A PowerShell class constructor is a special method that initializes a new instance of a class, allowing you to set properties and perform any other setup operations when an object is created.
Here’s a simple example of a class constructor in PowerShell:
class Person {
[string]$Name
[int]$Age
Person([string]$name, [int]$age) {
$this.Name = $name
$this.Age = $age
}
}
$person = [Person]::new("Alice", 30)
Write-Host "Name: $($person.Name), Age: $($person.Age)"
Understanding PowerShell Classes
What is a Class?
In programming, a class is a blueprint for creating objects. A class defines properties (attributes) and methods (functions or behaviors) that the created objects will have. By encapsulating data and functionality, classes allow for a structured approach to coding, enabling the creation of reusable components.
Benefits of Using Classes in PowerShell
Utilizing classes in PowerShell brings various advantages:
- Code Reusability and Organization: Classes enable developers to encapsulate related properties and methods, promoting code reuse and organized structures in scripts.
- Enhanced Readability and Maintainability: Classes facilitate clearer code organization, making it easier for programmers to read and maintain scripts.
- Encapsulation of Functionalities: Classes help in grouping functionalities, which means that data and the functions that operate on that data are bundled together. This supports a cleaner separation of concerns.
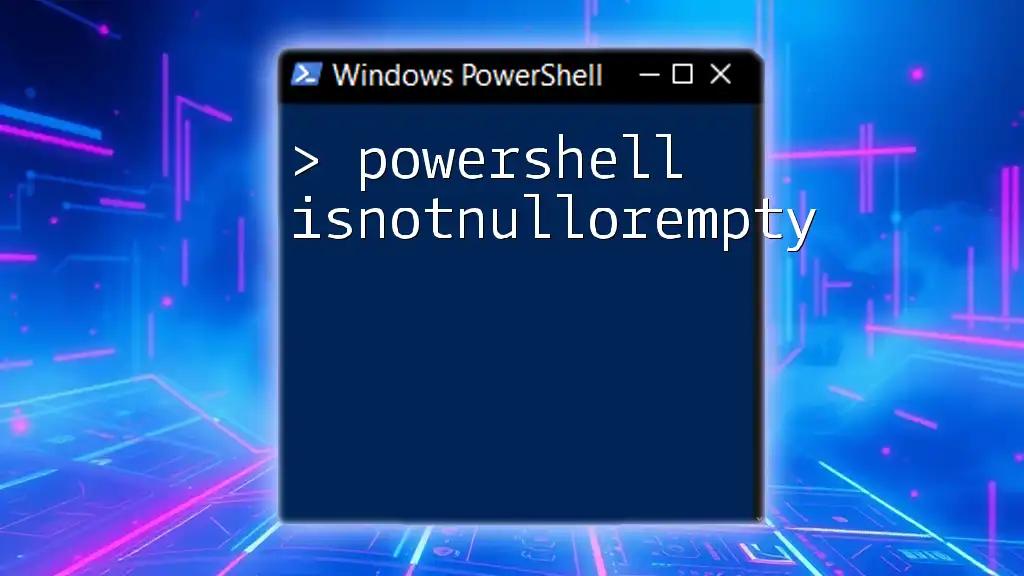
Class Constructors in PowerShell
What is a Constructor?
A constructor is a special method within a class that is invoked when a new instance (object) of the class is created. Constructors are pivotal because they initialize the properties of the class, ensuring that any object derived from the class starts its life in a valid state.
Role of the Constructor in Object Initialization
The constructor's primary role is to initialize the object’s properties. By setting default values and assigning parameters to properties, constructors ensure that every new object is correctly configured upon creation. This guarantees that any necessary setup is conducted right from the outset.
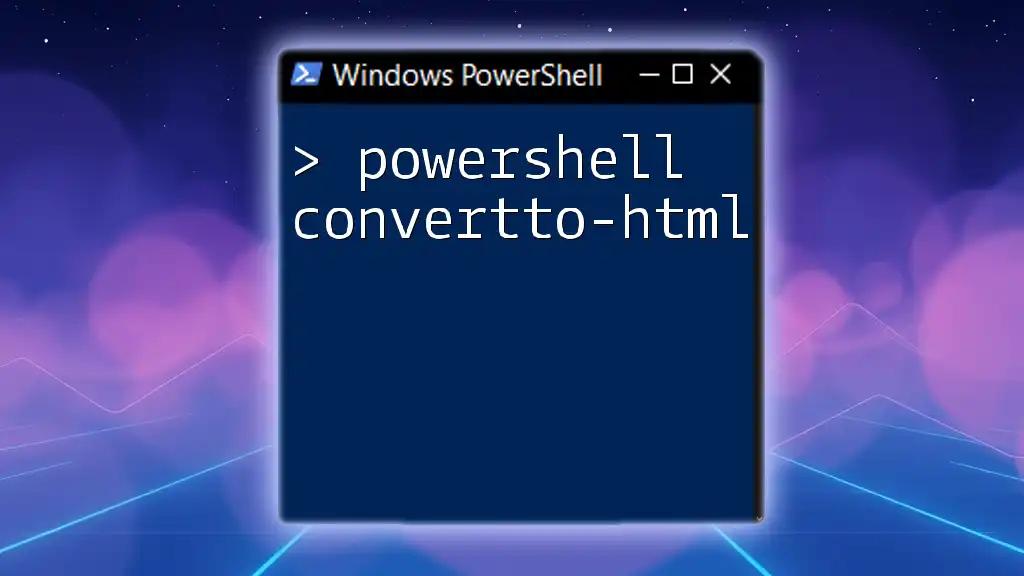
Creating a Class and Constructor in PowerShell
Defining a PowerShell Class
To define a class in PowerShell, utilize the following syntax:
class MyClass {
[string]$Property1
[int]$Property2
}
In this snippet, we declare a class named `MyClass` that contains two properties: `Property1` of type `string` and `Property2` of type `int`.
Adding a Constructor to the Class
To add a constructor to our class, define it within the class structure using the same name as the class:
class MyClass {
[string]$Property1
[int]$Property2
MyClass([string]$property1, [int]$property2) {
$this.Property1 = $property1
$this.Property2 = $property2
}
}
In this example, the constructor `MyClass` takes two parameters, which are used to initialize the object's properties.
Example of a PowerShell Class with a Constructor
Consider the following example of a class representing a car:
class Car {
[string]$Make
[string]$Model
Car([string]$make, [string]$model) {
$this.Make = $make
$this.Model = $model
}
[string] GetCarInfo() {
return "Make: $($this.Make), Model: $($this.Model)"
}
}
$myCar = [Car]::new("Toyota", "Corolla")
$myCar.GetCarInfo()
In this case, the `Car` class has properties for `Make` and `Model`. The constructor initializes these properties, and the method `GetCarInfo` returns a string with the car's details. The instantiation of the class using `$myCar = [Car]::new("Toyota", "Corolla")` creates a new `Car` object with designated properties.
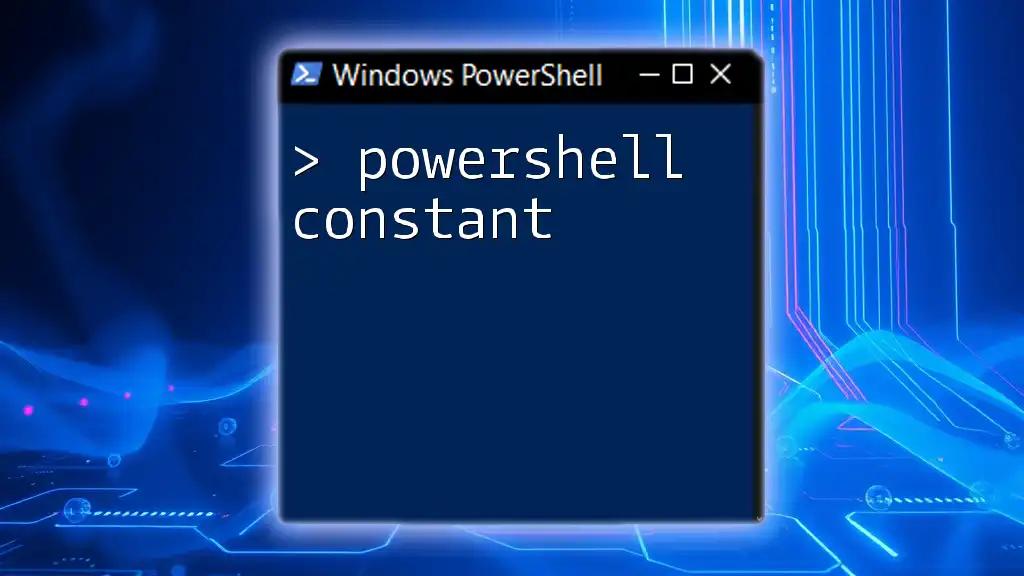
Using Constructors with Default Values
Implementing Default Parameters
Default parameters in constructors allow for greater flexibility when creating an object. If no arguments are supplied during instantiation, the constructor will apply predefined values.
class Person {
[string]$Name
[int]$Age
Person([string]$name = "Unknown", [int]$age = 0) {
$this.Name = $name
$this.Age = $age
}
}
$defaultPerson = [Person]::new()
In this example, if you don't provide a name or age when creating a new `Person`, it will default to `"Unknown"` for the name and `0` for the age, thus ensuring that all instances of `Person` start with meaningful default values.
Example of Using Default Constructor Behavior
When creating an object without providing specific values, such as `$defaultPerson = [Person]::new()`, the `Person` object will have default values assigned, showcasing the usefulness of default parameters in constructors where flexibility is required.
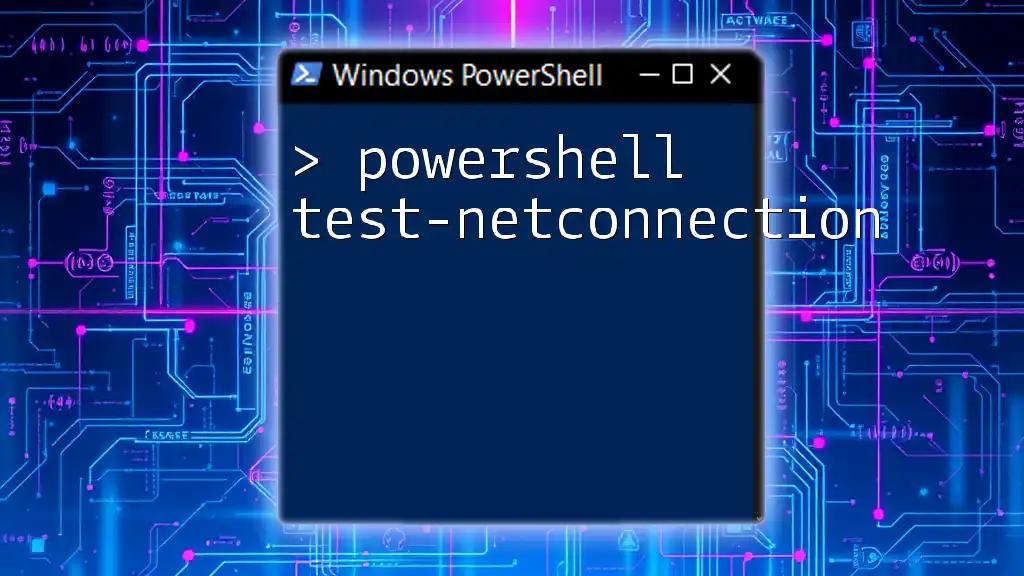
Understanding Constructor Overloading
What is Constructor Overloading?
Constructor overloading refers to the ability to have multiple constructors within the same class, each with a different set of parameters. This feature allows you to create objects in various ways, depending on the needs of the program.
Implementing Constructor Overloading in PowerShell
An example of constructor overloading can be illustrated as follows:
class Rectangle {
[int]$Length
[int]$Width
Rectangle([int]$length) {
$this.Length = $length
$this.Width = $length # Square case
}
Rectangle([int]$length, [int]$width) {
$this.Length = $length
$this.Width = $width # Rectangle case
}
}
This `Rectangle` class has two constructors: one that creates a square when only one dimension is provided, and another that takes both length and width for rectangles. This showcases how overloading can make class instantiation more versatile and aligned with various scenarios.
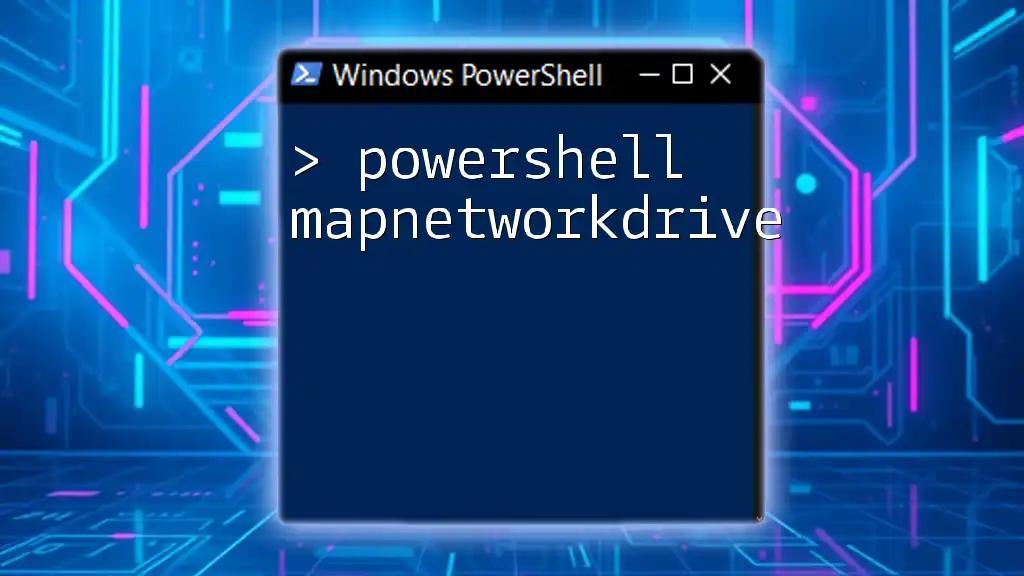
Common Use Cases of Class Constructors
Practical Applications in Scripting
Constructors are particularly useful in scenarios like automation and script creation, where structured data handling can lead to cleaner, more manageable scripts. For instance, when creating a deployment script, you could define a class with properties for server details and methods to carry out deployment operations.
Real-World Example with a Constructor
Imagine a user registration process where each user's details (like username and password) need to be encapsulated. You might create a class like this:
class User {
[string]$Username
[string]$Password
User([string]$username, [string]$password) {
$this.Username = $username
$this.Password = $password
}
}
In this example, the `User` class encapsulates details about a user securely, ensuring that all user objects are created with the essentials provided upon creation.
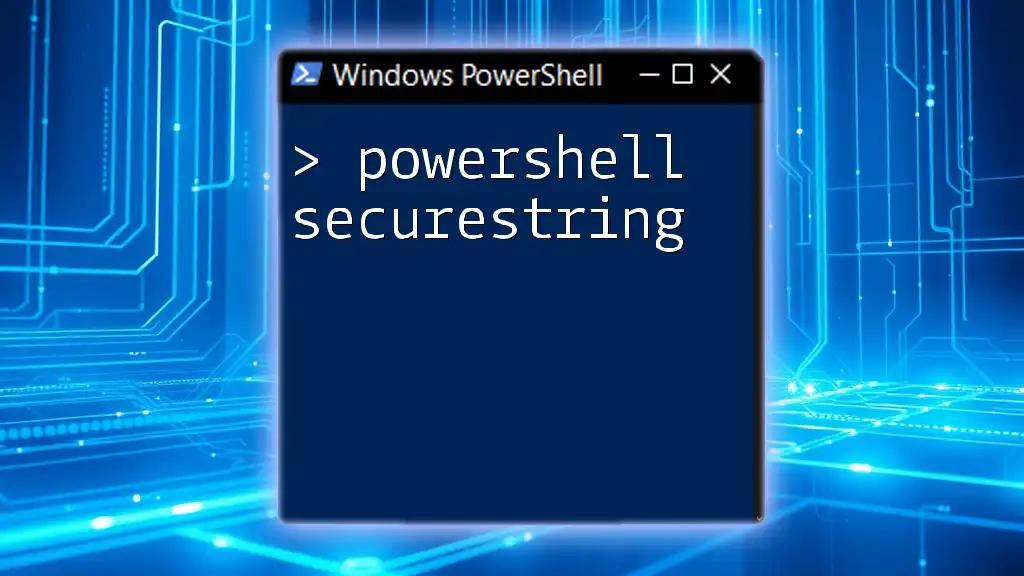
Best Practices for Using Constructors in PowerShell
Writing Readable and Maintainable Code
To maintain clarity in your code, employ clear and consistent naming conventions for your classes and properties. This added layer of organization allows anyone who reads your code (including future you) to easily understand its structure and functionality.
Performance Considerations
While using constructors is beneficial for organization and reuse, it's important to consider any potential performance impacts. Constructors may introduce slight overhead during object creation compared to standalone functions, but their advantages in code maintenance and clarity significantly outweigh these costs in most cases.
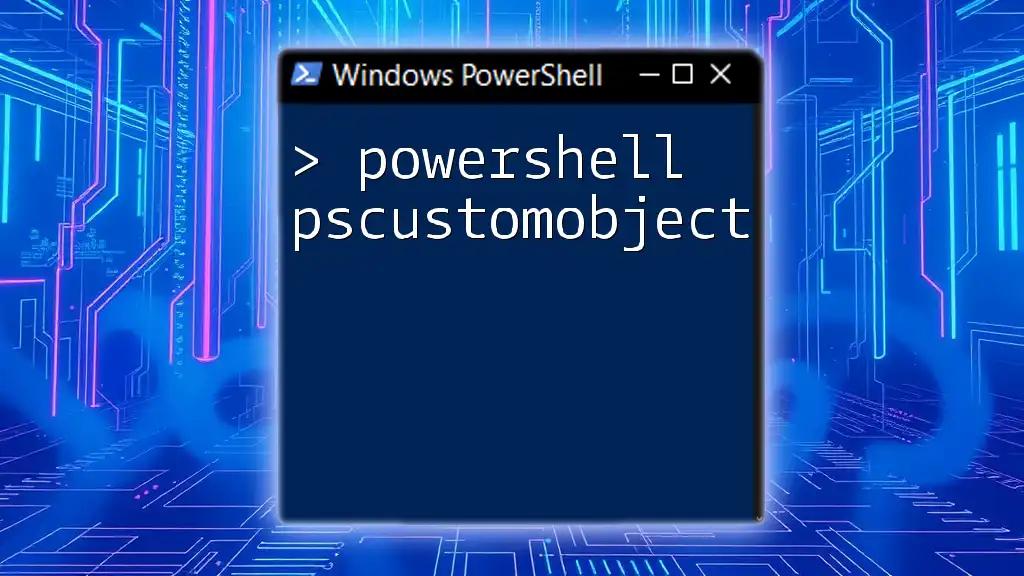
Conclusion
In summary, PowerShell class constructors play an essential role in object-oriented programming by ensuring that all instances of a class are initialized properly. By encapsulating properties and behaviors, constructors contribute significantly to the clarity, maintainability, and reusability of your scripts and applications. As you continue to explore PowerShell, consider experimenting with classes and their constructors to deepen your understanding of object-oriented concepts. Engage with the PowerShell community to share your experiences and glean insights from fellow developers as you progress on your scripting journey.
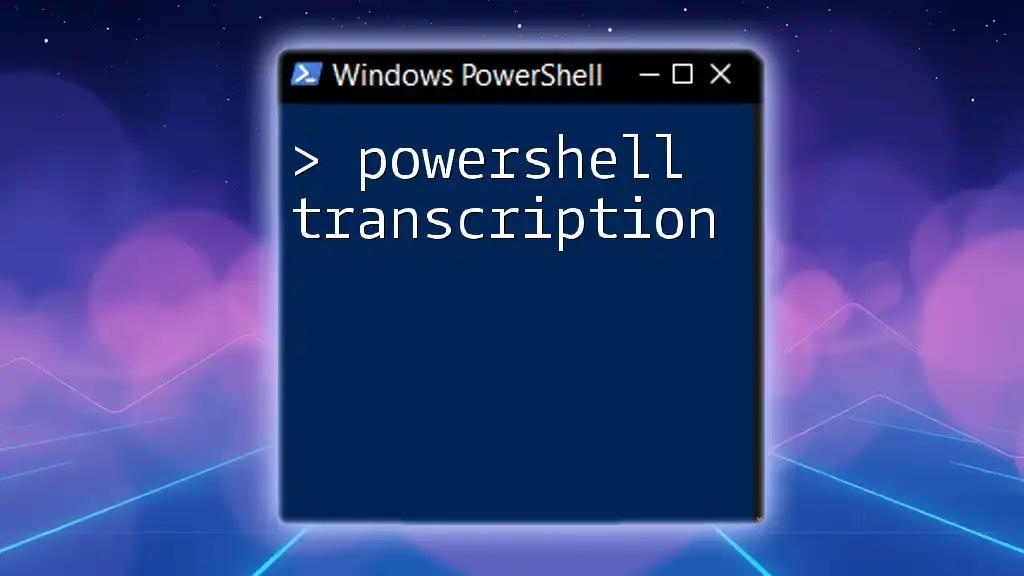
Additional Resources
For further learning, refer to PowerShell's official documentation, online courses, and communities where you can interact with experts and refine your skills.