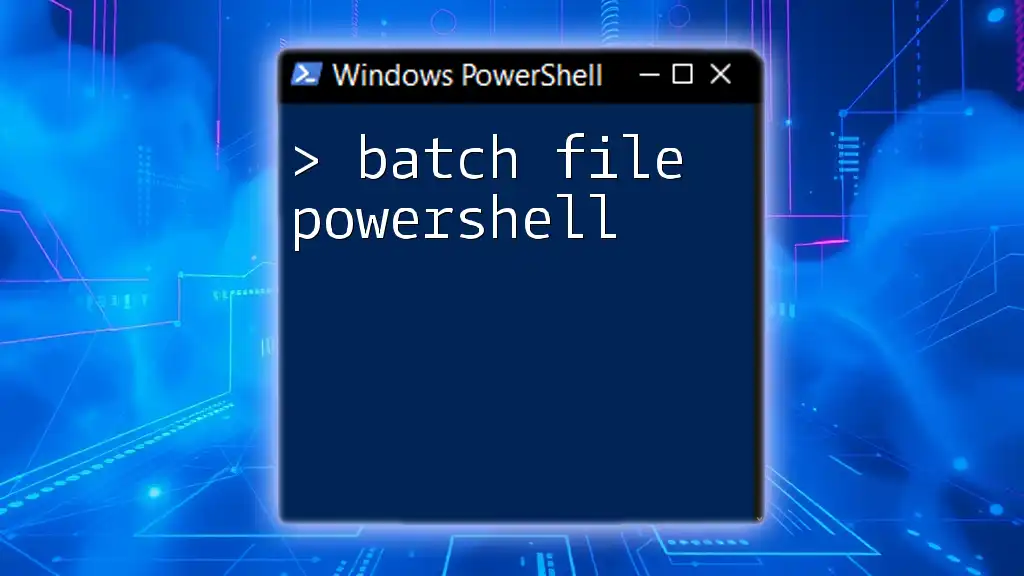
Additional Tips and Best Practices
When clearing file contents, it's essential to do so carefully to avoid unintentional data loss. Here are a few best practices:
Using the `-Force` Parameter
In some instances, you may encounter files that require administrative privileges or possess certain protections. By using the `-Force` parameter, you can bypass such issues effectively.
While `-Force` is particularly resonant with deleting files, it can also help if you're facing restrictions when trying to clear files. Always ensure you understand the implications of using this parameter, especially as it can lead to loss of data when used indiscriminately.
Automating File Clearing with Scripts
PowerShell enables the automation of repetitive tasks, including file clearing. By creating simple scripts, you can streamline this process.
For example, consider creating a PowerShell script to clear multiple clogging log files:
$files = "C:\Logs\*.log"
Get-ChildItem -Path $files | ForEach-Object { Clear-Content -Path $_.FullName }
This script clears all `.log` files in the designated folder efficiently.
Scheduling Tasks to Clear Files Automatically
For regular maintenance, you might want to automate file clearing through the built-in Task Scheduler in Windows. This enables you to run your clearing scripts at preset intervals, ensuring your files remain in check.
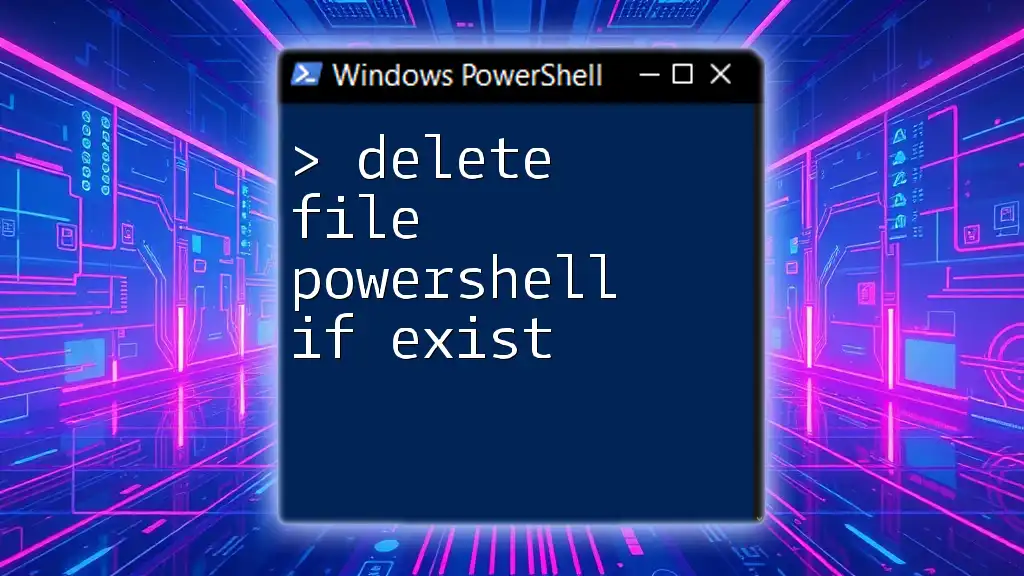
Troubleshooting Common Issues
Despite the ease of using PowerShell, users may encounter several common issues when attempting to clear files.
Permission Issues
Common errors can arise from insufficient permissions to modify files. Make sure your PowerShell session is running with the required privileges. You may need to run PowerShell as an administrator to successfully execute the clearing commands.
Read-Only Files
When you attempt to clear the contents of a read-only file, you’ll likely receive an error. To circumvent this issue, you’ll need to first change the file attributes before clearing, using:
Set-ItemProperty -Path "C:\path\to\your\file.txt" -Name IsReadOnly -Value $false
Once the read-only property is cleared, you can then safely proceed to clear its contents.
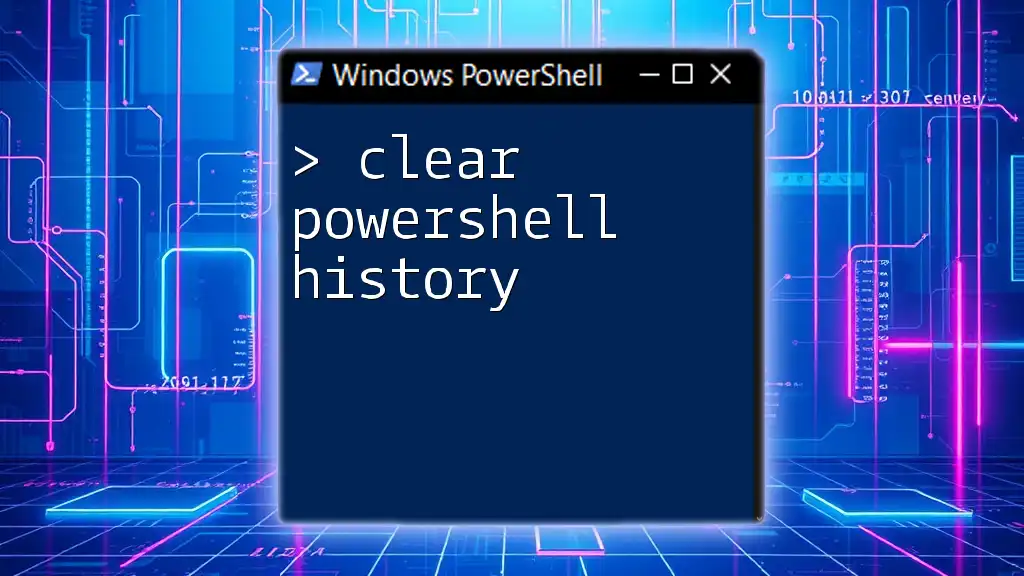
Conclusion
Clearing file contents in PowerShell is a straightforward yet powerful capability that enhances file management. Whether through commands like `Clear-Content`, redirection operators, or automation scripts, mastering these techniques will significantly improve your workflow and system performance. Practice these commands and consider exploring additional PowerShell functionalities for further enhancement in your system administration tasks.