In PowerShell, the `goto` statement allows you to jump to a specific label in your script, enabling non-linear control flow.
Here's a simple example:
goto Label1
Write-Host 'This will be skipped.'
:Label1
Write-Host 'You have jumped to Label1!'
Understanding PowerShell Goto
What is Goto in PowerShell?
The `goto` statement is a programming mechanism used to jump to different points within a script. It enables control over the flow of execution, allowing the script to move from one labeled section to another. In PowerShell, `goto` can be particularly useful for looping back or skipping sections of code based on certain conditions.
Syntax of Goto in PowerShell
The syntax for using `goto` is straightforward:
goto :label
In this context, `label` refers to a specific tag within your script. Labels must begin with a colon and represent the target point where the script execution should jump.

Key Concepts of PowerShell Goto
The Goto Label
A goto label acts as a marker in your script, indicating where the execution flow should continue upon calling it. You create a label by simply prefixing the label name with a colon:
:labelName
For example, to create a simple label that sends the flow to a section of code, consider the following:
:start
Write-Host 'This is the start label.'
When you use the `goto` statement, you can jump to this label, allowing you to control where the script execution continues.
Navigating with Goto
Why Use Goto? The `goto` statement can be beneficial in scenarios where you need to repeatedly execute certain sections or where you need a quick way to skip entire areas of code, especially in larger scripts.
Flow Control is crucial for scripting, and `goto` can introduce flexibility. However, moderation is key, as excessive use can lead to confusion.
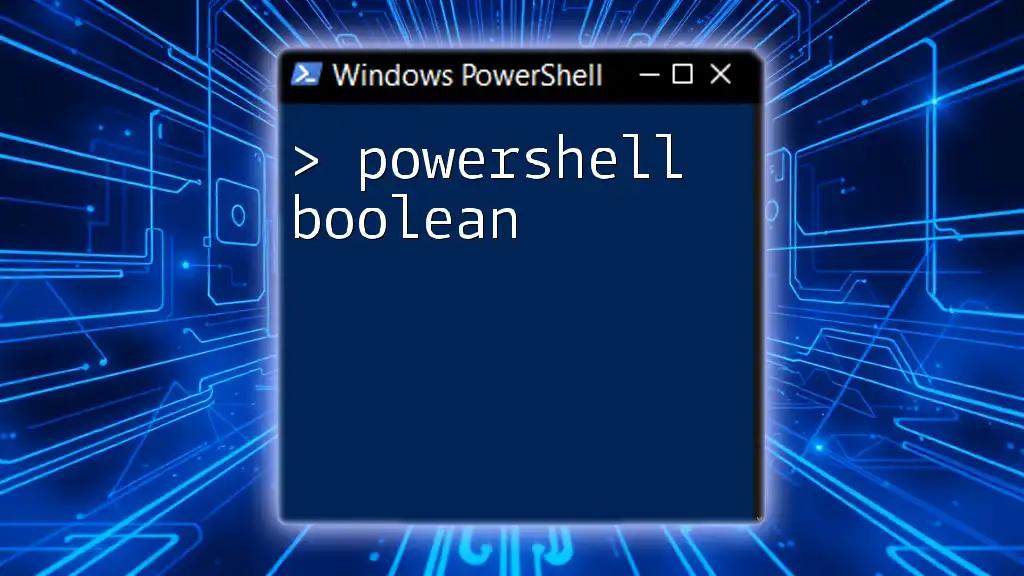
Practical Examples of Goto in PowerShell
Basic Example of Goto in Action
Here's a simple script utilizing `goto` to demonstrate its functioning clearly:
:start
Write-Host "Welcome! Do you want to continue? (y/n)"
$response = Read-Host
if ($response -eq 'y') {
# Proceed with script
Write-Host "Continuing..."
} else {
goto :end
}
:end
Write-Host "Exiting..."
In this example, the script prompts the user for a response. If the user enters y, the script continues to execute; if the response is anything else, it jumps to the :end label.
Advanced Goto Usage
Using Goto for Error Handling can simplify scripts, especially when dealing with complex logic or multiple potential error states. An example might look like this:
:start
try {
# Code that may generate an error
Get-Content "fileThatMayNotExist.txt"
} catch {
Write-Host "An error occurred. Jumping to the error handler."
goto :errorHandler
}
:normalFlow
Write-Host "Code that runs successfully."
:errorHandler
Write-Host "Handling error now..."
In this script, if the `Get-Content` command fails, the execution jumps directly to the `:errorHandler` label, thereby isolating error handling from the normal flow of operations.
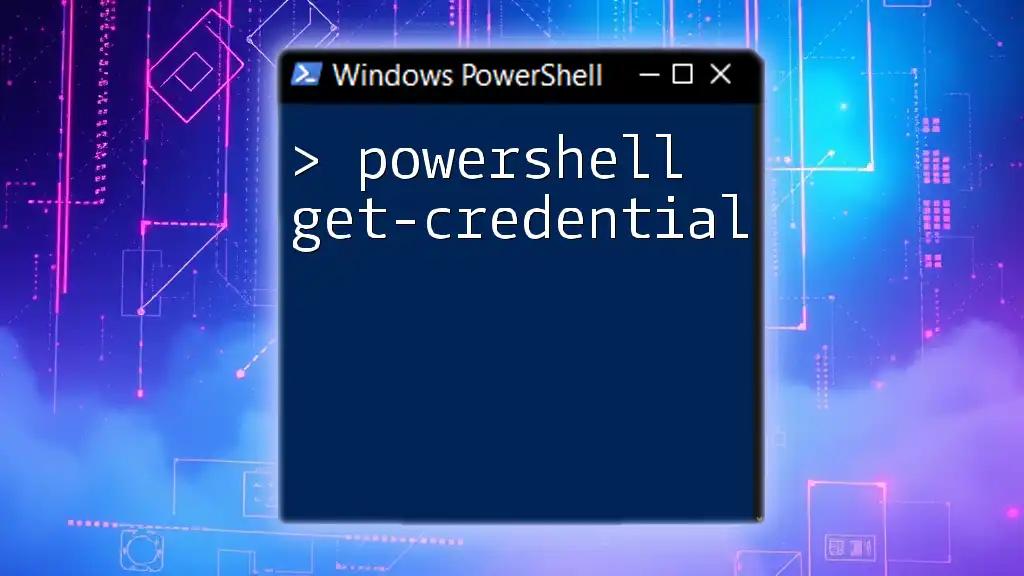
Best Practices for Using Goto
When to Avoid Goto
While `goto` has its advantages, it can lead to lack of readability if overused. Scripts can quickly become jumbled, making it hard to trace the program's flow. Instead of relying solely on `goto`, consider using loops (`for`, `while`) or functions to maintain cleaner code. These structures enhance clarity and maintainability.
Tips for Effective Use
- Keep It Simple: When you do use `goto`, limit its scope. Stick to simple, essential jumps rather than complex pathways.
- Documentation: Commenting your code is vital when incorporating `goto`. Clear explanations of why you are utilizing jumps can prevent confusion for yourself and others who might read your code later.
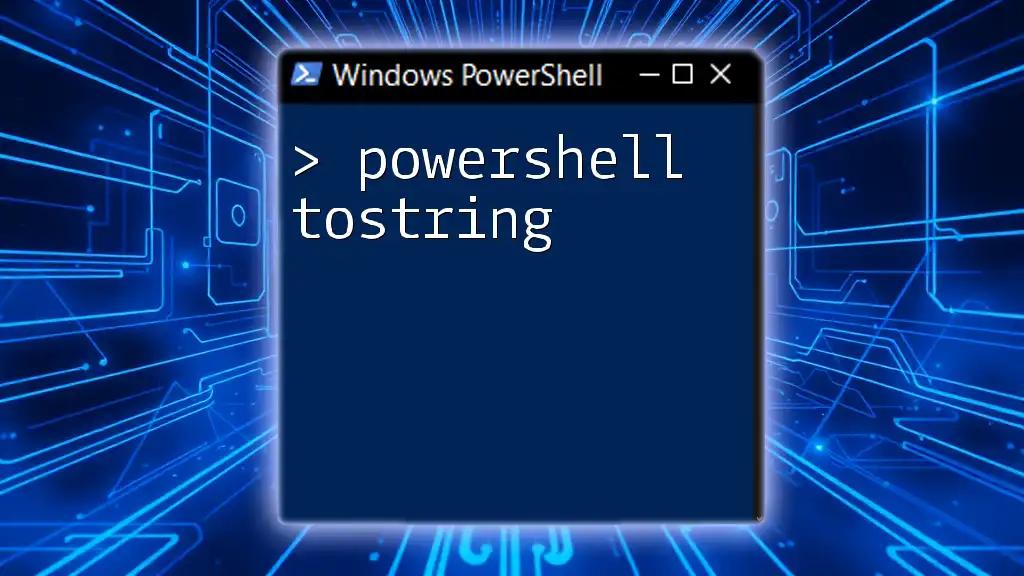
Conclusion
Summary of Key Points
In summary, the `goto` statement in PowerShell gives you a unique way of manipulating the flow of your script. Its utility ranges from basic task repetition to complex error handling, but it requires careful consideration to maintain readability.
Final Thoughts
As you experiment with `goto`, remember to use it judiciously. Strive for clear, logical scripts that maximize the potential of PowerShell while avoiding the pitfalls of "spaghetti code."
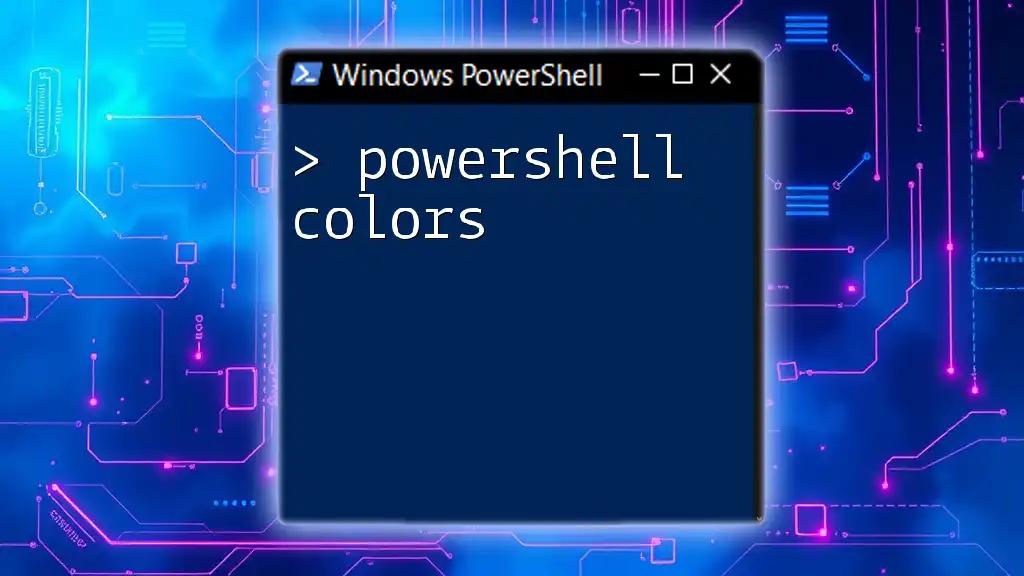
Further Reading
Resources
To deepen your understanding of PowerShell commands and best practices, consider diving into established PowerShell documentation or engaging with community forums where script writing and optimization techniques are frequently shared. These resources can help enhance your coding skills and familiarity with PowerShell characteristics beyond `goto`.