To delete a file in PowerShell only if it exists, you can use the following command:
if (Test-Path 'C:\path\to\your\file.txt') { Remove-Item 'C:\path\to\your\file.txt' }
Understanding File Management in PowerShell
What is PowerShell?
PowerShell is a powerful command-line interface and scripting language designed for system administration and automation. It provides a robust environment for managing system configurations, automating tasks, and interacting with various system components. With its ability to handle complex tasks easily, PowerShell is a go-to tool for IT professionals and system administrators.
Why Use PowerShell for File Deletion?
Using PowerShell for file deletion offers numerous advantages:
- Flexibility and Control: Unlike traditional file management methods, PowerShell commands can be combined and scripted, allowing for tailored file management solutions.
- Automation Capabilities: By automating file management tasks, you save time and reduce repetitive manual efforts, increasing productivity.
- Simplification of Repetitive Tasks: Scripts can perform complex tasks in a single command line, enabling streamlined workflows.
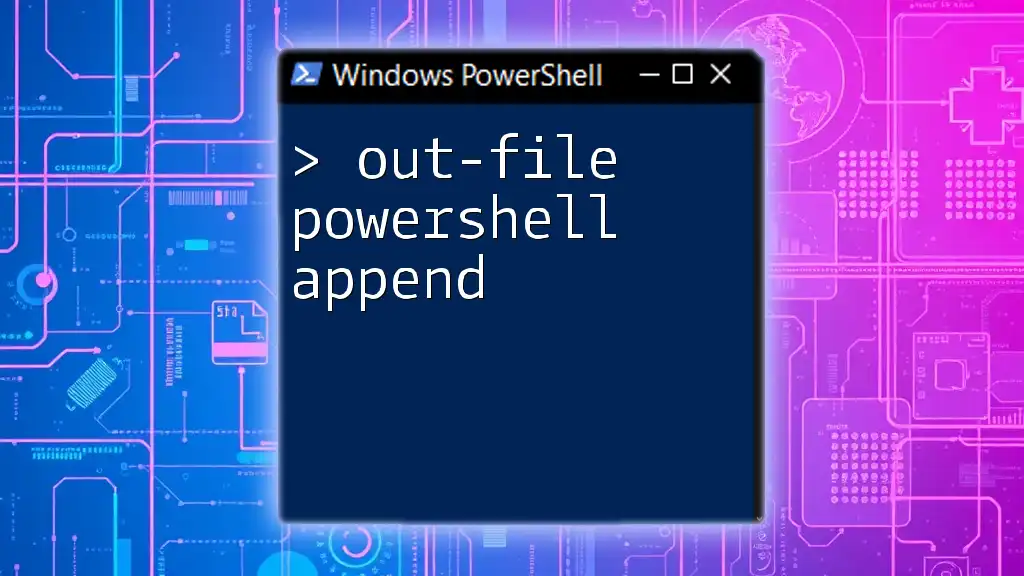
Checking If a File Exists
Using the `Test-Path` Cmdlet
Before attempting to delete a file, the first step is to check if it exists. The `Test-Path` cmdlet is the perfect tool for this purpose. It verifies the presence of a file or directory and returns a Boolean value.
Code Snippet:
$filePath = "C:\path\to\your\file.txt"
if (Test-Path $filePath) {
Write-Host "File exists."
} else {
Write-Host "File does not exist."
}
In this snippet, the `$filePath` variable holds the path to the file you want to check. If the file exists, a message will confirm it; otherwise, you receive a notification stating that the file is not found.
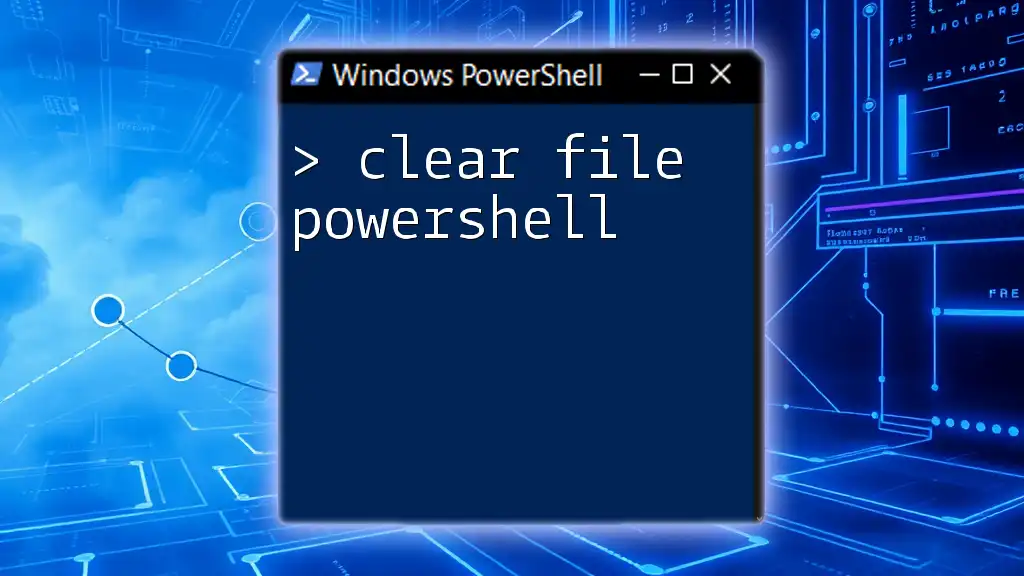
Deleting a File in PowerShell
Using the `Remove-Item` Cmdlet
Once you've confirmed that the file exists, you can proceed to delete it using the `Remove-Item` cmdlet. This cmdlet removes items, which can include files, directories, or even registry keys.
Code Snippet:
Remove-Item -Path $filePath -Force
In this example, the `-Path` parameter specifies the file location, while the `-Force` parameter allows deletion without additional prompts, ensuring a swift operation.
Combining Checks and Deletion
To create a more efficient script, you can combine the existence check with the deletion command. This way, you ensure that you only attempt to delete the file if it exists.
Code Snippet:
if (Test-Path $filePath) {
Remove-Item -Path $filePath -Force
Write-Host "File deleted."
} else {
Write-Host "No file to delete."
}
This complete script first checks if the file exists; if it does, it deletes it and notifies you of the action taken. If not, it simply states that there’s nothing to delete.
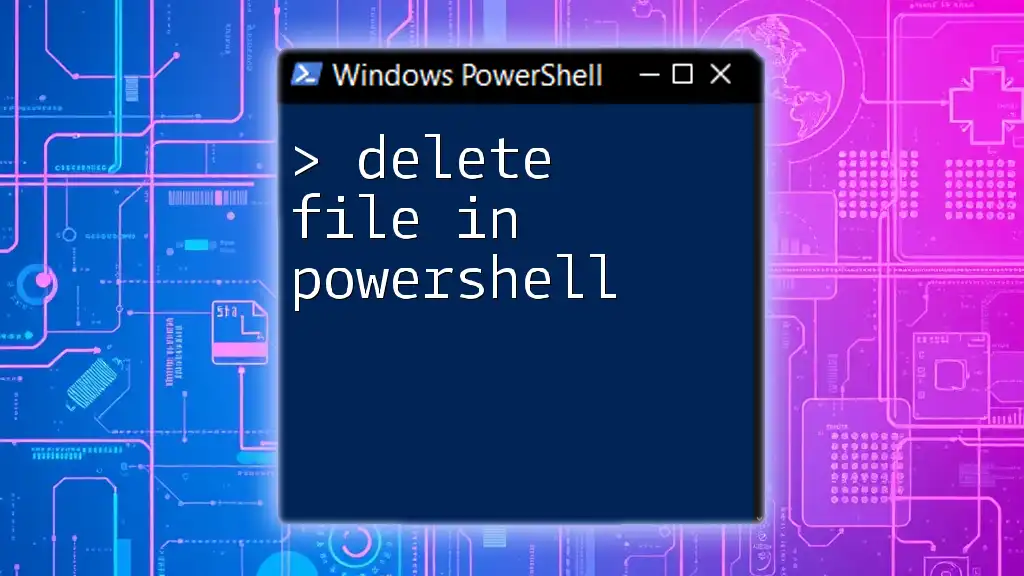
Adding Safety Measures
Using the `-Confirm` Parameter
When performing critical operations like file deletion, using the `-Confirm` parameter can be a valuable safety measure. This parameter prompts for confirmation before executing the deletion, allowing you to prevent accidental data loss.
Code Snippet:
if (Test-Path $filePath) {
Remove-Item -Path $filePath -Confirm
}
With this command, PowerShell will ask for your confirmation before deleting the file, providing an additional layer of protection.
Logging File Deletions
Keeping a log of the files you delete can be useful for traceability. By recording deletion actions, you can review what changes were made and potentially recover lost files if needed.
Code Snippet:
$logPath = "C:\path\to\log.txt"
if (Test-Path $filePath) {
Remove-Item -Path $filePath -Force
"Deleted: $filePath - $(Get-Date)" | Out-File -FilePath $logPath -Append
}
In this snippet, the script logs each deletion, including the file path and the date, to a specified log file. This can be essential for auditing and recovery purposes.
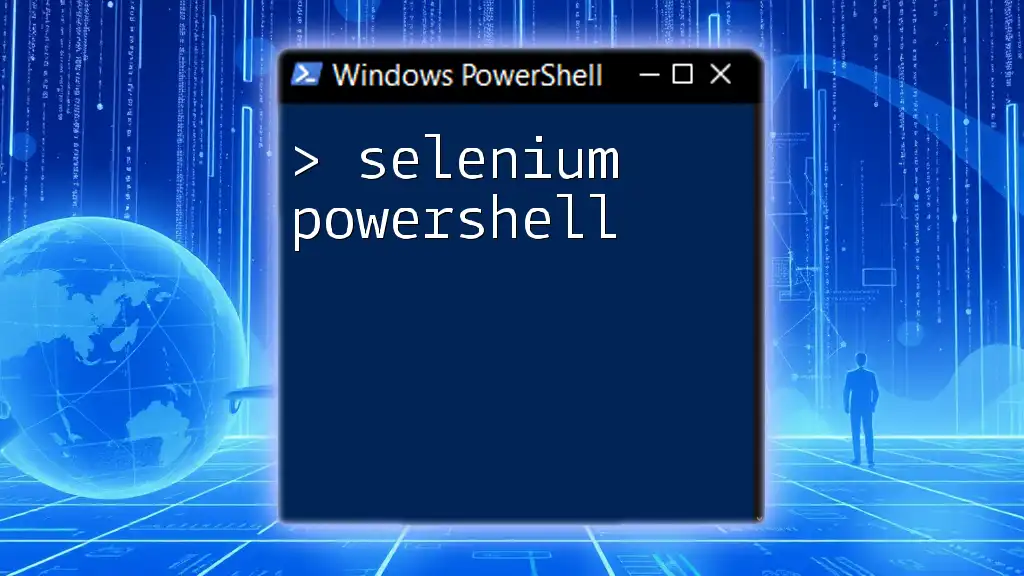
Advanced Usage
Deleting Multiple Files
PowerShell can efficiently handle operations on multiple files using wildcards or filters. This can be particularly beneficial when cleaning up directories.
Code Snippet:
$folderPath = "C:\path\to\directory\"
Get-ChildItem -Path $folderPath -Filter "*.log" | Remove-Item -Force
Here, `Get-ChildItem` retrieves all `.log` files in the specified directory, and the `Remove-Item` cmdlet deletes them. This command showcases how PowerShell can simplify batch operations.
Using Try/Catch for Error Handling
In professional settings, robust error handling is essential. Utilizing `try/catch` blocks in PowerShell allows you to manage errors gracefully and maintain a stable user experience.
Code Snippet:
try {
if (Test-Path $filePath) {
Remove-Item -Path $filePath -Force
}
} catch {
Write-Host "Error: $_"
}
This script attempts to delete the file, and if any issues arise during the process, it catches the error and displays a message. This can help you troubleshoot issues effectively and ensure your scripts run smoothly.
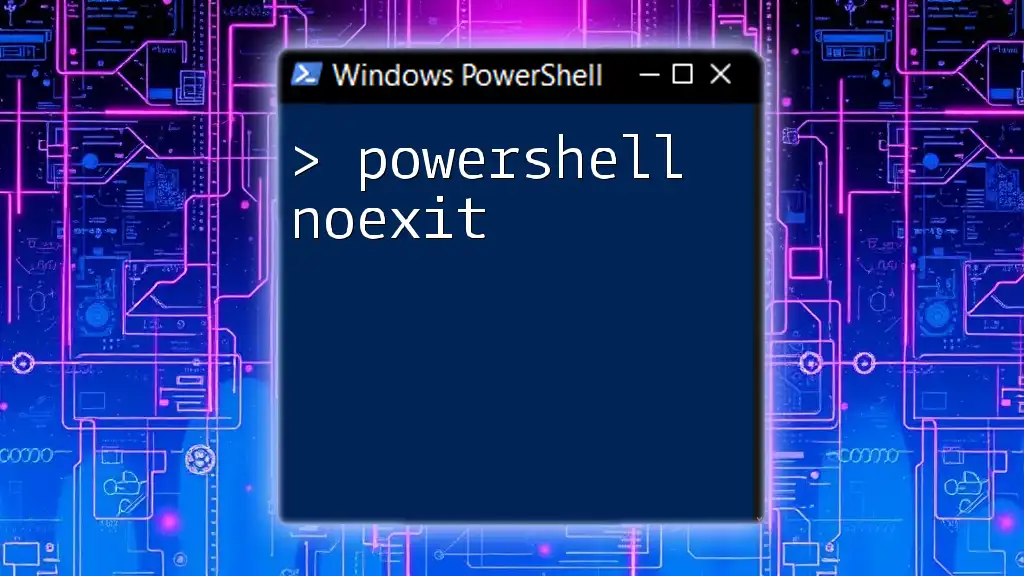
Conclusion
Understanding how to delete a file in PowerShell if it exists is a fundamental skill for anyone working with system administration. Mastering these commands can greatly enhance productivity and streamline workflow. As you practice implementing these commands, you’ll become more adept at file management through automation. This opens up endless possibilities for optimizing your tasks and improving efficiency. Remember, always test in a safe environment and consider best practices in automation—happy scripting!
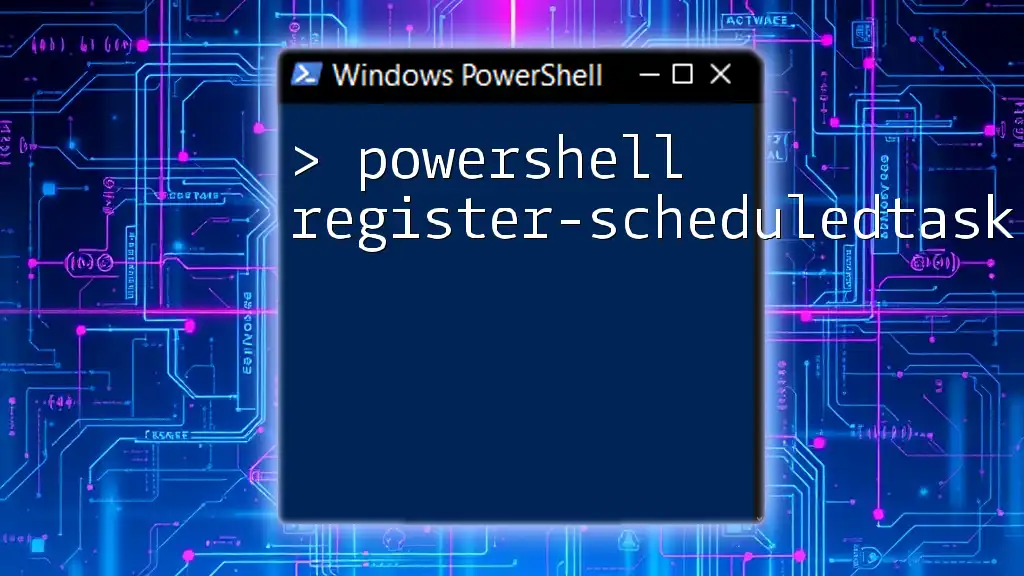
Additional Resources
For further exploration of PowerShell capabilities, consider checking official PowerShell documentation and relevant community forums. These resources can provide additional insights and connect you with other PowerShell enthusiasts eager to share their knowledge.