To print a variable in PowerShell, you can use the `Write-Host` command, which outputs the value of the variable to the console.
$greeting = 'Hello, World!'
Write-Host $greeting
Understanding PowerShell Variables
What are PowerShell Variables?
In PowerShell, variables are fundamental components that store information for use throughout scripts and commands. They begin with a dollar sign (`$`) followed by a name that you assign. For example, to create a variable that holds your name, you might write:
$name = "John Doe"
It’s essential to remember that variables can store various types of data, including strings, integers, and arrays. Naming conventions are crucial; a variable name must start with a letter and can include letters, numbers, and underscores.
Types of Variables in PowerShell
PowerShell supports several data types for variables, enabling you to work with a variety of data formats:
- String: A sequence of characters, e.g., `"$name"`.
- Integer: A numerical value, e.g., `$count = 5`.
- Array: A collection of items, e.g., `$array = @(1, 2, 3)`.
Understanding these types will help you manipulate and print variables effectively.
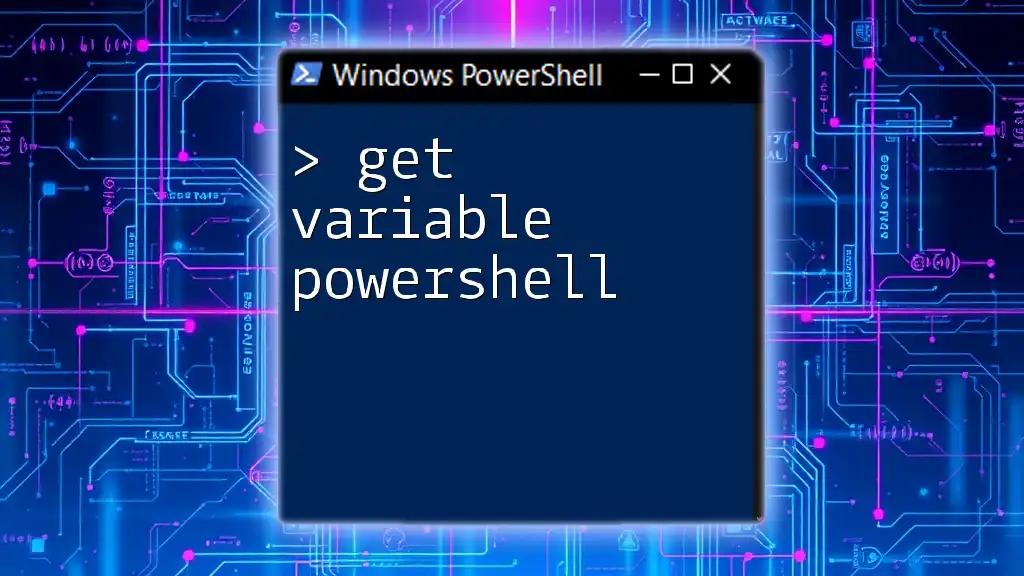
Printing Variables in PowerShell
Overview of Printing Variables
When you print a variable in PowerShell, you display its value in a readable format. This action can help you verify the contents of a variable at any point in your script and is crucial for debugging and logging purposes.
Using the `Write-Output` Cmdlet
What is `Write-Output`?
The `Write-Output` cmdlet is used to send output to the pipeline or display data on the console. You can think of it as a means to print your variable contents.
Example of Using `Write-Output`:
$myVariable = "Hello, World!"
Write-Output $myVariable
Explanation of the Output:
When this command is executed, the console displays "Hello, World!". The variable `$myVariable` passes its content through `Write-Output`, demonstrating how easily you can print variable values.
The `Echo` Command in PowerShell
Understanding the `Echo` Alias:
In PowerShell, `echo` is an alias for `Write-Output`. This means using `echo` achieves the same result as invoking `Write-Output`.
Example of `Echo` in Action:
$greeting = "Welcome to PowerShell!"
echo $greeting
Similar to `Write-Output`, this will print "Welcome to PowerShell!" to the console. In PowerShell, using aliases can increase your efficiency, as they often require less typing.
Advanced Usage:
You can also pipe `echo` output to another command. For example:
$filename = "output.txt"
echo "This is a test." | Out-File $filename
This command redirects the echoed string to a file named `output.txt`.
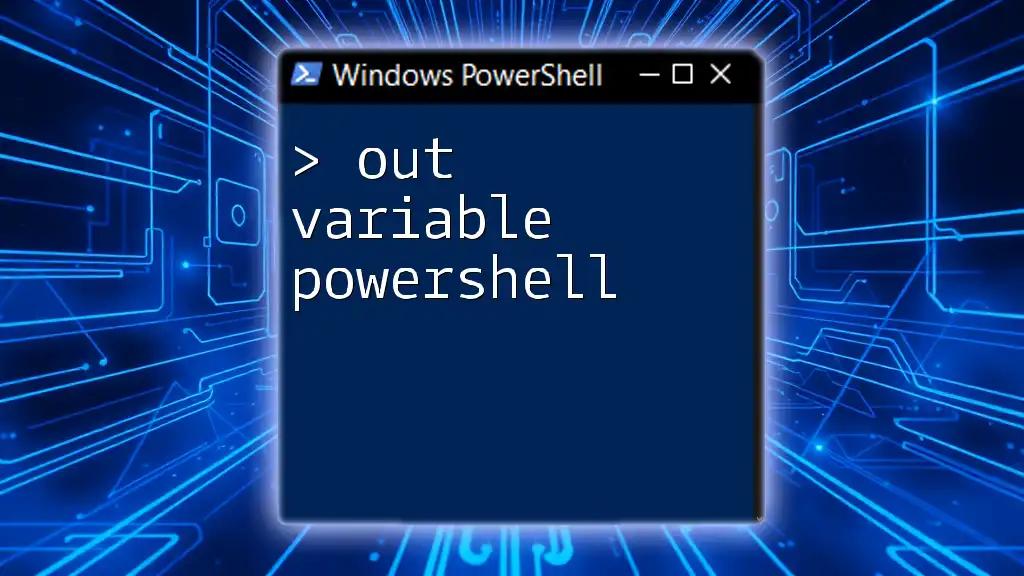
Using `Write-Host` to Print Variables
What is `Write-Host`?
`Write-Host` is another cmdlet for printing messages in PowerShell. However, it specifically writes directly to the console and does not send its output down the pipeline. This means the printed message cannot be captured or redirected.
When to use `Write-Host` instead of `Write-Output`:
Use `Write-Host` primarily when you want to provide information intended solely for the user, and not meant for further processing.
Example of `Write-Host`:
$username = "User1"
Write-Host "Current user: $username"
This command prints "Current user: User1" directly to the console. Because `Write-Host` focuses on screen output, it can display colorful messages using parameters to control text color:
Write-Host "This message is in green!" -ForegroundColor Green
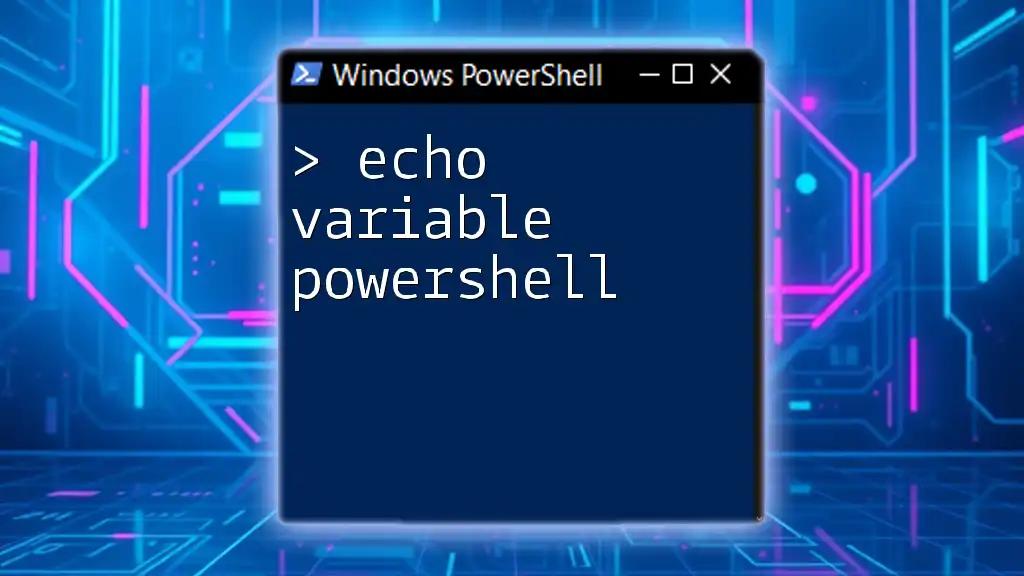
Printing Variables in PowerShell Scripts
Importance of Printing in Scripts
In scripts, printing variables is an invaluable practice. It helps in debugging by allowing you to see variable values at given points in execution. This visibility can identify logical errors, loops not functioning correctly, or values not being calculated as expected.
Example Script to Print Variables
A simple script that demonstrates printing variables is one that sums two numbers and prints the result:
$number1 = 10
$number2 = 20
$sum = $number1 + $number2
Write-Output "The sum of $number1 and $number2 is: $sum"
When executed, the output will be "The sum of 10 and 20 is: 30". This example highlights how printing can provide real-time feedback during script execution.
Using Format-String for Formatted Output
Formatting strings enhance the readability of output. PowerShell allows you to format text easily using the `-f` operator, which enables you to insert variable values into a string.
Example:
$user = "Alice"
$age = 30
Write-Output ("User: {0}, Age: {1}" -f $user, $age)
Output: "User: Alice, Age: 30". This method ensures that your output is organized and professional-looking.
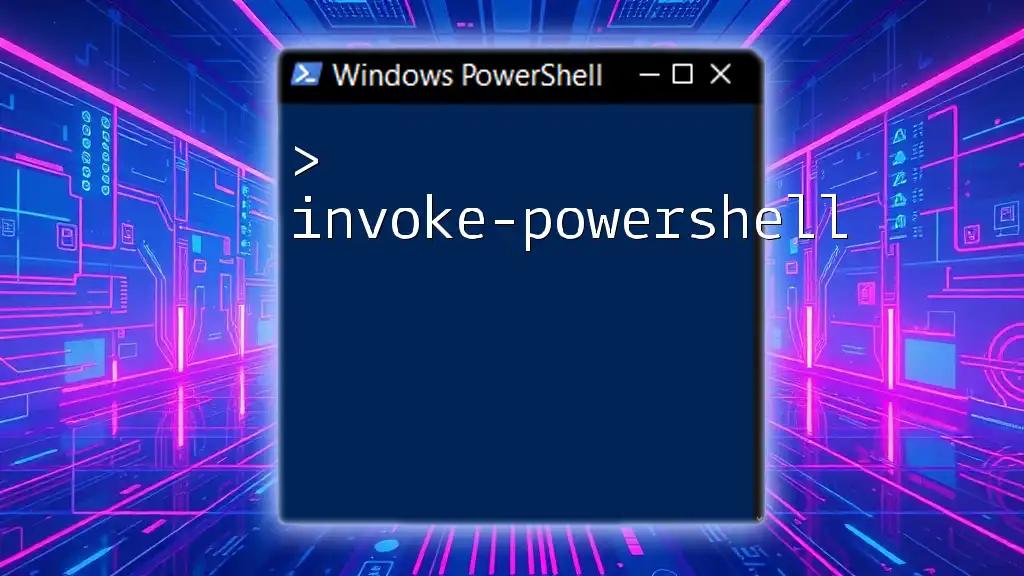
Common Mistakes When Printing Variables
Common Pitfalls
As you experiment with printing variables, you may encounter some common mistakes, such as:
- Forgetting to enclose strings in quotes.
- Using `Write-Host` rather than `Write-Output` when you want to capture output later.
- Incorrectly using variable names (e.g., typos).
Solutions to Common Problems
When troubleshooting output issues, start by checking for syntax errors and ensuring you're using the correct cmdlets. Remember, the difference in output between `Write-Host` and `Write-Output` may cause frustration if not understood.
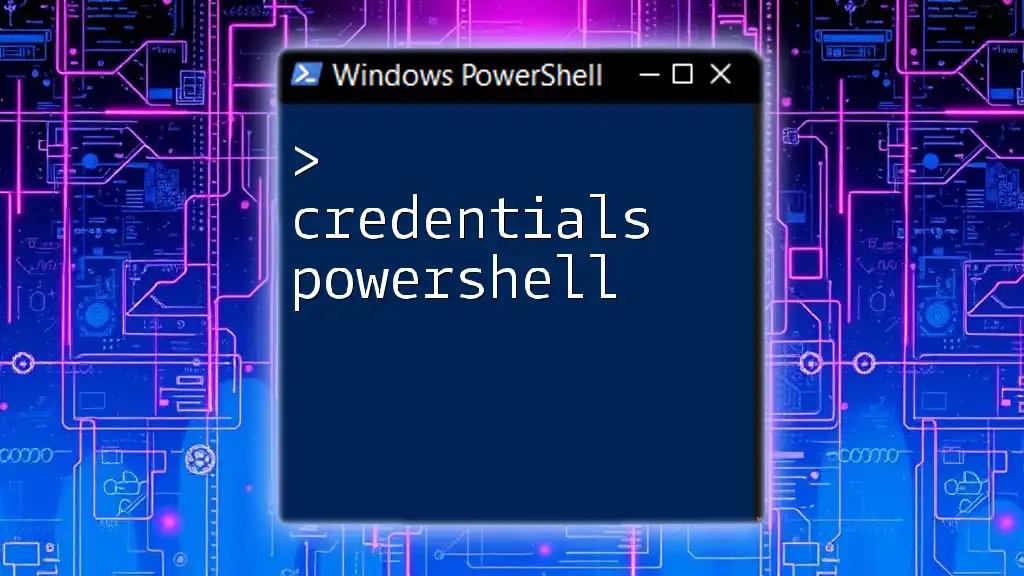
Conclusion
In this guide, we've explored how to effectively print variables in PowerShell. Understanding how to use `Write-Output`, `echo`, and `Write-Host` not only enhances your scripting skills but also facilitates better debugging practices. Whether you are a beginner or an experienced scripter, mastering these skills will streamline your workflow and improve your PowerShell scripts. Practice often to become proficient in printing and manipulating variables, and you'll see the benefits in your coding endeavors!