The `FileSystemWatcher` in PowerShell allows you to monitor changes to a specified directory and respond to events such as file creation, modification, or deletion in real-time.
Here's a simple code snippet to demonstrate its usage:
# Set up the FileSystemWatcher to monitor the specified directory
$watcher = New-Object System.IO.FileSystemWatcher
$watcher.Path = "C:\Your\Directory\Path"
$watcher.Filter = "*.*"
# Define the event action for when a file is created
$action = {
Write-Host "File created: $($Event.SourceEventArgs.FullPath)"
}
# Register the event
Register-ObjectEvent $watcher "Created" -Action $action
# Start monitoring
$watcher.EnableRaisingEvents = $true
Write-Host "Monitoring directory for changes. Press [Enter] to exit..."
[Console]::ReadLine()
# Cleanup
Unregister-Event -SourceIdentifier $watcher.InstanceId
$watcher.Dispose()
Introduction to FilesystemWatcher
What is FilesystemWatcher?
The FilesystemWatcher is a powerful class in the .NET framework that enables users to monitor file system changes in real-time. It acts as an observer, detecting changes made to files and directories, including creations, modifications, deletions, and renaming of files. This capability can be extremely beneficial for various applications, such as logging changes, triggering automated processes, or monitoring critical directories.
Why Use FilesystemWatcher with PowerShell?
Incorporating FilesystemWatcher in PowerShell scripts can vastly improve workflows by automating the response to file events. This is particularly useful in environments where file changes require immediate action, such as:
- IT Operations: Monitoring system logs for alterations, deletions, or additions.
- DevOps: Automating CI/CD pipelines in response to file changes.
- Backup Solutions: Triggering automatic file backups upon creation or modification.
By utilizing FilesystemWatcher in PowerShell, users can create robust, reactive scripts that save time and reduce errors.
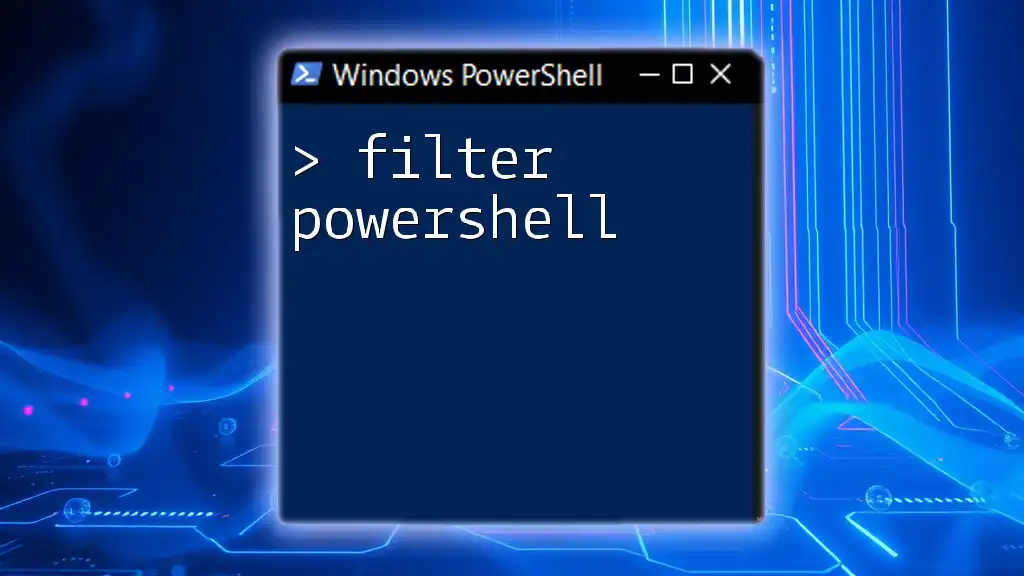
Setting Up FilesystemWatcher in PowerShell
Prerequisites
Before implementing FilesystemWatcher in your PowerShell scripts, ensure you possess a basic understanding of PowerShell scripting along with the .NET Framework, as the FilesystemWatcher class is part of this framework.
Importing Required Assemblies
To use the FilesystemWatcher, you'll need to import the necessary .NET assembly into your PowerShell session. This can be achieved using the following command:
# Import System.IO assembly
Add-Type -AssemblyName System.IO
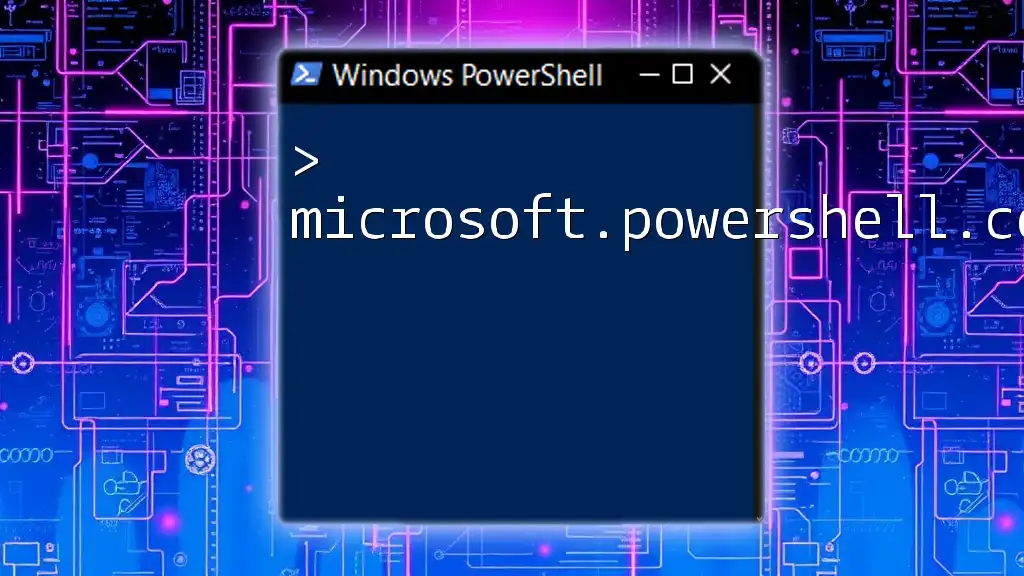
Creating a Basic FilesystemWatcher
Defining the Path to Monitor
The first step in setting up a FilesystemWatcher instance is defining the folder path you want to monitor. Here’s an example of how to set this up:
# Define the folder path
$folderPath = "C:\Path\To\Your\Folder"
Initializing the FilesystemWatcher Object
Once you have your path defined, you can initialize your FilesystemWatcher object with the following code:
# Initialize the FileSystemWatcher
$watcher = New-Object System.IO.FileSystemWatcher
$watcher.Path = $folderPath
Configuring the Watcher
Watching for Specific Changes
With the watcher created, you can configure it to respond to specific file system change events. The types of change you can monitor include creation, changes, deletion, and renaming of files. Here’s how you can enable these features:
$watcher.EnableRaisingEvents = $true
$watcher.IncludeSubdirectories = $true
$watcher.Filter = "*.txt" # Monitor TXT files only
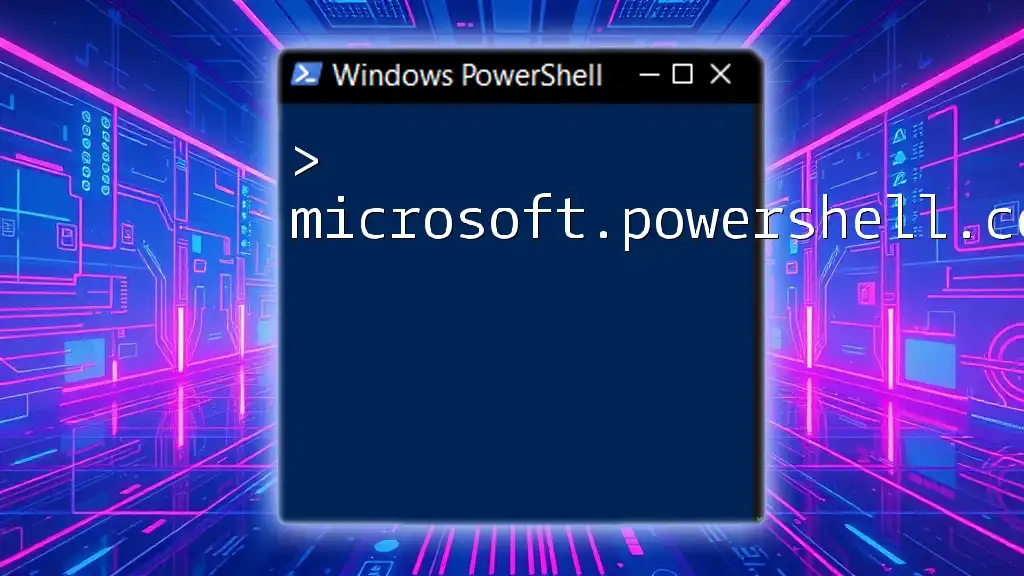
Handling Events from FilesystemWatcher
Defining the Event Handlers
To trigger actions upon file events, you need to define what happens when certain events occur. Below is an example of how to set up event handlers for file creation and deletion:
# Define what happens on file creation
$onCreated = Register-ObjectEvent -InputObject $watcher -EventName Created -Action {
Write-Host "File created: $($Event.SourceEventArgs.Name)"
}
# Define what happens on file deletion
$onDeleted = Register-ObjectEvent -InputObject $watcher -EventName Deleted -Action {
Write-Host "File deleted: $($Event.SourceEventArgs.Name)"
}
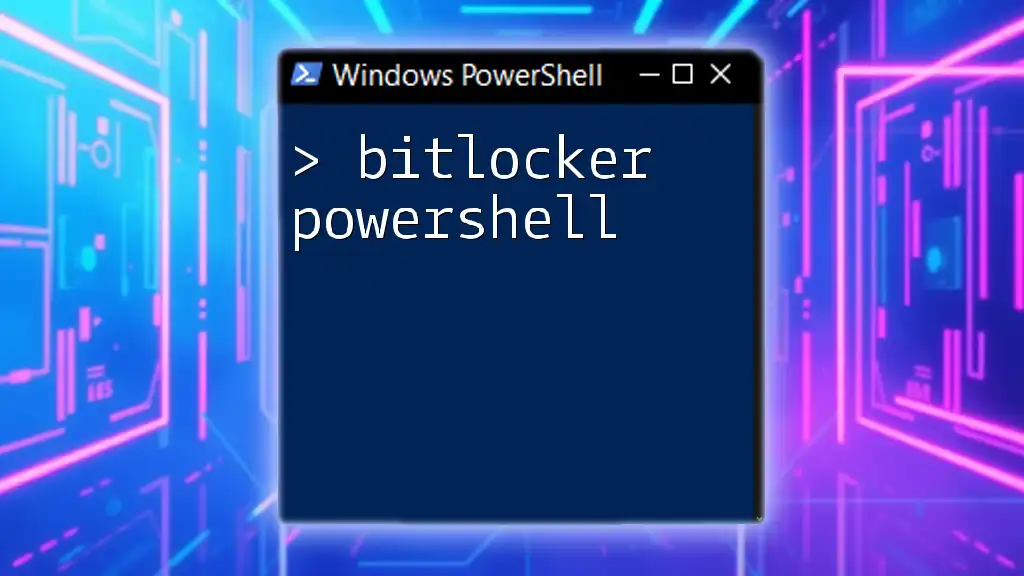
Example Script: Monitoring a Folder
Complete Code Snippet
Here is a comprehensive script that combines all the previous components to monitor a folder for changes. This example will alert you upon the creation or deletion of `.txt` files in the specified directory.
# Complete script to monitor a folder
$folderPath = "C:\Path\To\Your\Folder"
$watcher = New-Object System.IO.FileSystemWatcher
$watcher.Path = $folderPath
$watcher.EnableRaisingEvents = $true
$watcher.IncludeSubdirectories = $true
$watcher.Filter = "*.txt"
$onCreated = Register-ObjectEvent -InputObject $watcher -EventName Created -Action {
Write-Host "File created: $($Event.SourceEventArgs.Name)"
}
$onDeleted = Register-ObjectEvent -InputObject $watcher -EventName Deleted -Action {
Write-Host "File deleted: $($Event.SourceEventArgs.Name)"
}
# Keep the script running
while ($true) { Start-Sleep -Seconds 1 }
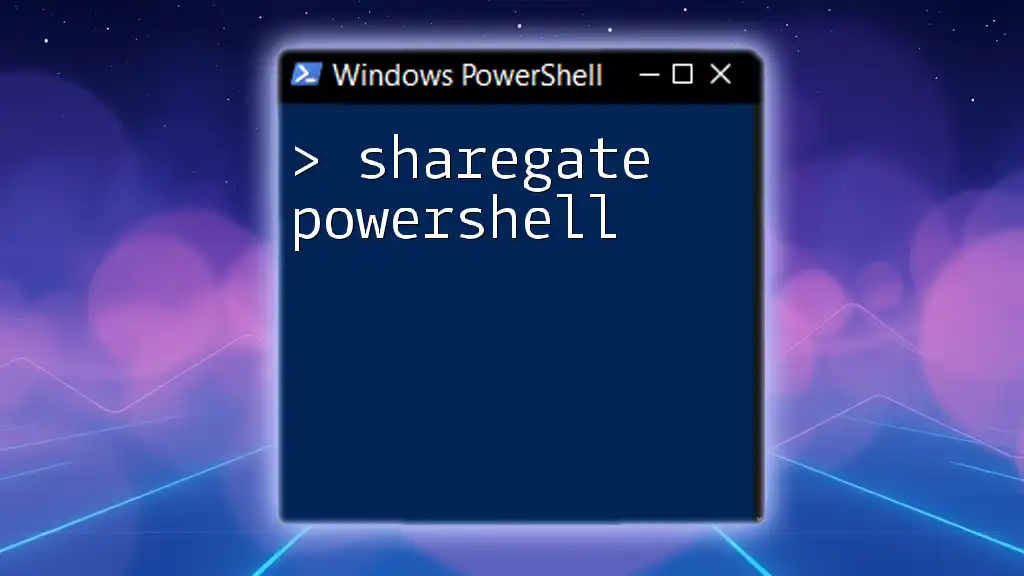
Customizing Your FilesystemWatcher
Setting Up Multiple Watchers
In scenarios where you need to monitor multiple directories, you can instantiate additional FilesystemWatcher objects for each directory. This allows for comprehensive monitoring across several locations.
# Example of setting up multiple watchers
$folderPath1 = "C:\Path\To\First\Folder"
$folderPath2 = "C:\Path\To\Second\Folder"
# Create watchers for each folder...
Filtering with Regular Expressions
Advanced filtering can be achieved using regular expressions to monitor specific file naming patterns or extensions. This enables more granular control over what file changes to respond to.
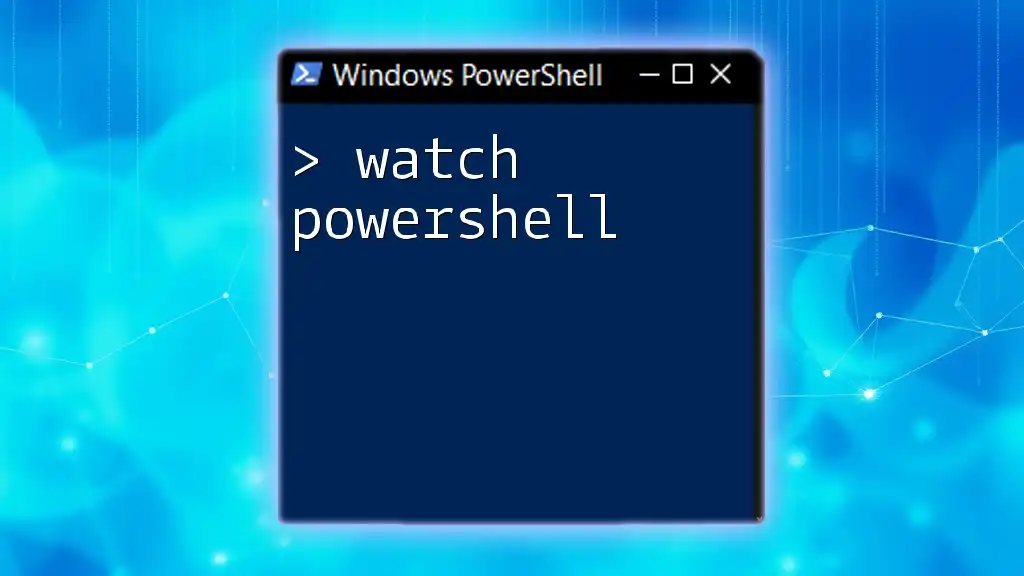
Best Practices for Using FilesystemWatcher
Resource Management
Properly disposing of FilesystemWatcher objects and event handlers is critical to avoid resource leaks. This can be performed using the `Unregister-Event` command to cleanly remove event subscriptions and `Dispose()` method on the watcher when it's no longer needed.
Error Handling
In any monitoring solution, it’s important to handle exceptions gracefully. Implementing robust error handling strategies ensures your script continues to run smoothly even when unexpected issues arise.
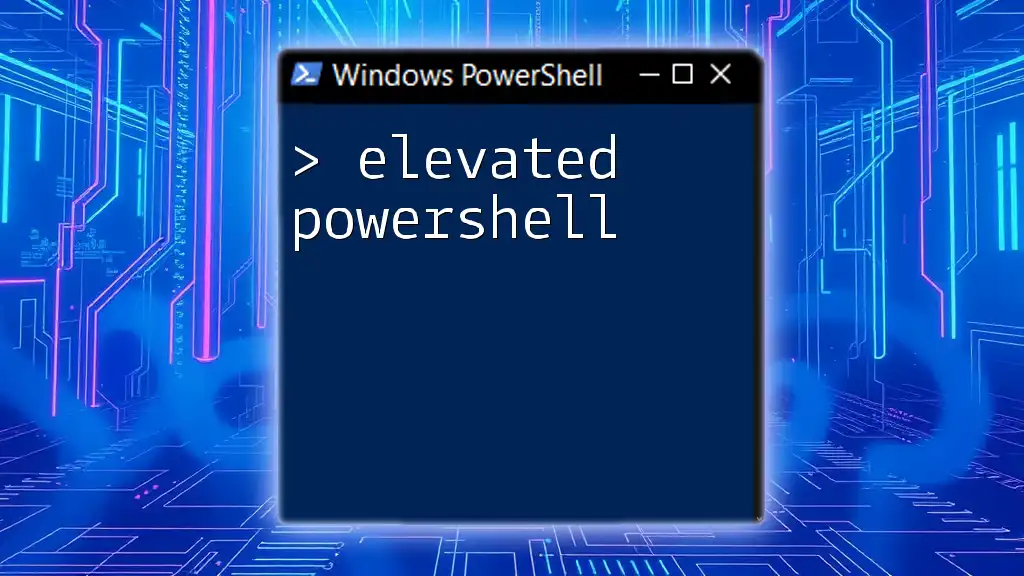
Common Use Cases
Automating Backups
One practical application of FilesystemWatcher is to automate backup solutions. Upon detecting a file creation or modification event, a backup script can automatically be triggered to ensure data integrity.
Alerting Systems
You could also set up an alerting system where notifications (via email, webhooks, etc.) are sent whenever a significant file operation occurs. This setup is particularly valuable for critical environments that require constant surveillance.
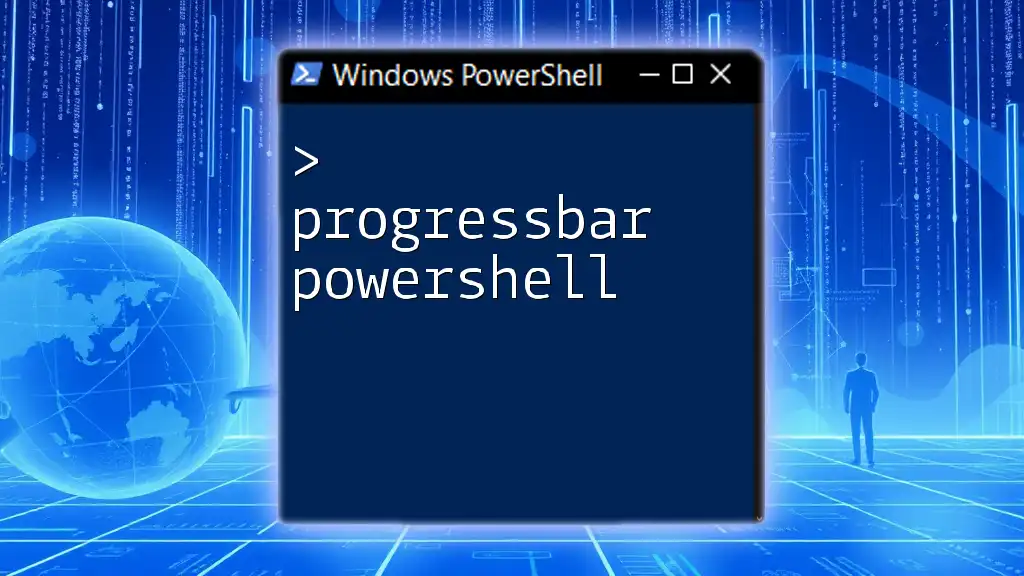
Troubleshooting FilesystemWatcher Issues
Common Problems and Solutions
There are a few challenges users may face when implementing FilesystemWatcher:
- Delays in events being fired: This can occur due to system performance issues. Ensure your system is optimized to handle multiple events efficiently.
- Issues with file locks: Resolved by adding error handling to manage access conflicts appropriately.
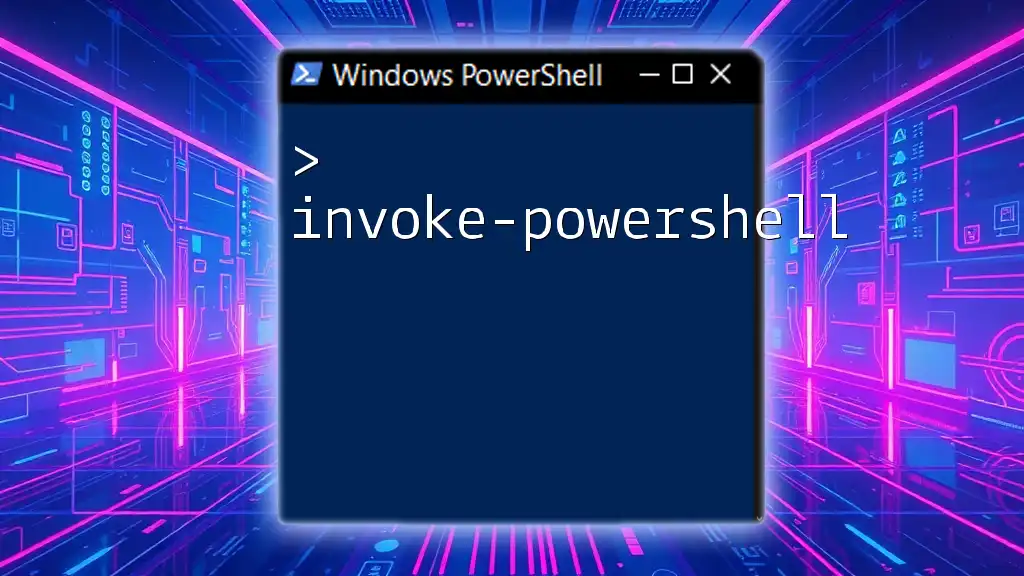
Conclusion
Utilizing FilesystemWatcher in PowerShell empowers users to create dynamic and responsive scripts that enhance their workflows. By automating file monitoring and incorporating event-driven actions, organizations can leverage real-time insights and improve operational efficiency. Experimenting with the provided code snippets can lead to greater understanding and innovative applications tailored to your specific needs.
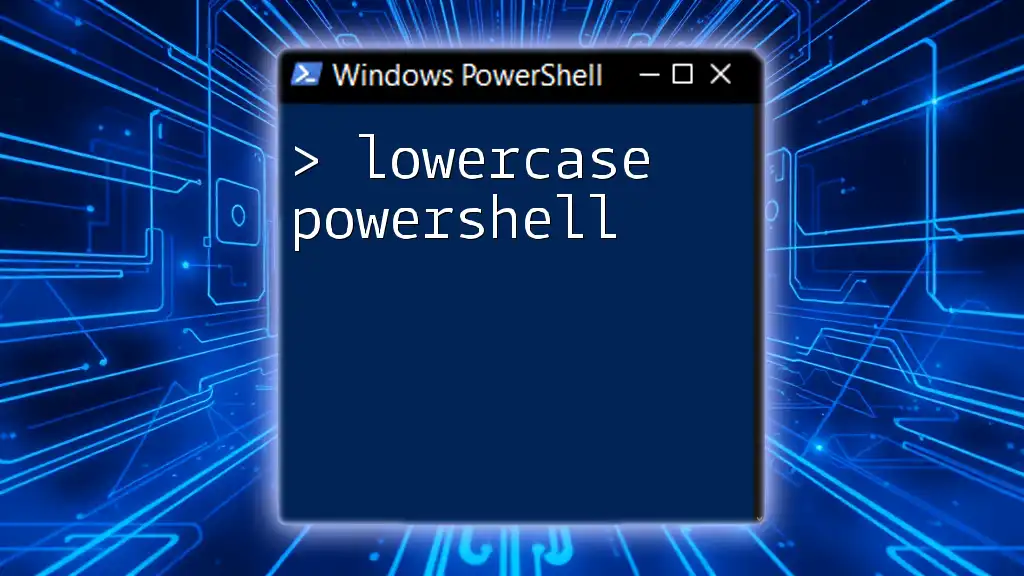
Additional Resources
For further learning, consider exploring the official Microsoft documentation on FilesystemWatcher, as well as recommended books or online courses focused on PowerShell scripting and .NET integration. This foundational knowledge will enhance your ability to implement FilesystemWatcher effectively in diverse environments.