Writing a PowerShell script involves creating a text file containing a series of PowerShell commands that automate tasks or manage system configurations, enabling users to execute complex processes with ease.
Write-Host 'Hello, World!'
Understanding PowerShell Scripting Basics
What is a PowerShell Script?
A PowerShell script is a file that contains a series of PowerShell commands and functions that automate tasks. While cmdlets are built-in PowerShell commands, scripts allow users to create customized sequences of operations that can be executed in one go.
Essential Elements of a PowerShell Script
Syntax and Structure
The basic structure of a PowerShell script is straightforward and consists mainly of cmdlets and functions. Here's a simple example of a script that outputs "Hello, World!" to the console:
Write-Host "Hello, World!"
This one-liner demonstrates how easy it is to write a PowerShell script. The Write-Host cmdlet is used to display output in PowerShell.
Comments
Comments are an essential part of scripting that allows you to annotate your code for clarity and future reference. In PowerShell, single-line comments start with a `#`, while multi-line comments can be enclosed using `<#` at the beginning and `#>` at the end.
# This is a single-line comment
<#
This is a
multi-line comment
#>
Good commenting practices help improve maintainability and readability, making it easier for you and others to understand the code later.
File Extensions and Execution Policies
File Extensions
PowerShell scripts are saved with the `.ps1` file extension. This is the default format that PowerShell recognizes when executing scripts.
Execution Policies
Execution policies are a security feature that helps determine which scripts can run on your system. You need to be aware of your current execution policy and how to change it. To check your current policy, use:
Get-ExecutionPolicy
To set a policy that allows scripts from your local machine, you can use:
Set-ExecutionPolicy RemoteSigned
It's crucial to know how execution policies work to avoid running into issues when executing scripts.
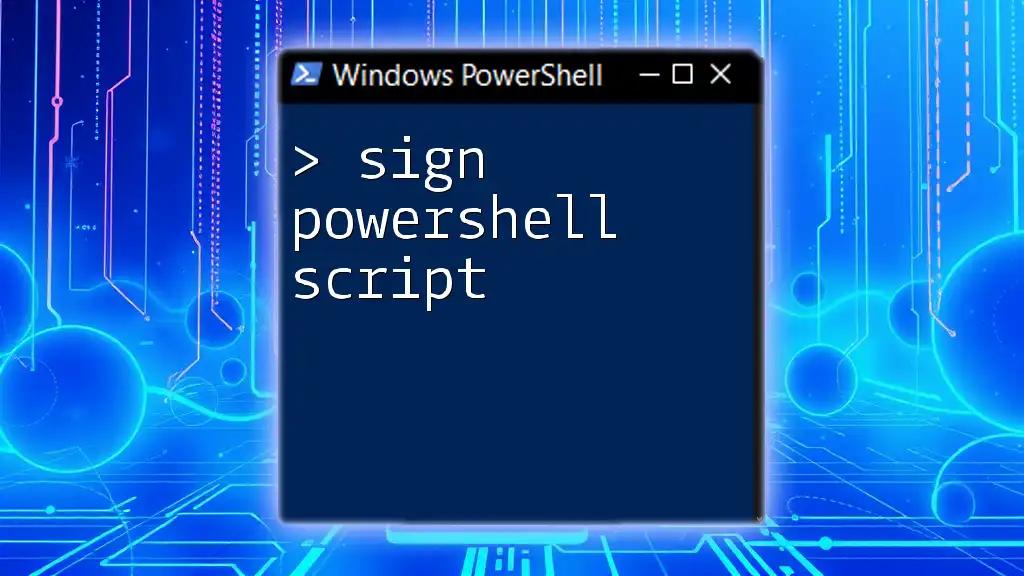
Writing Your First PowerShell Script
Setting Up Your Environment
To start creating PowerShell scripts, you need an environment conducive to coding. Two popular options are the PowerShell Integrated Scripting Environment (ISE) and Visual Studio Code. Both of these IDEs offer features like syntax highlighting and debugging tools that facilitate a smoother scripting experience.
Step-by-Step Guide to Creating Your First Script
-
Open Your PowerShell IDE: Start by launching your chosen IDE. Familiarize yourself with its layout to navigate through code easily.
-
Write the Script: Create a script that retrieves system information. This is a straightforward and practical example. The script can be as simple as:
Get-ComputerInfo
This command gathers and displays detailed information about the computer.
-
Save the Script: Save your new script as `systemInfo.ps1` in a directory you can easily access.
-
Run the Script: To execute your script, open PowerShell, navigate to the directory where your script is saved, and run:
.\systemInfo.ps1
By following these steps, you have successfully created and executed your first PowerShell script!
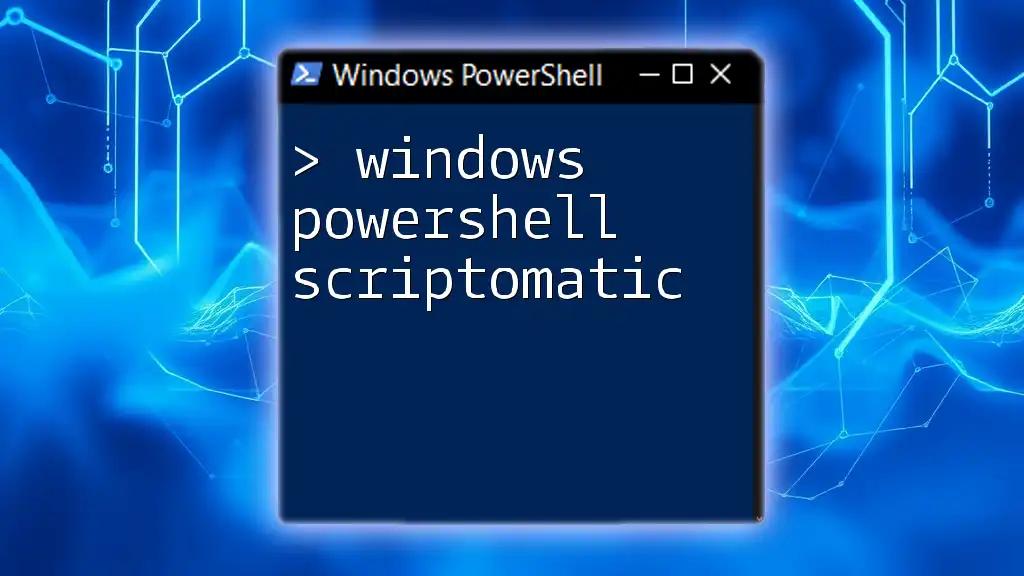
Key Scripting Concepts
Variables and Data Types
Variables are crucial in PowerShell scripting because they allow you to store and manipulate data. To declare a variable, use the `$` symbol:
$myVariable = "Hello, PowerShell"
PowerShell supports various data types, including strings, integers, arrays, and hashtables, enabling you to work with diverse information types efficiently.
Control Structures
Conditional Statements
Conditional statements allow your script to make decisions based on conditions. The `if`, `else`, and `switch` statements are commonly used. For example:
if ($myVariable -eq "Hello, PowerShell") {
Write-Host "Match found!"
}
The above code checks if `myVariable` equals "Hello, PowerShell" and outputs "Match found!" if the condition is true.
Loops
Loops enable repetitive execution of a block of code. PowerShell supports several types, such as `for`, `foreach`, `while`, and `do-while`. Here's how you can use a `foreach` loop:
$items = 1..5
foreach ($item in $items) {
Write-Host "Item: $item"
}
This script outputs the numbers 1 to 5, demonstrating how to iterate over a range of items.
Functions
Creating Functions
Functions are reusable blocks of code that perform a particular task. They help in modularizing the script and enhancing readability. Here's an example of a simple function:
function Get-Greeting {
param($name)
return "Hello, $name!"
}
To call this function, you simply pass a name:
Get-Greeting "Alice"
This outputs "Hello, Alice!" allowing for reusable greeting functionality.
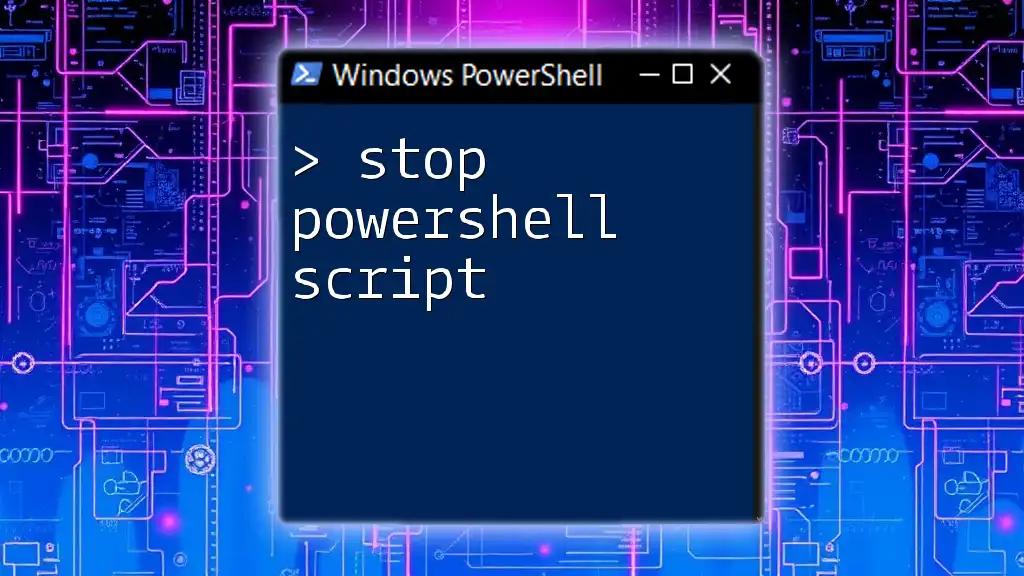
Advanced PowerShell Scripting Techniques
Error Handling
Handling errors gracefully is vital in scripting. Using `try`, `catch`, and `finally` blocks can help manage exceptions effectively. Here’s an example:
try {
# Code that may throw an error
Get-Content "nonexistentfile.txt"
} catch {
Write-Host "An error occurred: $_"
}
Should the file not exist, the script captures the error and prints a message without stopping execution.
Working with Objects
Understanding Objects in PowerShell
PowerShell treats everything as an object, making it powerful for handling data. You can manipulate these objects easily using properties and methods.
Pipelines
Pipelines allow you to pass the output of one command directly into another command. This is a core feature of PowerShell that enables more streamlined and efficient coding. For example:
Get-Process | Where-Object {$_.CPU -gt 100}
This command retrieves a list of processes that use more than 100 CPU seconds, showcasing the power of chaining commands.
Script Parameters
Creating Parameterized Scripts
Accepting user input can make your scripts more dynamic. Here’s how to create scripts that take parameters:
param(
[string]$name,
[int]$age
)
Write-Host "Name: $name, Age: $age"
When you execute this script, you can provide values for `name` and `age`, allowing your script to respond to user input effectively.
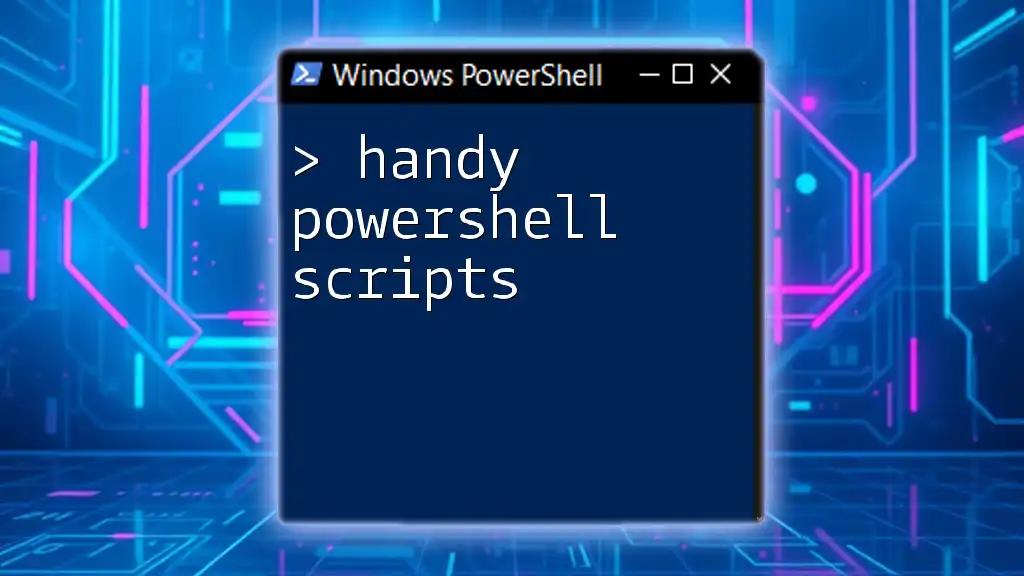
Debugging PowerShell Scripts
Techniques for Debugging
Debugging is crucial for finding and fixing errors in your code. Use the `Write-Debug` cmdlet to output debugging information while executing your script. You can also set breakpoints in the IDE to pause execution and inspect variable values.
Common Debugging Commands
Additionally, commands like `Get-Error` and `Write-Error` can help identify issues. For example:
Write-Error "This is an error message"
This outputs a specified error message, providing more context about issues that may arise.
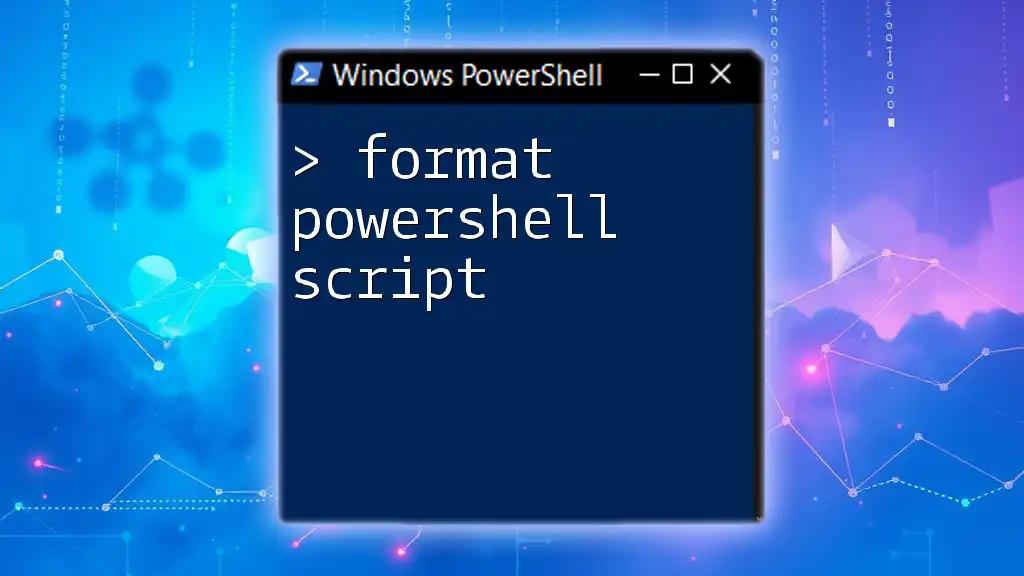
Best Practices for PowerShell Scripting
Code Readability
Maintainability is crucial in scripting. Indentation and appropriate spacing can significantly enhance the readability of your code. Always strive to write clear and well-structured scripts.
Version Control
Using Git or similar version control systems for your scripts can help manage changes and collaborate effectively. Version control allows you to navigate through different versions of your scripts quickly.
Documentation
Documenting your scripts is essential for clarity and future reference. Consider adding a README file or using inline comments to explain your code.
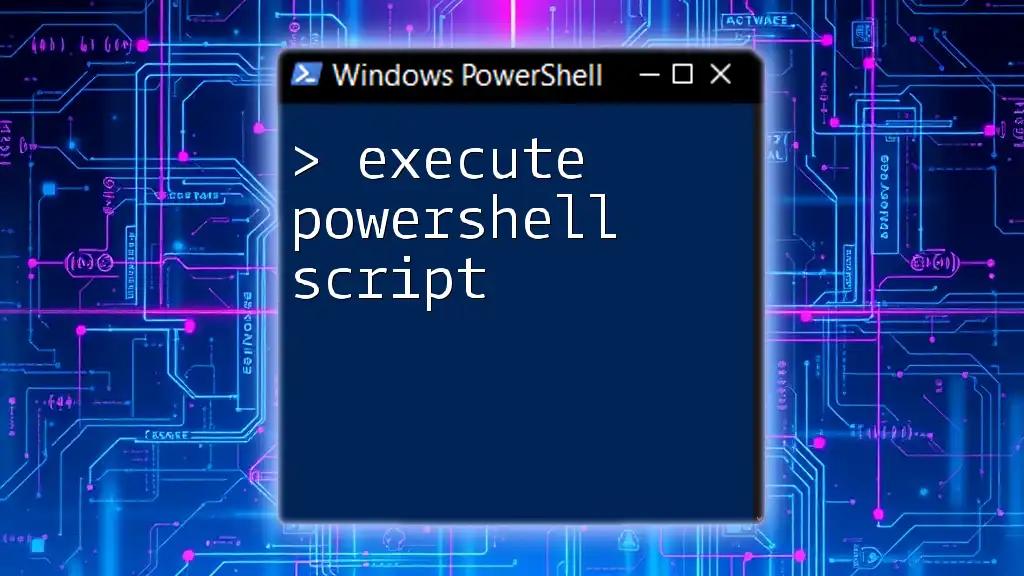
Conclusion
Building your skills in writing a PowerShell script can enhance your productivity and efficiency significantly. Practice regularly and experiment with different scripts to consolidate your knowledge and mastery of this powerful tool.
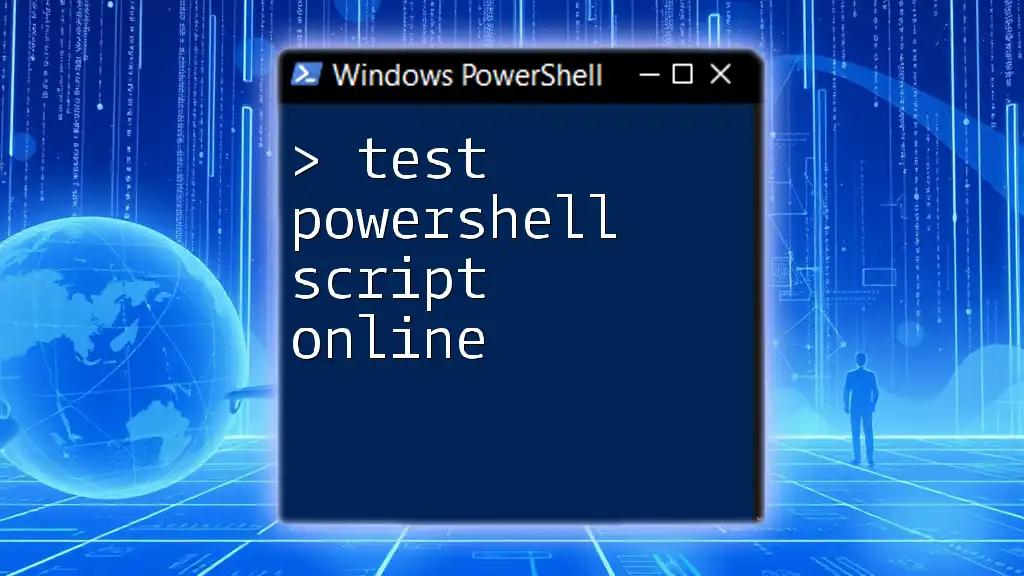
Additional Resources
To expand your expertise, explore books, online courses, and community forums dedicated to improving PowerShell scripting skills. With continual learning, you can develop proficient scripting abilities that will serve you well in various tasks and projects.