In PowerShell, you can easily import JSON data into a PowerShell object using the `ConvertFrom-Json` cmdlet, which converts JSON strings into native PowerShell objects for further manipulation.
$jsonData = Get-Content -Path 'data.json' | ConvertFrom-Json
Understanding JSON and PowerShell
What is JSON?
JSON, or JavaScript Object Notation, is a lightweight text-based format for data interchange that is easy for humans to read and write. It is primarily used for representing structured data. JSON is widely adopted in web applications for sending and receiving data between clients and servers due to its ease of use and lightweight nature.
When comparing JSON to XML, JSON tends to be less verbose and easier to parse, making it a preferred choice for data interchange in many modern applications.
Why Use PowerShell for JSON?
PowerShell, with its rich command-line interface and scripting capabilities, offers powerful tools for JSON manipulation. Its ability to easily parse, transform, and work with JSON data makes it an invaluable resource for system administrators, developers, and data analysts.
Using PowerShell to import JSON enables automation of data processes, quick analysis, and supports integration with various APIs, making it a versatile tool in today's data-driven world.
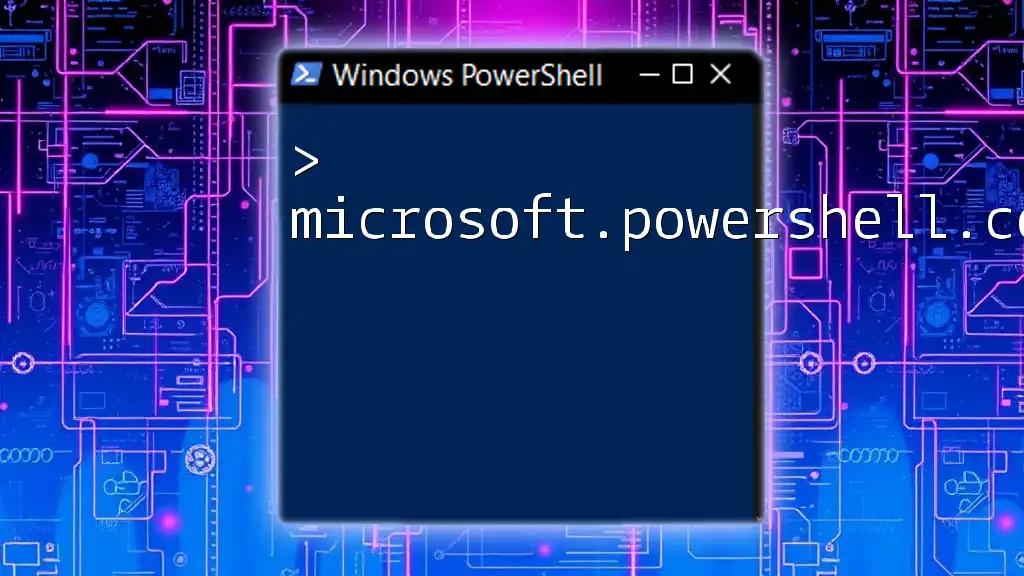
How to Import JSON in PowerShell
Basic Command Structure
PowerShell leverages the `ConvertFrom-Json` cmdlet to convert JSON-formatted text into PowerShell objects. This conversion is critical for making data manageable within PowerShell scripts.
Syntax:
$jsonObject = $jsonText | ConvertFrom-Json
Importing a JSON File
Steps to Import a JSON File
To import a JSON file, you typically use the `Get-Content` cmdlet to read the content of the file and pass that content to `ConvertFrom-Json` for processing. Here’s how you can accomplish that:
$jsonData = Get-Content -Path "path\to\your\file.json" | ConvertFrom-Json
Example: Importing a Sample JSON File
Let’s assume you have a sample JSON file located at `C:\Data\sample.json` with the following structure:
{
"Name": "John Doe",
"Age": 30,
"Skills": ["PowerShell", "JSON", "Automation"]
}
You can import this JSON file in PowerShell using the following command:
$sampleData = Get-Content -Path "C:\Data\sample.json" | ConvertFrom-Json
Accessing Data within Imported JSON
Navigating JSON Objects
After importing JSON, you can easily access properties of the resulting PowerShell object. For instance, to access the `Name` property from `sampleData`, you would run:
$sampleData.Name
This would output:
John Doe
Working with Arrays in JSON
JSON often contains arrays, which you can access similarly within PowerShell. For example, to retrieve the first skill from the `Skills` array, use:
$sampleData.Skills[0]
This will display:
PowerShell
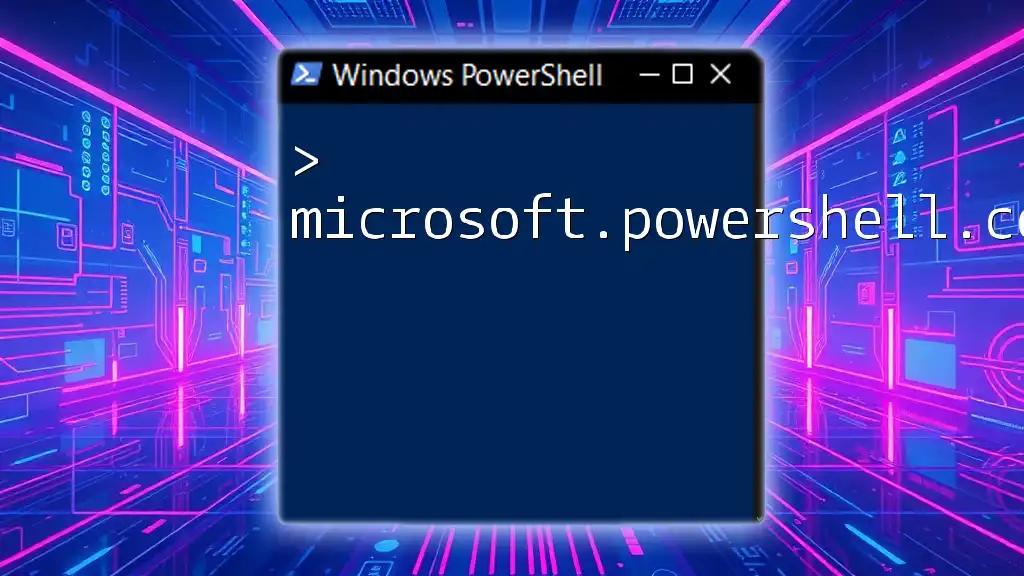
Common Scenarios
Importing Nested JSON Files
Many JSON files contain nested structures, meaning one JSON object can contain others or arrays. Understanding how to navigate these structures is essential for effective data manipulation.
Consider a nested JSON example:
{
"Employee": {
"Name": "Jane Smith",
"Department": {
"Name": "IT",
"Location": "Building A"
}
}
}
To import and access this nested JSON:
$nestedData = Get-Content -Path "C:\Data\nested.json" | ConvertFrom-Json
$nestedData.Employee.Department.Location
This will yield:
Building A
Handling Errors during the Import Process
When importing JSON, errors can arise from various sources such as malformed JSON or incorrect file paths. PowerShell provides error handling mechanisms to manage these situations gracefully.
Utilizing `Try-Catch` blocks allows you to catch and handle exceptions effectively. Here’s an example of how to implement this:
try {
$jsonData = Get-Content -Path "C:\Data\wrongfile.json" | ConvertFrom-Json
} catch {
Write-Host "An error occurred: $_"
}
This approach will provide a clear message regarding the error while maintaining the flow of your script.
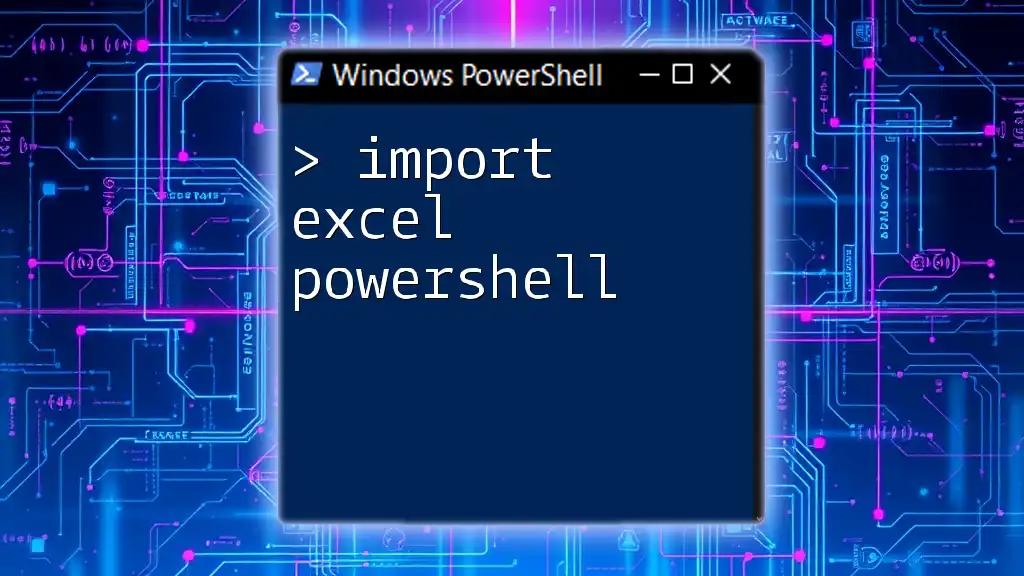
Advanced Techniques
Importing JSON via Web API
In addition to local files, you can import JSON data from web APIs directly. PowerShell’s `Invoke-RestMethod` cmdlet simplifies this process. For example, to retrieve JSON data from a hypothetical API, run:
$apiData = Invoke-RestMethod -Uri "https://api.example.com/data" -Method Get
This command will retrieve JSON data directly into a PowerShell object.
Exporting JSON from PowerShell
After manipulating JSON in PowerShell, you might want to export the data back to JSON format. The `ConvertTo-Json` cmdlet allows you to convert PowerShell objects back into JSON strings.
For instance, you can convert the `$sampleData` object back to JSON as follows:
$jsonOutput = $sampleData | ConvertTo-Json
This will return a JSON representation of the `$sampleData` object.
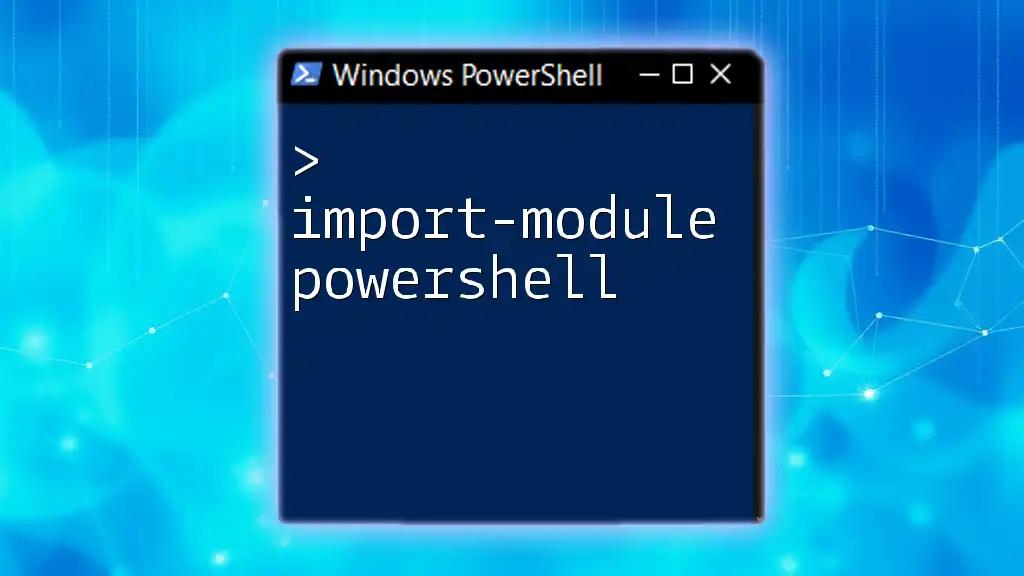
Conclusion
This guide has outlined the fundamental aspects of how to import JSON in PowerShell effectively. From understanding JSON and PowerShell's functionality to importing files, accessing data, and handling errors, you now have the tools necessary to work with JSON data seamlessly.
Engage with the PowerShell community to further your skills, and practice manipulating JSON data to deepen your understanding. With these skills, you're well-equipped to harness the power of PowerShell in managing JSON data.
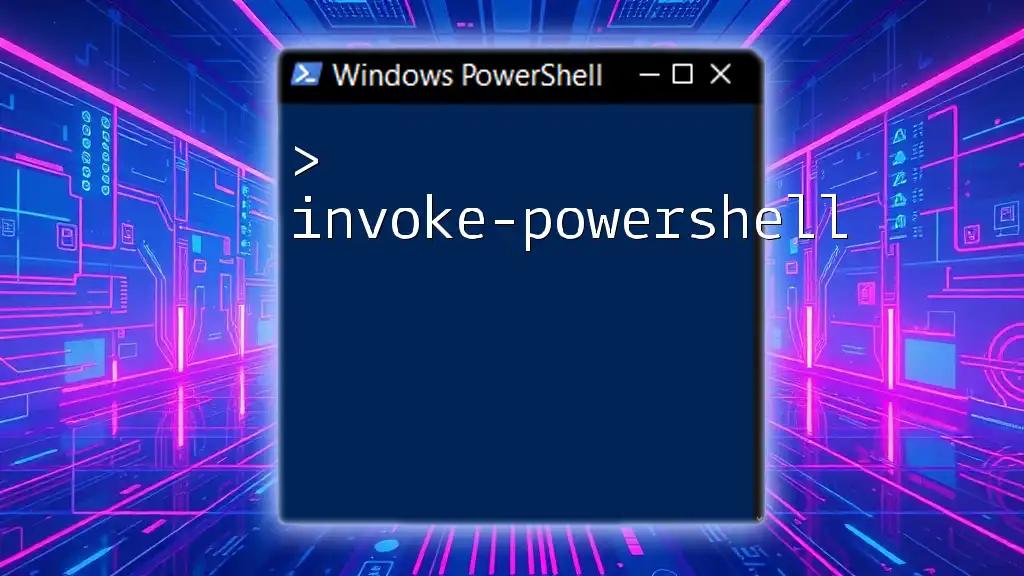
Additional Resources
Useful PowerShell Cmdlets for JSON
- ConvertFrom-Json: Converts JSON-formatted strings into PowerShell objects.
- ConvertTo-Json: Converts PowerShell objects into JSON format for exporting or sharing.
- Invoke-RestMethod: Fetches data from RESTful APIs in JSON format.
Community and Forums
For further learning and community support, consider visiting websites such as PowerShell.org, Reddit’s r/PowerShell, and Stack Overflow, where you can engage with other enthusiasts and experts in the field.
Call to Action
Join our upcoming workshops or sign up for courses focused on PowerShell to enhance your skills further. Take the next step in your professional journey by mastering PowerShell and its capabilities!