You can pass a dictionary as a parameter in PowerShell by using a hashtable, which allows you to organize key-value pairs for easy access and manipulation.
Here's a code snippet demonstrating how to create and pass a hashtable:
# Define hashtable
$dictionary = @{
"Name" = "John"
"Age" = 30
"City" = "New York"
}
# Function that takes a hashtable as a parameter
function Show-PersonDetails {
param (
[hashtable]$Person
)
Write-Host "Name: $($Person['Name'])"
Write-Host "Age: $($Person['Age'])"
Write-Host "City: $($Person['City'])"
}
# Call the function with the hashtable
Show-PersonDetails -Person $dictionary
What is a Dictionary (Hash Table) in PowerShell?
Definition of Dictionary
In PowerShell, a dictionary, often referred to as a hash table, is a data structure that stores information in key-value pairs. This format allows for efficient data retrieval using a unique key, making it particularly advantageous when managing configurations, parameters, or any variable-length data scenarios. For example, if you need to store user information, a hash table can easily provide quick access to values like name, age, or city by their corresponding keys.
Creating a Hash Table
Creating a hash table in PowerShell is straightforward. Syntax-wise, it is defined using the `@{}` braces containing key-value pairs separated by semicolons. Here’s a simple example:
$myDictionary = @{"Name"="Alice"; "Age"=30; "City"="New York"}
In this code snippet, the hash table `$myDictionary` contains three keys: “Name”, “Age”, and “City”, each paired with its corresponding value. This structure allows you to manage and retrieve user-related information efficiently.
Common Use Cases for Hash Tables
Hash tables are beneficial in various scenarios, including but not limited to:
- Storing Configuration Settings: Keep track of application settings or script variables.
- Managing Complex Data: Handle user inputs or record multiple attributes of an entity.
- Optimization for Lookups: Provide fast lookups based on unique keys, improving the performance of scripts.
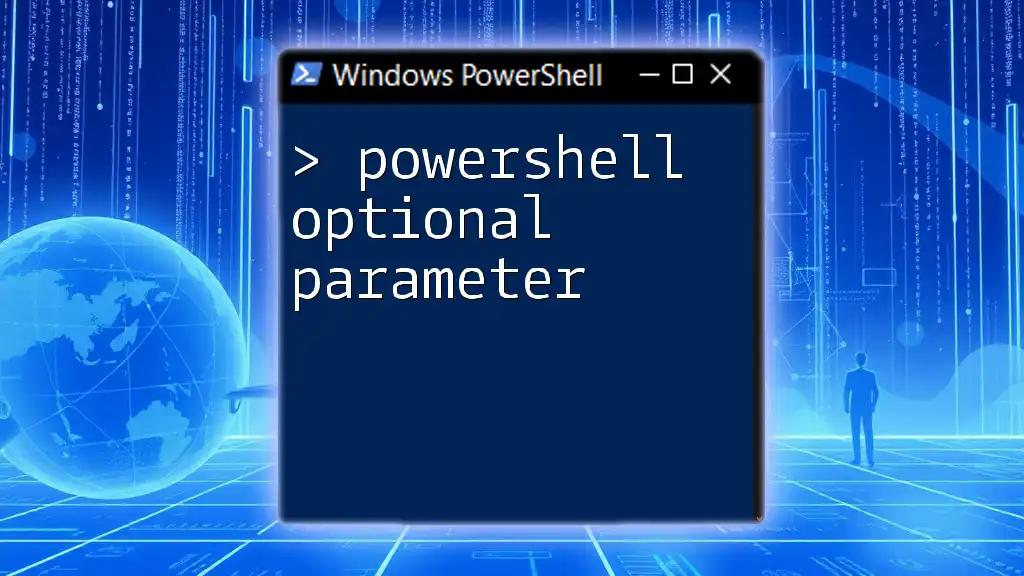
Passing a Dictionary as a Parameter
Why Pass a Dictionary?
Using a dictionary as a parameter offers flexibility and readability. When functions need to manage multiple related values, passing a hash table can significantly simplify the function's signature and enhance code clarity. Instead of numerous individual parameters, a single hash table encapsulates all necessary information.
Defining a Function that Accepts a Dictionary
To create a function that accepts a hash table, you need to specify the parameter type as `[hashtable]`. Here’s an example of a simple function called `Get-UserInfo` that takes a hash table as its parameter:
function Get-UserInfo {
param (
[hashtable]$userInfo
)
Write-Host "Name: $($userInfo['Name'])"
Write-Host "Age: $($userInfo['Age'])"
Write-Host "City: $($userInfo['City'])"
}
In this function, the `param()` block defines that `$userInfo` is a hash table. Within the body of the function, we utilize `$userInfo['Key']` syntax to access the values stored under specific keys.
Example of Passing a Dictionary to a Function
Let’s illustrate the concept further with a practical example. Here’s how you can pass a dictionary to `Get-UserInfo`:
$userInfo = @{"Name"="Alice"; "Age"=30; "City"="New York"}
Get-UserInfo -userInfo $userInfo
When you run this code, the expected output will be:
Name: Alice
Age: 30
City: New York
This demonstrates the function extracting the values from the hash table, showcasing its effectiveness in managing structured data.
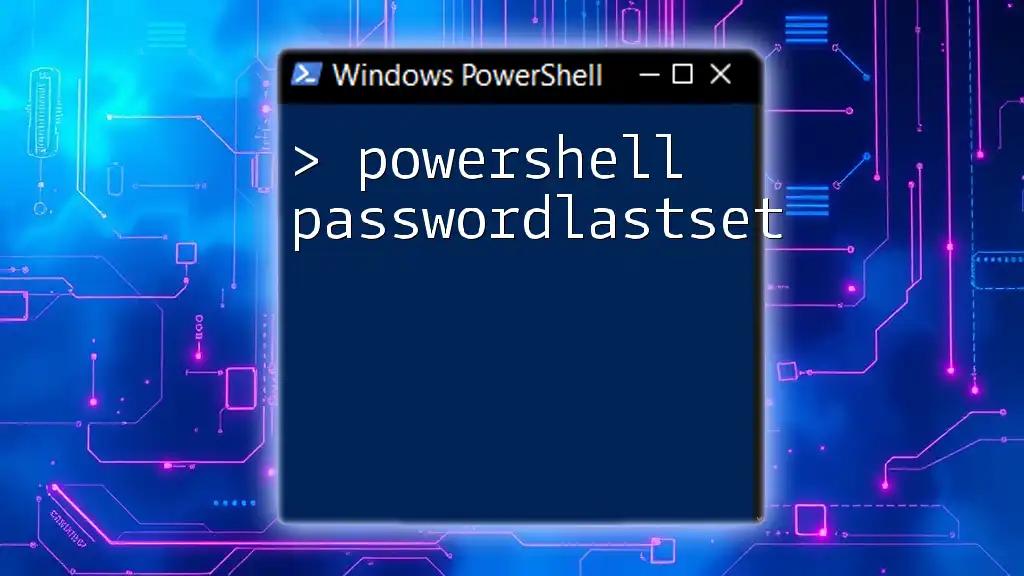
Advanced Usage of Dictionaries
Nested Dictionaries
Nested dictionaries, or hash tables containing other hash tables, allow for representing more complex data structures. This capability is particularly useful when you need to manage related data elegantly.
Example of Passing Nested Dictionaries
Here’s how you might create and utilize nested dictionaries:
$nestedDictionary = @{
"User1" = @{"Name"="Bob"; "Age"=25}
"User2" = @{"Name"="Charlie"; "Age"=35}
}
function Get-NestedUserInfo {
param (
[hashtable]$users
)
foreach ($user in $users.Keys) {
Write-Host "$user: Name - $($users[$user]['Name']), Age - $($users[$user]['Age'])"
}
}
Get-NestedUserInfo -users $nestedDictionary
This function iterates through each user in the nested hash table and prints out their name and age. The output will provide an organized view of data from multiple related entries:
User1: Name - Bob, Age - 25
User2: Name - Charlie, Age - 35
Handling Errors When Passing Dictionaries
Handling errors when passing dictionaries is vital to ensure your scripts execute without unexpected failures. Common issues include:
- Type Mismatches: If the function is designed for a hashtable but a different type is provided, PowerShell will throw an error.
- Missing Keys: Attempting to access non-existent keys can also cause runtime errors.
To resolve these issues, use validations within your functions. For example:
function Get-UserInfo {
param (
[hashtable]$userInfo
)
if (-not $userInfo.ContainsKey('Name')) {
Write-Host "Error: Name key is missing."
return
}
# Proceed with fetching values
Write-Host "Name: $($userInfo['Name'])"
}
This approach enhances your function's robustness, preventing execution errors related to missing data.
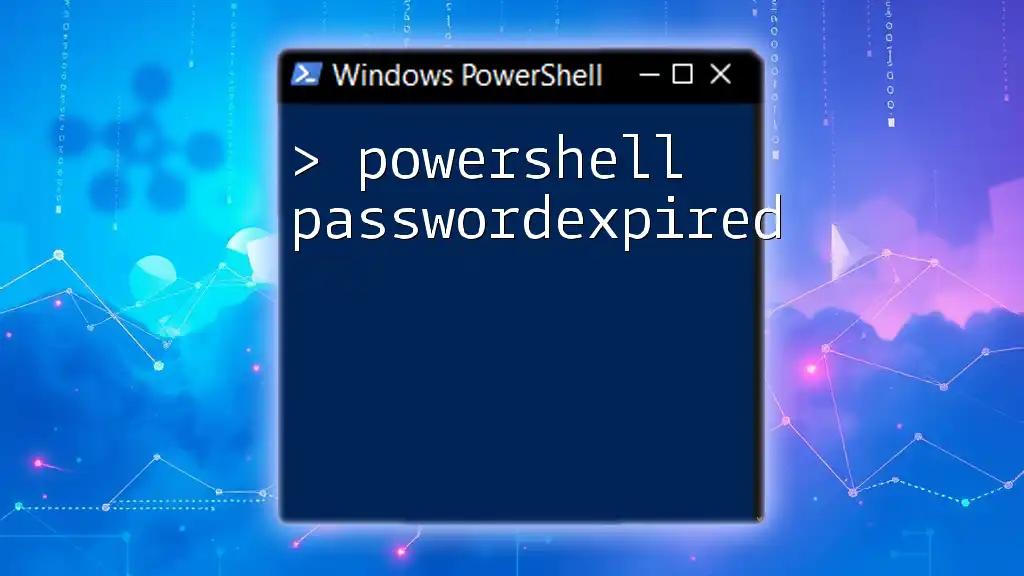
Conclusion
In summary, understanding how to pass a dictionary (hash table) as a parameter in PowerShell is instrumental for enhancing script efficiency and readability. With the ability to encapsulate multiple values in a single structure, dictionaries simplify passing complex data directly to functions.
I encourage you to experiment with passing dictionaries in your own PowerShell scripts. As you incorporate these techniques, you'll see the benefits of structuring your data more effectively and simplifying your code logic.
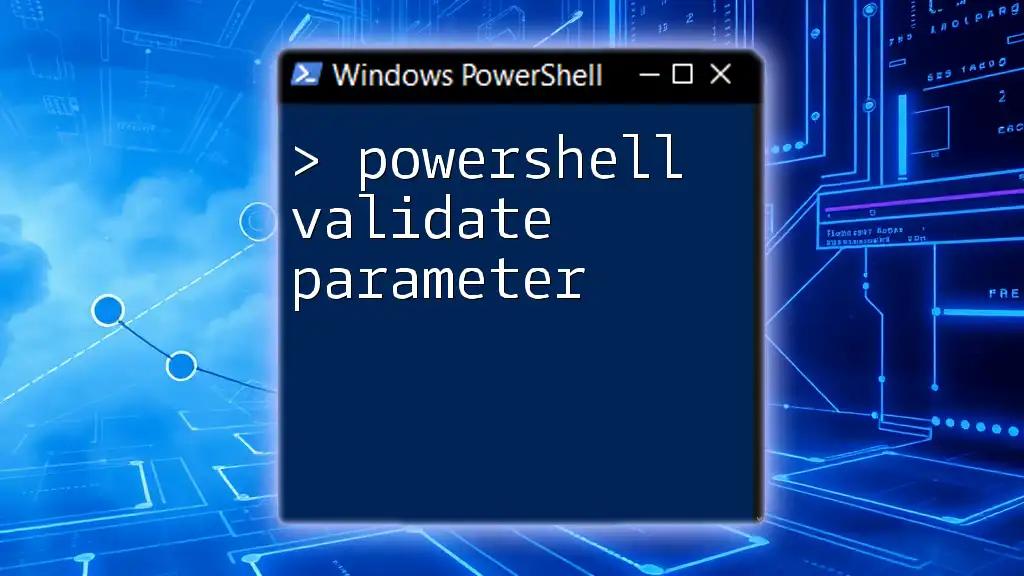
Additional Resources
To deepen your knowledge of PowerShell and understanding dictionaries, refer to the following resources:
- PowerShell Documentation: This is the official guide for everything related to PowerShell, providing comprehensive learning materials.
- Recommended Books and Courses: Explore various books and online courses that focus on PowerShell scripting and advanced concepts.
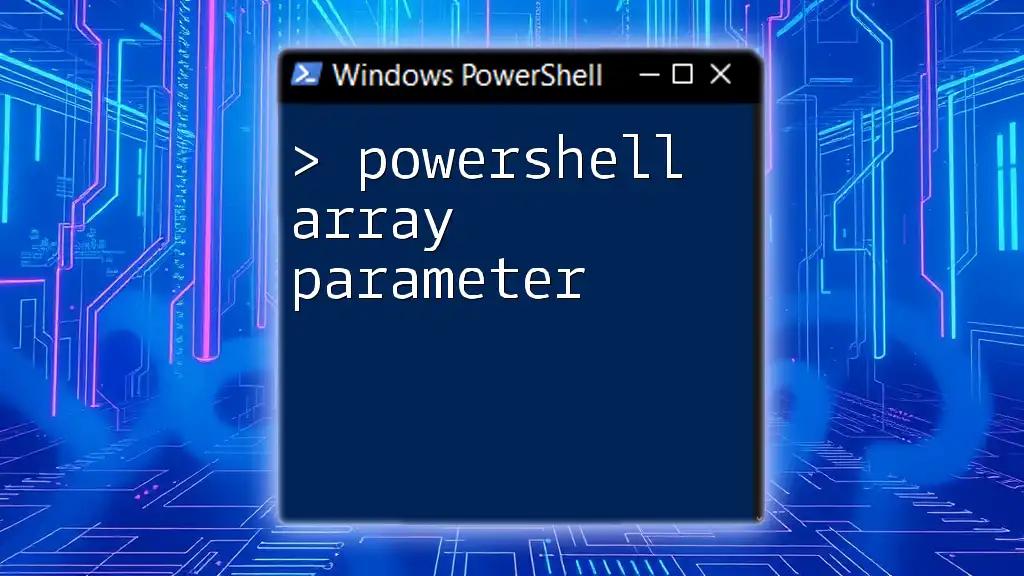
FAQs
What is the difference between a dictionary and an array in PowerShell?
Dictionaries are collections of key-value pairs allowing for non-sequential access using unique keys, while arrays are indexed collections that require positional access.
Can I pass a dictionary from one script to another? If yes, how?
Yes, you can pass dictionaries between scripts using script arguments or by employing the `dot-sourcing` technique to import variables.
How can I manipulate a dictionary after it’s passed as a parameter?
You can modify or retrieve elements within the hash table by referencing its keys, just like any other variable, thereby allowing for dynamic changes.