To delete a file in PowerShell, you can use the `Remove-Item` cmdlet followed by the file path.
Remove-Item 'C:\path\to\your\file.txt'
What is PowerShell?
PowerShell is a powerful scripting language and command-line shell developed by Microsoft, primarily designed for system administration and automation. It provides users with the ability to perform complex tasks in an efficient manner, leveraging cmdlets that streamline the management of systems, applications, and files.
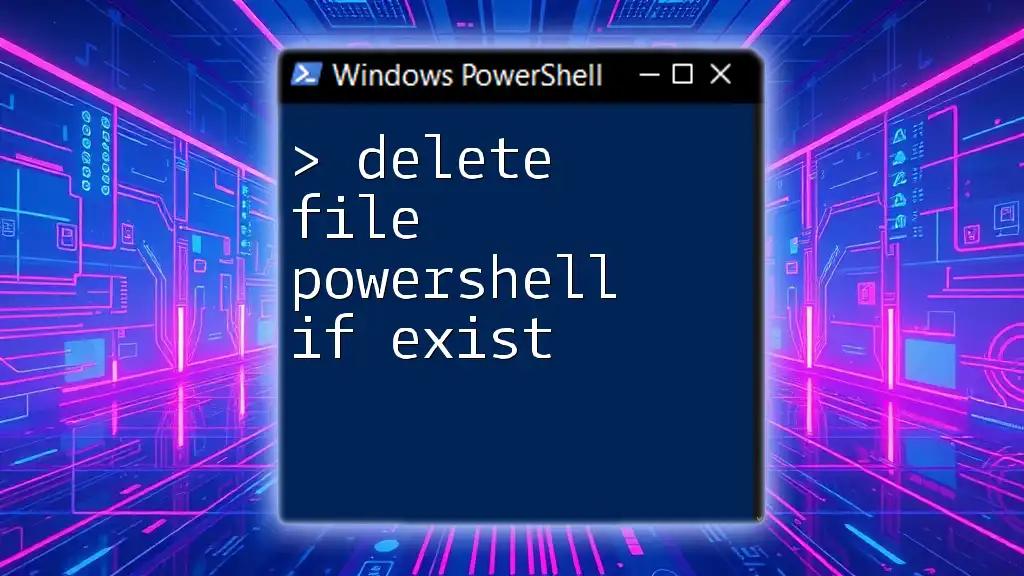
Why Use PowerShell for File Management?
Using PowerShell for file management offers several advantages over traditional methods:
- Efficiency: PowerShell enables batch processing of files, allowing users to execute multiple commands at once, saving time and effort.
- Automation: Automate repetitive tasks with scripts, reducing the potential for human error and increasing productivity.
- Robustness: Provides advanced options for error handling, filtering, and recursive operations.
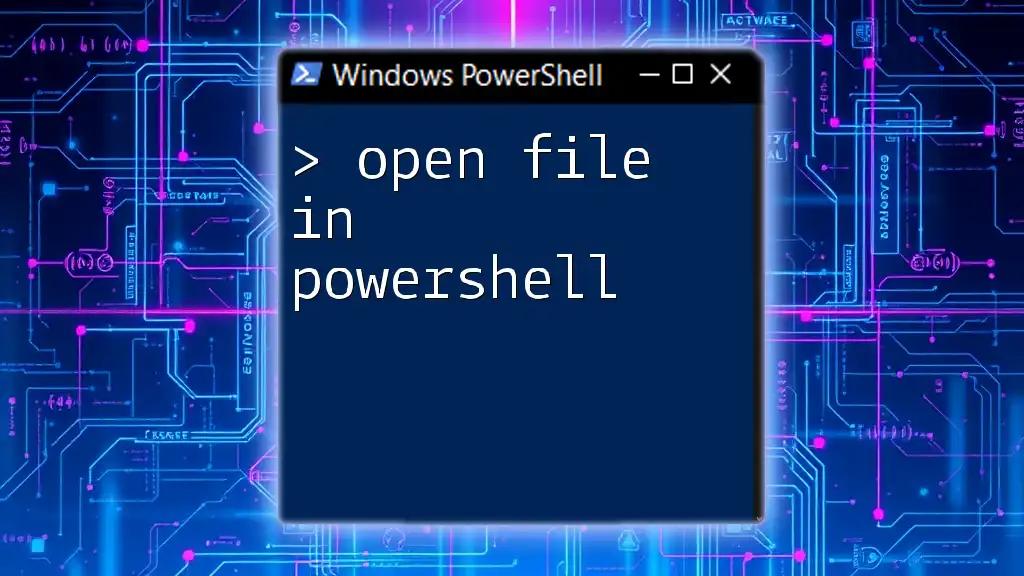
How to Delete a File in PowerShell
Basic Deletion Command
Deleting a file in PowerShell is straightforward using the `Remove-Item` cmdlet. The basic syntax is as follows:
Remove-Item -Path "C:\example\file.txt"
This command specifies the location of the file you want to delete. It is crucial to ensure the path is correct to avoid accidental deletion of important files.
Confirming File Deletion
To add a layer of safety, you can use the `-Confirm` switch. This prompts you for confirmation before the file is deleted, helping prevent unintentional removal:
Remove-Item -Path "C:\example\file.txt" -Confirm
By doing so, you help mitigate the risk of deleting the wrong file.
Deleting Multiple Files
Using Wildcards
PowerShell supports the use of wildcards, making it easy to delete multiple files at once. For instance, to delete all text files in a directory, you can use:
Remove-Item -Path "C:\example\*.txt"
Here, the `*` wildcard matches any file with a `.txt` extension in the specified folder.
Specifying Multiple File Paths
You can also specify multiple files explicitly. This method is useful when you want to delete various individual files:
Remove-Item -Path "C:\example\file1.txt", "C:\example\file2.txt"
This command ensures that only the specified files are removed.
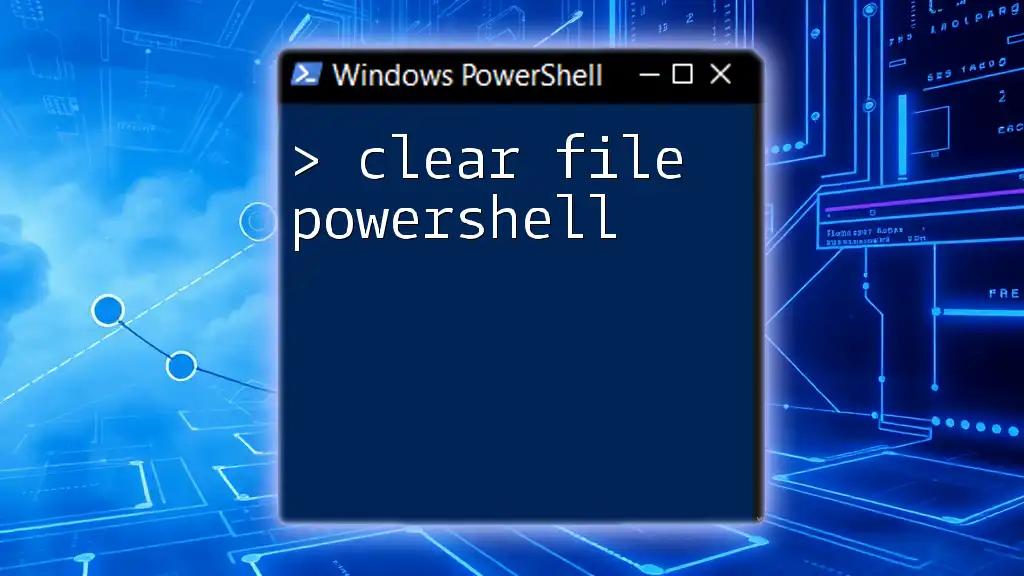
Advanced File Deletion Techniques
Deleting Files Recursively
For bulk deletion within a folder, the `-Recurse` switch comes in handy. It allows you to delete all files and subfolders within a specified path:
Remove-Item -Path "C:\example\*" -Recurse
Be cautious when using this command, as it removes everything in the directory without further prompts.
Deleting Read-Only Files
Some files may be set to read-only, which requires additional handling. Using the `-Force` switch forces the deletion of read-only files without additional warning:
Remove-Item -Path "C:\example\readonly.txt" -Force
This command should be used judiciously to avoid unintentionally deleting important data.
Using the `Where-Object` Cmdlet for Conditional Deletion
You can filter files based on specific conditions before deleting them. This adds a layer of control to your file management tasks. For example, to delete files larger than 1MB in a directory, you can use:
Get-ChildItem "C:\example\" | Where-Object { $_.Length -gt 1MB } | Remove-Item
This command retrieves all items in the specified path, filters them by size, and then deletes those that meet the condition.
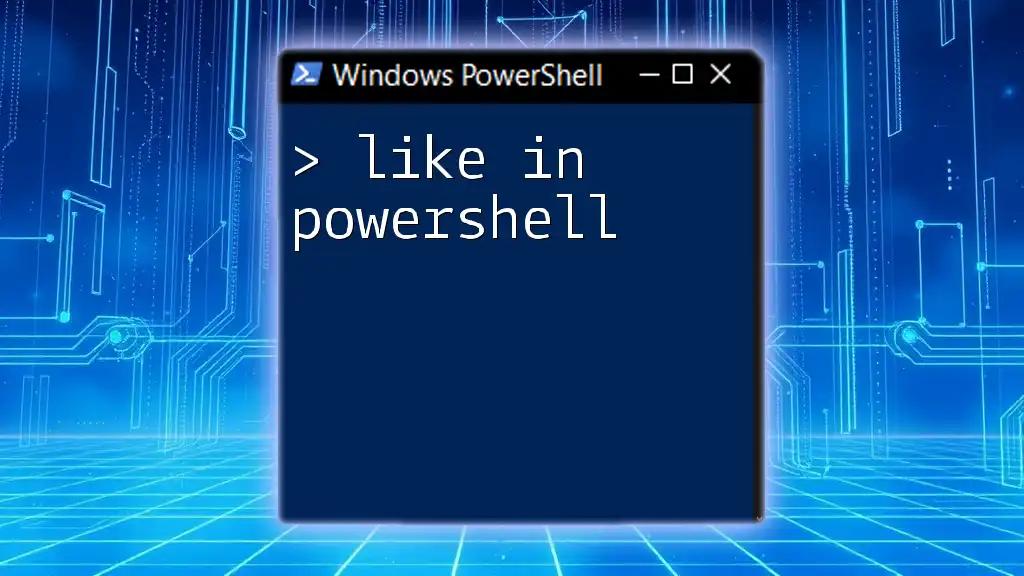
Error Handling in PowerShell Commands
Common Errors and Their Solutions
Errors may occur during file deletion. Two common issues are:
- File Not Found Error: If you attempt to delete a file that does not exist, you will receive an error message. Always verify the file's existence before running the delete command.
- Access Denied Error: If you do not have sufficient permissions to delete the file, you will encounter this error. Running PowerShell with elevated privileges may resolve this issue.
Using Try/Catch for Robust Scripts
To enhance your script's robustness, implement error handling using the `try/catch` construct. This could look like:
try {
Remove-Item -Path "C:\example\file.txt" -ErrorAction Stop
} catch {
Write-Host "An error occurred: $_"
}
This approach provides feedback if something goes wrong during the deletion process.
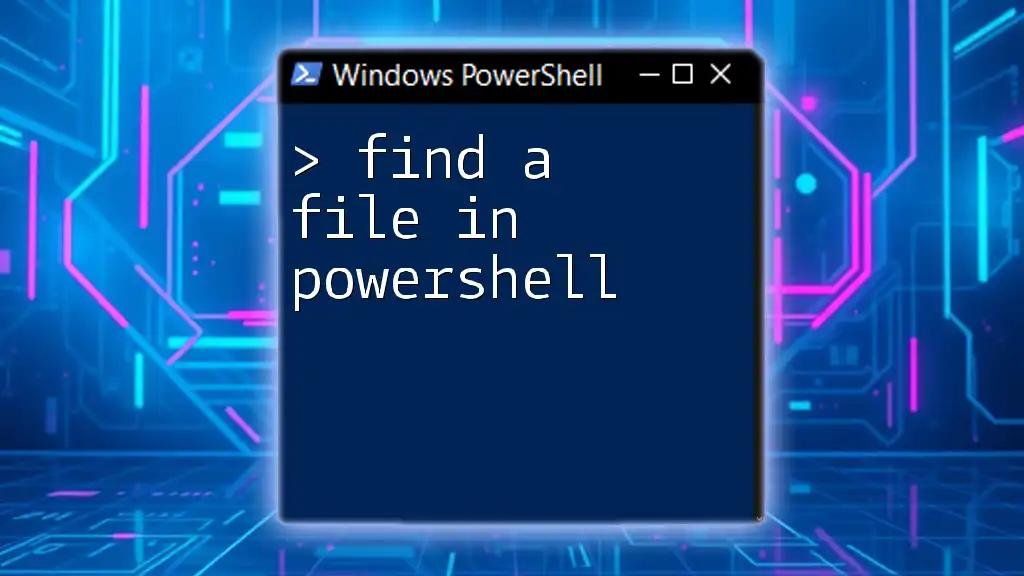
Tips for Safe File Deletion in PowerShell
Best Practices for Removing Files
When deleting files, adhere to these best practices:
- Verify File Paths: Always double-check the file paths provided in your commands to avoid accidental data loss.
- Importance of Backups: Regularly back up critical data before running deletion commands.
Using `-WhatIf` for Simulation
To preview what would happen if you execute a deletion command without actually deleting files, use the `-WhatIf` switch:
Remove-Item -Path "C:\example\file.txt" -WhatIf
This command outputs what would occur if the command were run, allowing you to verify the action without making changes.
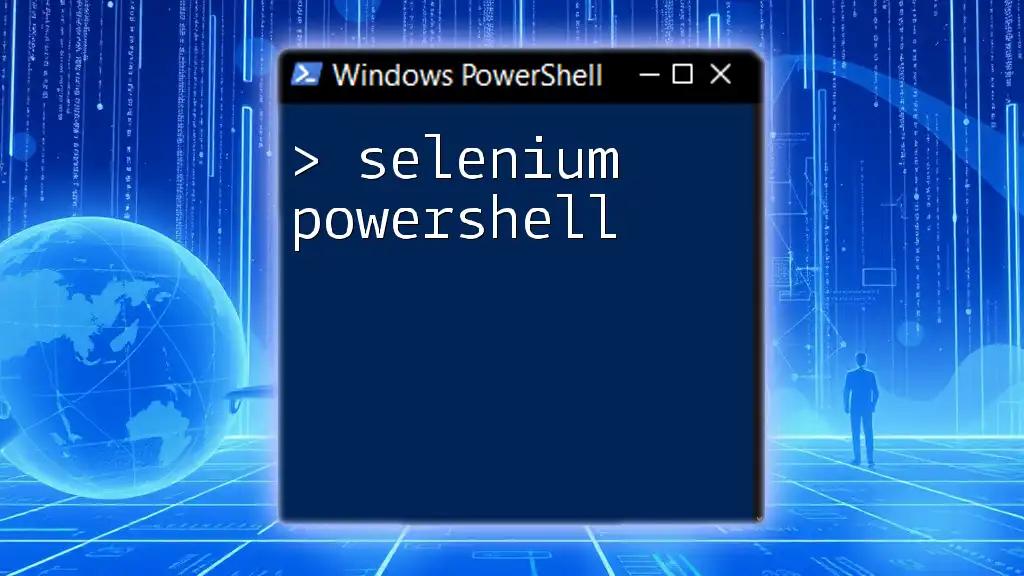
Conclusion
In conclusion, deleting a file in PowerShell is a straightforward process that can be tailored to meet various needs—whether you're deleting single files, multiple files, or even large directories. Understanding how to safely and efficiently manage file deletions can significantly enhance your productivity and reduce the risk of errors.
Call to Action
Practice these commands in a safe environment to build your skill set. For more tips and tutorials on PowerShell commands, consider subscribing to our updates for the latest insights and resources.
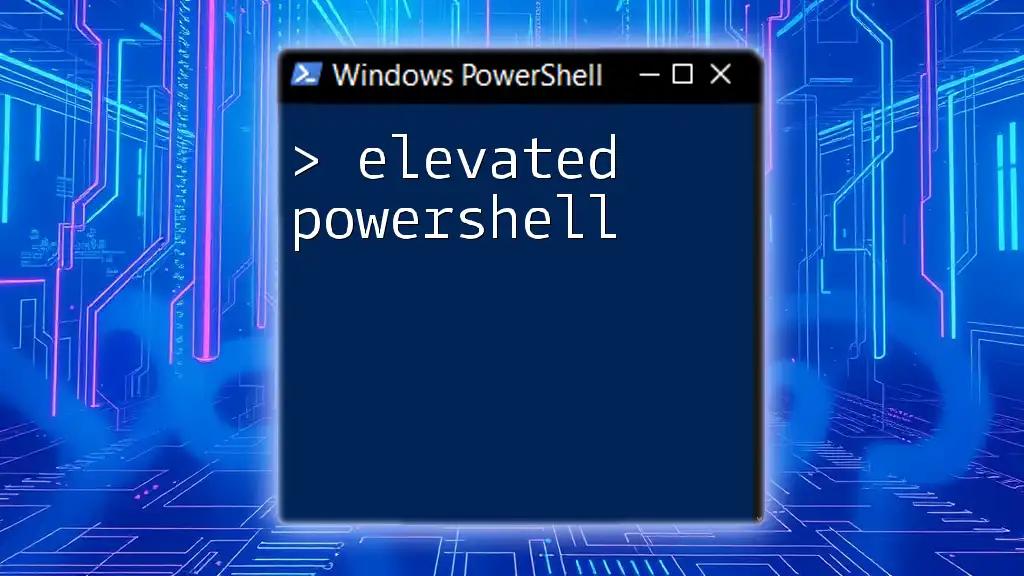
Additional Resources
For further reading, consider exploring the official Microsoft documentation to gain deeper insights into PowerShell and its capabilities. You may also find tools and extensions beneficial for enhancing your PowerShell experience.
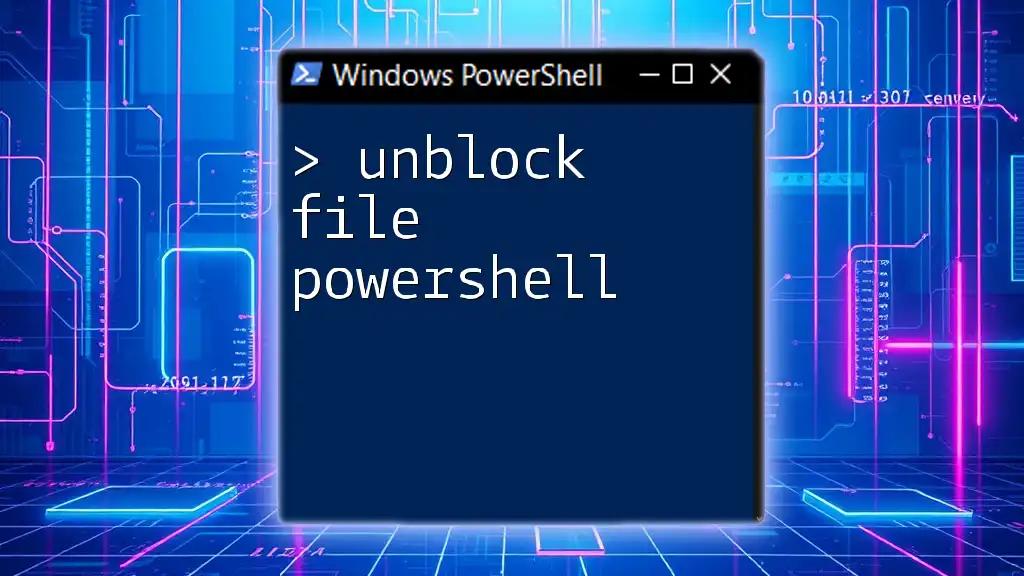
Frequently Asked Questions
Can I recover deleted files using PowerShell?
Recovering deleted files can be challenging, especially if they have been permanently removed. However, third-party tools or restore options may help recover lost data. Always ensure you have a robust backup plan.
Is PowerShell safe for file management?
PowerShell is generally safe when used correctly, but users must remain vigilant. Always double-check commands and utilize features like `-Confirm` and `-WhatIf` to ensure safe operations.