You can call a PowerShell script from another PowerShell script by using the `&` (call operator) followed by the script path. Here’s a code snippet demonstrating this:
& 'C:\Path\To\Your\Script.ps1'
Understanding PowerShell Script Basics
A PowerShell script is a text file containing a series of PowerShell cmdlets and functions that automate tasks in the Windows environment. Scripts are typically saved with a `.ps1` file extension. The primary advantage of scripting in PowerShell is its ability to perform repetitive tasks efficiently, allowing users to save time and reduce the potential for human error.
Benefits of Using PowerShell for Automation
PowerShell offers a robust scripting environment, empowering users to automate mundane tasks. The flexibility of PowerShell allows you to interact with not only the Windows OS but also cloud services, databases, and applications, making it an essential tool for IT professionals and system administrators. By modularizing your scripts, you make them easier to maintain, test, and reuse across multiple projects.
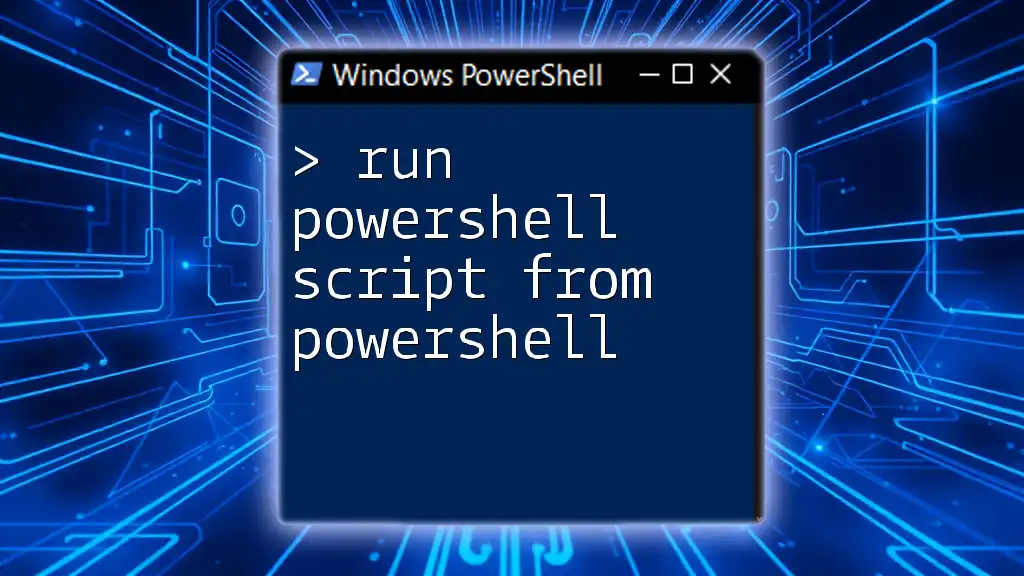
PowerShell Script Execution Fundamentals
Before diving into calling scripts from one another, it’s essential to understand how to execute a PowerShell script effectively. You can run scripts in several environments:
- Windows PowerShell ISE: This integrated scripting environment allows you to create, edit, and run PowerShell scripts seamlessly.
- Windows PowerShell and PowerShell Core: You can execute scripts directly from the command line using these methods.
Make sure you understand the execution policies in PowerShell. These policies determine whether scripts can run within your environment, with defaults often preventing the execution of unsigned scripts. You can check your current execution policy using:
Get-ExecutionPolicy
To set your execution policy to allow script execution, you may run:
Set-ExecutionPolicy RemoteSigned
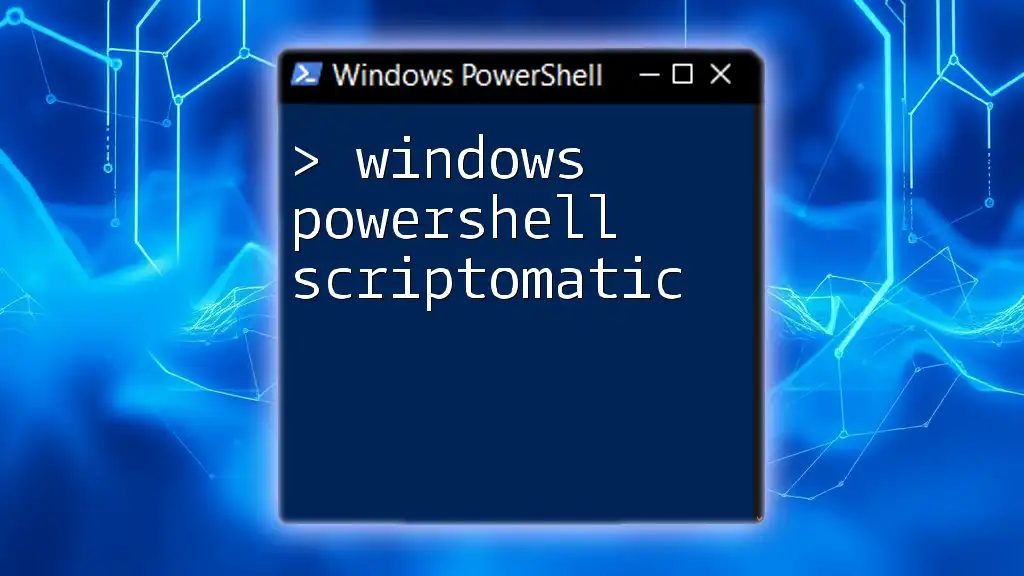
Calling a PowerShell Script from Another PowerShell Script
The Basic Syntax
To call a PowerShell script from another PowerShell script, you need to utilize the call operator `&`. This operator allows you to execute a script file within another script, enabling modular programming.
For example, you can call a script named `Script1.ps1` stored in the same directory with the following command:
& ".\Script1.ps1"
Using the call operator ensures that you run the script as expected.
Calling with Parameters
Often, scripts will require parameters to operate effectively, especially when passing data between scripts. You can define parameters in your target script and call it with specific values.
Example: Calling a Script with Parameters
Consider the following `Script1.ps1`, which accepts parameters:
param (
[string]$Param1,
[string]$Param2
)
Write-Host "Parameter 1: $Param1"
Write-Host "Parameter 2: $Param2"
You can call `Script1.ps1` from another script like this:
& ".\Script1.ps1" -Param1 "Value1" -Param2 "Value2"
This design significantly enhances the script’s flexibility and usability.
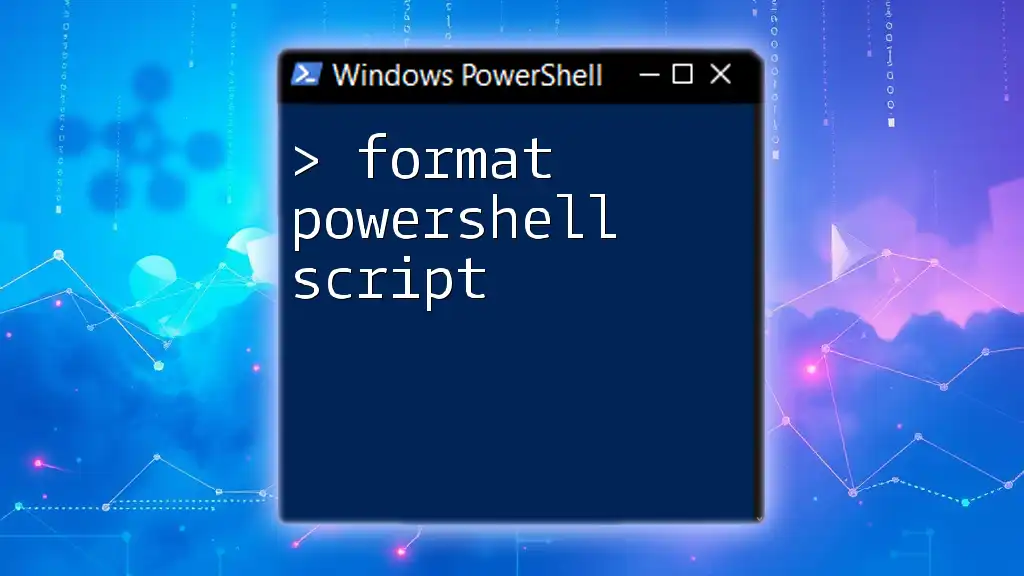
Best Practices for Calling Scripts
Organizing Your Scripts
Keeping scripts in the same directory is a standard practice that simplifies the call process. However, as your projects grow, you may organize scripts across multiple folders. Using relative paths can prevent issues when calling scripts from different directories, maintaining portability and reducing hard-coding of paths.
Error Handling
To make your scripts robust, use error handling practices, such as `Try-Catch` blocks. This strategy allows you to catch potential errors from executing the called script and handle them gracefully.
Example of Error Handling While Calling Another Script
try {
& ".\Script1.ps1"
} catch {
Write-Host "An error occurred: $_"
}
This implementation not only prevents the whole script from crashing but also gives you insight into what went wrong.
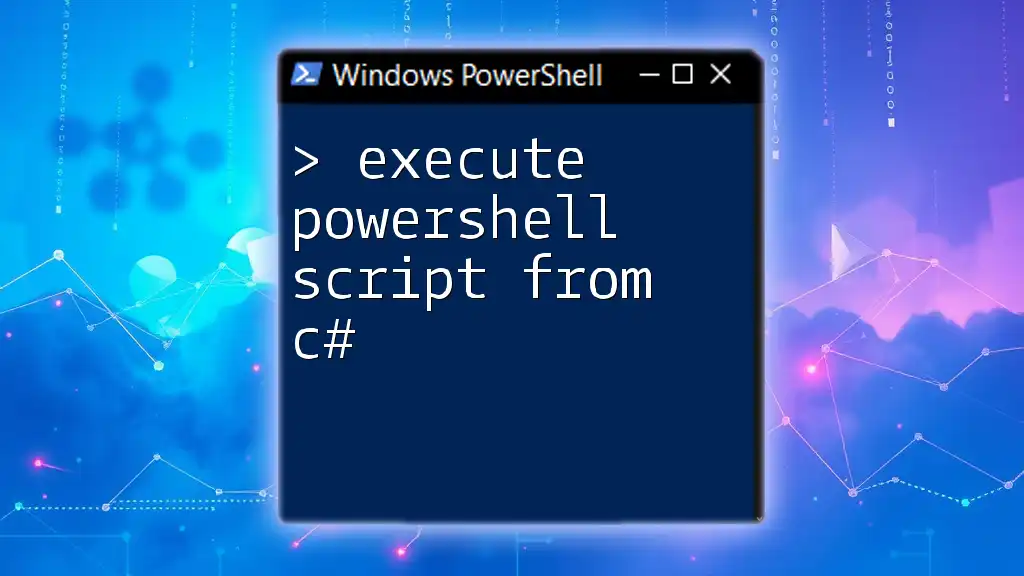
Advanced Techniques
Using Script Blocks
Script blocks are a powerful feature in PowerShell that allows you to package a set of commands together. You can create a script block that calls another script and execute it seamlessly.
Example:
$scriptBlock = { & ".\Script1.ps1" }
Invoke-Command -ScriptBlock $scriptBlock
This technique can be particularly useful when you need to invoke scripts in different execution conditions or scopes.
Executing Scripts in a Different Context
Advanced users can leverage Windows PowerShell jobs to run scripts in the background without blocking the current session. This capability is especially handy for long-running tasks.
Example of Running a Script in the Background:
Start-Job -ScriptBlock { & ".\Script1.ps1" }
This command submits `Script1.ps1` as a background job, allowing your main session to remain active and perform other tasks simultaneously.
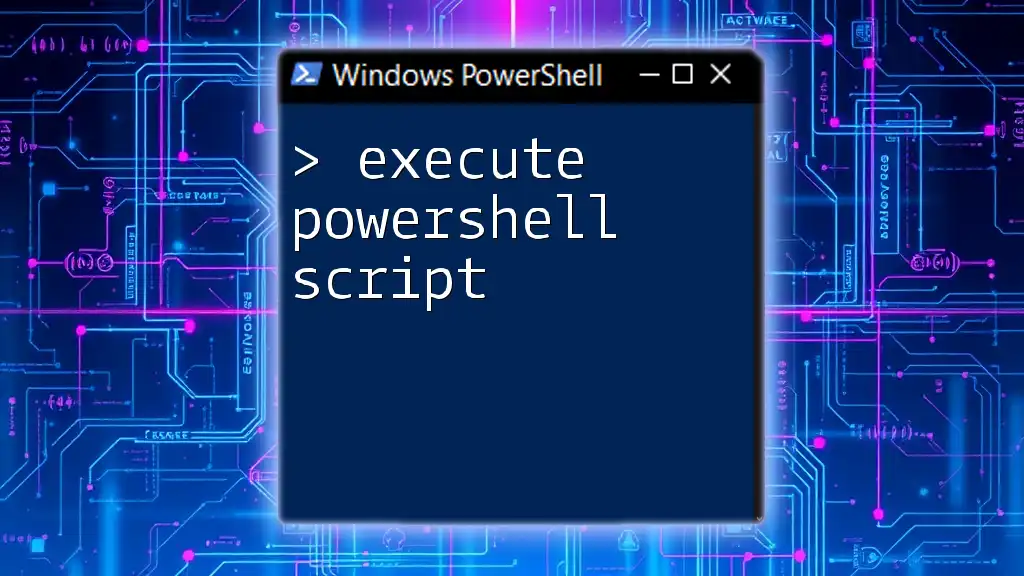
Troubleshooting Common Issues
When calling scripts, common errors may arise, typically related to script path issues or execution policies. Ensure that the path to the script is accurate; using quotes around the path helps when dealing with spaces.
For debugging, leverage Write-Debug and Set-PSDebug to gain insights into how your scripts are running and identify potential areas where things go awry.
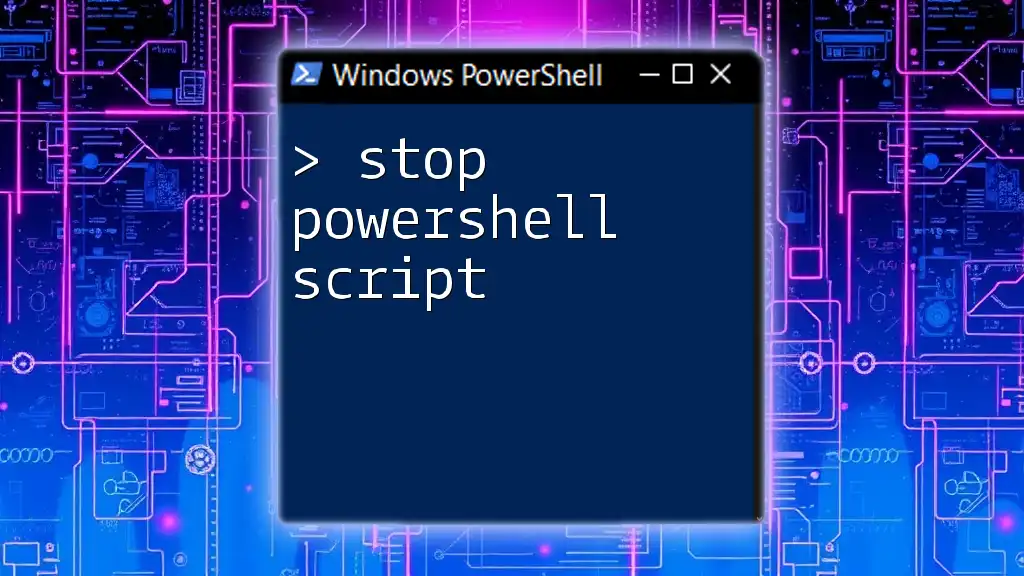
Conclusion
Calling a PowerShell script from another PowerShell script is a straightforward yet powerful feature that can dramatically enhance your scripting capabilities. By understanding the syntax, organizing your scripts well, and implementing best practices, you can streamline your automation tasks and improve maintainability. Embrace the modular philosophy and always be open to experimenting with different techniques, as PowerShell is an ever-evolving tool that offers endless opportunities for automation and efficiency.
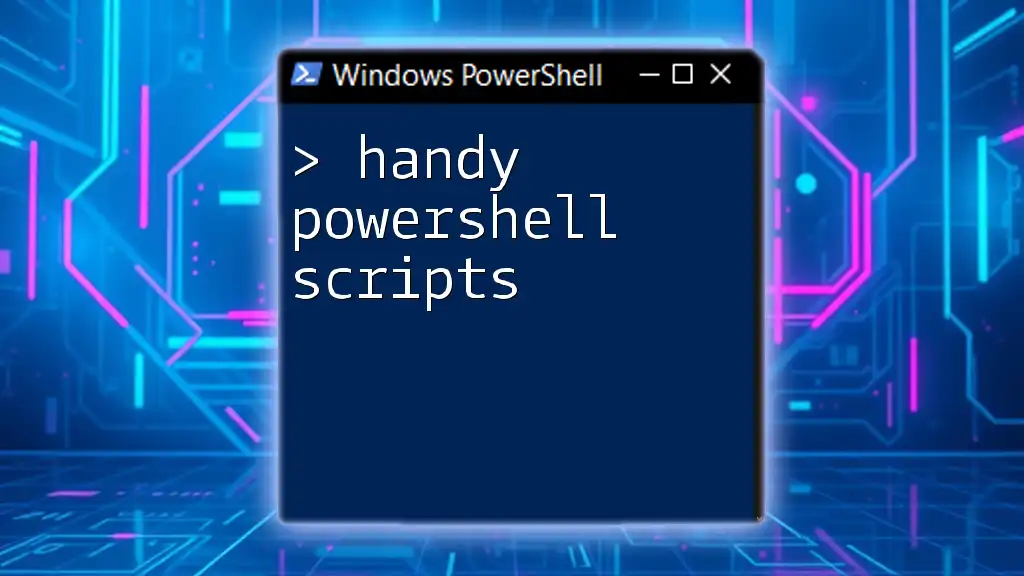
Call to Action
I encourage you to start trying out different scenarios where you can call one script from another. Explore the power of PowerShell scripting, and don’t hesitate to consult additional resources for an in-depth understanding as you continue your journey in automation.