To stop a PowerShell script that is currently running, you can use the `CTRL + C` keyboard shortcut, but if you want to programmatically exit the script, you can use the `Exit` command.
Here’s a code snippet demonstrating how to exit a PowerShell script:
Write-Host 'Stopping the script...'
Exit
Understanding PowerShell Script Execution
What Happens During Script Execution
When a PowerShell script runs, it processes a series of commands sequentially. PowerShell reads each line of code, executes it and moves on to the next. The execution environment is governed by the Windows Execution Policy, which can restrict certain commands based on the script's provenance (i.e., whether it’s signed, downloaded from the internet, etc.). This policy can impact the ability to stop or control scripts, so being familiar with it is critical.
Common Reasons for Stopping a Script
There are several situations where you might need to stop a PowerShell script:
-
Infinite Loops: A common programming mistake where logic mistakenly allows the script to run indefinitely.
-
Long-Running Processes: Scripts that perform extensive operations might need to be stopped if they are consuming too many resources or taking too long.
-
Debugging and Testing Scenarios: During development, you may want to halt a script to troubleshoot or to make adjustments on the fly.
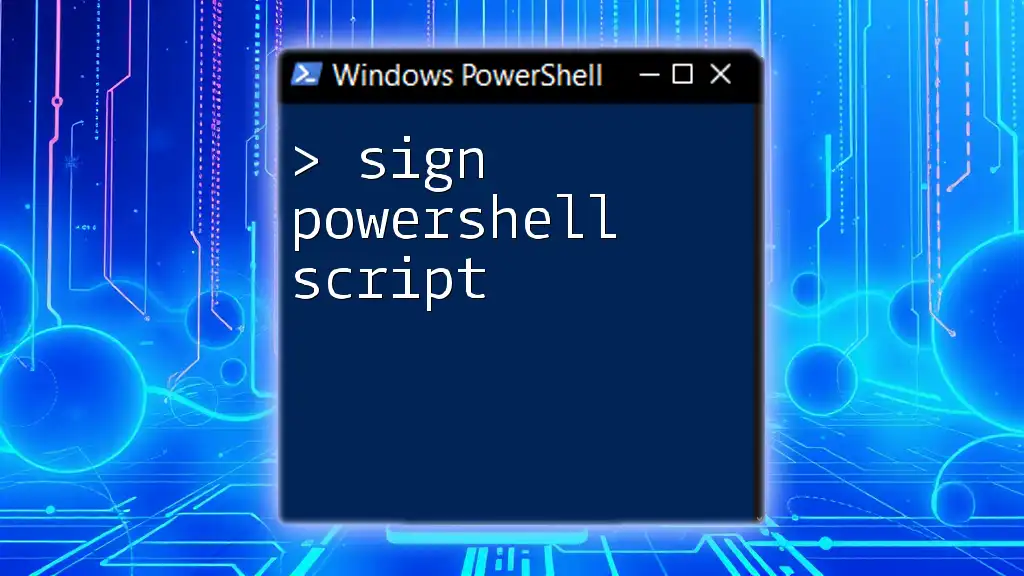
How to Stop a PowerShell Script
Using Keyboard Shortcuts
One of the simplest ways to stop a PowerShell script that is currently running is to use the keyboard shortcut Ctrl + C. This sends an interrupt signal to the script, terminating its execution immediately.
Example: Infinite Loop
Consider the following script, which will run indefinitely unless stopped:
while ($true) {
"Running indefinitely..."
}
If you execute the above script, press Ctrl + C to break out of the loop, terminating the script.
Using the Stop-Process Cmdlet
For scripts running as separate processes, the `Stop-Process` cmdlet is your friend. This cmdlet allows you to terminate a process by name or ID.
Example of Using Stop-Process
Below is a code snippet demonstrating how to identify a script's process and kill it:
$process = Get-Process -Name YourScriptName
Stop-Process -Id $process.Id
In this snippet, replace `YourScriptName` with the actual name of your PowerShell script's process. This method is effective when the script runs in a separate PowerShell instance.
Breaking Script Execution with Conditional Statements
Using conditional statements can also help to stop PowerShell scripts gracefully. Setting up exit conditions enables you to control the flow of the script and terminate it gently.
Example of Conditional Exit
Here’s a snippet demonstrating how you can implement a break condition:
$shouldStop = $false
while (-not $shouldStop) {
"Running script..."
if (<some condition>) {
$shouldStop = $true
}
}
In the above code, replace `<some condition>` with a valid condition based on your requirements. When the condition becomes true, `$shouldStop` changes to true, and the loop exits.
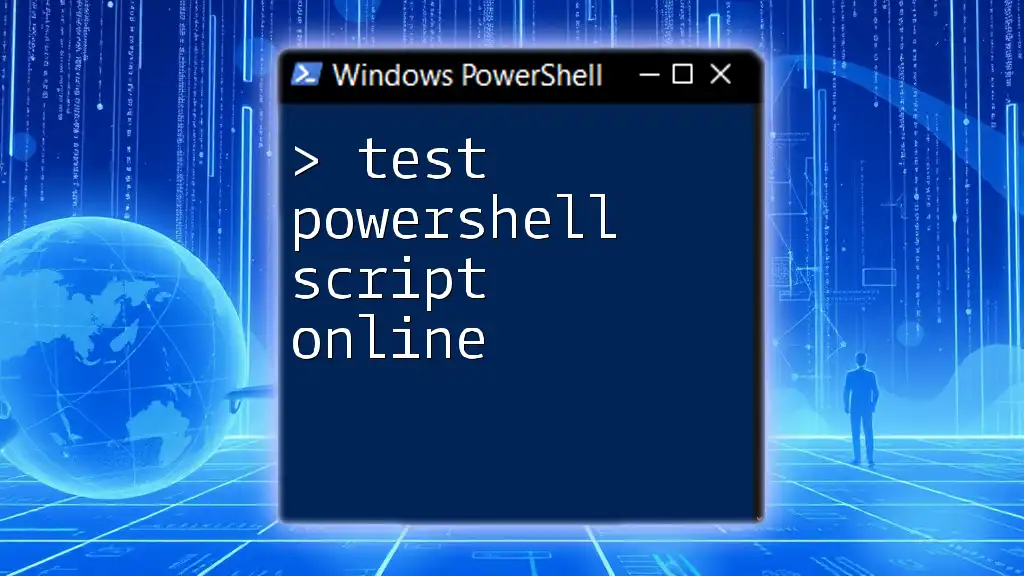
PowerShell Stop Script Techniques
Graceful vs. Forceful Stopping
Understanding the difference between graceful and forceful stopping is crucial when working with PowerShell scripts. Graceful stopping involves allowing the script to finish its current task and exit cleanly. On the other hand, forceful stopping abruptly stops the script execution without cleanup, which may lead to partial operations or resource leaks.
Use `Stop-Process` for immediate termination when necessary, but prefer graceful methods like setting conditional exits when possible.
Use of Try-Catch for Error Handling
Implementing try-catch blocks can help manage unexpected stops effectively. By wrapping sections of your script in a try-catch statement, you ensure that errors do not crash your script entirely.
Example of Try-Catch Implementation
Here’s how you can implement error handling in your script:
try {
# Code that might fail
# Example: Invoke-WebRequest for a URL that may not exist
} catch {
# Cleanup code or notification
Write-Host "An error occurred: $_"
}
In this code, if an error occurs within the try block, control shifts to the catch block, allowing you to handle the error gracefully.
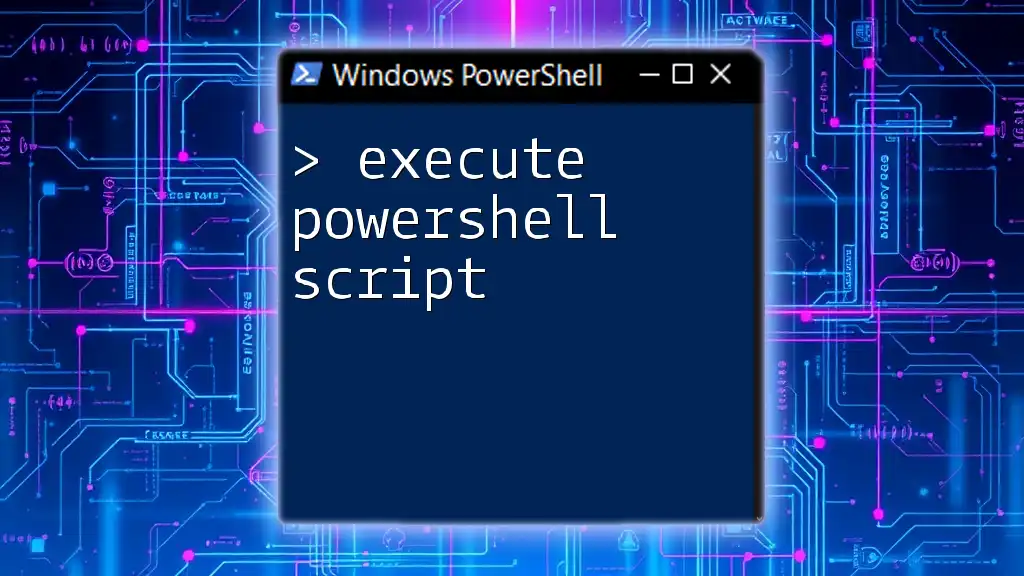
Best Practices for Stopping PowerShell Scripts
Logging and Notification
It’s a good practice to implement logging within your scripts. Logging keeps track of execution states and can provide context if a script needs to be stopped. Consider using `Write-Host` or `Add-Content` cmdlets to log messages and errors.
Script Limitations and Timeouts
Setting time limits can prevent scripts from running longer than expected. Implementing timeouts can be simple and effective in stopping excessive resource consumption.
Example of Timeout Implementation
Below is a code snippet demonstrating how to stop a script after a specific duration:
Start-Sleep -Seconds 10
throw "Script timed out!"
In this example, if the script exceeds the allotted time, it throws an exception, effectively signaling that it needs to stop.
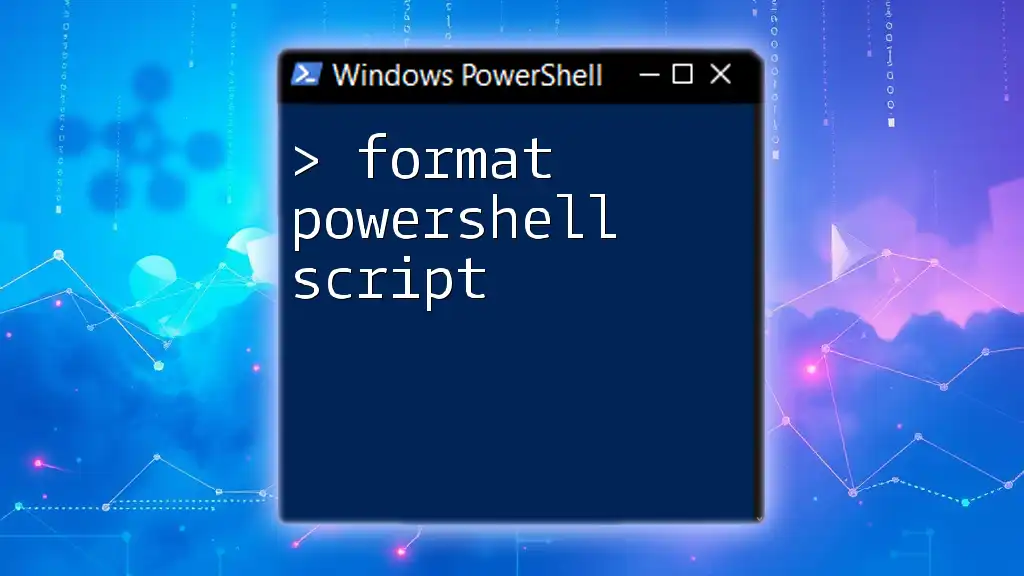
Conclusion
In managing PowerShell scripts, knowing how to stop a PowerShell script effectively is essential for developers and system administrators alike. From utilizing basic keyboard shortcuts and cmdlets to implementing graceful exits and comprehensive error handling, these techniques not only improve control over script execution but also promote an efficient scripting environment. By applying the best practices outlined, you can ensure that your PowerShell scripts run smoothly and reliably, even when faced with the need to halt execution.