To execute a PowerShell script from C#, you can use the `System.Diagnostics.Process` class to start a new PowerShell process and run your script efficiently.
Here's a code snippet in C#:
using System.Diagnostics;
class Program
{
static void Main()
{
Process process = new Process();
process.StartInfo.FileName = "powershell.exe";
process.StartInfo.Arguments = "-File \"C:\\path\\to\\your\\script.ps1\"";
process.StartInfo.UseShellExecute = false;
process.StartInfo.RedirectStandardOutput = true;
process.Start();
string output = process.StandardOutput.ReadToEnd();
process.WaitForExit();
Console.WriteLine(output);
}
}
Understanding PowerShell
What is PowerShell?
PowerShell is a powerful task automation and configuration management framework developed by Microsoft. It consists of a command-line shell and a scripting language built on the .NET framework. Unlike traditional shell languages, PowerShell enables users to work seamlessly with objects, making it an essential tool for system administrators and developers alike.
One of the most significant advantages of using PowerShell is its ability to automate repetitive tasks and streamline workflows. With its extensive libraries of cmdlets and support for custom scripting, PowerShell provides adaptability that empowers users to accomplish a wide range of tasks from simple file manipulations to complex system configurations.
When to Use PowerShell from C#
Integrating PowerShell with C# can be particularly beneficial in various scenarios. This capability not only enhances the functionality of applications but also allows for complex operations without reinventing the wheel for common tasks.
Some scenarios where executing PowerShell scripts from C# can be advantageous include:
- System Administration: Automate system diagnostics, manage services, and fetch system information using familiar PowerShell commands.
- Application Automation: Trigger automated tasks within applications that rely on PowerShell, such as deployments or updates.
- Reporting: Generate system reports or logs in user-friendly formats by executing PowerShell commands directly from your C# application.
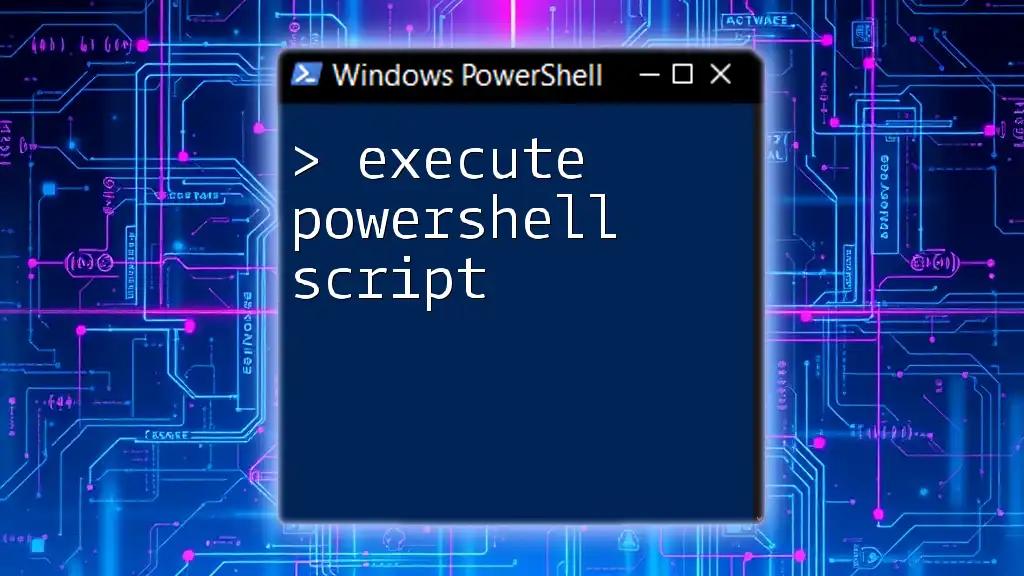
Setting Up Your Development Environment
Necessary Software
To execute PowerShell scripts from C#, you will need to have the following installed:
- Visual Studio: The IDE for building and testing your C# applications.
- .NET Framework: Required to run C# applications.
- PowerShell: Usually pre-installed, but check for the latest version to ensure compatibility.
Ensure that you have all the necessary components installed and configured correctly before proceeding.
Creating a New C# Project
Start by creating a new C# console application in Visual Studio:
- Open Visual Studio and select "Create a new project."
- Choose "Console App" under C# applications.
- Name your project and select the desired location.
- Click "Create" to initialize your project.
Once your new console application is created, you can add references for utilizing PowerShell commands effectively.
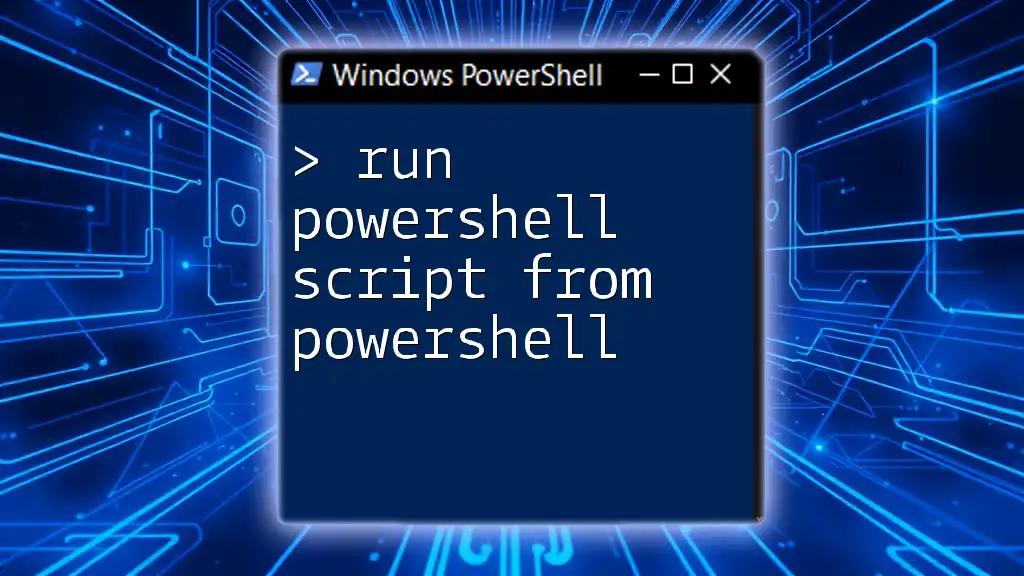
Executing PowerShell Scripts from C#
Using the `System.Management.Automation` Namespace
Overview of `System.Management.Automation`
The `System.Management.Automation` namespace is essential for creating and executing PowerShell commands within C#. This namespace provides types that allow you to run PowerShell scripts, manage sessions, and access advanced features of PowerShell.
Example Code Snippet
To get started with executing PowerShell commands, you can use the following code snippet:
using System;
using System.Management.Automation;
class Program
{
static void Main()
{
using (PowerShell powerShell = PowerShell.Create())
{
powerShell.AddScript("Get-Process");
foreach (var result in powerShell.Invoke())
{
Console.WriteLine(result);
}
}
}
}
In this example, the code initializes a PowerShell instance and executes the `Get-Process` command. The results are then printed to the console, providing a straightforward way to retrieve and display currently running processes.
Running PowerShell Scripts from File
How to Reference a Script File
If you want to execute a PowerShell script that is stored in a file, you will need to read the script contents and execute it. Ensure that the script is stored securely and has the correct execution permissions.
Example Code Snippet
Here's how to reference a script file and execute it:
string scriptPath = @"C:\Scripts\MyScript.ps1";
using (PowerShell powerShell = PowerShell.Create())
{
powerShell.AddScript(System.IO.File.ReadAllText(scriptPath));
var results = powerShell.Invoke();
// Handle results
}
In this example, the script located at `C:\Scripts\MyScript.ps1` is read into the PowerShell instance and executed. This method allows for the seamless execution of complex scripts without hardcoding commands directly into your C# application.

Handling Errors
Catching Exceptions
When executing PowerShell scripts from C#, it is crucial to handle potential errors. Various factors, such as script bugs or permission issues, can lead to exceptions during execution.
To catch these exceptions, you can encapsulate your code in a try-catch block:
try
{
powerShell.Invoke();
}
catch (Exception ex)
{
Console.WriteLine($"An error occurred: {ex.Message}");
}
This error handling pattern ensures that any issues during script execution can be gracefully caught and reported, preventing your application from crashing unexpectedly.
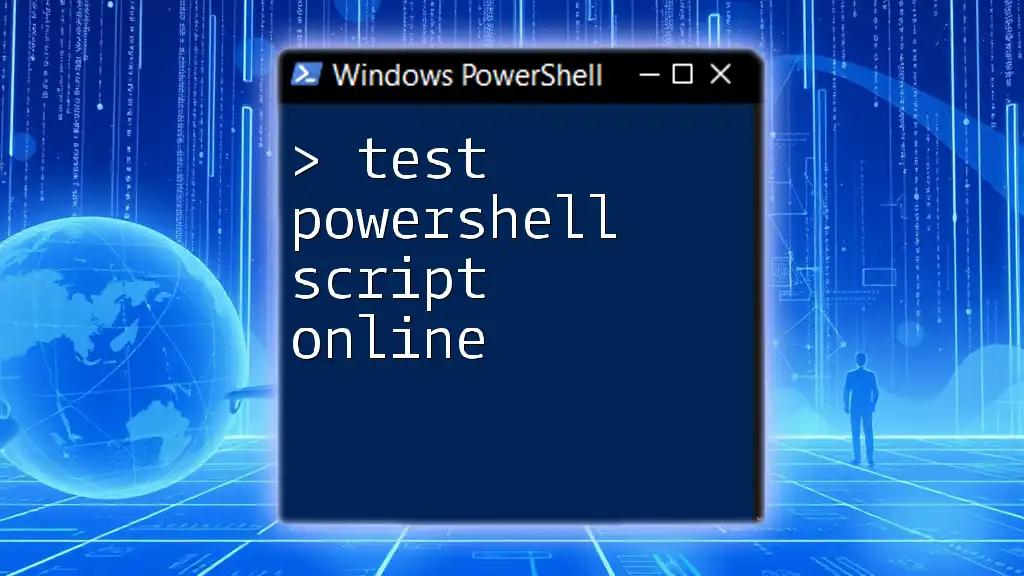
Best Practices for Integrating PowerShell with C#
Performance Considerations
While integrating PowerShell into C# applications is powerful, performance considerations should not be ignored. Every time you invoke a PowerShell command, it involves inter-process communication, which can impact performance.
To optimize your PowerShell execution:
- Batch Commands: Instead of executing multiple single commands, batch them into a single script whenever possible to minimize overhead.
- Reduce Data Transmissions: Limit the amount of data being passed back and forth between PowerShell and C#.
Security Concerns
When executing PowerShell scripts from C#, security is a paramount concern. Running scripts with elevated permissions can expose the host system to vulnerabilities.
To ensure secure PowerShell execution:
- Set Execution Policies: Configure PowerShell execution policies to prevent unauthorized script execution.
- Use Script Signing: Sign your scripts to verify their integrity and authenticity, enhancing security during execution.

Conclusion
In summary, understanding how to execute PowerShell scripts from C# opens up a myriad of possibilities for automation and streamlined workflows. By leveraging PowerShell's powerful capabilities alongside C#, developers can efficiently handle system administration, application automation, and reporting tasks.
As you practice these techniques and refine your skills, consider joining resources and communities that focus on C# and PowerShell to enhance your learning journey. Embrace the power of integration and start automating today!
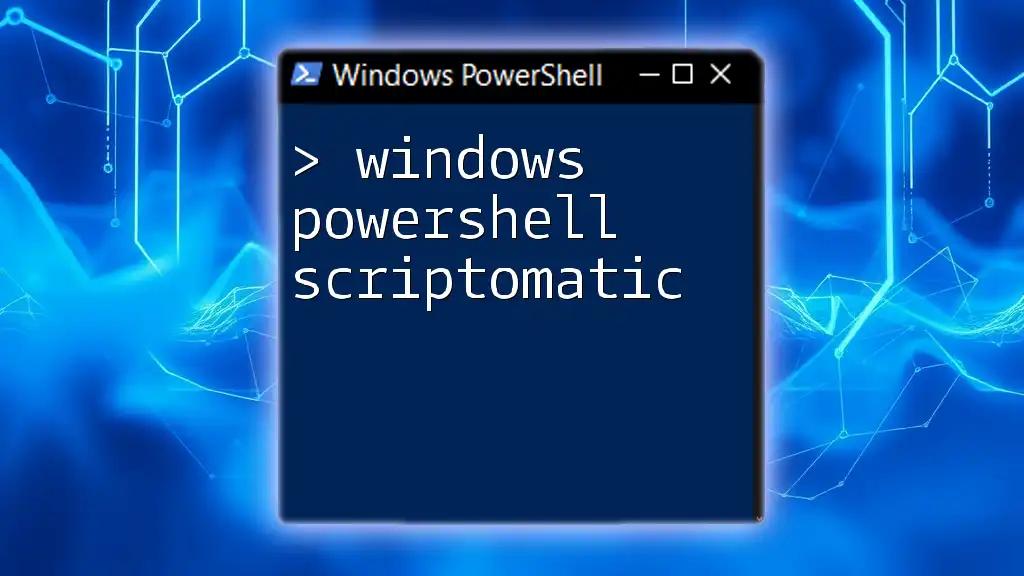
Additional Resources
For further learning, explore the official PowerShell documentation, recommended books, online courses, and community forums dedicated to both C# and PowerShell enthusiasts. These resources can provide deeper insights and help you stay updated on best practices and emerging trends in scripting and automation.