To securely sign a PowerShell script using a domain Certificate Authority (CA), you can utilize the `Set-AuthenticodeSignature` cmdlet along with a code signing certificate issued by your CA.
Here's a code snippet to demonstrate how to sign a script:
$cert = Get-ChildItem Cert:\CurrentUser\My | Where-Object { $_.Subject -like "*YourCA*"} # Replace *YourCA* with the subject name of your certificate
Set-AuthenticodeSignature -FilePath "C:\Path\To\YourScript.ps1" -Certificate $cert
Understanding Digital Signatures
What is a Digital Signature?
A digital signature is a cryptographic technique that allows individuals and organizations to ensure the authenticity and integrity of statements or documents. When applied to PowerShell scripts, a digital signature verifies that the script comes from a verified source and has not been altered during transmission. This is crucial in environments where scripts can be shared or executed across different systems.
Benefits of Signing PowerShell Scripts
Signing your PowerShell scripts offers several vital advantages:
- Enhanced Security: By creating a signature, you protect the script against tampering. Any unauthorized changes will invalidate the signature, alerting users to potential issues.
- Establishes Trust: A signature assures users that the script is authentically from the claimed publisher, creating a level of trust in automated processes and internal tools.
- Compliance: In many organizations, policies require scripts to be signed to meet security standards and auditing processes.

Key Concepts in Signing PowerShell Scripts
PowerShell Execution Policy
Understanding the execution policy is fundamental to working with signed scripts. Execution policies are a safety feature in PowerShell that determines the rules for running scripts. The key types include:
- Restricted: No scripts can run.
- RemoteSigned: Only downloaded scripts must be signed by a trusted publisher.
- AllSigned: All scripts, including local ones, must be signed by a trusted publisher.
You can check the execution policy settings with the following command:
Get-ExecutionPolicy -List
Code Signing Certificates
A Code Signing Certificate is a digital certificate that allows you to sign your scripts. There are two primary types of certificates:
- Self-Signed Certificates: Useful for development environments but generally not trusted by systems without additional configuration.
- Domain CA-issued Certificates: Certificates obtained from a trusted Certificate Authority (CA) that are universally recognized, allowing smooth execution of signed scripts across various systems.
The importance of obtaining a certificate from a trusted CA cannot be overstated, as it plays a significant role in establishing credibility.
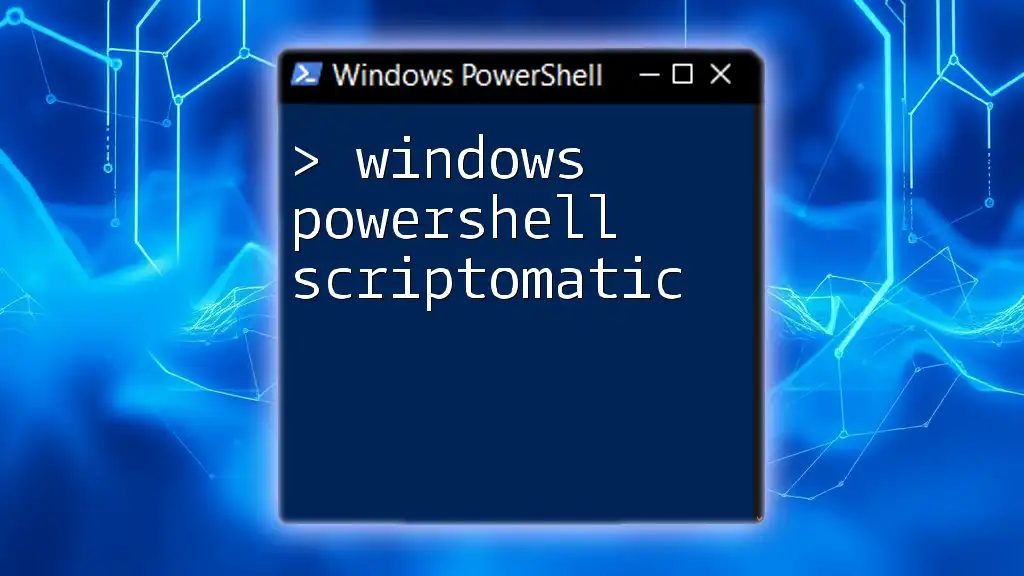
Pre-requisites for Signing with Domain CA
Obtaining a Code Signing Certificate
The journey to sign a PowerShell script with a Domain CA begins with obtaining a code signing certificate. Follow these essential steps:
- Request Certificate: Initiate a request through your organization's domain CA. You might typically utilize a form or an internal request system, depending on your organization's policies.
- Required Information: Prepare the necessary information, such as your organization's details, specific DNS names, and other identifiers.
Setting Up PowerShell to Use the Signed Scripts
Before signing, ensure that your environment is ready. Use the `Get-AuthenticodeSignature` cmdlet to view the signature status of your scripts. For example, run:
Get-AuthenticodeSignature -FilePath "C:\Scripts\SampleScript.ps1"
This command shows the current signature status, allowing you to verify if the script is already signed or needs attention.
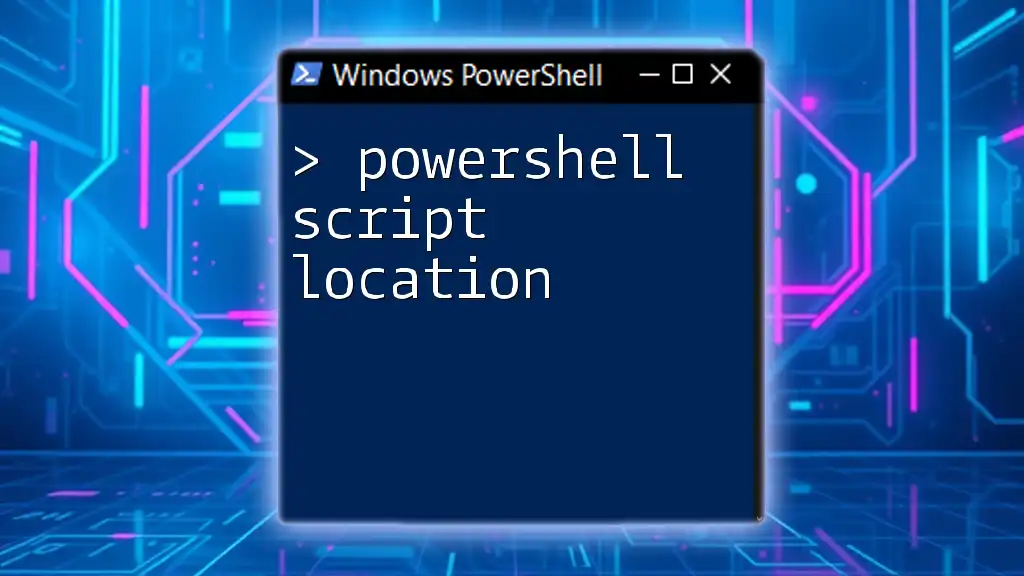
Signing a PowerShell Script
Step-by-Step Guide to Sign a Script
To sign a PowerShell script, follow these detailed steps:
-
Load Required Assembly: Start by loading the necessary .NET assembly, which provides the classes required for signing functions:
[Reflection.Assembly]::LoadWithPartialName('System.Security')
-
Retrieve the Certificate: Use the following example to get your domain CA-issued certificate. This command filters for certificates that match your domain:
$certificate = Get-ChildItem Cert:\CurrentUser\My | Where-Object { $_.Subject -like "*YourDomain*" }
-
Sign the Script: Use the `Set-AuthenticodeSignature` cmdlet to apply the signature to your script:
Set-AuthenticodeSignature -FilePath "C:\Scripts\SampleScript.ps1" -Certificate $certificate
Verifying the Signature
After signing the script, it’s essential to verify that the signature is secure and valid. You can run the following command to check the signature status:
Get-AuthenticodeSignature -FilePath "C:\Scripts\SampleScript.ps1"
This command provides information regarding the signature, including whether it’s valid or has issues.
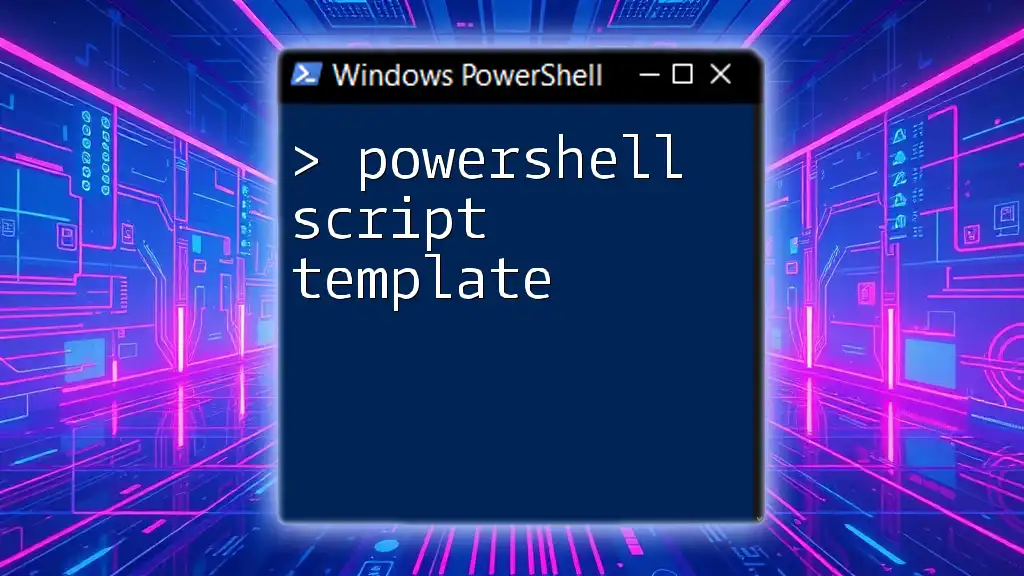
Troubleshooting Common Issues
Signature Trust Issues
If users encounter trust issues while executing the signed script, common reasons might include the absence of the CA in their trusted root, or the certificate has expired. To resolve these issues, ensure that the certificate authority is trusted on all machines, and renew expired or invalid certificates promptly.
Script Execution Policy Errors
Sometimes, even signed scripts can encounter execution policy errors. To resolve these errors, you may need to change the execution policy. You can do so using the command:
Set-ExecutionPolicy RemoteSigned
Choose the least restrictive policy that still protects your environment effectively.
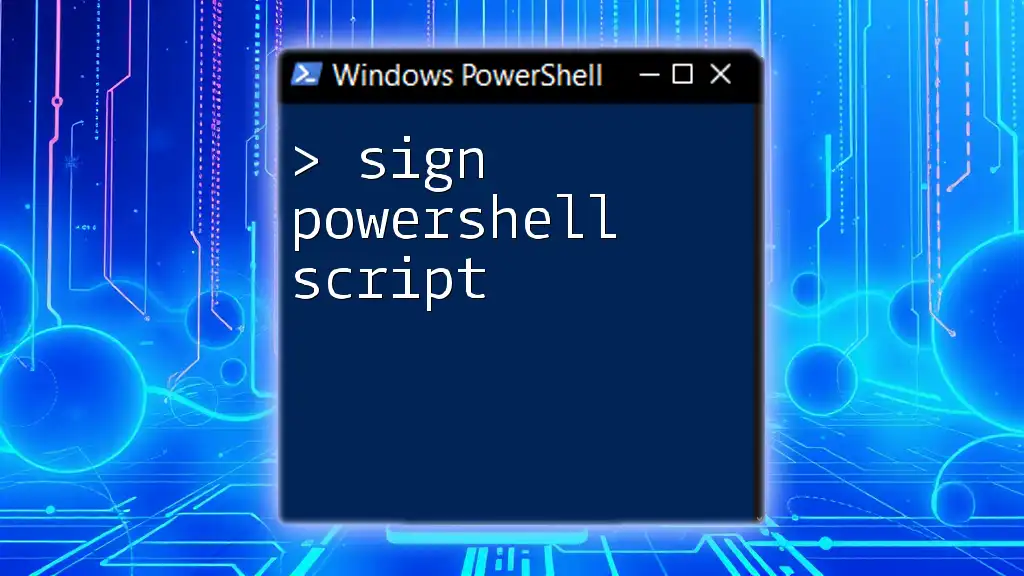
Best Practices for Signing PowerShell Scripts
Regularly Update Certificates
Maintaining the security of your scripts includes keeping your code signing certificates up to date. Certificates have validity periods and should be renewed well before they expire to ensure uninterrupted capability to sign scripts.
Controlling Script Distribution
Distributing signed scripts should be done with care. Implementing proper permissions and access control can prevent unauthorized use or sharing of sensitive scripts within your organization.
Educating Users About Signed Scripts
It’s crucial to educate your team about recognizing signed scripts. Training sessions on sign verification and the importance of only running trusted scripts can significantly reduce security risks.
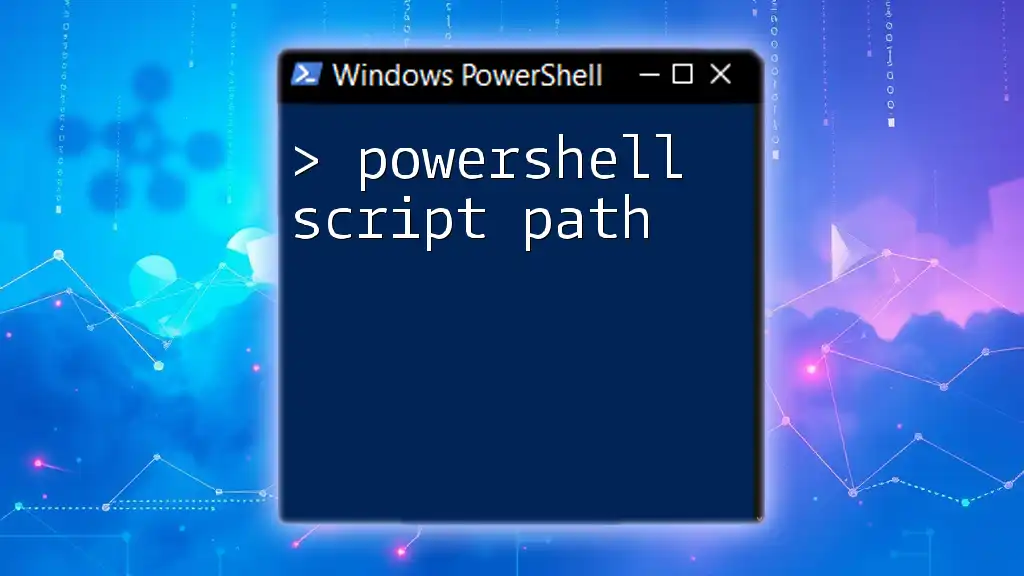
Conclusion
Signing PowerShell scripts using a Domain CA is an essential practice for ensuring script integrity and authenticity in secure environments. By following the outlined steps and best practices, organizations can enhance their cybersecurity posture and comply with relevant policies. Implement these strategies to bring a higher level of trust and security to your PowerShell scripting practices.
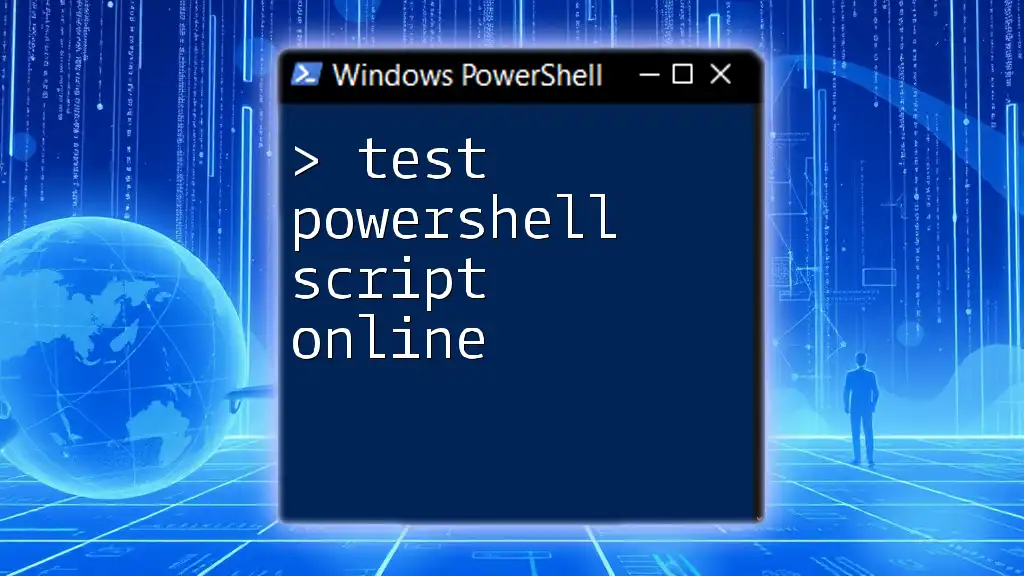
Additional Resources
Useful Links and References
For more information, refer to the official PowerShell documentation on signing scripts, which provides in-depth guidance and updates.
Further Learning
Consider exploring courses on PowerShell security and scripting best practices to deepen your understanding and application of these concepts.