Handy PowerShell scripts are concise and efficient snippets of code that automate tasks and streamline workflows, making system administration easier and more accessible.
Here's a simple script to display a greeting:
Write-Host 'Hello, World!'
Getting Started with PowerShell
Understanding PowerShell
PowerShell is a powerful task automation framework, built on the .NET framework. It allows users to automate administrative tasks and manage system configurations through a command-line interface. PowerShell differs significantly from other scripting languages, like Bash, due to its object-oriented approach rather than a string-based one. This capability enables direct manipulation of data structures, making it especially useful for dealing with complex data.
Setting Up Your Environment
To begin using PowerShell, it's essential to set up your environment correctly. PowerShell is cross-platform and can be installed on Windows, macOS, and Linux. Here’s a quick guide on installing it on different operating systems:
- Windows: PowerShell is built into Windows 10 and later versions. You can launch it by typing "PowerShell" in the Start menu search.
- macOS: Use Homebrew to install PowerShell:
brew install --cask powershell
- Linux: Use the package manager appropriate for your distribution. For Ubuntu:
sudo apt-get install -y powershell
Once installed, consider using the Integrated Scripting Environment (ISE) or Visual Studio Code to write and execute your scripts. These tools offer features like syntax highlighting and integrated help, which greatly enhance the scripting experience.
Basic PowerShell Commands
Before diving into handy PowerShell scripts, familiarize yourself with cmdlets. Cmdlets are built-in PowerShell functions that perform specific tasks. A few fundamental cmdlets include:
- `Get-Command`: Lists all available cmdlets.
- `Get-Help`: Provides help on cmdlets and functions, ensuring you understand how to use them.
- `Get-Process`: Displays a list of currently running processes on your machine.
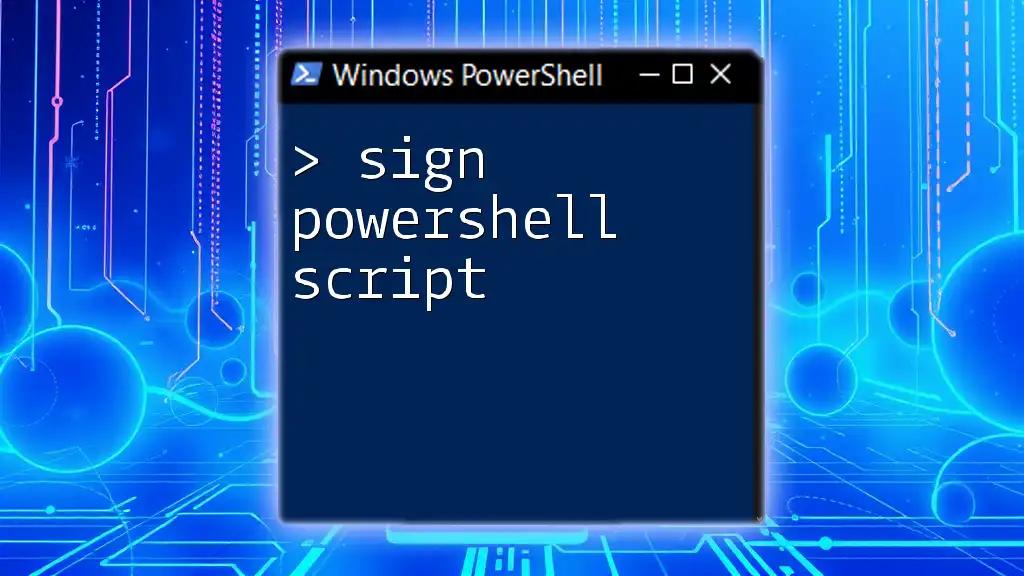
Handy PowerShell Scripts
File Management Scripts
Copying Files
Managing files is a common task for system administrators. A straightforward script to copy files from one directory to another can be implemented using the `Copy-Item` cmdlet. For example, to copy all files from a "Source" directory to a "Destination" folder, use this script:
Copy-Item -Path "C:\Source\*" -Destination "C:\Destination" -Recurse
This command copies all contents (including subfolders) from "C:\Source" to "C:\Destination". It’s essential to use the `-Recurse` switch when you want to include all subdirectories.
Deleting Files
Sometimes, you need to clean up directories by deleting unwanted files. To remove all temporary files (`.tmp`) from a specific folder, you can use the following script:
Get-ChildItem -Path "C:\Temp" -Filter "*.tmp" | Remove-Item
This command retrieves all temporary files in the "C:\Temp" directory and pipes them to `Remove-Item` to be deleted. Always ensure you specify the correct path and filter to avoid accidental deletions.
System Monitoring Scripts
Checking System Uptime
Knowing how long your system has been running can assist in monitoring system health. Use this simple script to display system uptime:
(Get-Culture).DateTimeFormat.ShortDatePattern
This command retrieves the culture-specific date format, which helps you understand the context of output as you build on this for more detailed system monitoring.
Monitoring Disk Space
A critical administrative task is checking disk space to prevent overflow. The following script checks available disk space on all drives:
Get-PSDrive | Where-Object {$_.Used -gt 0} | Select-Object Name, @{Name='Used';Expression={[math]::round($_.Used/1GB, 2)}}, @{Name='Free';Expression={[math]::round($_.Free/1GB, 2)}}
This command retrieves disk drives, filters out any unused drives, and selects the name, used space, and free space while converting values to gigabytes for better readability.
User Account Management Scripts
Adding a New User
In environments where Active Directory is deployed, creating new users is a routine task. Use the following script to add a new user:
New-ADUser -Name "JohnDoe" -GivenName "John" -Surname "Doe" -SamAccountName "jdoe" -UserPrincipalName "jdoe@domain.com" -AccountPassword (ConvertTo-SecureString "P@ssw0rd!" -AsPlainText -Force) -Enabled $true
In this command, you define user details and set a password. Note that executing this script requires the necessary administrative permissions in Active Directory.
Listing All User Accounts
To efficiently manage user accounts, retrieving a list can be tremendously helpful. The following command lists all local user accounts:
Get-LocalUser | Select-Object Name, Enabled, LastLogon
This command fetches local users on the system and displays their names, whether their accounts are enabled, and their last logon time. Adjust the `Select-Object` to include other properties based on your specific needs.
Network Management Scripts
Checking Network Connectivity
Verifying network connectivity is essential for troubleshooting. The following script pings multiple servers and logs the results:
$servers = @("google.com", "microsoft.com", "yahoo.com")
foreach ($server in $servers) {
Test-Connection -ComputerName $server -Count 2 | Select-Object Address, StatusCode
}
Here, the command stores server names in an array and iteratively pings each one, capturing the response status. This allows you to quickly determine which servers are reachable.
Retrieving IP Address Information
For network diagnostics, knowing your public IP address can be crucial. Use this script to retrieve IP address information:
Invoke-RestMethod -Uri "http://ipinfo.io/json"
This command fetches and outputs your public IP details in JSON format. Understanding how PowerShell can work with APIs allows for broader applications in network automation.
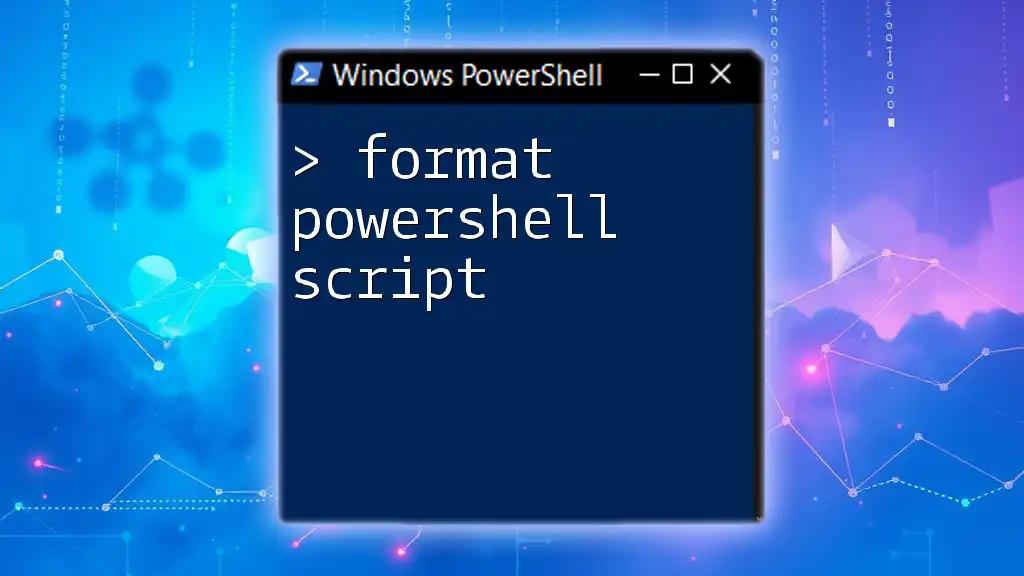
Advanced PowerShell Scripting Techniques
Creating Functions
Creating reusable functions is vital for maintaining clean and manageable scripts. Here’s how you can create a simple backup function:
function Backup-Files {
param (
[string]$source,
[string]$destination
)
Copy-Item -Path $source -Destination $destination -Recurse
}
This function takes source and destination parameters, making it easy to utilize it multiple times in different contexts. Remember to define function parameters clearly for better usability.
Error Handling in Scripts
Implementing error handling ensures your scripts run smoothly and can address issues as they arise. Using `Try`, `Catch`, and `Finally` blocks makes your scripts more robust. Here’s a simple example:
try {
# Code that may fail
} catch {
Write-Host "An error occurred: $_"
} finally {
Write-Host "Execution completed."
}
In the `Catch` block, you can handle errors accordingly, such as logging them or providing user feedback. This aspect is critical in production scripts to maintain reliability and traceability.
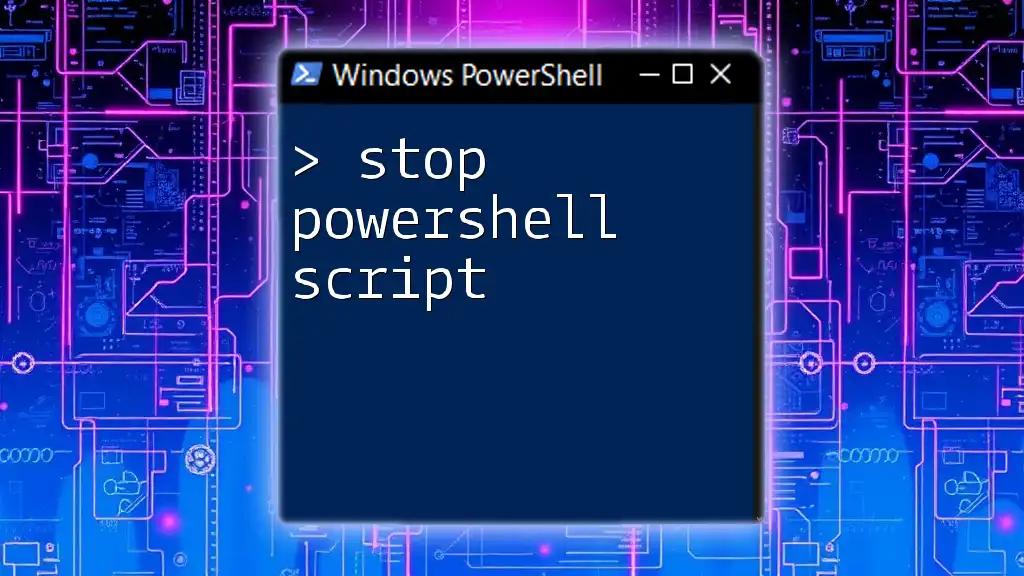
Conclusion
Utilizing handy PowerShell scripts can significantly enhance productivity and systems management efficiency. By understanding the basics and exploring the versatility of PowerShell through practical examples, you can automate routine tasks, streamline workflows, and effectively manage resources.
Additional Resources
For those eager to delve deeper into PowerShell, consider checking out the official PowerShell documentation and joining community forums. Many books and online courses are available to bolster your scripting skills, allowing you to harness the full power of PowerShell.
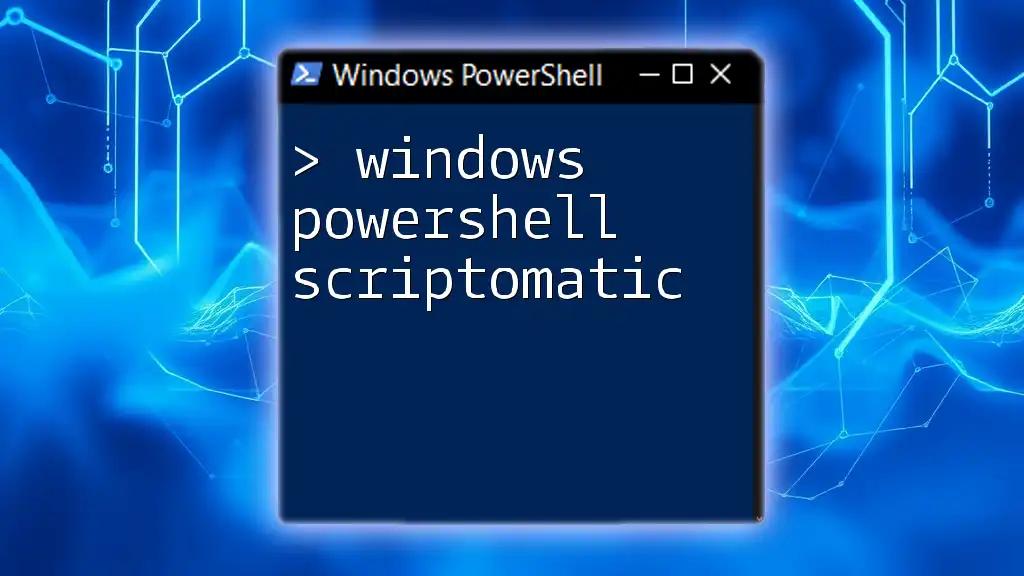
Call to Action
Now that you’re equipped with these handy PowerShell scripts, we invite you to explore them further. Share your experiences and personal scripts, and join us to enhance your PowerShell learning journey through our tailored training sessions and workshops.