In PowerShell, you can sort an array in ascending order using the `Sort-Object` cmdlet, as demonstrated in the following code snippet:
$array = 5, 3, 8, 1, 4
$sortedArray = $array | Sort-Object
Write-Host $sortedArray
Understanding PowerShell Arrays
What is an Array in PowerShell?
In PowerShell, an array is a data structure that holds a collection of items. These items can be of different data types, including strings, numbers, or objects. Arrays are essential for organizing and manipulating data efficiently within your scripts. They allow you to store multiple values, which you can access and modify as needed.
Example of Creating an Array
Here's a simple example of how to create an array in PowerShell:
$myArray = @("apple", "orange", "banana")
In this example, we define an array called `$myArray` that contains three elements: "apple," "orange," and "banana". This array can be utilized in various ways, including looping through elements, accessing individual items, and performing operations like sorting.
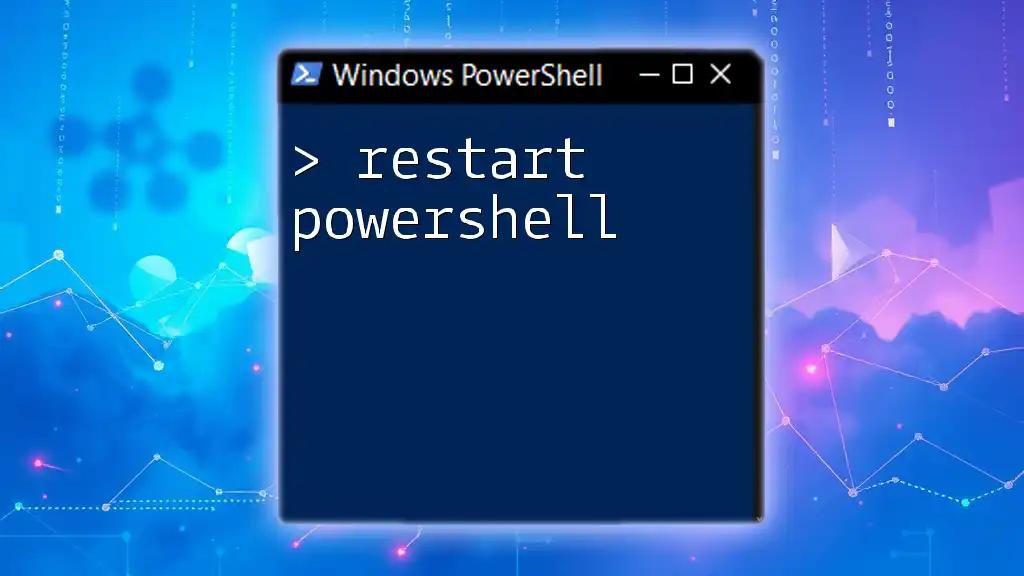
PowerShell Sort Array Basics
What is Array Sorting?
Array sorting is the process of arranging the elements of an array in a specific order, typically alphabetical or numerical. Sorting is a fundamental operation in programming and is often necessary when organizing data for easy access and readability. Sorting helps to make sense of data, especially when dealing with large datasets.
Key Concepts of Sorting Arrays in PowerShell
When sorting arrays in PowerShell, it's crucial to understand the different order types—ascending and descending. Ascending order will arrange the elements from the smallest to largest (A-Z or 0-9), while descending order will do the opposite.
Furthermore, handling different data types is essential. For example, sorting strings and numbers follows different logic. String sorting is based on alphabetical order, while numerical sorting relies on the numerical value.
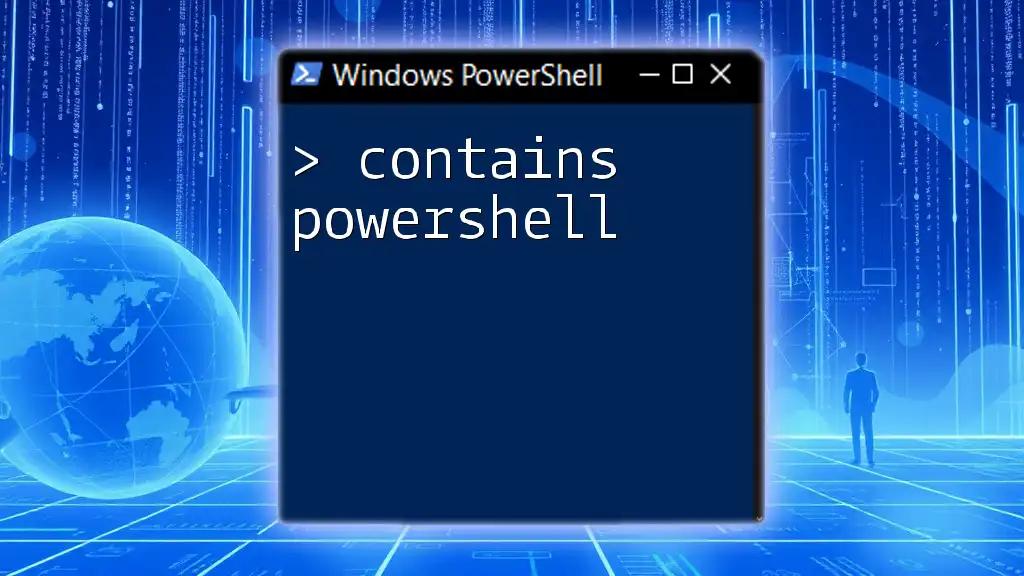
Using the Sort-Object Cmdlet
Overview of the Sort-Object Cmdlet
The `Sort-Object` cmdlet is the primary tool in PowerShell for sorting arrays. It allows you to sort data based on specified properties, making it highly versatile. The basic syntax for using this cmdlet is straightforward:
$sortedArray = $myArray | Sort-Object
This line of code takes `$myArray`, pipes it to `Sort-Object`, and returns a new array with its elements sorted in ascending order by default.
Sorting an Array of Strings
Sorting strings in PowerShell is a breeze. Here’s how you can do it:
$fruits = @("apple", "banana", "orange")
$sortedFruits = $fruits | Sort-Object
In this case, the output will be:
apple
banana
orange
PowerShell sorts the array in alphabetical order, which is helpful when you need data in a consistent format.
Sorting an Array of Numbers
Similarly, you can sort numeric arrays easily:
$numbers = @(5, 2, 9, 1)
$sortedNumbers = $numbers | Sort-Object
The sorted output will be:
1
2
5
9
This output demonstrates how PowerShell arranges numbers from the lowest to the highest value, which can facilitate numerical analysis efficiently.
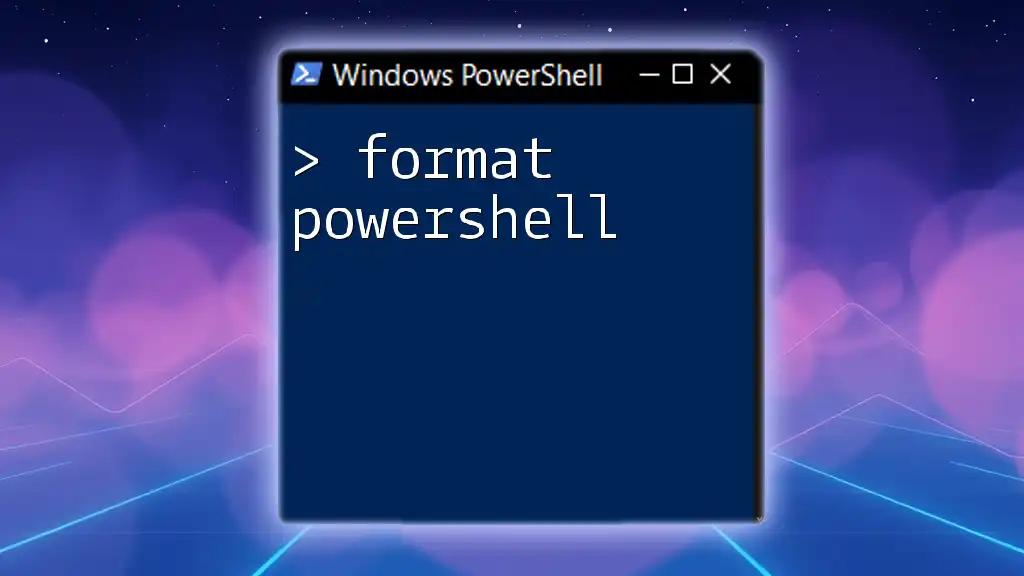
Sorting in Descending Order
Using the -Descending Parameter
To sort an array in descending order, you can simply use the `-Descending` parameter:
$sortedFruitsDesc = $fruits | Sort-Object -Descending
In this case, the output will be:
orange
banana
apple
This output shows that PowerShell has reversed the sorting order, placing "orange" at the top as it comes last alphabetically.
Example of Sorting Numbers in Descending Order
You may also sort numeric values in descending order using the same method:
$sortedNumbersDesc = $numbers | Sort-Object -Descending
The result will be:
9
5
2
1
As expected, PowerShell sorts the values from highest to lowest, which may be useful in reporting scenarios where the largest figures are of primary interest.

Custom Sorting with Sort-Object
Sorting by Length of String
One interesting feature of PowerShell is the ability to sort by custom criteria. For example, if you want to sort an array of strings by their length, you can use a script block with `Sort-Object`:
$words = @("cat", "elephant", "dog")
$sortedByLength = $words | Sort-Object { $_.Length }
The output will look like this:
cat
dog
elephant
Here, "cat" appears first as it has the fewest characters. This approach allows for a flexible sorting mechanism tailored to specific needs.
Sorting Objects by Property
PowerShell can also sort more complex data structures like objects. Here’s how to sort an array of objects by a specific property:
$people = @(
[PSCustomObject]@{ Name = "Alice"; Age = 30 },
[PSCustomObject]@{ Name = "Bob"; Age = 25 },
[PSCustomObject]@{ Name = "Charlie"; Age = 35 }
)
$sortedPeople = $people | Sort-Object Age
The output will be:
Name Age
---- ---
Bob 25
Alice 30
Charlie 35
In this example, individuals in the `$people` array have been sorted by their age property. This feature is incredibly useful when dealing with datasets containing various attributes.
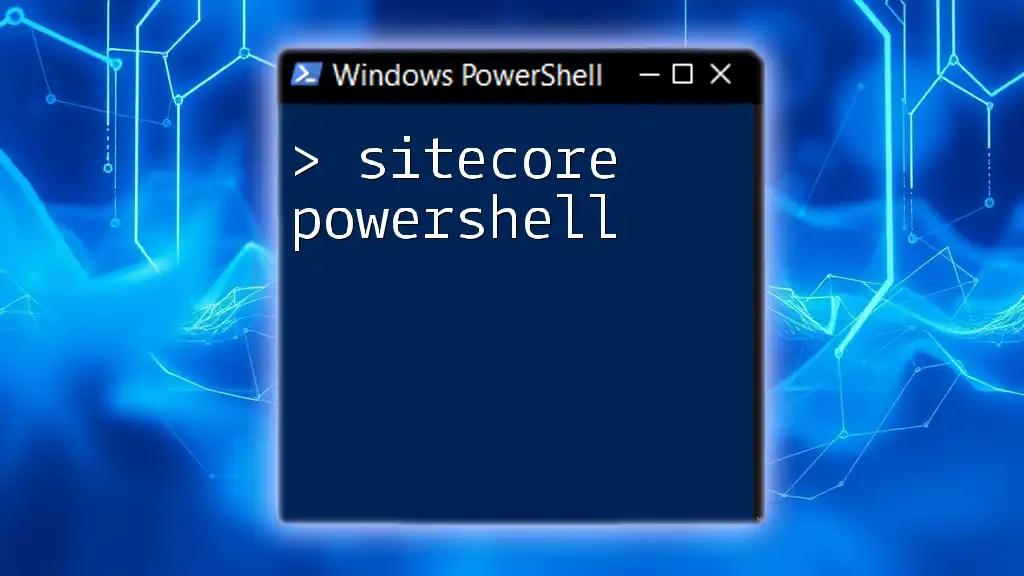
Working with Complex Data Structures
Sorting Multidimensional Arrays
Multidimensional arrays can be a bit more complex but still manageable in PowerShell. Here’s an example of sorting a two-dimensional array:
$array2D = @(
@(1, 3, 2),
@(4, 6, 5)
)
$sortedArray2D = $array2D | ForEach-Object { $_ | Sort-Object }
This example sorts each row of the multidimensional array. Note that the output might require additional processing to visualize the final structure.
Pyramid of Sorting
For more advanced uses, you might want to implement custom comparison logic. This allows you to create sorting mechanisms based on more intricate rules, such as multiple criteria or even conditions within data fields.
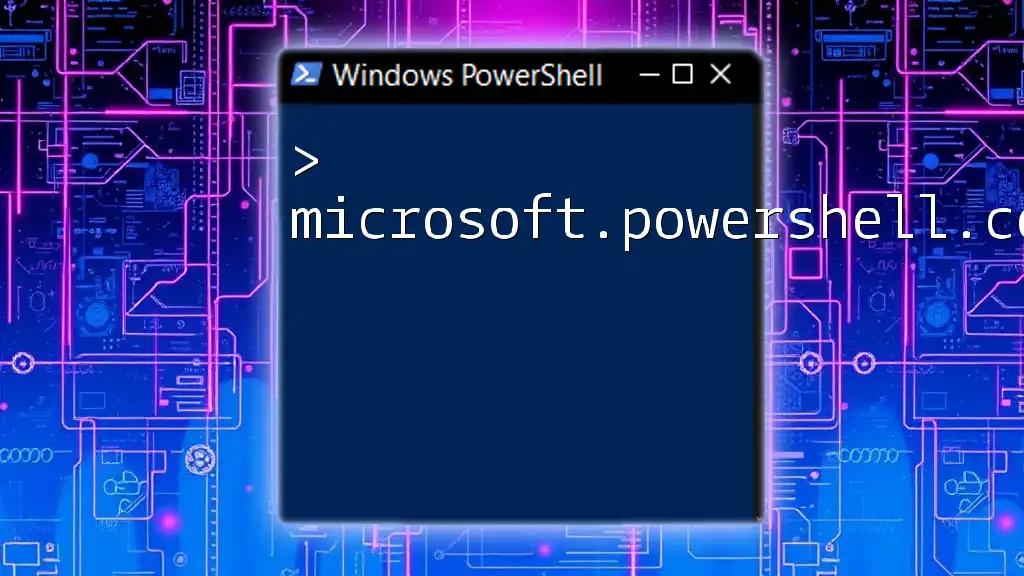
Common Errors and Troubleshooting
Common Mistakes When Sorting Arrays
When sorting arrays in PowerShell, beginners may encounter common pitfalls. Make sure to consider the following:
-
Data Types: Sorting strings with numbers or mixing data types may lead to unexpected results. PowerShell will sort strings alphabetically and numbers numerically, possibly leading to confusion.
-
Sort Order: Remember that `Sort-Object` defaults to ascending order. Always specify `-Descending` if you want to reverse this behavior.
Example of Error Handling and Debugging
If an error occurs while sorting, first check if your array contains mixed data types or if you have referenced the correct property names when sorting objects. Implementing error handling can help resolve these issues, enhancing your scripts' robustness.
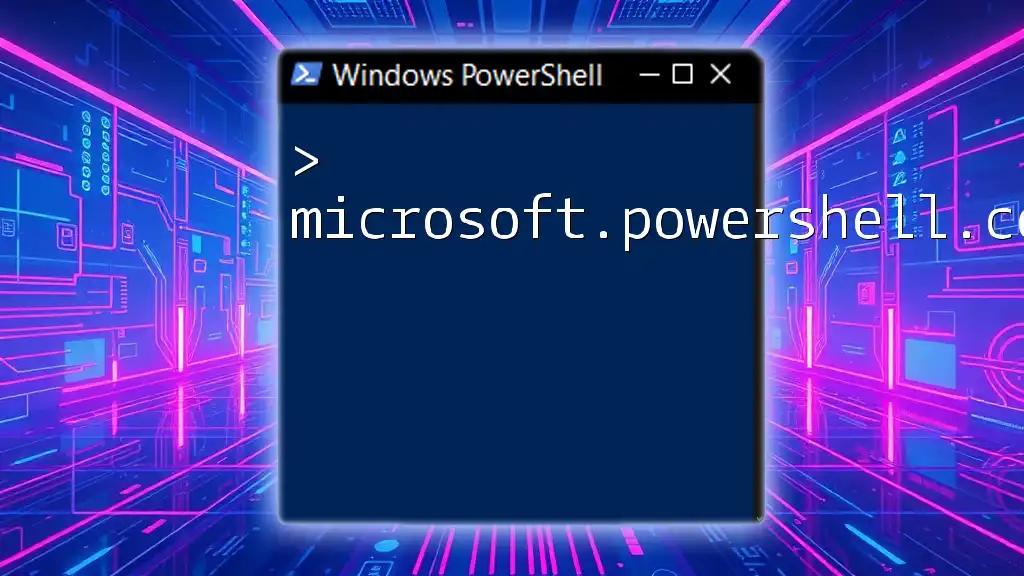
Conclusion
In summary, sorting arrays in PowerShell is a fundamental yet powerful skill. With the `Sort-Object` cmdlet, you can effortlessly manipulate data, whether it be strings, numbers, or complex objects. Understanding how to sort in both ascending and descending orders, as well as utilizing custom sorting methods, will significantly enhance your scripting capabilities. Practice these techniques with various examples, and you'll find sorting arrays becomes second nature.
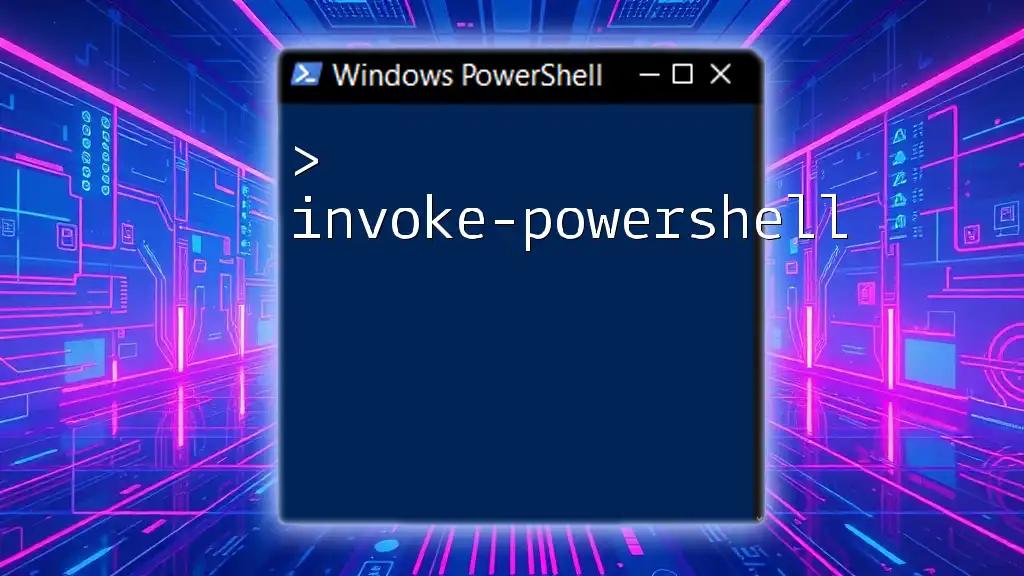
Additional Resources
For more information, consider checking the [official PowerShell documentation](https://docs.microsoft.com/powershell/) for in-depth guides and examples. There are also numerous resources available for mastering arrays and data manipulation, helping you deepen your understanding as you continue your PowerShell journey.