In PowerShell, the `try` and `catch` blocks are used for error handling, allowing you to execute a block of code, and if an error occurs, to catch and handle it gracefully.
try {
# Attempt to divide by zero to trigger an error
$result = 10 / 0
} catch {
Write-Host "An error occurred: $_"
}
Understanding Error Handling in PowerShell
What is Error Handling?
Error handling is a critical aspect of any programming language, including PowerShell. It refers to the process of responding to and managing errors that occur during the execution of a script or application. Handling errors effectively ensures that your scripts do not crash unexpectedly and can provide informative feedback to users.
Types of Errors in PowerShell
Errors in PowerShell are primarily categorized into two types: non-terminating errors and terminating errors.
-
Non-terminating errors are errors that do not stop the execution of a script. Instead, PowerShell continues running the script, often logging the error. For example, invoking a cmdlet that does not find any items may result in a non-terminating error.
-
Terminating errors occur when a command fails completely, causing the script to terminate immediately. Such errors are severe and indicate that a significant failure has taken place, such as failing to connect to a file system or a network resource.
Understanding these types of errors is essential for implementing effective error handling strategies.
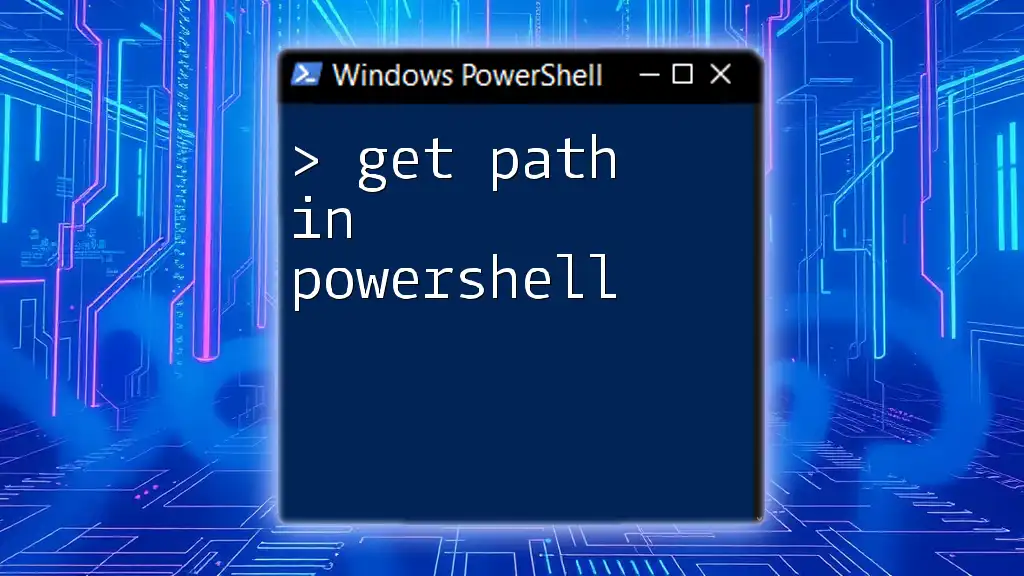
Introduction to Try and Catch in PowerShell
What is Try and Catch?
The `try` and `catch` blocks in PowerShell are constructs that allow developers to handle exceptions gracefully. When you place code that might produce an error within a `try` block, you can use the corresponding `catch` block to determine how to respond when that error occurs.
Basic Structure of Try and Catch
The syntax of `try` and `catch` is straightforward:
try {
# Code that may cause an error
}
catch {
# Code that handles the error
}
In this structure, if an error occurs in the `try` block, PowerShell will immediately jump to the `catch` block to handle the error without crashing the script.
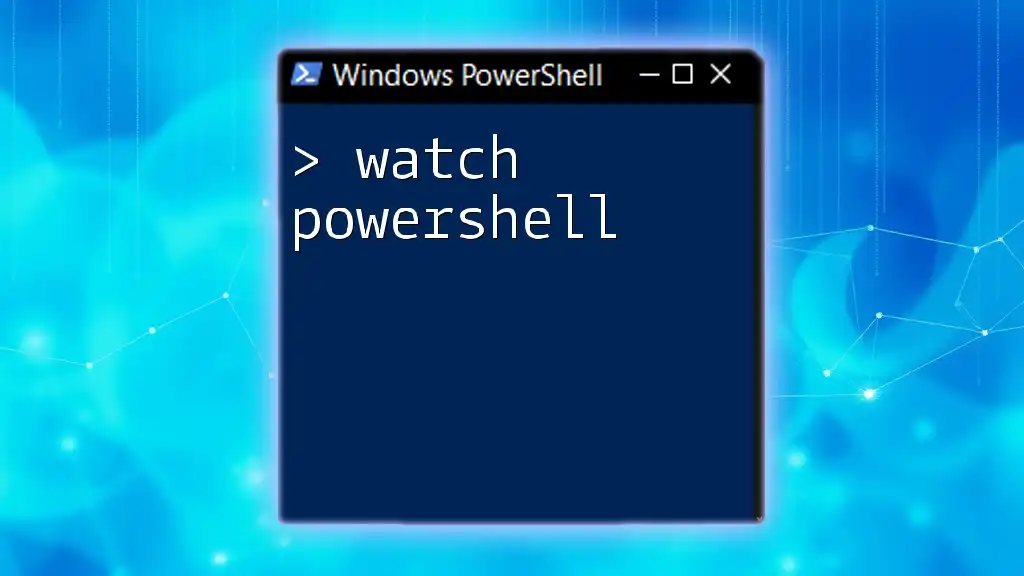
How to Use Try and Catch in PowerShell Scripts
Writing a Simple Try-Catch Block
Let’s start with a simple example. Imagine you're trying to read from a file that doesn't exist:
try {
Get-Content "nonexistentfile.txt"
}
catch {
Write-Host "An error occurred: $_"
}
In this example, when attempting to fetch content from a non-existent file, PowerShell triggers the `catch` block. The output will inform you of the error, along with its details using the `$_` variable.
Catching Specific Exceptions
It is often useful to handle specific exceptions that may occur during script execution. You can achieve this by defining multiple `catch` blocks for different exception types.
Using Catch to Handle Different Errors
try {
# Code that may fail
}
catch [System.IO.FileNotFoundException] {
Write-Host "File not found."
}
catch {
Write-Host "An unexpected error occurred: $_"
}
In this case, if the script encounters a `FileNotFoundException`, the first `catch` block will execute. For all other errors, the second `catch` block serves as a fallback.
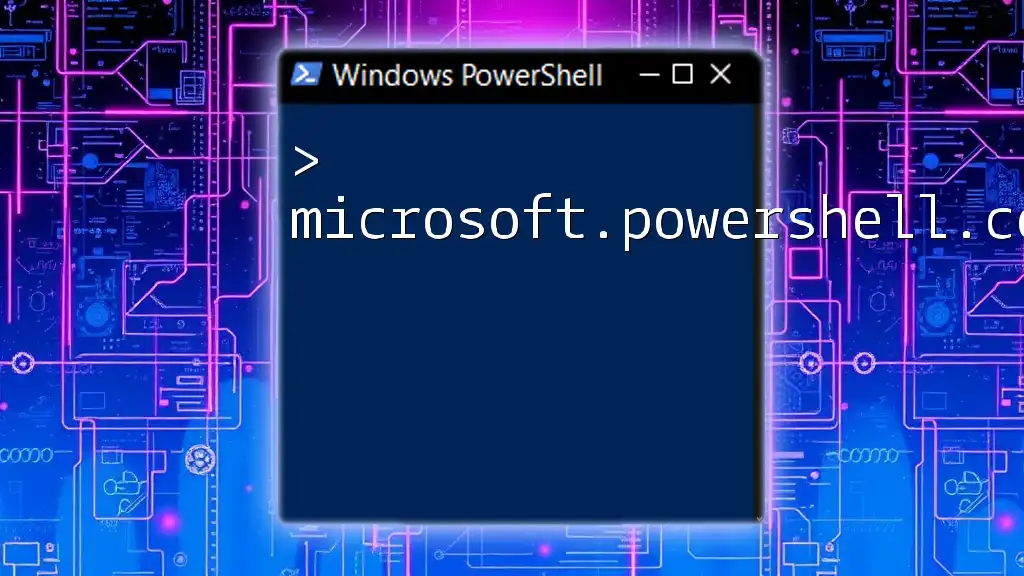
PowerShell Try Catch Error and Exception Properties
Accessing Error Information
Within the `catch` block, the variable `$_` represents the error that was caught. You can use this variable to extract detailed information regarding the error.
For example, consider this code snippet:
try {
# Code
}
catch {
Write-Host "Error Details: $($_.Exception.Message)"
}
Here, you can log the specific message associated with the exception, allowing for better debugging and understanding of what went wrong.
The Error Variable `$Error`
PowerShell maintains an array variable `$Error`, which contains information about the most recent errors that have occurred during the session. You can access this variable to get insights into prior errors, which can be useful for error logging and analysis.
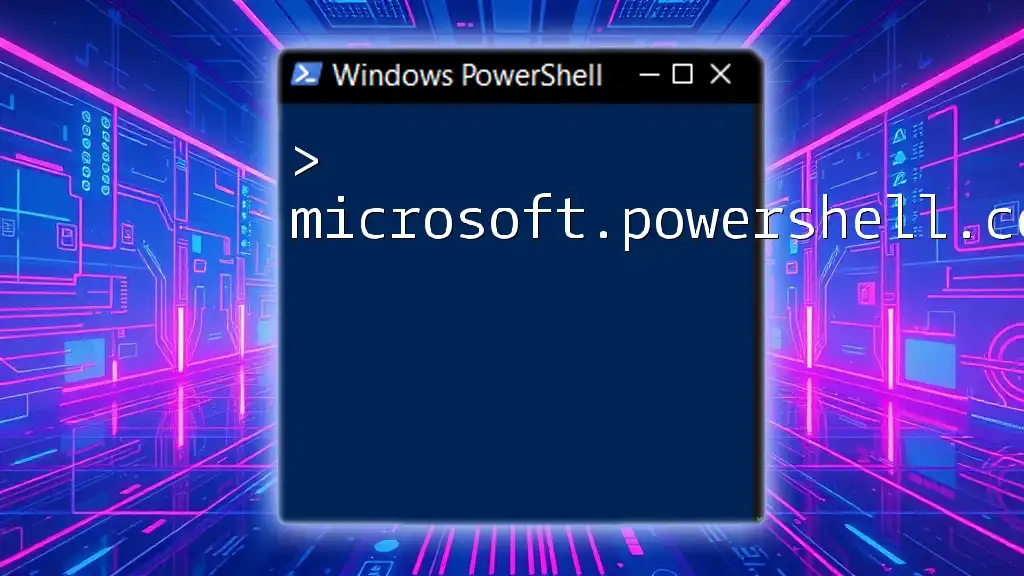
Best Practices for Using Try and Catch in PowerShell
When to Use Try and Catch
Strategically using `try` and `catch` can significantly enhance your PowerShell scripts. They are particularly beneficial in scenarios involving:
- File operations: such as reading or writing to files that may not exist.
- Database interactions: where connectivity issues may arise.
- Web requests: handling network-related errors.
Avoiding Overuse of Try and Catch
While `try-catch` is powerful, over-relying on them can lead to complex and harder-to-read code. It's essential to use them judiciously—only where errors are expected, and where they provide value.
Writing Clean Try-Catch Code
The `finally` block can be used alongside `try` and `catch`. This block executes whether an error occurred or not, making it ideal for cleanup operations.
try {
# Code that might fail
}
catch {
Write-Host "An error occurred."
}
finally {
Write-Host "This always runs, even if an error occurred."
}
This ensures that your script can gracefully clean up resources, such as closing database connections or releasing file handles.
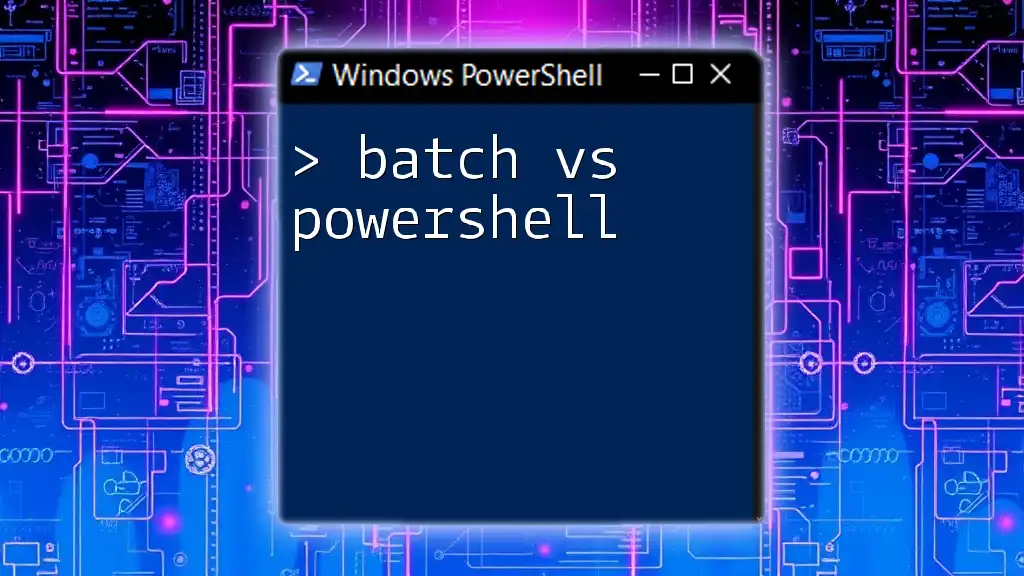
Real-world Examples of Try Catch in PowerShell
Example 1: Handling File I/O Errors
Consider a scenario where you want to read content from a file:
try {
$content = Get-Content "C:\path_to_file.txt"
Write-Host $content
}
catch {
Write-Host "Failed to read the file: $($_.Exception.Message)"
}
In this example, if the file path is incorrect or the file is inaccessible, the script will handle the error without terminating, providing feedback about the exact issue.
Example 2: Handling API Requests
When making REST API calls, you can use the `try-catch` structure to manage connectivity issues, such as server unavailability:
try {
$response = Invoke-RestMethod -Uri "https://api.example.com/resource"
Write-Host "API response: $response"
}
catch {
Write-Host "Failed to call API: $($_.Exception.Message)"
}
This allows you to catch exceptions related to network failures and handle them gracefully rather than letting your entire script fail.
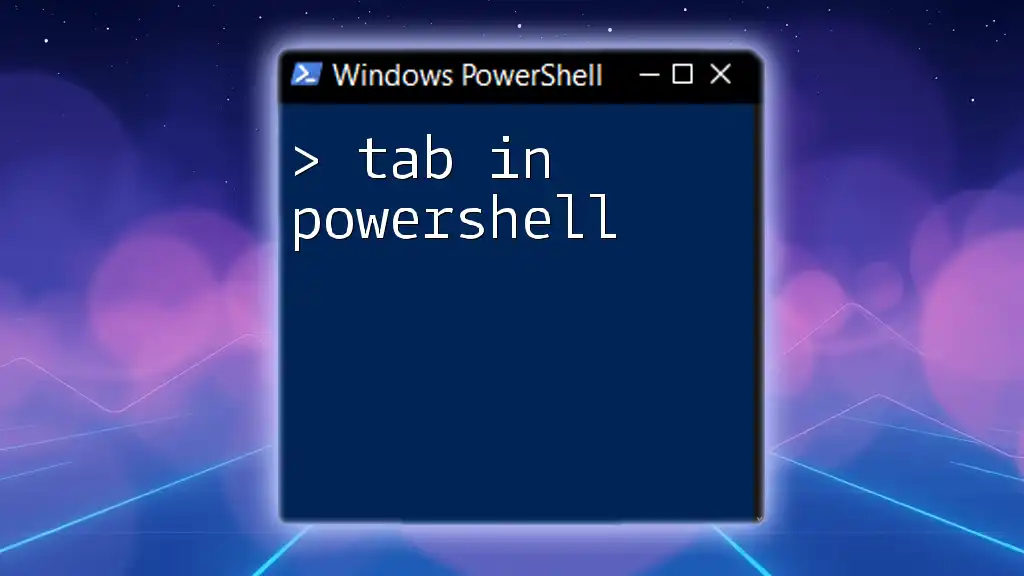
Advanced Usage of Try and Catch
Custom Error Handling with `Throw`
In certain situations, you may want to re-throw an exception once you've processed it. This can be achieved using the `throw` statement.
try {
# Code
}
catch {
Write-Host "An error occurred. Rethrowing..."
throw $_
}
This approach is useful when you want to log an error but still want the exception to bubble up for further handling.
Using `-ErrorAction` in conjunction with Try Catch
You can also utilize the `-ErrorAction` parameter in cmdlets to dictate how PowerShell should handle errors. Combining this with `try-catch` allows for robust error management.
For instance:
try {
Get-Content "nonexistentfile.txt" -ErrorAction Stop
}
catch {
Write-Host "Caught error: $($_.Exception.Message)"
}
Here, setting `-ErrorAction Stop` forces any errors to be handled in the `catch` block, allowing for clearer error management protocols in your scripts.
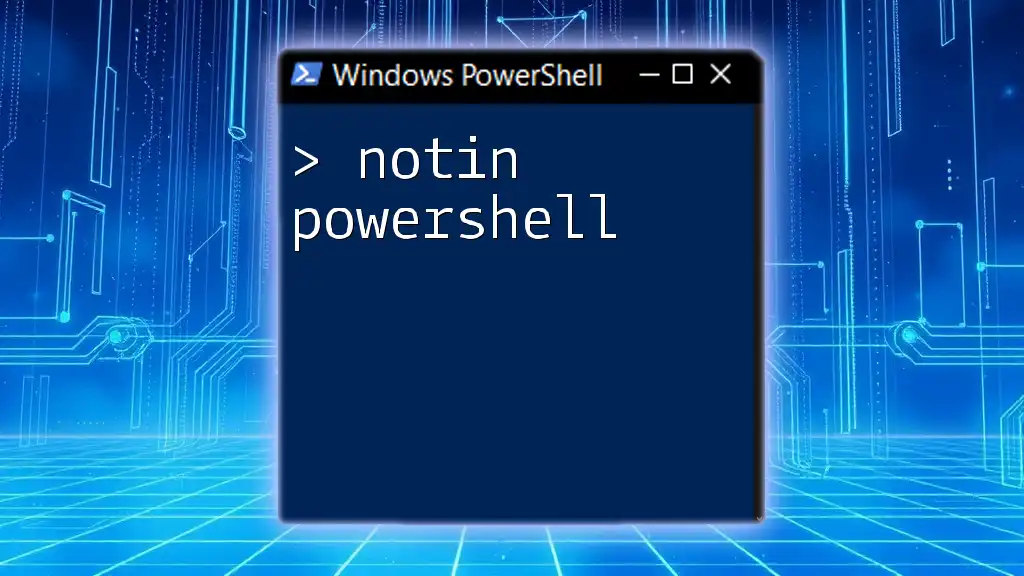
Conclusion
In conclusion, utilizing the `try` and `catch` constructs in PowerShell allows you to create scripts that are not only resilient to errors but also informative. Effective error management is essential for delivering a smooth user experience and understanding what goes wrong in your applications. Practicing various scenarios, employing best practices, and continually refining your error handling strategies will significantly enhance your PowerShell scripting skills.
Take the time to explore these features and see how they can streamline your workflow, readying your scripts for the unpredictable nature of real-world tasks.