The `Get-WmiObject` cmdlet in PowerShell retrieves management information from local and remote computers, allowing users to access various system properties and configurations.
Get-WmiObject -Class Win32_OperatingSystem
Understanding Get-WmiObject
Get-WmiObject is a versatile cmdlet in PowerShell that allows you to interact with Windows Management Instrumentation (WMI) objects. Its basic syntax is straightforward:
Get-WmiObject -Class <WMI_Class> -Namespace <Namespace> -ComputerName <Computer>
In this syntax:
- Class is the WMI class you want to query.
- Namespace is the namespace that contains the class.
- ComputerName specifies the target computer. If omitted, it assumes the local machine.
Key Parameters
-
Class: Choosing the correct WMI class is crucial for accurate data retrieval. The class corresponds to the type of information you want to access, such as processes, services, or BIOS information.
-
Namespace: WMI organizes data into namespaces, similar to folders. The most commonly used namespace is `root\cimv2`, which contains most WMI classes available to end users.
-
Query: You can use WQL (WMI Query Language) to extend the functionality of Get-WmiObject. A basic understanding of WQL will help you retrieve data with precision.
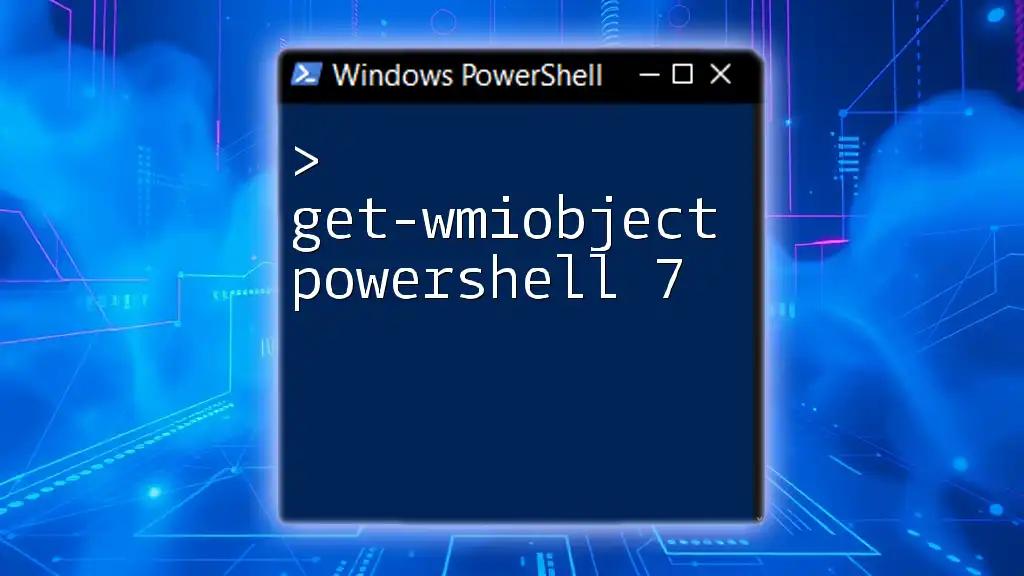
Commonly Used WMI Classes
System Information
Win32_OperatingSystem
The `Win32_OperatingSystem` class is used to retrieve operating system details. Here's how you can invoke it:
Get-WmiObject Win32_OperatingSystem
This command will provide a wealth of information, including the OS name, version, and architecture.
Win32_ComputerSystem
Querying the `Win32_ComputerSystem` class gives insights into computer hardware configurations. For instance:
Get-WmiObject Win32_ComputerSystem
You’ll obtain details about the computer model, manufacturer, and the total number of physical memory.
Networking Information
Win32_NetworkAdapterConfiguration
To gather network adapter settings and configurations, utilize the `Win32_NetworkAdapterConfiguration` class:
Get-WmiObject Win32_NetworkAdapterConfiguration
This query responds with adapter settings like IP addresses, MAC addresses, and description.
Disk Information
Win32_LogicalDisk
If you want to check available disk space, the `Win32_LogicalDisk` class is your go-to. Run the following command:
Get-WmiObject Win32_LogicalDisk
You’ll receive status information on drives, including free and total space.
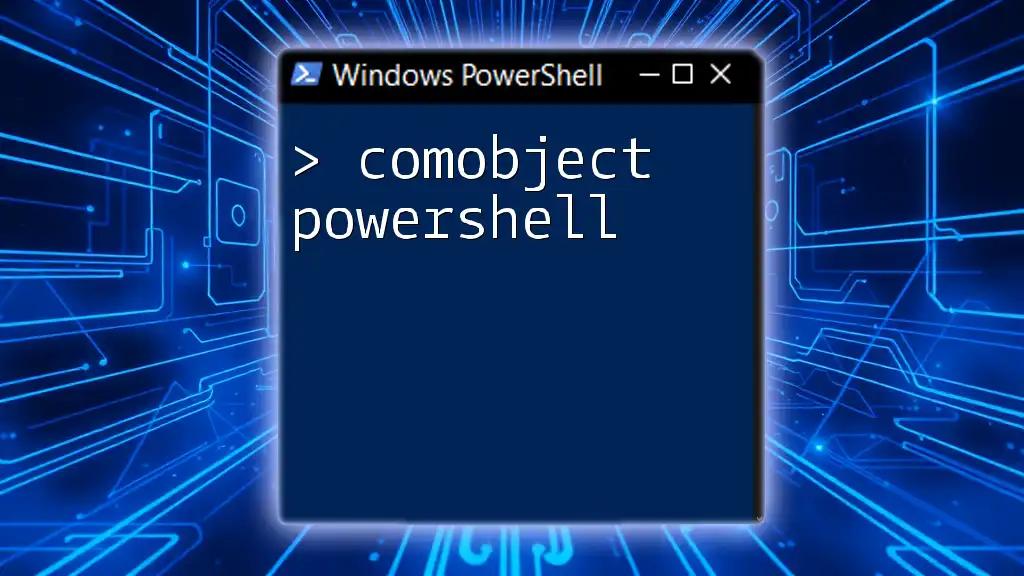
How to Use Get-WmiObject Effectively
Filtering Results
Filtering is crucial when dealing with large datasets. By combining Get-WmiObject with the `Where-Object` cmdlet, you can create powerful filters. For example, if you want to find the process ID of `notepad.exe`, you can use:
Get-WmiObject Win32_Process | Where-Object { $_.Name -eq 'notepad.exe' }
Selecting Specific Properties
Often, you may not need all properties. By employing the `Select-Object` cmdlet, you can narrow down the output. For example:
Get-WmiObject Win32_Process | Select-Object Name, ProcessId
This command focuses exclusively on the name and process ID of running processes.
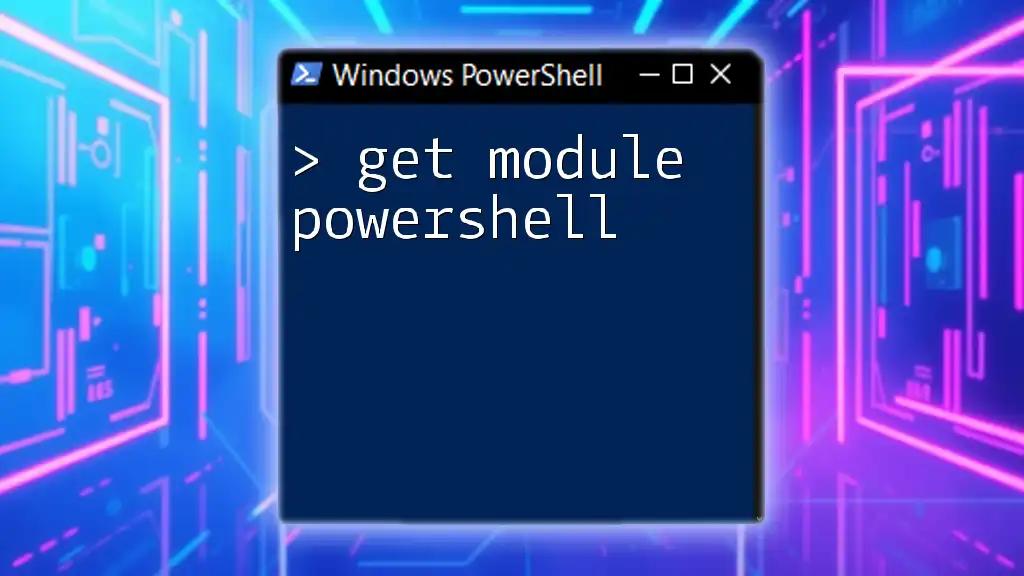
Advanced Usage of Get-WmiObject
Creating Custom Queries
For more intricate data retrieval, writing custom WQL queries provides flexibility. An example would be querying for a specific process:
Get-WmiObject -Query "SELECT * FROM Win32_Process WHERE Name = 'notepad.exe'"
This tailored command isolates the `notepad.exe` process, returning just that instance.
Handling Remote Systems
To query a remote system, you can use the `-ComputerName` parameter. However, ensure the executing user has the necessary permissions:
Get-WmiObject -Class Win32_OperatingSystem -ComputerName 'RemotePC'
This can be invaluable for remote management of multiple systems.
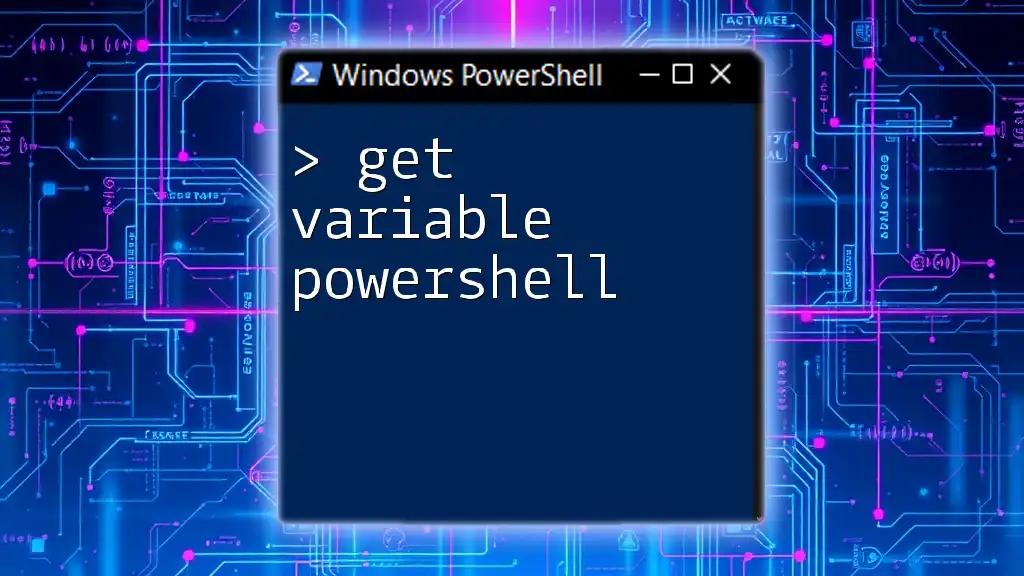
Troubleshooting Common Issues
Permission Errors
Running into permission errors is common. Always check user access rights, especially when executing queries on remote computers.
Timeout and Connectivity Issues
If you experience timeouts or connectivity errors when querying remote systems, ensure that the target system allows WMI requests through its firewall.
Class Not Found Errors
If you encounter issues stating that a WMI class is not found, confirm that you are querying a valid class in the correct namespace using:
Get-WmiObject -Namespace root\cimv2 -List
This command lists all available classes in the `cimv2` namespace.
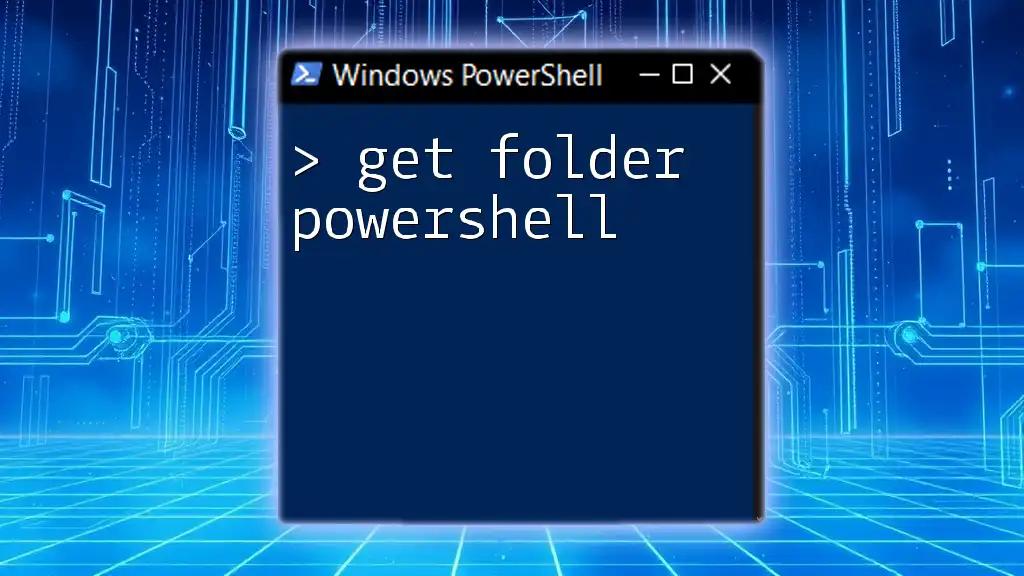
Best Practices
Performance Considerations
Consider using Get-CimInstance in place of Get-WmiObject when dealing with large datasets or requiring specific performance enhancements. CIM (Common Information Model) is a more modern approach and may yield better performance in some situations.
Security Practices
Always adhere to the principle of least privilege when running scripts that involve Get-WmiObject. Limiting permissions can safeguard against potential security risks.
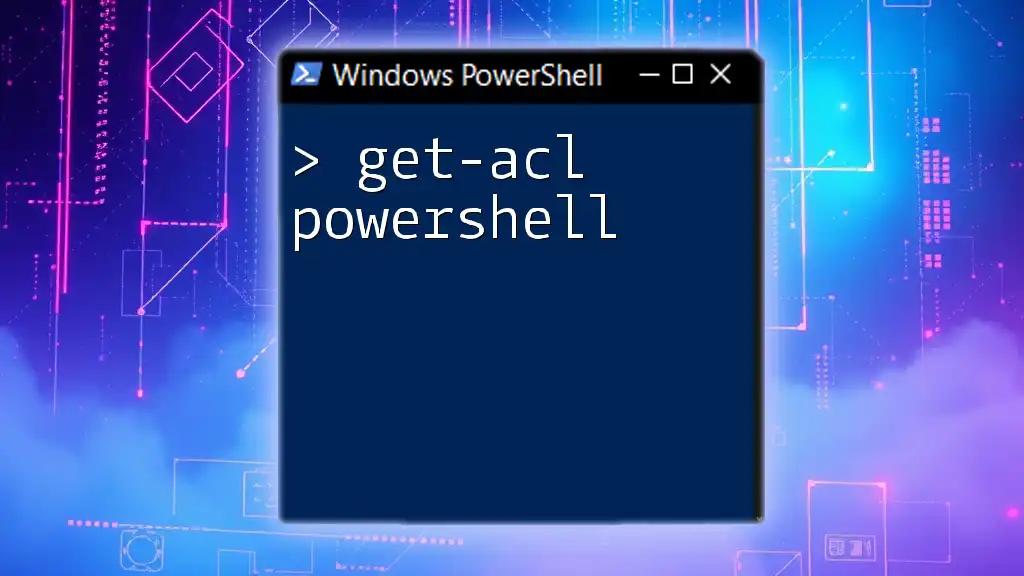
Conclusion
In conclusion, mastering the Get-WmiObject cmdlet is essential for effective PowerShell scripting and system management. By understanding WMI classes and how to query them, you pave the way to automate numerous administrative tasks. Experimenting with the examples provided will enhance your PowerShell skills and make your scripting endeavors more efficient.
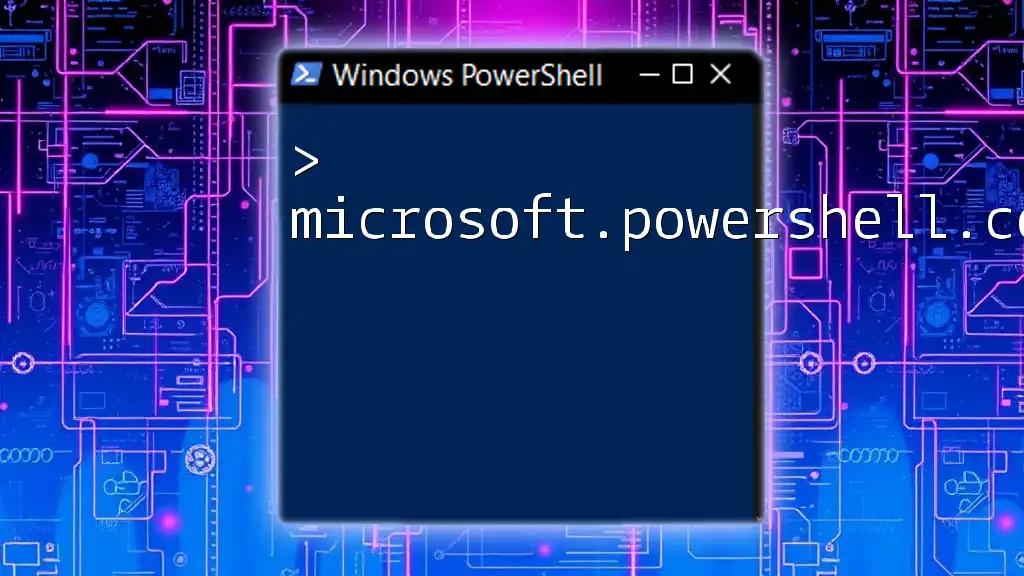
Additional Resources
To further your skills, explore the official Microsoft documentation, recommended books, and engaging community forums. Continuous learning will help you stay abreast of PowerShell developments and best practices in WMI.