In PowerShell, you can retrieve the current working directory path using the `Get-Location` cmdlet, which provides a straightforward way to see your current file context.
Get-Location
Understanding File Paths in PowerShell
What is a File Path?
A file path is a string that provides a unique location to access a file or directory within a file system. Understanding file paths is crucial for scripting and automation in PowerShell because it enables users to manipulate files and directories effectively. File paths can be categorized into two types: absolute and relative paths.
Types of Paths
Absolute Path
An absolute path specifies a location in the file system from the root directory. It contains all the necessary information to locate a file from the very beginning of the file hierarchy. For example, `C:\Users\YourName\Documents\MyFile.txt` is an absolute path that directly points to a file.
Relative Path
A relative path provides a location in relation to the current working directory. It lacks the root directory information, which makes it more concise for navigation within the present directory. For instance, if your current directory is `C:\Users\YourName`, then a relative path to your file would simply be `Documents\MyFile.txt`.
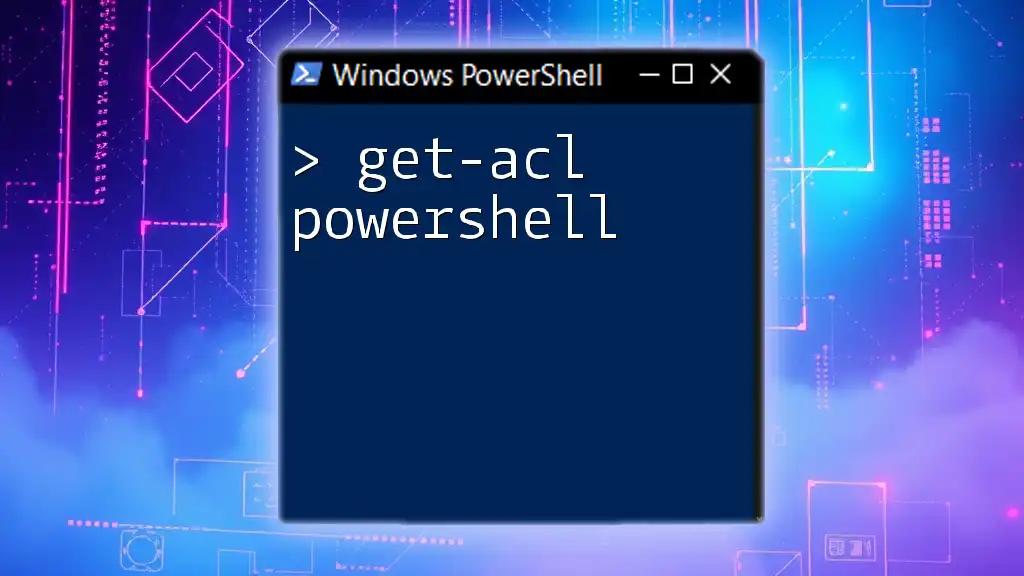
Basic Commands to Get Path in PowerShell
Using the `Get-Location` Command
The `Get-Location` command retrieves the current working directory in PowerShell. It’s a straightforward way to find out where you are in your file system.
Get-Location
When you run this command, you will receive output similar to this:
Path
----
C:\Users\YourName
This shows you your working directory, which is critical when constructing paths for scripts.
Using the `Resolve-Path` Command
The `Resolve-Path` command is invaluable for converting paths specified in a script to their full absolute form. This command resolves a relative path into an absolute path, ensuring you know exactly where something is located.
Resolve-Path .\MyFolder
When executed, if `MyFolder` exists in your current directory, you'll receive an output such as:
Path
----
C:\Users\YourName\MyFolder
This confirms the complete path to `MyFolder`, aiding in file manipulations.
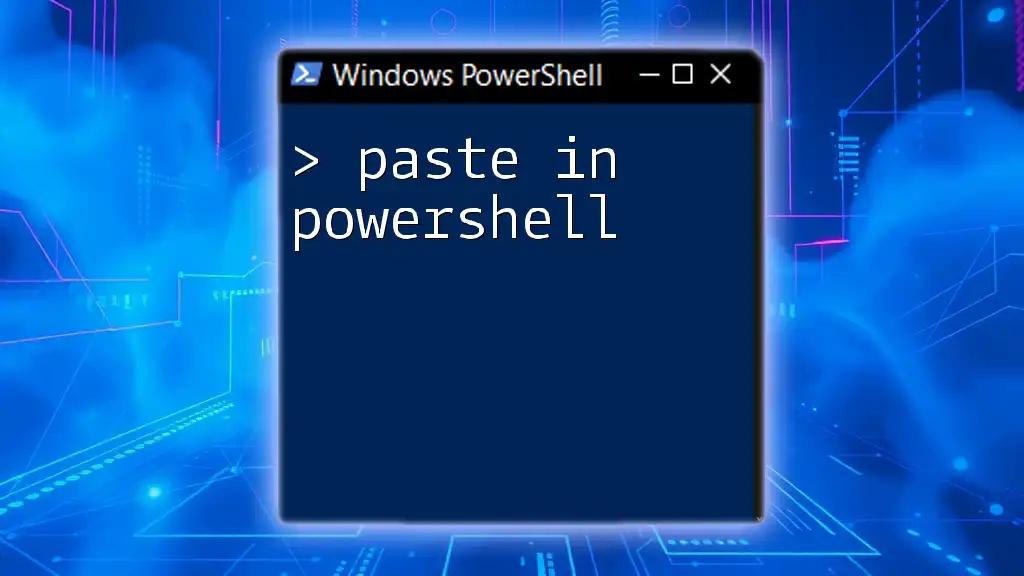
Working with Paths in PowerShell Scripting
Creating a Path Variable
In PowerShell, you can easily create variables to store paths, making your scripts cleaner and more maintainable. Using a dedicated variable allows for easy referencing multiple times.
$path = "C:\MyFolder"
Now, every time you need to reference `C:\MyFolder`, you can use the `$path` variable. This avoids redundancy and minimizes risks in complex scripts.
Checking if a Path Exists
Prior to performing operations on a file or directory, it's wise to ensure its existence using the `Test-Path` cmdlet. This command returns `True` if the path exists, or `False` if it doesn’t.
Test-Path $path
This command will return `True` if the `C:\MyFolder` exists, allowing you to safeguard against errors before executing subsequent commands.
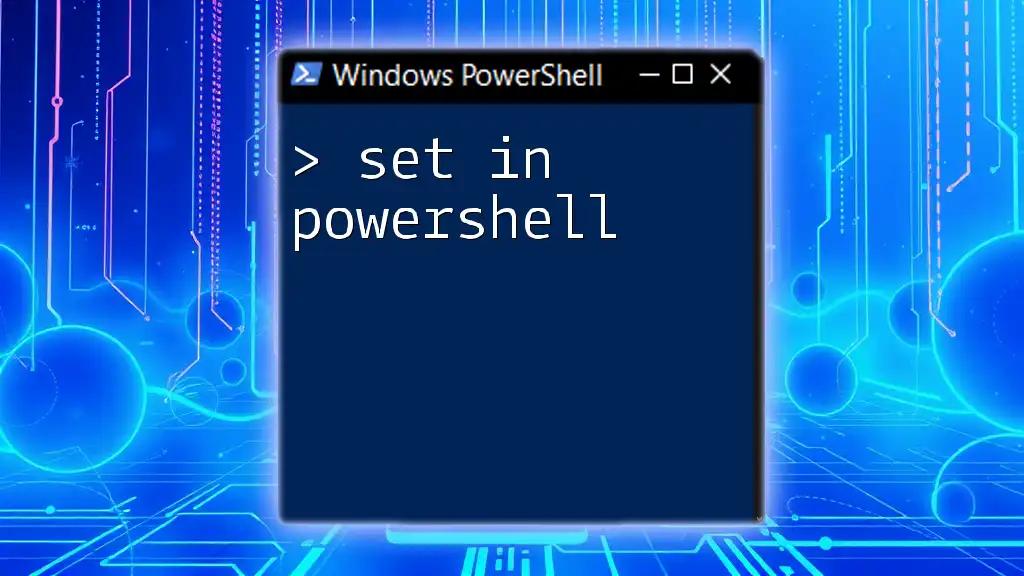
Advanced Path Manipulations
Combining Paths
Combining different parts of paths can often be required during scripting. The `Join-Path` cmdlet assists in this by creating a complete path string using a base path and adding a child path.
$combinedPath = Join-Path -Path $path -ChildPath "SubFolder"
If `$path` is set to `C:\MyFolder`, the above example will yield `C:\MyFolder\SubFolder`, ensuring that paths are constructed correctly without manual errors.
Extracting Path Components
There may be occasions when you need to break down paths into their individual components. The `Split-Path` cmdlet is designed for extracting desired pieces from a complete path.
$filePath = "C:\MyFolder\MyFile.txt"
$directory = Split-Path $filePath -Parent
$fileName = Split-Path $filePath -Leaf
In this case:
- `$directory` will contain `C:\MyFolder`.
- `$fileName` will retrieve just `MyFile.txt`.
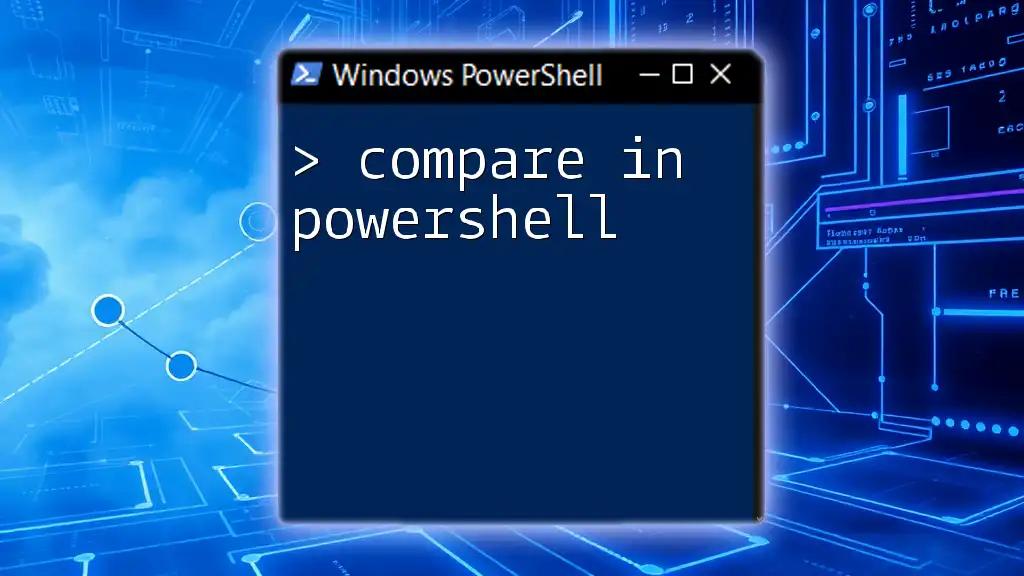
Environment Variables and Paths
Introduction to Environment Variables
Environment variables store system-wide values that can be very useful in scripting. Variables such as `$env:ProgramFiles` or `$env:HOMEPATH` provide paths related to system configurations.
Accessing and Using Environment Variables
You can easily access environment variables in your scripts. For example:
$programFilesPath = $env:ProgramFiles
This retrieves the path to the Program Files directory, making your scripts adaptable across different user environments and system configurations.
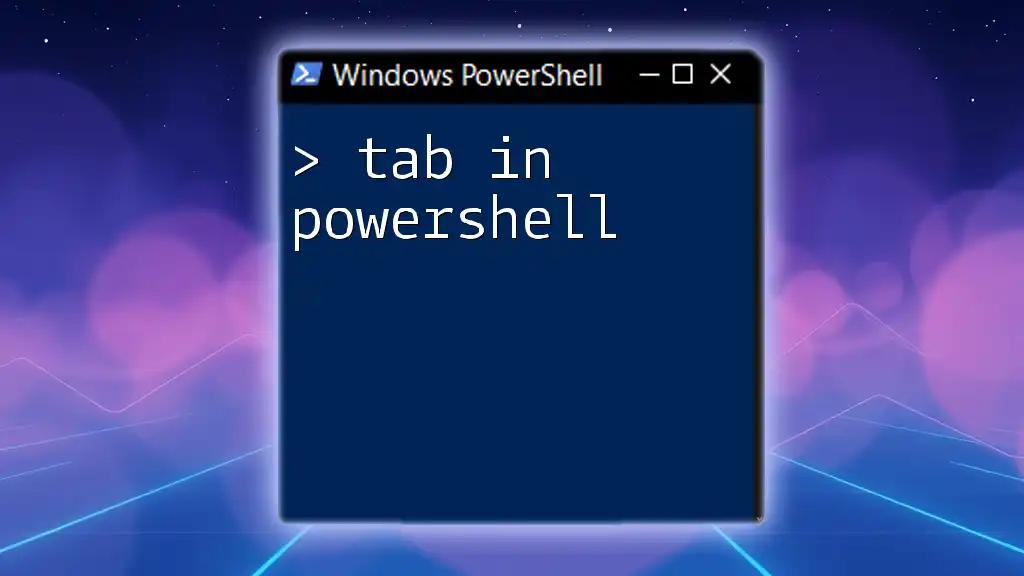
Using Built-in PowerShell Variables for Getting Paths
Understanding Automatic Variables
PowerShell has several automatic variables which can be utilized to retrieve paths directly. Two notable variables are `$PWD` and `$HOME`, which represent the current directory and the user’s home directory, respectively.
$currentDir = $PWD.Path
$homeDir = $HOME
Now, `$currentDir` contains the complete path of the current working directory, while `$homeDir` provides the path to your user’s home directory, making navigation easier in scripts.
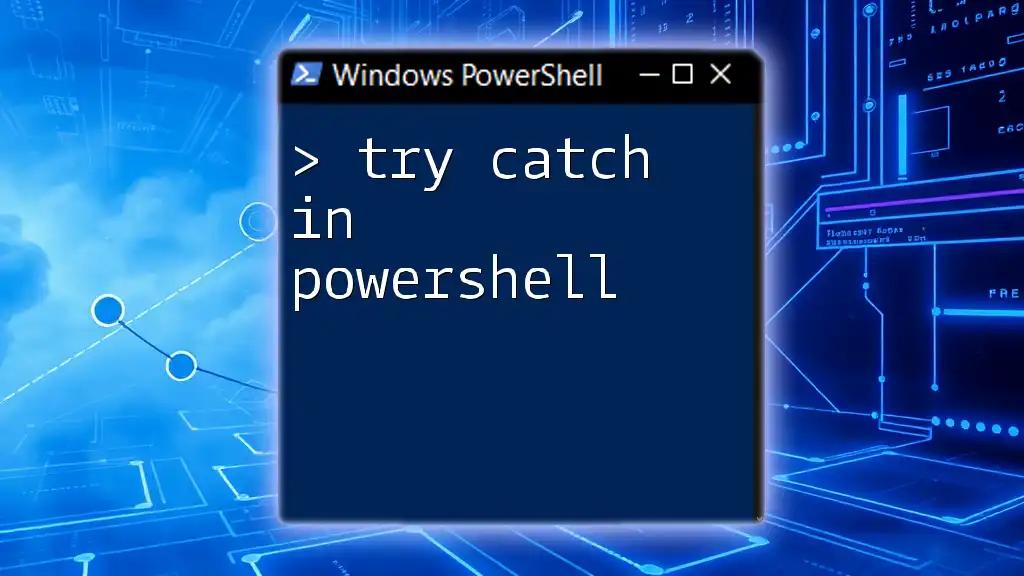
Best Practices for Path Management
Using Quotes and Escape Characters
When dealing with paths that contain spaces or special characters, it's essential to encapsulate those paths in quotes. This helps PowerShell parse the path correctly. For example:
$pathWithSpaces = "C:\My Documents"
Not using quotes could lead to syntax errors or unintended consequences in your scripts.
Maintaining Portability in Scripts
For scripts that will be run on various systems, it’s crucial to employ relative paths and utilize environment variables where applicable. This will help ensure that your scripts are adaptable to different file structures and configurations.
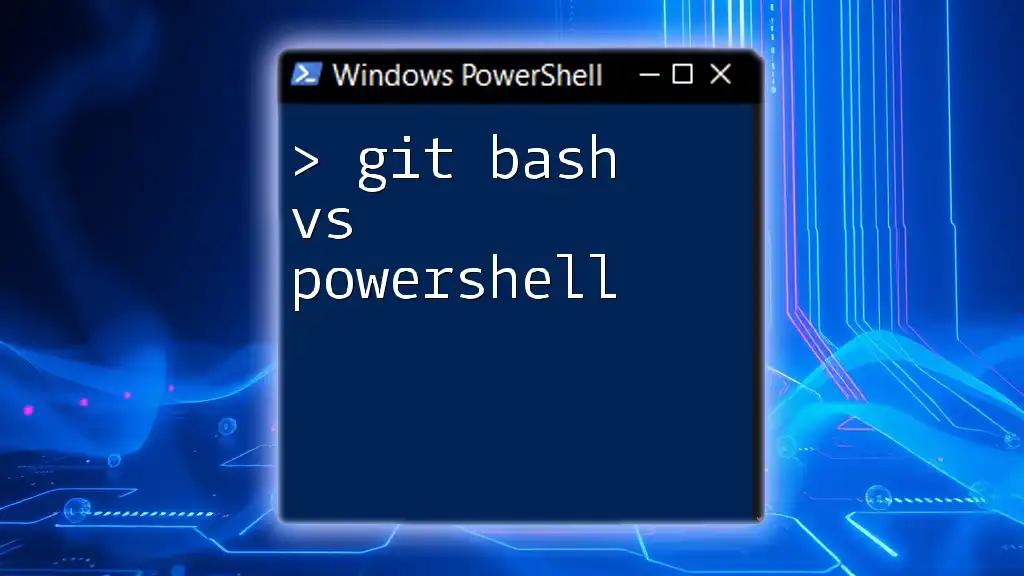
Troubleshooting Common Path Issues
Error Messages Related to Paths
You might encounter errors while dealing with paths, such as "Path not found." This typically occurs if you reference a path that does not exist.
Best Practices for Debugging Path Issues
Using the `-Verbose` parameter can provide you with helpful insights while executing commands:
Get-ChildItem -Path "C:\MyFolder" -Verbose
This additional output can help you track down issues and understand what PowerShell is doing at each step.
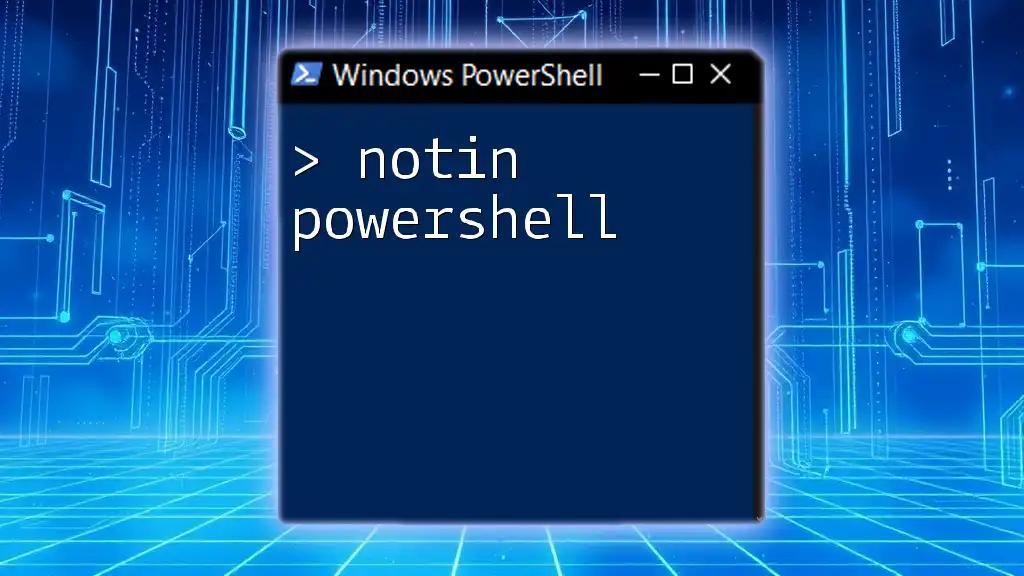
Conclusion
Understanding how to get path in PowerShell is a fundamental skill that can dramatically improve your scripting and automation capabilities. Mastering commands like `Get-Location`, `Resolve-Path`, and managing paths through variables will empower you to perform a myriad of tasks efficiently. Practice developing your path-related commands, and don’t hesitate to explore additional resources that can deepen your understanding.