Formatting a PowerShell script involves structuring the code in a clear and organized manner, which enhances readability and maintainability.
Here's an example of a well-formatted PowerShell script:
# This script displays a greeting message
Write-Host 'Hello, World!'
Understanding PowerShell Script Structure
Key Components of a PowerShell Script
When writing a script, it's crucial to understand its main components. A typical PowerShell script will include:
-
Comments: Comments are essential for detailing the purpose and functionality of your script, making it easier for you and others to understand it in the future. Using comments effectively can significantly enhance the readability of your script.
-
Variables: PowerShell utilizes variables to store data temporarily. It's advisable to use meaningful names that clearly indicate the variable's purpose. For instance, `$userName` is better than `$u`.
-
Commands and Cmdlets: PowerShell operates primarily through commands and cmdlets. Understanding how to use these properly will help you structure your scripts.

Formatting Techniques for PowerShell Scripts
Indentation and Spacing
Proper indentation plays a significant role in the readability of your script. It visually represents the structure, making it easier to follow nested conditions and loops. Consistent use of indentation helps prevent errors and facilitates quicker debugging.
Here’s an example demonstrating indentation:
if ($condition) {
Write-Output "Condition is True"
} else {
Write-Output "Condition is False"
}
Best practices suggest using either spaces or tabs consistently throughout your script, though spaces are generally preferred in multi-team environments.
Line Length and Breaks
Keeping your lines concise is essential for readability. Aim for a maximum line length of around 80-120 characters. If a command is long, consider breaking it across multiple lines. You can use the backtick (`) for line continuation or organize your commands within parentheses.
Here's how to wrap long commands effectively:
$result = Get-Process | Where-Object { $_.CPU -gt 100 } `
| Select-Object Name, CPU
Organizing your code this way helps maintain clarity and reduces the chances of scrolling problems in your editor.
Using White Space Effectively
Effective use of white space helps group related commands together. This creates visual separation and aids in understanding the script's functionality at a glance. For example:
# Get System Information
Get-ComputerInfo
# Get Network Configuration
Get-NetIPAddress
Inserting blank lines between logical sections allows readability to flourish, making it much easier for others (or your future self) to navigate through your script.
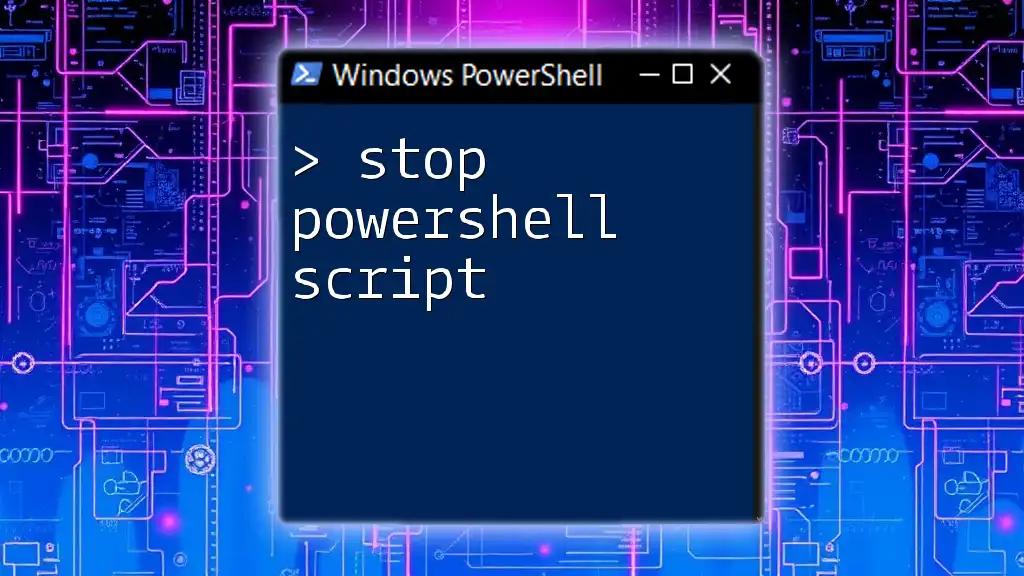
Commenting Your Code
Importance of Comments
Comments serve as communication tools in your script. They clarify the intent behind the code and explain complex logic, which is invaluable as scripts grow in size and complexity.
Types of Comments
PowerShell supports both single-line and multi-line comments.
Single-line comments begin with a `#`, while multi-line comments are encapsulated within `<#` and `#>`. Here’s how you might use each:
Single-line comment:
# This retrieves the current user
Get-User
Multi-line comment:
<#
This section handles data retrieval
from the database.
#>
Techniques for Effective Comments
Utilize comments to document complex logic or to remind yourself of items that need future attention. For example, you can tag sections of the code with TODO or FIXME to highlight work that remains outstanding:
# TODO: Optimize this function for better performance
Get-Data | Where-Object { $_.Status -eq "Active" }
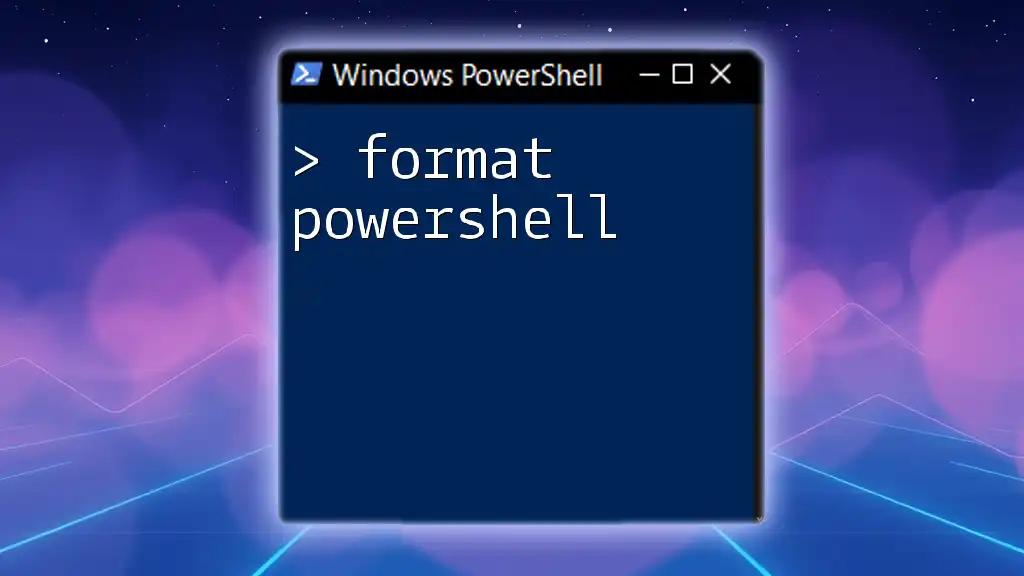
Consistency in Naming and Casing
Variables and Function Naming Conventions
Adhering to consistent naming conventions for variables and functions is vital for clear communication through your code. Choose a style—either CamelCase or snake_case—and stick with it throughout your scripts.
For example:
$userAge = 25 # CamelCase
$user_age = 25 # snake_case
Cmdlet and Function Capitalization
When creating functions, follow the Verb-Noun format. This approach increases the clarity of the script's purpose. Using capitalization for both verbs and nouns also enhances readability:
function Get-UserData {
# Function logic here
}
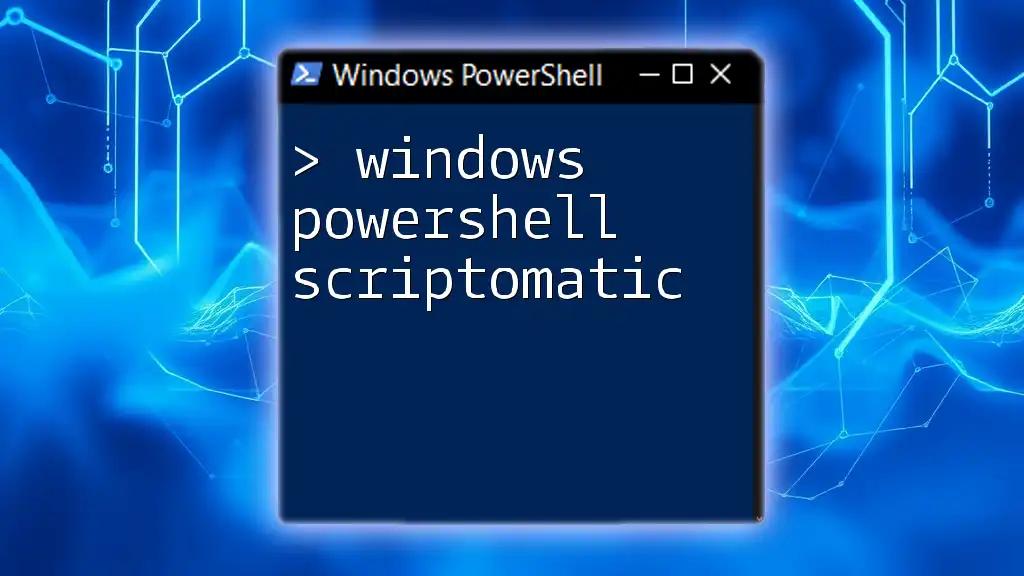
Using the PowerShell ISE and Other Tools
Benefits of PowerShell ISE
The PowerShell Integrated Scripting Environment (ISE) provides a built-in editor that supports syntax highlighting, snippet insertion, and execution testing. These features facilitate script formatting and help you spot errors immediately.
Third-party Tools and Editors
Various third-party tools, such as Visual Studio Code, offer robust support for PowerShell scripting. Extensions like `Prettier` and `PSScriptAnalyzer` automate formatting and enforce best practices, ensuring your scripts remain clean and standardized.
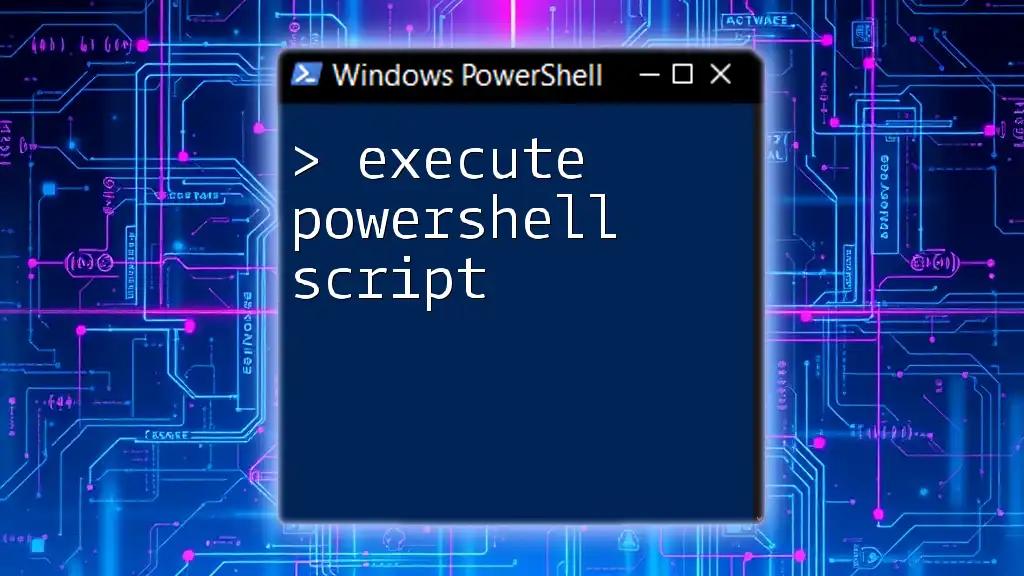
Best Practices for Script Formatting
Review and Refactor
Regularly reviewing and refactoring your code helps maintain script health. It encourages you to look for improvements, catch syntax errors, and ensure adherence to formatting conventions. Even small tweaks can lead to significant improvements over time.
Code Reviews
Engaging in code reviews with peers not only enhances your scripts but also fosters a culture of quality. Utilizing Pull Requests can streamline the process of maintaining formatting standards across teams.
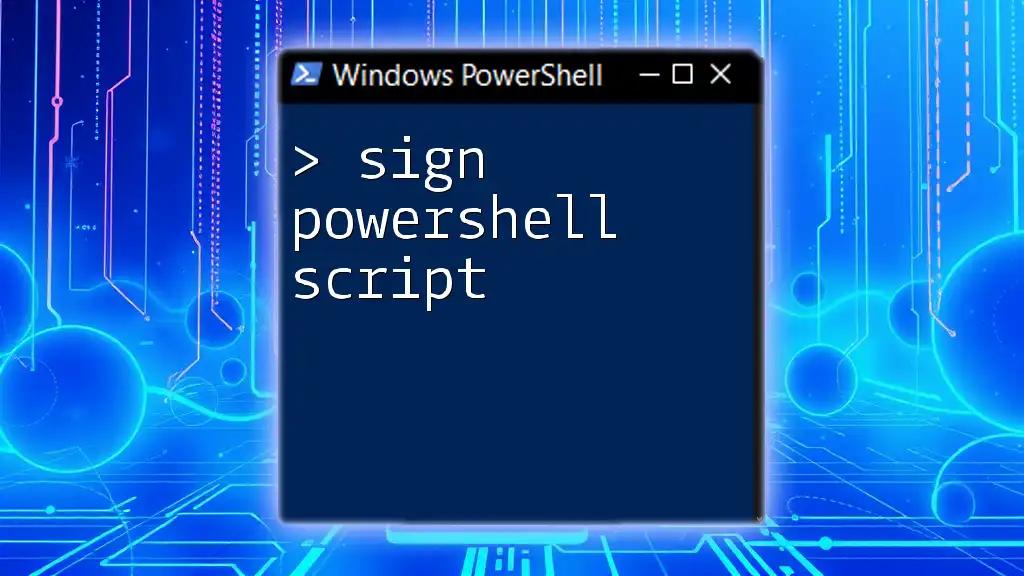
Conclusion
Properly formatting a PowerShell script is more than just adhering to aesthetic norms—it's about ensuring clarity, maintainability, and effectiveness in your scripting efforts. By adopting these best practices, you're setting yourself up for success as you write and share PowerShell scripts. Embrace these techniques, and experience the improved readability and functionality they bring.