You can test PowerShell scripts online using various web-based environments that allow you to execute and validate your commands without needing to install anything locally.
Here’s a simple example:
Write-Host 'Hello, World!'
What is PowerShell?
PowerShell is a powerful task automation framework that combines a command-line shell with an associated scripting language. Designed specifically for system administrators, PowerShell enables users to automate and manage system tasks, making it an essential tool in any IT professional's toolkit.
Core Components
Cmdlets
Cmdlets are simple, built-in functions that perform specific tasks within PowerShell. For instance, the `Get-Process` cmdlet fetches all running processes on your system.
Pipelines
Pipelines in PowerShell allow you to pass the output of one cmdlet as input to another. This feature is vital for chaining commands together to perform more complex actions efficiently. For example:
Get-Process | Sort-Object CPU -Descending
This command retrieves all processes and sorts them by their CPU usage in descending order.
Functions and Scripts
While cmdlets are single commands, functions are reusable blocks of code, and scripts are collections of cmdlets or functions saved in `.ps1` files. Understanding these fundamentals is critical for writing effective and reusable scripts.
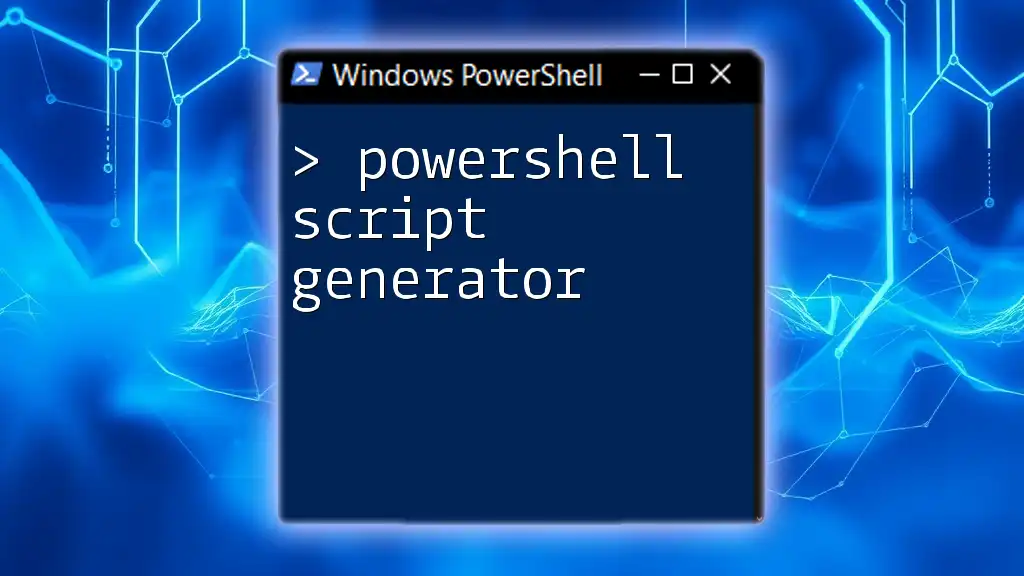
Importance of Testing PowerShell Scripts
Testing PowerShell scripts is essential for multiple reasons:
- Avoiding Errors: When scripts are not tested, they can produce errors that may disrupt operations. Testing helps catch these issues before deployment.
- Efficiency: A well-tested script runs smoothly and utilizes system resources effectively, contributing to overall system performance.
- Debugging: Testing is a crucial part of the debugging process. It allows developers to isolate issues and fix them, ensuring the script behaves as intended.
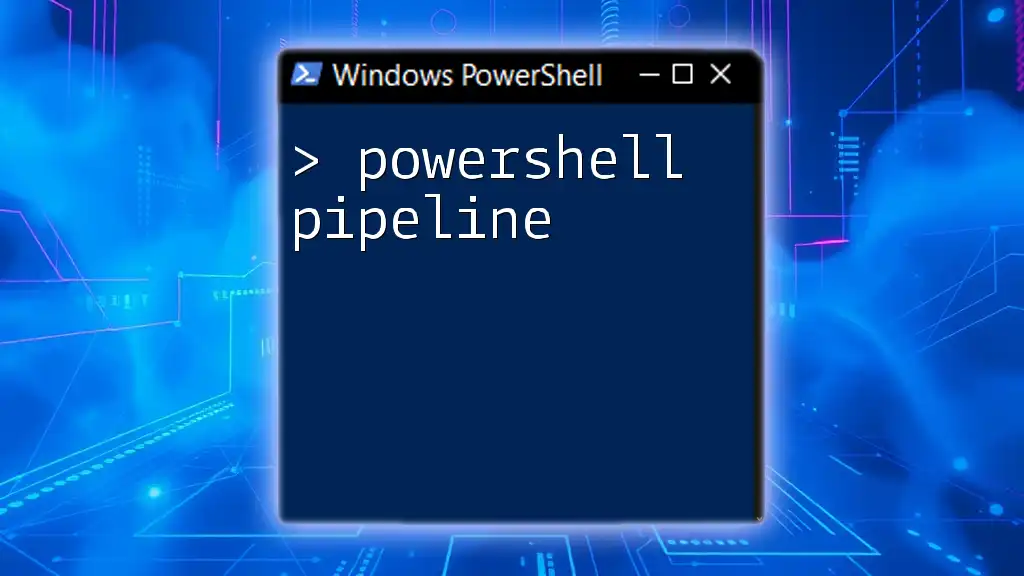
Popular Online Tools to Test PowerShell Scripts
With a plethora of online tools available, testing your PowerShell scripts has never been easier. These platforms allow you to write, execute, and debug your scripts without requiring a local installation of PowerShell.
Tool 1: PowerShell Online
PowerShell Online is a simple and effective platform for testing your scripts. It offers a clean interface, enabling users to run cmdlets without local setup.
To access it, simply navigate to the site and input your script in the provided window.
Example Usage
You can easily check running processes by copying the following command:
Get-Process
You’ll instantly see a list of processes currently active on your machine.
Tool 2: PowerShell Pro Tools
This tool enhances your testing experience with features like a script editor and debugging capabilities.
Interactive Features
With PowerShell Pro Tools, you can execute commands and see live results. This feature is particularly useful for testing code snippets on the fly.
Tool 3: Repl.it
Repl.it is a versatile online IDE that supports PowerShell scripts seamlessly. It provides a collaborative environment where you can write, share, and test your scripts.
Coding Environment
To start testing, navigate to the PowerShell environment within Repl.it, write your script, and click “Run.”
Example
Here’s a simple command you can test:
Write-Output "Hello, World!"
Executing this will display “Hello, World!” confirming that your script runs correctly.
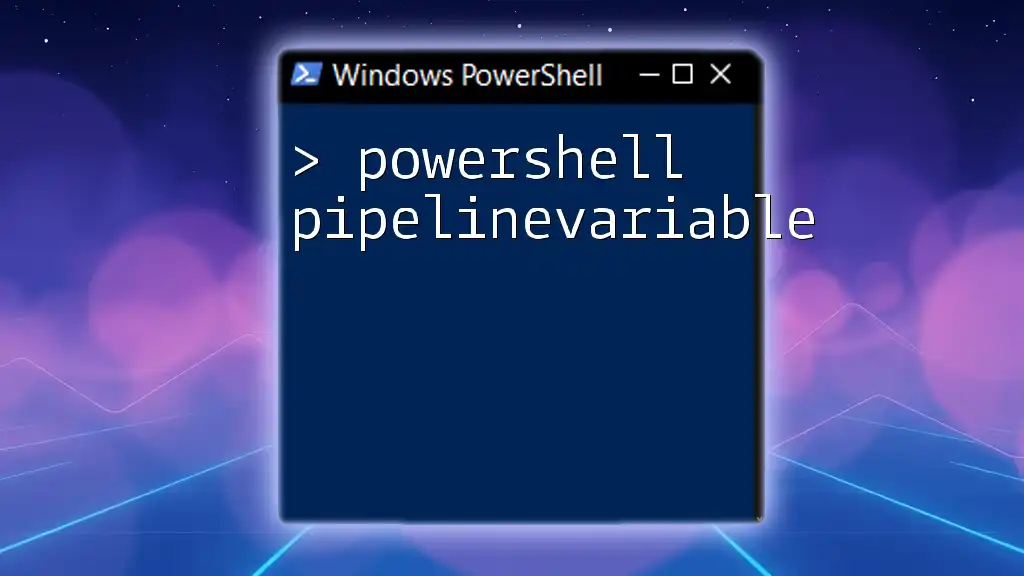
Steps to Test PowerShell Scripts Online
To effectively test your PowerShell scripts online, follow these straightforward steps:
Step 1: Choose Your Tool
Choose an online testing platform based on your needs. Consider user interface, features, and ease of use.
Step 2: Write Your Script
When writing scripts, focus on best practices:
- Use clear and descriptive variable names.
- Include comments for clarity.
- Structure your scripts logically.
Step 3: Run and Test the Script
After writing your script, execute it on the chosen platform. For example:
$currentTime = Get-Date
Write-Output "The current time is: $currentTime"
Step 4: Reviewing the Output
Check the output carefully. If the output is not as expected, review your code for errors or inconsistencies.
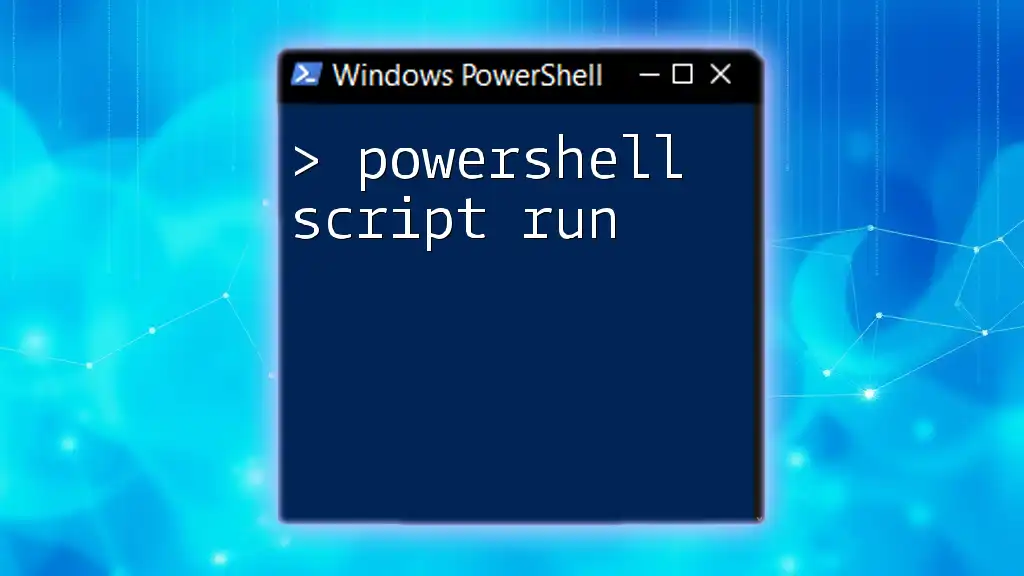
Best Practices for Testing PowerShell Scripts
Use Comments Effectively
Comments can significantly enhance the readability of your code. Using comment blocks to explain the purpose of code sections will help both you and others understand the script better.
Test in a Safe Environment
Whenever possible, test scripts in a controlled environment to prevent unintended changes to production systems.
Version Control
Maintain different versions of your scripts, especially before major changes. Utilize tools like Git to keep a history of updates.
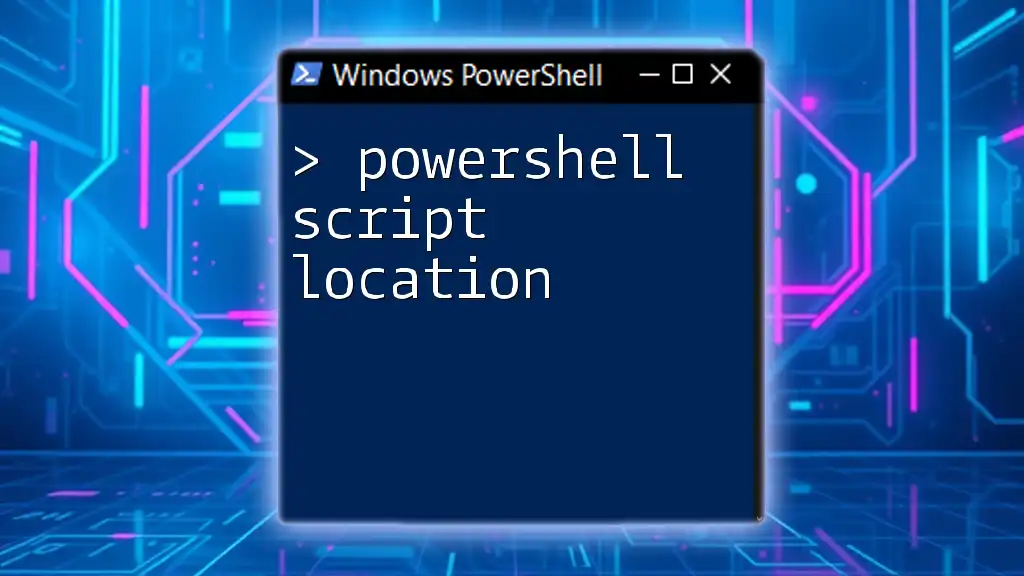
Troubleshooting Common Issues
Common Errors
While testing online, you might encounter common issues such as syntax errors or runtime exceptions. It’s essential to review error messages closely to identify the cause.
Debugging Tips
- Use `Write-Host` or `Write-Verbose` for tracking variables.
- Test smaller sections of the script independently to isolate problems.
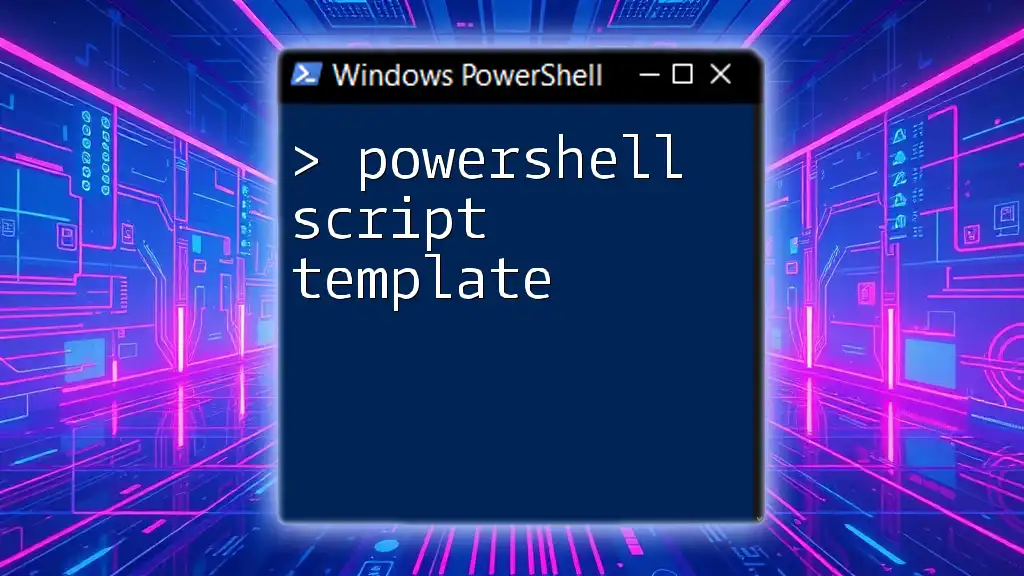
Conclusion
Testing your PowerShell scripts online is a valuable skill that can save time and improve efficiency in your workflow. By leveraging online tools and adhering to best practices, you can ensure your scripts are robust and reliable.
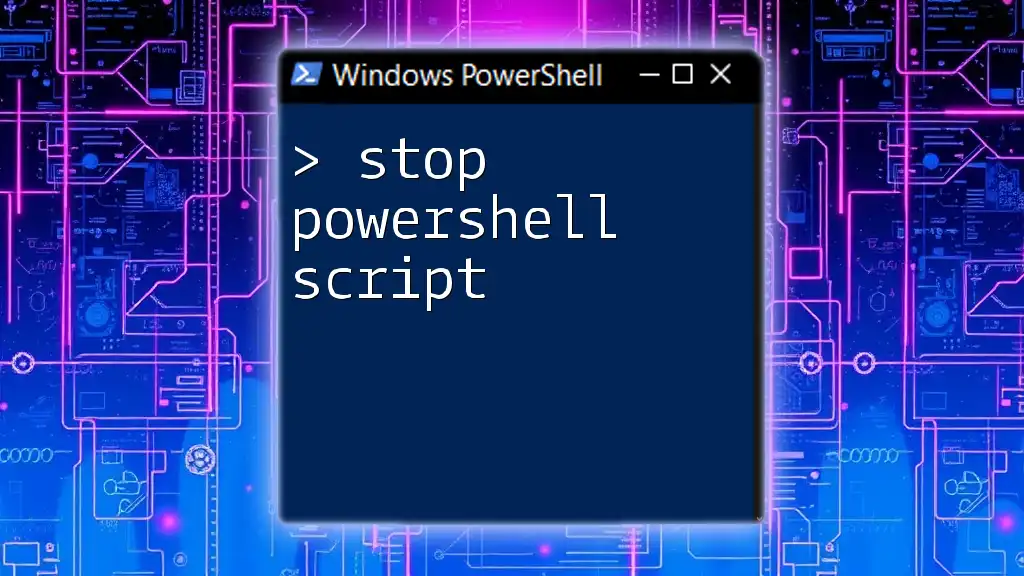
Call to Action
We encourage you to start testing your PowerShell scripts using the tools discussed in this guide. Share your experiences or ask questions in the comments section—your journey in mastering PowerShell scripting begins now!
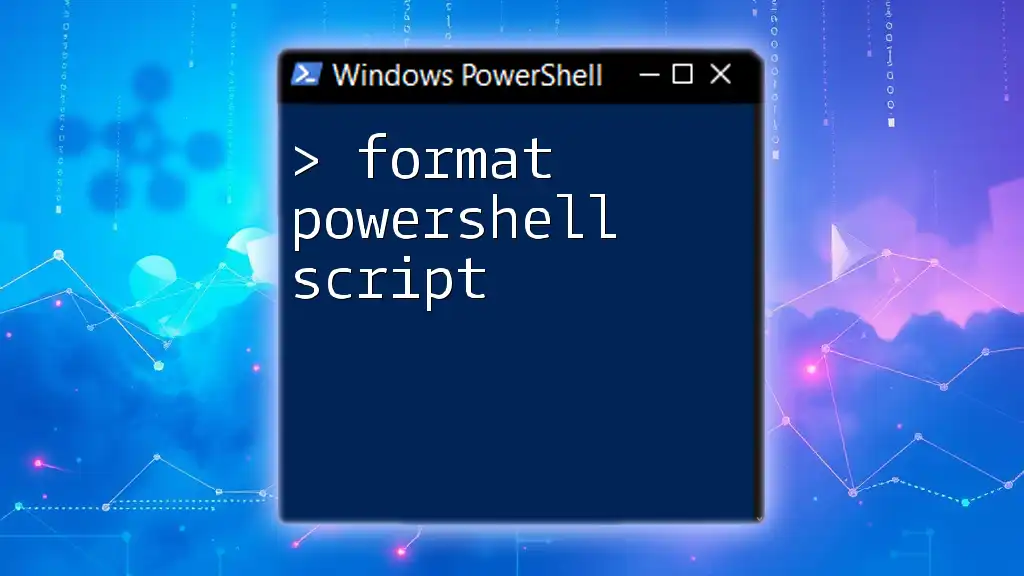
Additional Resources
For further reading, explore blogs, forums, and official documentation to deepen your understanding of PowerShell scripting and testing techniques. Happy scripting!