Certainly! Here's a concise explanation along with a code snippet:
To convert a simple PowerShell command that outputs 'Hello, World!' into Python, you would use the print function as shown below:
print('Hello, World!')
Understanding PowerShell and Python
What is PowerShell?
PowerShell is a powerful scripting language designed primarily for system administrators. It provides a command-line interface and is built on the .NET framework, making it highly versatile for automation, configuration management, and task automation. PowerShell utilizes cmdlets (command-lets) that are specialized .NET classes, which allow users to perform various operations such as managing systems, interacting with the file system, and querying data.
What is Python?
Python is a high-level, interpreted programming language known for its readability and versatility. Widely adopted in various fields, Python is particularly favored for data analysis, web development, and automation due to its rich ecosystem of libraries and simple syntax. Python emphasizes code clarity, making it easier for developers to express ideas without unnecessary complexity.
Key Differences between PowerShell and Python
When discussing how to convert PowerShell to Python, it’s critical to understand the differences in their syntax and operational paradigms.
- Syntax Differences: PowerShell uses a cmdlet format, while Python employs traditional programming language syntax. For example, PowerShell cmdlets often include verbs and nouns (`Get-Process`), whereas Python uses function names (`psutil.process_iter()`).
- Data Handling: PowerShell excels at object manipulation, outputting .NET objects, while Python utilizes simple data structures like lists and dictionaries, which can make programming in Python more straightforward but less integrated for system tasks.
- Execution: PowerShell operates primarily in a shell environment, while Python code can be executed within various environments, such as scripts or web applications.
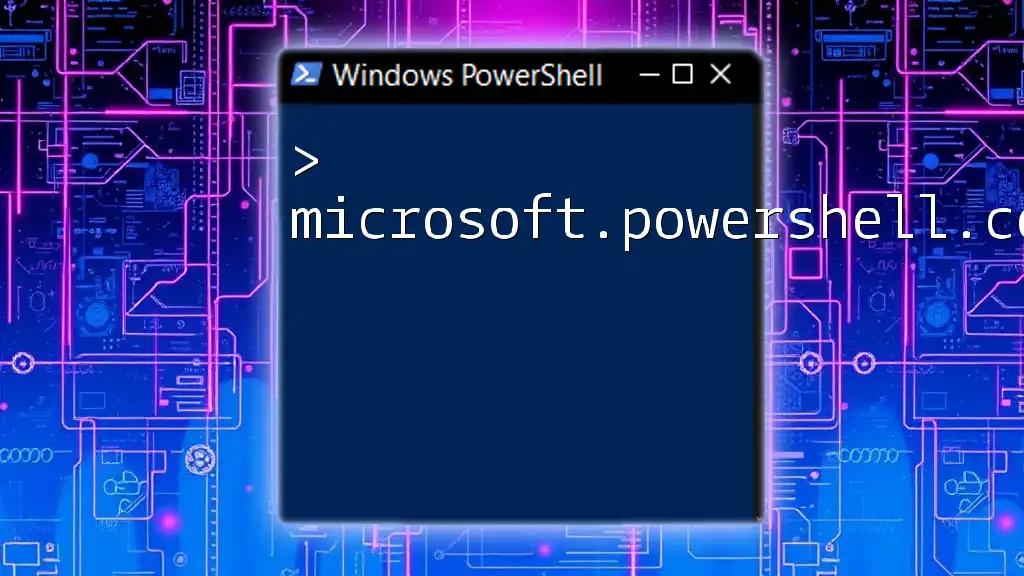
Preparing for the Conversion
Setting Up Your Environment
Before you begin converting PowerShell commands to Python, ensure your environment is ready. Install Python from the official website and choose preferred libraries based on your needs. A popular choice for scripting is the `psutil` library for process management, which aligns closely with PowerShell's capabilities.
Recommended code editors and IDEs include PyCharm and Visual Studio Code, both of which offer excellent support for Python development.
Understanding PowerShell Command Structure
PowerShell commands follow a basic structure that includes the use of cmdlets, parameters, and output types. Understanding this structure is essential for effective conversion.
For example, consider the following simple PowerShell command that retrieves processes using significant CPU resources:
Get-Process | Where-Object {$_.CPU -gt 10}
This command retrieves all processes and filters them based on their CPU usage.
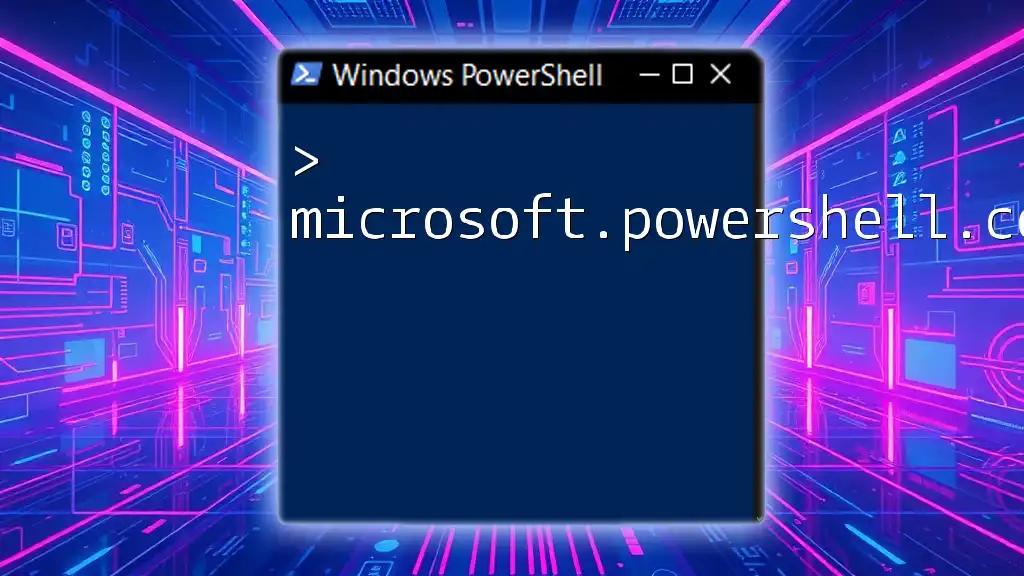
Translating PowerShell Commands to Python
Basic Command Conversion
Variables and Data Types
In PowerShell, you declare a variable with the `$` prefix. For instance:
$process = Get-Process
In Python, this becomes straightforward:
import psutil
process = psutil.process_iter()
Control Structures
Control structures such as `if`, `for`, and `while` are crucial in script execution. Here’s how you can convert an `if` statement from PowerShell to Python.
PowerShell example:
if ($CPU -gt 10) { "High CPU Usage" }
Python version:
if CPU > 10:
print("High CPU Usage")
Working with Arrays and Collections
Arrays in PowerShell can be compared to lists in Python, with both being used for storing collections of items. For example, in PowerShell:
$services = Get-Service
The equivalent in Python would likely use a subprocess to query services:
import subprocess
services = subprocess.check_output(["sc", "query"]).splitlines()
Functions and Exception Handling
Defining functions has a different syntax in each language. Here’s how you can convert a simple function:
PowerShell:
function Get-MyData { return "Some Data" }
Python:
def get_my_data():
return "Some Data"
Exception handling also differs. In PowerShell, it might look like this:
try { Get-Process } catch { "Error" }
In Python, you'd write:
try:
process = psutil.process_iter()
except Exception as e:
print("Error", e)
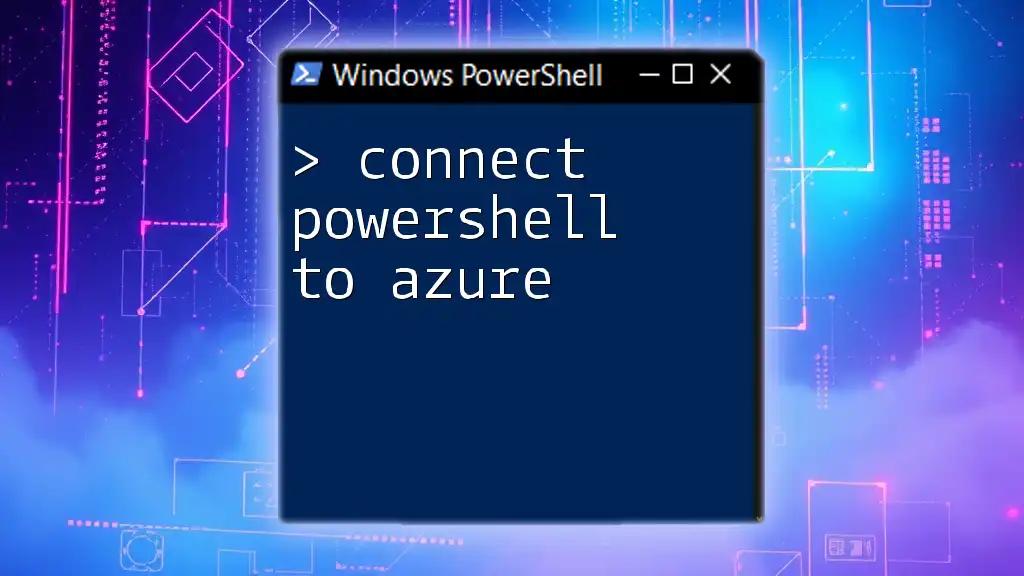
Advanced Conversion Techniques
Working with APIs
Exciting capabilities arise when using PowerShell and Python to interact with web APIs. In PowerShell, a simple API call might look like this:
Invoke-RestMethod -Uri 'https://api.example.com/data' -Method Get
In Python, you can use the highly regarded `requests` library:
import requests
response = requests.get('https://api.example.com/data')
File and Directory Operations
File handling also contrasts across PowerShell and Python. For instance, reading a file in PowerShell is done with:
Get-Content "example.txt"
In Python, you would use:
with open("example.txt") as f:
content = f.readlines()
Scheduling and Automation
Scheduling tasks in PowerShell often utilizes the Task Scheduler, while Python can achieve similar results with the `schedule` library. An example in PowerShell might look like this:
New-ScheduledTask -Action (New-ScheduledTaskAction -Execute "MyScript.ps1") -Trigger (New-ScheduledTaskTrigger -At 12am)
In Python:
import schedule
import time
def job():
print("Job is running")
schedule.every().day.at("00:00").do(job)
while True:
schedule.run_pending()
time.sleep(1)

Best Practices for Conversion
Code Readability
When you convert PowerShell to Python, focus on crafting clear, maintainable code. Python’s philosophy directs developers to value readability. Utilize meaningful variable names and appropriate commenting to enhance understanding.
Performance Considerations
Consider the trade-offs involved when transitioning from PowerShell to Python. While Python can be more flexible, PowerShell is often optimized for direct system interaction. Understand your project requirements to make informed decisions about which tool to employ.

Conclusion
Converting PowerShell commands to Python can greatly expand your toolkit, allowing you to leverage the strengths of both languages. Practice by selecting a PowerShell script and attempting an equivalent conversion in Python. Dive deeper into both environments to better understand their capabilities and best practices.
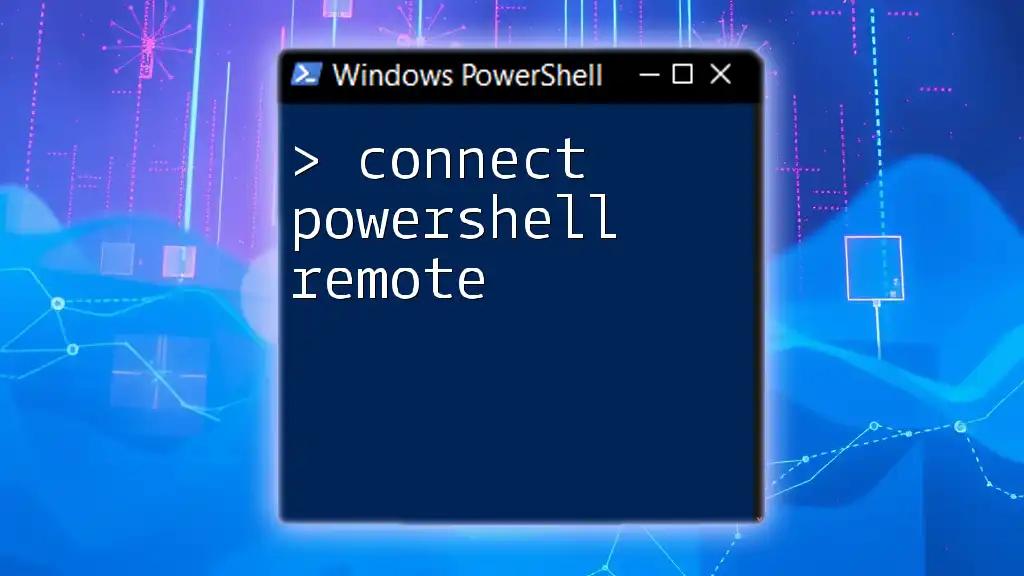
Additional Resources
Continue your learning journey by exploring recommended books and websites dedicated to PowerShell and Python. Online communities, such as forums and discussion groups, also provide valuable support from fellow enthusiasts.
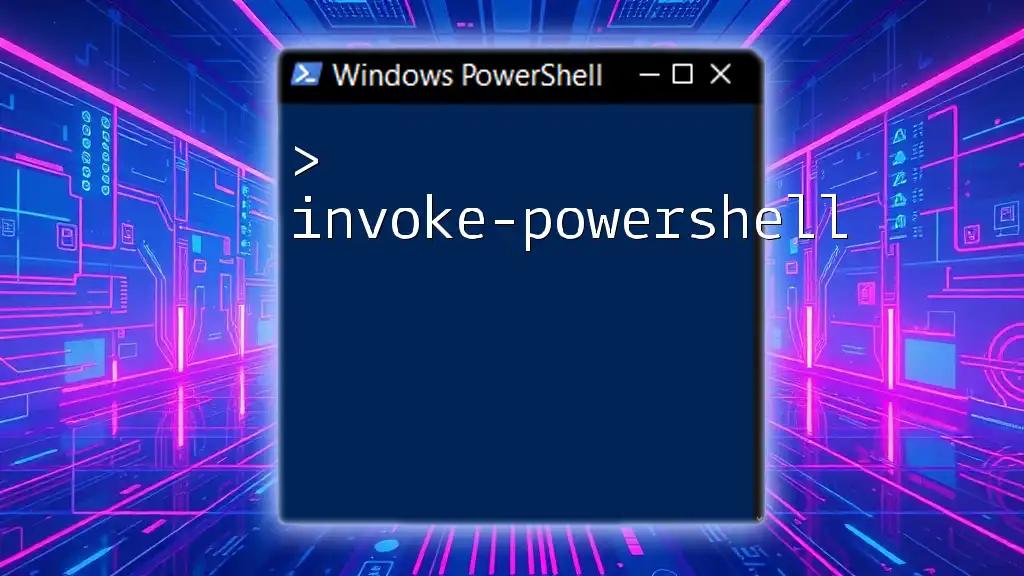
FAQ
-
What tools can I use to convert PowerShell scripts to Python?
You can manually translate the commands utilizing the insights from this guide or use automated tools, though manual conversions often provide better understanding and customization. -
Is Python better suited for automation compared to PowerShell?
It depends on the context. For cross-platform automation, Python might be more advantageous, while PowerShell is tailored for system automation within Windows environments. -
What are the common pitfalls to avoid when converting commands?
Watch for syntax differences and consider how each language handles data types, as these can lead to errors if overlooked.